7. The use of special reference "$this"
Now we know how to access the members in the object, which is accessed through the "Object->Members" method, which is outside the object
part to access the members in the object, then if I want to let the method in the object access the properties of this object inside the object, or
is the method in the object to call other methods of this object, then how do we manage? Because all members in the object must be called using objects, including calls between internal members of the object, PHP provides me with a reference to this object $this,
each object There is an object reference $this to represent the object and complete the call of the internal members of the object. The original meaning of this is
"this". In the above example, we instantiate three instance objects $P1, $ P2, $P3, each of these three objects
has its own $this, which represents the objects $p1, $p2, and $p3 respectively.
We can see from the above figure that $this is the reference that represents the object inside the object. The method used to call the
members of this object inside the object and the members of the object outside the object is the same.
$this->属性$this->name; $this->age; $this->sex; $this->方法$this->say(); $this->run();Modify the above example so that everyone can tell their name, gender and age:
<?php class Person { //下面是人的成员属性 var $name; //人的名字 var $sex; //人的性别 var $age; //人的年龄 //下面是人的成员方法 function say() //这个人可以说话的方法 { echo "我的名字叫:".$this->name." 性别:".$this->sex." 我的年龄是: ".$this->age."<br>"; }f unction run() //这个人可以走路的方法 { echo "这个人在走路"; } } $p1=new Person(); //创建实例对象$p1 $p2=new Person(); //创建实例对象$p2 $p3=new Person(); //创建实例对象$p3 //下面三行是给$p1对象属性赋值 $p1->name="张三"; $p1->sex="男"; $p1->age=20; //下面访问$p1对象中的说话方法 $p1->say(); //下面三行是给$p2对象属性赋值 $p2->name="李四"; $p2->sex="女"; $p2->age=30; //下面访问$p2对象中的说话方法 $p2->say(); //下面三行是给$p3对象属性赋值 $p3->name="王五"; $p3->sex="男"; $p3->age=40; //下面访问$p3对象中的说话方法 $p3->say(); ?>The output result is:
我的名字叫:张三性别:男我的年龄是:20 我的名字叫:李四性别:女我的年龄是:30 我的名字叫:王五性别:男我的年龄是:40Analyze this method:
function say() //这个人可以说话的方法 { echo "我的名字叫:".$this->name." 性别:".$this->sex." 我的年龄是: ".$this->age."<br>"; }There is a say() method in the three objects $p1, $p2 and $p3. $this represents these three objects respectively. Call the corresponding
attribute and print out the value of the attribute. This is the way to access the object's properties inside the object. If you call
the say() method, you can also use the run() method. In say () This method uses $this->run() to complete the call.
8. Constructor and destructor methods
Most classes have a special method called a constructor. When an object is created, it will automatically call the constructor, that is, the constructor will be automatically called when the new keyword is used to instantiate the object.
The declaration of the constructor is the same as the declaration of other operations, except that its name must be __construct(). This is a change in PHP5. In
previous versions, the name of the constructor must be the same as the class name. This can still be used in PHP5, but now few
people use it. Do this The advantage is that the constructor can be made independent of the class name. When the class name changes, there is no need to change the corresponding constructor
name. For backward compatibility, if there is no method named __construct() in a class, PHP will search for a constructor method written in php4 with the same name as the class name.
Format:
function __construct ( [参数] ) { ... ... }
Only one constructor can be declared in a class, but the constructor will only be called once every time an object is created.
This method cannot be called actively. So it is usually used to perform some useful initialization tasks. For example, the paired properties are assigned initial values when creating the object.
<? //创建一个人类 class Person { //下面是人的成员属性 var $name; //人的名字 var $sex; //人的性别 var $age; //人的年龄 //定义一个构造方法参数为姓名$name、性别$sex和年龄$age function __construct($name, $sex, $age) { //通过构造方法传进来的$name给成员属性$this->name赋初使值 $this->name=$name; //通过构造方法传进来的$sex给成员属性$this->sex赋初使值 $this->sex=$sex; //通过构造方法传进来的$age给成员属性$this->age赋初使值 $this->age=$age; }/ /这个人的说话方法 function say() { echo "我的名字叫:".$this->name." 性别:".$this->sex." 我的年龄是: ".$this->age."<br>"; } } //通过构造方法创建3个对象$p1、p2、$p3,分别传入三个不同的实参为姓名、性别和年龄 $p1=new Person(“张三”,”男”, 20); $p2=new Person(“李四”,”女”, 30); $p3=new Person(“王五”,”男”, 40); //下面访问$p1对象中的说话方法 $p1->say(); //下面访问$p2对象中的说话方法 $p2->say(); //下面访问$p3对象中的说话方法 $p3->say(); ?>
The output result is:
我的名字叫:张三性别:男我的年龄是:20 我的名字叫:李四性别:女我的年龄是:30 我的名字叫:王五性别:男我的年龄是:40
Destructor:
The opposite of the constructor is the destructor. The destructor is a newly added content of PHP5. There is no destructor in PHP4.
The destructor will delete all references to an object or Executed when the object is explicitly destroyed, that is, the destructor is called before the object
is destroyed in memory. Similar to the name of the constructor, the name of a class's destructor must be __destruct(). Destruction
The function cannot take any parameters.
Format:
function __destruct ( ) { ... ... }
<? //创建一个人类 class Person { //下面是人的成员属性 var $name; //人的名字 var $sex; //人的性别 var $age; //人的年龄 //定义一个构造方法参数为姓名$name、性别$sex和年龄$age function __construct($name, $sex, $age) { //通过构造方法传进来的$name给成员属性$this->name赋初使值 $this->name=$name; //通过构造方法传进来的$sex给成员属性$this->sex赋初使值 $this->sex=$sex; //通过构造方法传进来的$age给成员属性$this->age赋初使值 $this->age=$age; } //这个人的说话方法 function say() { echo "我的名字叫:".$this->name." 性别:".$this->sex." 我的年龄是: ".$this->age."<br>"; } //这是一个析构函数,在对象销毁前调用 function __destruct() { echo “再见”.$this->name.”<br>”; } //通过构造方法创建3个对象$p1、p2、$p3,分别传入三个不同的实参为姓名、性别和年龄 $p1=new Person(“张三”,”男”, 20); $p2=new Person(“李四”,”女”, 30); $p3=new Person(“王五”,”男”, 40); //下面访问$p1对象中的说话方法 $p1->say(); //下面访问$p2对象中的说话方法 $p2->say(); //下面访问$p3对象中的说话方法 $p3->say(); ?>
The output result is:
我的名字叫:张三性别:男我的年龄是:20 我的名字叫:李四性别:女我的年龄是:30 我的名字叫:王五性别:男我的年龄是:40 再见张三 再见李四 再见王五The above is the content of PHP object-oriented tutorial 4. For more related content, please pay attention to the PHP Chinese website (www.php. cn)!
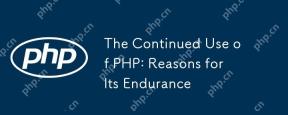
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
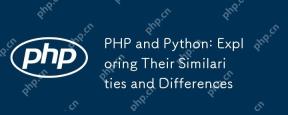
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
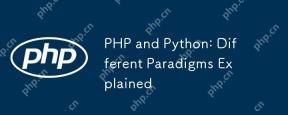
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
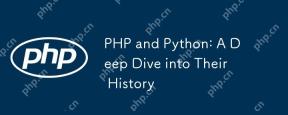
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
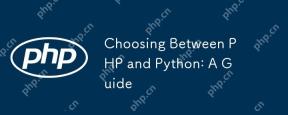
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
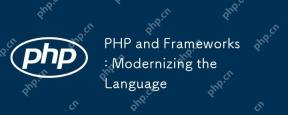
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
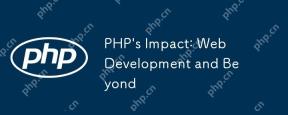
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
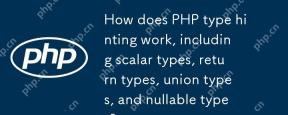
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
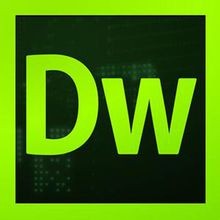
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool