The life cycle of a function is divided into creation and activation phases (when called), let us study it in detail.
Function Creation
As we all know, function declaration is put into variable/activity (VO/AO) object when entering context. Let's look at the variable and function declarations in the global context (here the variable object is the global object itself, we remember that, right?)
var x = 10; function foo() { var y = 20; alert(x + y); } foo(); // 30
On function activation, we get the correct (expected) result - 30 . However, there is one very important feature.
Previously, we only talked about variable objects related to the current context. Here, we see that the variable "y" is defined in the function "foo" (meaning it is in the AO of the foo context), but the variable "x" is not defined in the "foo" context, and accordingly, it does not Added to the AO of "foo". At first glance, the variable "x" does not exist at all relative to the function "foo"; but as we see below - and only at a "glance", we find that the active object of the "foo" context only contains one Attribute - "y".
fooContext.AO = { y: undefined // undefined – 进入上下文的时候是20 – at activation };
How does function "foo" access variable "x"? In theory, the function should be able to access the variable object of a higher-level context. In fact, it is exactly like this. This mechanism is implemented through the [[scope]] attribute inside the function.
[[scope]] is the hierarchical chain of all parent variable objects, which is above the current function context and is stored in it when the function is created.
Note this important point - [[scope]] is stored when the function is created - static (immutable), forever and ever, until the function is destroyed. That is: the function can never be called, but the [[scope]] attribute has been written and stored in the function object.
Another thing to consider is that - compared with the scope chain, [[scope]] is an attribute of the function rather than the context. Considering the above example, the [[scope]] of function "foo" is as follows:
foo.[[Scope]] = [ globalContext.VO // === Global ];
For example, we use the usual ECMAScript arrays to represent the scope and [[scope]].
Continuing, we know that the context is entered when the function is called. At this time, the active object is created, and this and the scope (scope chain) are determined. Let us consider this moment in detail.
Function activation
As mentioned in the definition, after entering the context to create AO/VO, the Scope attribute of the context (a scope chain for variable search) is defined as follows:
Scope = AO|VO + [[Scope]]
The meaning of the above code is: active object Is the first object in the scope array, that is, the front end added to the scope.
Scope = [AO].concat([[Scope]]);
This feature is very important for the processing of identifier parsing. Identifier resolution is the process of determining which variable object a variable (or function declaration) belongs to.
In the return value of this algorithm, we always have a reference type, its base component is the corresponding variable object (or null if not found), and the attribute name component is the name of the identifier looked up. Details of reference types are discussed later.
The identifier resolution process includes the lookup of the attributes corresponding to the variable names, that is, the continuous lookup of the variable objects in the scope, starting from the deepest context, bypassing the scope chain to the top level.
In this way, in upward search, local variables in a context have higher priority than variables in the parent scope. In case two variables have the same name but come from different scopes, the first one found is in the deepest scope.
We use a slightly more complicated example to describe what we have mentioned above.
var x = 10; function foo() { var y = 20; function bar() { var z = 30; alert(x + y + z); } bar(); } foo(); // 60
For this, we have the following variables/activities, the [[scope]] attribute of the function and the scope chain of the context:
The variable object of the global context is:
globalContext.VO === Global = { x: 10 foo: <reference to function> };
When "foo" is created, The [[scope]] attribute of "foo" is:
foo.[[Scope]] = [ globalContext.VO ];
When "foo" is activated (enters the context), the active object of the "foo" context is:
fooContext.AO = { y: 20, bar: <reference to function> };
The scope chain of the "foo" context is:
fooContext.Scope = fooContext.AO + foo.[[Scope]] // i.e.: fooContext.Scope = [ fooContext.AO, globalContext.VO ];
When the internal function "bar" is created, its [[scope]] is:
bar.[[Scope]] = [ fooContext.AO, globalContext.VO ];
When "bar" is activated, the active object of the "bar" context is:
barContext.AO = { z: 30 };
The scope chain of the "bar" context is:
barContext.Scope = barContext.AO + bar.[[Scope]] // i.e.: barContext.Scope = [ barContext.AO, fooContext.AO, globalContext.VO ];
The identifiers of "x", "y", and "z" are analyzed as follows:
- "x" -- barContext.AO // not found -- fooContext.AO // not found -- globalContext.VO // found - 10 - "y" -- barContext.AO // not found -- fooContext.AO // found - 20 - "z" -- barContext.AO // found - 30
The above is the second part of the JavaScript scope chain: the content of the life cycle of the function. For more related content, please pay attention to the PHP Chinese website (www .php.cn)!
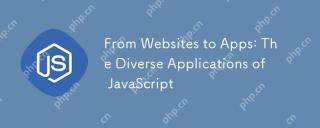
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
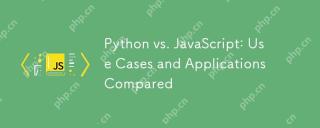
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
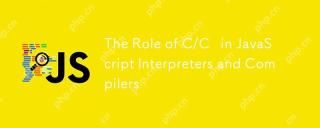
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
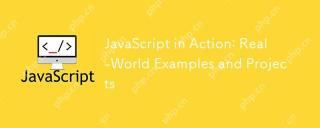
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
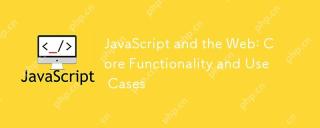
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
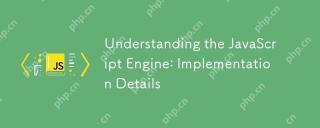
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
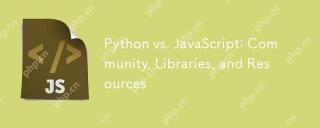
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
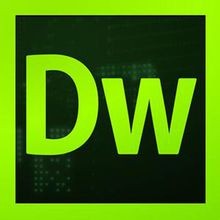
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
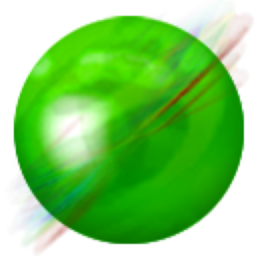
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment