First of all, I would like to thank bigeagle for his help. This is also made with reference to her bbs.
The message board is divided into three modules: list message list, display detailed content, and post message
notepage.cs
namespace
notpage
{
using System;
using System.Data.SQL;
using System.Data
;
using System.Collections ;
//////////////////////////////////////////////////// ////////////////////
//
//
Class Name: Message Board
//
// Description:
Construct a message board object
//
// date: 2000/06/06
//
// Author: God
///
///////////////////////////////////////////////////// //////////////
///
private string n_strAuthor; //Lecturer
private string
n_strContent; //Message content
//Attributes
public int ID
{
{
return n_intID
;
}
set
{
n_intID = value;
}
}
public
string Title
{
get
{
return n_strTitle
;
}
set
{
n_strTitle = value;
}
}
public
string Author
{
get
{
return n_strAuthor
;
}
set
{
n_strAuthor = value ;
}
}
public string
Content
{
get
{
return n_strContent
;
}
set
{
n_strContent = value ;
}
}
public DateTime
adddate
{
get
{
return n_dateTime;
}
set
{
n_dateTime =
value;
}
//Constructor
public notepage()
{
//
// TODO: Add
Constructor Logic here
//
this.n_intID = 0 ;
this.n_strTitle = ""
;
this.n_strAuthor = "" ;
this.n_strContent = "" ;
this.n_dateTime =
System.DateTime.Now;
}
///
///
/// Get the content of the message
///
///
///
public notepage GetTopic(int a_intID)
{
// TODO:
Add Constructor Logic here
//
//Read database
myconn myConn = new
myconn();
SQLCommand myCommand = new SQLCommand() ;
myCommand.ActiveConnection =
myConn ;
myCommand.CommandText = "n_GetTopicInfo" ; //Call the stored procedure
myCommand.CommandType =
CommandType.StoredProcedure;
myCommand.Parameters["@a_intTopicID"].Value = a_intID ;
notepage objNp = new notepage();
try
{
myConn.Open() ;
SQLDataReader myReader ;
myCommand.Execute(out
myReader) ;
if (myReader.Read())
{
objNp.ID = (int)myReader["ID"]
;
objNp.Title = (string)myReader["Title"] ;
objNp.Author =
(string)myReader["Author"] ;
objNp.adddate =
(DateTime)myReader["adddate"];
}
//Clear up
myReader.Close();
myConn.Close() ;
}
catch(Exception e)
{
throw(new Exception("Failed to fetch post:" + e.ToString()))
;
}
return objNp;
}
///
///
/// Purpose: to store the content of the message into the database
///
/// Use constructors to pass information
///
///
///
{
//
// TODO: Add Constructor Logic here
//
//Read database
myconn myConn = new myconn();
SQLCommand myCommand = new SQLCommand() ;
myCommand.ActiveConnection =
myConn ;
myCommand.CommandText = "n_addTopic" ;
//调用存储过程
myCommand.CommandType = CommandType.StoredProcedure
;
myCommand.Parameters.Add(new SQLParameter("@a_strTitle" ,
SQLDataType.VarChar,100)) ;
myCommand.Parameters["@a_strTitle"].Value =
n_Topic.Title ;
myCommand.Parameters.Add(new
SQLParameter("@a_strAuthor" , SQLDataType.VarChar,50))
;
myCommand.Parameters["@a_strAuthor"].Value = n_Topic.Author
;
myCommand.Parameters.Add(new SQLParameter("@a_strContent" ,
SQLDataType.VarChar,2000)) ;
myCommand.Parameters["@a_strContent"].Value =
n_Topic.Content ;
try
{
myConn.Open() ;
myCommand.ExecuteNonQuery() ;
//清场
myConn.Close() ;
}
catch(Exception e)
{
throw(new
Exception("取贴子失败:" + e.ToString())) ;
}
return true;
}
///
///
取的贴子列表
///
///
/// 返回一个Topic数组
///
public ArrayList
GetTopicList()
{
//定义一个forum数组做为返回值
ArrayList arrForumList =new
ArrayList() ;
//从数据库中读取留言列表
myconn myConn = new
myconn();
SQLCommand myCommand = new SQLCommand()
;
myCommand.ActiveConnection = myConn ;
myCommand.CommandText =
"n_GetTopicList" ; //调用存储过程
myCommand.CommandType =
CommandType.StoredProcedure ;
try
{
myConn.Open()
;
SQLDataReader myReader ;
myCommand.Execute(out myReader)
;
for (int i = 0 ; myReader.Read() ; i++)
{
notepage
objItem = new notepage() ;
objItem.ID = myReader["ID"].ToString().ToInt32()
;
objItem.Title = myReader["Title"].ToString() ;
objItem.Author =
myReader["Author"].ToString() ;
objItem.adddate =
myReader["adddate"].ToString().ToDateTime();
objItem.Content =
myReader["Content"].ToString();
arrForumList.Add(objItem) ;
}
//清场
myReader.Close();
myConn.Close() ;
}
catch(SQLException e)
{
throw(new Exception("数据库出错:" + e.ToString()))
;
//return null ;
}
return arrForumList ;
}
}
}
以上就是利用c#制作简单的留言板(1)的内容,更多相关文章请关注PHP中文网(www.php.cn)!
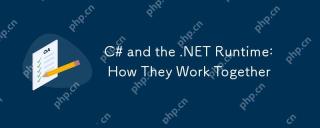
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
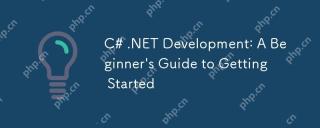
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
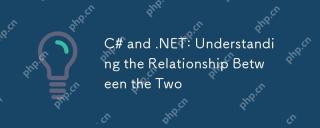
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
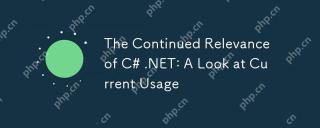
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
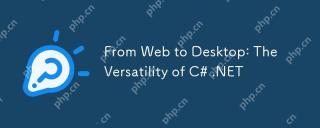
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
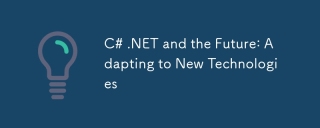
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
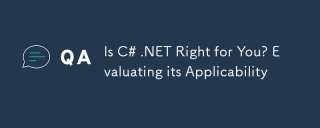
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
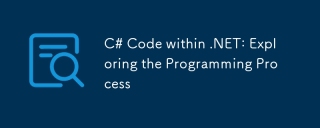
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
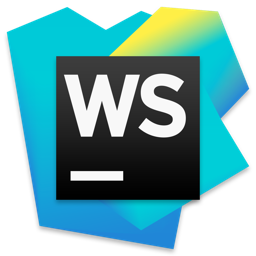
WebStorm Mac version
Useful JavaScript development tools
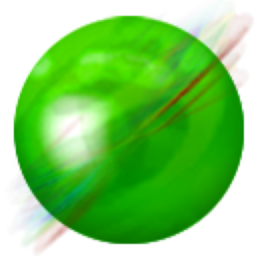
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.