


How to implement ViewPager multi-page sliding switching and animation effects in Android
The example of this article describes the method of Android programming to implement ViewPager multi-page sliding switching and animation effects. Share it with everyone for your reference, the details are as follows:
1. First, let’s take a look at the rendering, which is the Tab sliding effect of Sina Weibo. We can slide with gestures or click on the header above to switch. In the same way, the
white horizontal bar will move to the corresponding page card header. This is an animation effect, and the white bar slides past slowly. Okay, let's implement it next.
#2. Before we start, we must first get to know a control, ViewPager. It is a class in an additional package that comes with Google SDk and can be used to switch between screens.
This additional package is android-support-v4.jar, which will be provided to everyone in the final source code, in the libs folder. Of course, you can also search for the latest version yourself from the Internet.
After finding it, we need to add it to the project
3. Let’s do the interface first
The interface design is very simple, first There are three headers in one row, animated images in the second row, and page card content display in the third row.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:umadsdk="http://schemas.android.com/apk/res/com.LoveBus" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <LinearLayout android:id="@+id/linearLayout1" android:layout_width="fill_parent" android:layout_height="100.0dip" android:background="#FFFFFF" > <TextView android:id="@+id/text1" android:layout_width="fill_parent" android:layout_height="fill_parent" android:layout_weight="1.0" android:gravity="center" android:text="页卡1" android:textColor="#000000" android:textSize="22.0dip" /> <TextView android:id="@+id/text2" android:layout_width="fill_parent" android:layout_height="fill_parent" android:layout_weight="1.0" android:gravity="center" android:text="页卡2" android:textColor="#000000" android:textSize="22.0dip" /> <TextView android:id="@+id/text3" android:layout_width="fill_parent" android:layout_height="fill_parent" android:layout_weight="1.0" android:gravity="center" android:text="页卡3" android:textColor="#000000" android:textSize="22.0dip" /> </LinearLayout> <ImageView android:id="@+id/cursor" android:layout_width="fill_parent" android:layout_height="wrap_content" android:scaleType="matrix" android:src="@drawable/a" /> <android.support.v4.view.ViewPager android:id="@+id/vPager" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_weight="1.0" android:background="#000000" android:flipInterval="30" android:persistentDrawingCache="animation" /> </LinearLayout>
We want to display three page cards, so we also need the interface design of the content of the three page cards. Here we only set the background color, which can make a difference.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" android:background="#158684" > </LinearLayout>
4. Initialization work is required in the code part
(1) Let’s first define the variables
private ViewPager mPager;//页卡内容 private List<View> listViews; // Tab页面列表 private ImageView cursor;// 动画图片 private TextView t1, t2, t3;// 页卡头标 private int offset = 0;// 动画图片偏移量 private int currIndex = 0;// 当前页卡编号 private int bmpW;// 动画图片宽度
(2) Initialize the header
/** * 初始化头标 */ private void InitTextView() { t1 = (TextView) findViewById(R.id.text1); t2 = (TextView) findViewById(R.id.text2); t3 = (TextView) findViewById(R.id.text3); t1.setOnClickListener(new MyOnClickListener(0)); t2.setOnClickListener(new MyOnClickListener(1)); t3.setOnClickListener(new MyOnClickListener(2)); } /** * 头标点击监听 */ public class MyOnClickListener implements View.OnClickListener { private int index = 0; public MyOnClickListener(int i) { index = i; } @Override public void onClick(View v) { mPager.setCurrentItem(index); } };
I believe everyone will have no problem after reading it. Click on which page the content of the card will be displayed.
(3) Initialize the page card content area
/** * 初始化ViewPager */ private void InitViewPager() { mPager = (ViewPager) findViewById(R.id.vPager); listViews = new ArrayList<View>(); LayoutInflater mInflater = getLayoutInflater(); listViews.add(mInflater.inflate(R.layout.lay1, null)); listViews.add(mInflater.inflate(R.layout.lay2, null)); listViews.add(mInflater.inflate(R.layout.lay3, null)); mPager.setAdapter(new MyPagerAdapter(listViews)); mPager.setCurrentItem(0); mPager.setOnPageChangeListener(new MyOnPageChangeListener()); }
We will load three page card interfaces into it, and the first page card will be displayed by default. Here we also need to implement an adapter.
/*** ViewPager Adapter
*/
public class MyPagerAdapter extends PagerAdapter {
public List
public MyPagerAdapter(List
this.mListViews = mListViews;
}
@Override
public void destroyItem(View arg0, int arg1, Object arg2) {
((ViewPager) arg0).removeView(mListViews.get(arg1));
}
@Override
public void finishUpdate(View arg0) {
}
@Override
public int getCount() {
return mListViews.size();
}
@Override
public Object instantiateItem(View arg0, int arg1) {
((ViewPager) arg0).addView(mListViews.get(arg1), 0);
return mListViews.get( arg1);
}
@Override
public boolean isViewFromObject(View arg0, Object arg1) {
return arg0 == (arg1);
}
@Override
public void restoreState(Parcelable arg0, ClassLoader arg1) {
}
@Override
public Parcelable saveState() {
return null;
}
@Override
public void startUpdate( View arg0) {
}
}
Here we implement the loading and unloading of each page card
(3) Initialization animation
/** * 初始化动画 */ private void InitImageView() { cursor = (ImageView) findViewById(R.id.cursor); bmpW = BitmapFactory.decodeResource(getResources(), R.drawable.a) .getWidth();// 获取图片宽度 DisplayMetrics dm = new DisplayMetrics(); getWindowManager().getDefaultDisplay().getMetrics(dm); int screenW = dm.widthPixels;// 获取分辨率宽度 offset = (screenW / 3 - bmpW) / 2;// 计算偏移量 Matrix matrix = new Matrix(); matrix.postTranslate(offset, 0); cursor.setImageMatrix(matrix);// 设置动画初始位置 }
According to the screen resolution Calculate the offset of animation movement with the width of the picture
Realize page card switching monitoring
/** * 页卡切换监听 */ public class MyOnPageChangeListener implements OnPageChangeListener { int one = offset * 2 + bmpW;// 页卡1 -> 页卡2 偏移量 int two = one * 2;// 页卡1 -> 页卡3 偏移量 @Override public void onPageSelected(int arg0) { Animation animation = null; switch (arg0) { case 0: if (currIndex == 1) { animation = new TranslateAnimation(one, 0, 0, 0); } else if (currIndex == 2) { animation = new TranslateAnimation(two, 0, 0, 0); } break; case 1: if (currIndex == 0) { animation = new TranslateAnimation(offset, one, 0, 0); } else if (currIndex == 2) { animation = new TranslateAnimation(two, one, 0, 0); } break; case 2: if (currIndex == 0) { animation = new TranslateAnimation(offset, two, 0, 0); } else if (currIndex == 1) { animation = new TranslateAnimation(one, two, 0, 0); } break; } currIndex = arg0; animation.setFillAfter(true);// True:图片停在动画结束位置 animation.setDuration(300); cursor.startAnimation(animation); } @Override public void onPageScrolled(int arg0, float arg1, int arg2) { } @Override public void onPageScrollStateChanged(int arg0) { } }
5. After finishing the work, come and take a look at your own The fruits of your labor
#I hope this article will be helpful to everyone in Android programming.
For more related articles on how Android implements ViewPager multi-page sliding switching and animation effects, please pay attention to the PHP Chinese website!
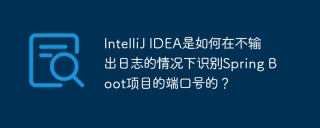
Start Spring using IntelliJIDEAUltimate version...
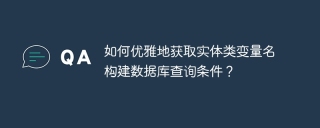
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
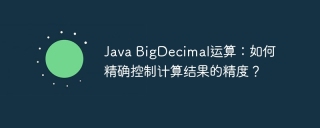
Java...
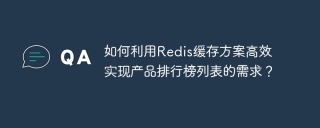
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
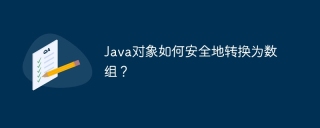
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
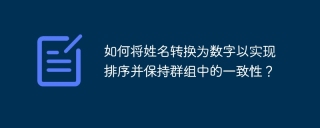
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
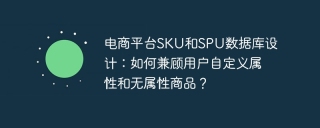
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
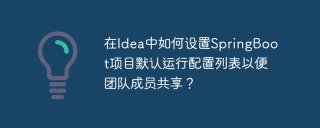
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use
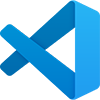
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software