Process control refers to the way to control the direction of the program while it is running. It is mainly divided into the following categories:
Sequential structure
Sequential structure, as the name suggests, means that the program is executed sequentially from top to bottom. There is no judgment or jump in the middle.
Branch structure
Java provides two branch structures: if and switch. The if statement uses Boolean expressions or Boolean values to judge the branch control, while the switch statement uses integers, String types, and enumeration types. .
if statement
if statement uses Boolean expressions or Boolean values to judge and control branches. There are mainly three structures:
if statement
if (condition) {
//Statement
}
Execution trend
Example:
int score = 65;if(score >= 60){
System.out.PRintln("You passed");
}
if…else statement
if (condition) {
Example:
int score = 65 ;if(score >= 60){
System.out.println("You passed, congratulations");
System.out.println("Failed, cleaning toilets for three months");
} //statement } else if (condition) {
//statement } else {
//statement
}
Example:
Evaluation of students’ final exam scores
Score>=60: Moderate
Score
int score = 70; //Examination score if ( score >= 90 ) {
System.out.println("Excellent");} else if (score >= 80 ) {
System.out. println("Good");} else if (score >= 60 ) {
System.out.println("Medium");} else {
System.out.println("Poor");}
contains another if statement in the if statement
If (condition 1) { if (condition 2) { if (condition 3) {
}else{
}
}………
}
Normally, the statement blocks of these types of judgment statements are wrapped in {} brackets, but if the statement has only one sentence, you don’t need to use curly braces, for example
boolean b = true;if( b)
System.out.println("You can not use curly braces"); else
System.out.println("It is recommended to put curly braces");
Break;
}
case "Passed":
System.out.println("Performing well"); break;
case "Failed":
break;
default:System.out.println("Didn't come for the exam? Call your parents");
break;}
int i = 1;switch(i){
case 1:
System.out.println("Won the first place");
break;
case 2:
System.out.println("Won the second place" );
break; case 3:
System.out.println("Get third place");
break;
default:
System.out.println("You are not the top three");
break;
}
Pay attention to the break statement when using it. If you miss it, hey!
Comparison of switch and multiple if
Same points:
Both can implement multi-branch structures
Differences:
Switch: can only handle conditional judgments of equal values, and the conditions are equal to integer variables or character variables Value judgment
Multiple if: The processing also includes other if structures in the else part, which is especially suitable for situations when a certain variable is in a certain range
Loop structure
Loop statements are also called iteration statements. Loop statements can satisfy Under the condition of conditions, repeatedly execute a certain code. The cycle statement includes:
while cycle
while (cycle condition) {// meet the conditions and continue to execute the cycle; Operation}
i++;
}
do-while loopdo {
Loop operation //Perform the loop operation first} while (loop condition); //If the conditions are met, the loop continues to execute; otherwise, Loop exit
System.out.println("Unlucky, need to clean the toilet");
} while(i
loop operation;
}
}
Control loop structurebreak statementin a certain Sometimes, we need to forcefully terminate the loop when a certain condition occurs, and we can use break to complete this function. For example:
System.out.println("Complete a circle") ;
}
In the above example, 10 laps should have been completed, but when reaching the 8th lap, I couldn’t hold on anymore and broke, and the rest will not be executed.
System.out.println("Complete one Lap");
}
He was supposed to run 10 laps, but he actually only ran 9 laps.
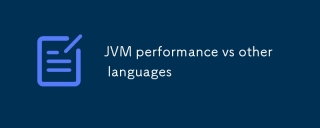
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
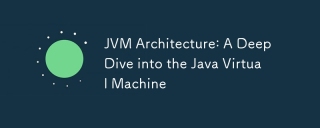
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
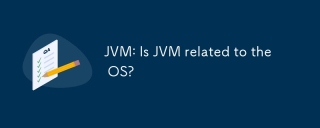
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
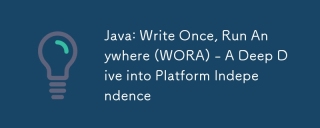
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
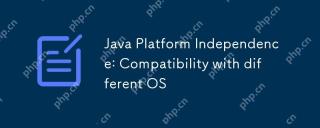
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
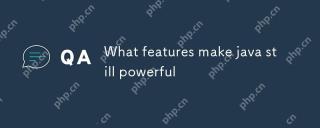
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
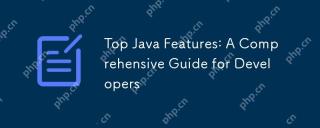
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
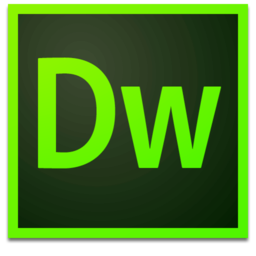
Dreamweaver Mac version
Visual web development tools
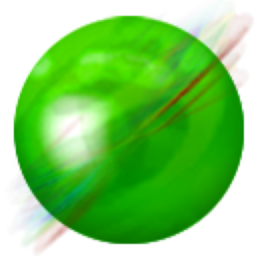
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
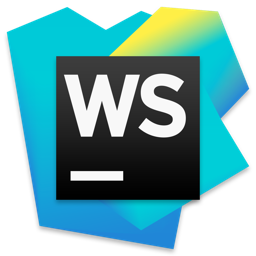
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
