


Foreword
The goto statement is also called an unconditional transfer statement. Its basic form is as follows:
The statement label consists of a valid identifier and the symbol ";", where the naming rules of the identifier are the same as the variable name, that is, It consists of letters, numbers and underscores, and the first character must be a letter or underscore. After executing the goto statement, the program will jump to the statement label and execute the subsequent statements.
Usually goto statements are used in conjunction with if conditional statements. However, while the goto statement brings flexibility to the program, it also makes the program structure unclear and difficult to read, so it must be used rationally.
Problem found
We often encounter the problem that variables are defined after goto and the compilation fails under Linux (error message: crosses initialization of). In fact, you just need to pay attention. Today, after asking the seniors in the company, I also looked through some information and recorded it to deepen my memory. I hope it can be of some help to some people.
Error sample code:
#include <iostream> using namespace std; int main() { goto Exit; int a = 0; Exit: return 0; }
Error report:
[root@localhost c-c++]# g++ goto_study.cpp goto_study.cpp: In function 'int main()': goto_study.cpp:31: error: jump to label 'Exit' goto_study.cpp:29: error: from here goto_study.cpp:30: error: crosses initialization of 'int a'
Correct way of writing
cannot be said to be correct, it can only be said to be a way of compiling OK.
Go directly to the code:
Writing method 1:
Change the domain and become a local variable:
int main() { goto Exit; { int a = 0; } Exit: return 0; }
Writing method 2
Magical writing method:
int main() { goto Exit; int a; a = 1; Exit: cout << "a = " << a << endl; return 0; }
The key is that it can still be accessed! Result:
[root@localhost c-c++]# g++ goto_study.cpp [root@localhost c-c++]# ./a.out a = 1259648
Research
Magical writing method
After seeing two writing methods that can be compiled and passed, the most puzzling thing is that the second writing method can be compiled and passed, and can it still be used? ? ?
C++ regulations
Reference [1][2] mentioned the regulations in the C++ standard: > It is possible to transfer into a block, but not in a way that bypasses declarations with initialization. A program that jumps from a point where a local variable with automatic storage duration is not in scope to a point where it is in scope is ill-formed unless the variable has POD type (3.9) and is declared without an initializer.
It means: if the execution path of a program jumps from point A in the code (a local variable x has not been defined) to another point B in the code (the local variable x has been defined and initialized when defined), then the compiler will report an error. Such a jump can be caused by executing a goto statement or a switch-case. Therefore, in the second way of writing, a is of int type, a POD type, and has not been initialized, so the compilation passes. However, it is obvious: if you use this variable a, the result is unknown. As the predecessor said, it is meaningless, it is better not to support it! If it is only used locally, it can be enclosed in curly braces! Some people on the Internet also said that although the C++ specification does not explicitly state that this is wrong, the provisions of the variable domain actually implicitly say that this approach is not advisable, see reference [4].
Implicit explanation
Goto can't skip over definitions of variables, because those variables would
not exist after the jump, since lifetime of variable starts at the point of
definition. The specification does not seem to explicitly mention goto must not
do that, but it is implied in what is said about variable lifetime.
-fpermissive flag
Reference [4] mentioned that the g++ compiler checks by default, you can set this flag of the compiler to become Warning, not implemented! ! !
After checking the information, the function of the fpermissive mark is to treat syntax errors in the code as a warning and continue the compilation process, so for the sake of safety, don’t think about it from this perspective, just code it!
POD type
Refer to [3]. According to the above C++ regulations, as long as it is a POD type and is not initialized, it can be compiled and passed. : Look at a paragraph of code:
#include <iostream> using namespace std; class A{ public: // 注意:和B不同的是有构造和析构函数, 所以编译报错 A(){} ~A(){} void testA(){ cout << "A::test." << endl; } }; class B{ public: void testB(){ cout << "B::test." << endl; } }; int main() { goto Exit; // int a = 1; // windows ok.linux failed! //A classA; // failed: B classB; // success: classB.testB(); Exit: classB.testB(); return 0; }Result:
[root@localhost c-c++]# g++ goto_study.cpp [root@localhost c-c++]# ./a.out a = 1259648 B::test.E
Summary:
1. The above code is compiled and executed in Windows and Linux; Compilation fails! Because A has a constructor and a destructor, it does not meet the conditions;
3. As for int a = 1; this way of writing can be passed under windows (msvc), but it is inconsistent with the C++ specification. Please explain! ! !
1. int, char, wchar_t, bool, float, double are POD types, these types are long/short and
The same is true for signed/unsigned versions;
2. Pointers (including function pointers and member pointers) are all POD types;
2. Do not skip definition and initialization of variables after goto. If it is a POD type, you can declare it first and then define it, and no compilation error will be reported. However, it is not recommended to use it this way. You can see that if the execution statement skips the assignment statement, the value of the variable is unknown and dangerous;
3. If there is a local variable after goto, it can be enclosed in curly braces to form a Local domain is safe.
The above is the entire content of this article. I hope the content of this article can be of some help to everyone's study or work. If you have any questions, you can leave a message to communicate.
For more related articles, please pay attention to the PHP Chinese website (www.php.cn)!
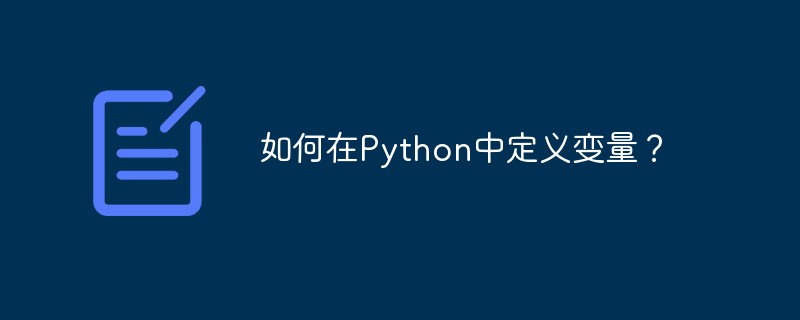
在Python中,变量可以理解为存储数据的容器。当我们需要使用或操作数据时,可以通过定义变量来存储数据,从而方便地调用和处理这些数据。下面将介绍Python中定义变量的方法。一、命名规则在Python中,变量的命名规则非常灵活,通常需要遵循以下规则:变量名由字母、下划线和数字组成,首位不能为数字。变量名可以使用大小写字母,但Python是区分大小写的。变量名
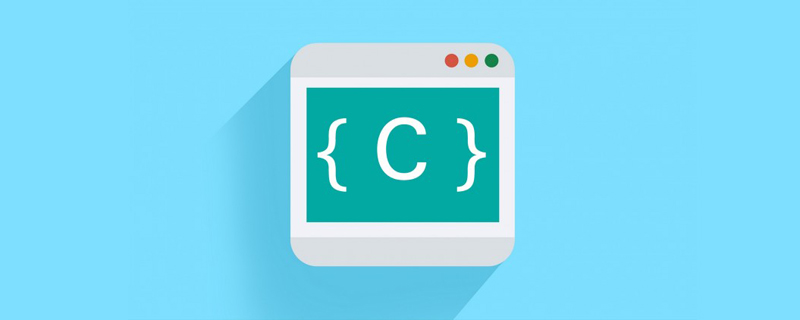
在c语言中,goto语句被称为无条件转移语句,允许把控制无条件转移到同一函数内的被标记的语句;语法“goto label;...label: statement;”,其中label可以是任何除C关键字以外的纯文本,它可以设置在C程序中goto语句的前面或者后面。
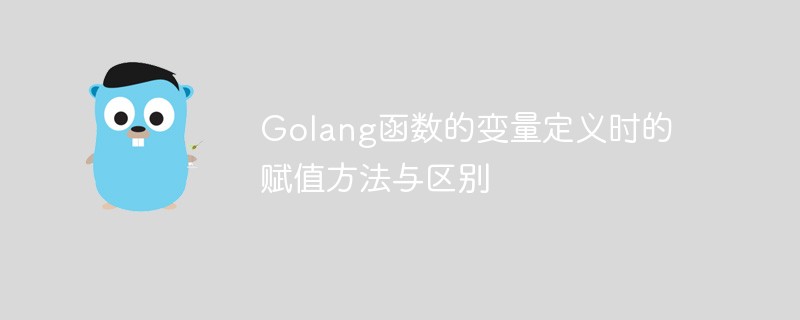
Golang是一种快速、高效、现代化的编程语言,它在编译时会自动检查类型,并且具有并发性和内存安全性等特点,因此被越来越多的开发者所青睐。在Golang中,我们经常需要使用函数来封装业务逻辑,而函数中的变量定义时的赋值方法是一个常见的问题,本文将详细讲解这个问题并分析其中的区别。变量定义在Golang中,可以使用var和:=两种方式来定义变量。其中,var方
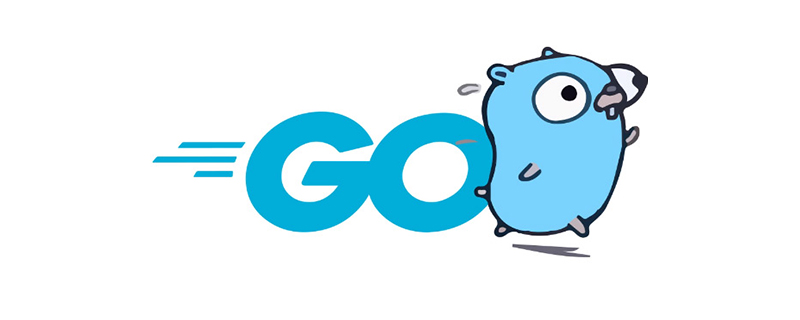
在go语言中,goto语句用于无条件跳转,可以无条件地转移到程序中指定的行;它通过标签进行代码间的无条件跳转。goto后接一个标签,这个标签的意义是告诉Go程序下一步要执行哪行的代码,语法“goto 标签;... ...标签: 表达式;”。goto打破原有代码执行顺序,直接跳转到指定行执行代码;goto语句通常与条件语句配合使用,可用来实现条件转移、构成循环、跳出循环体等功能。
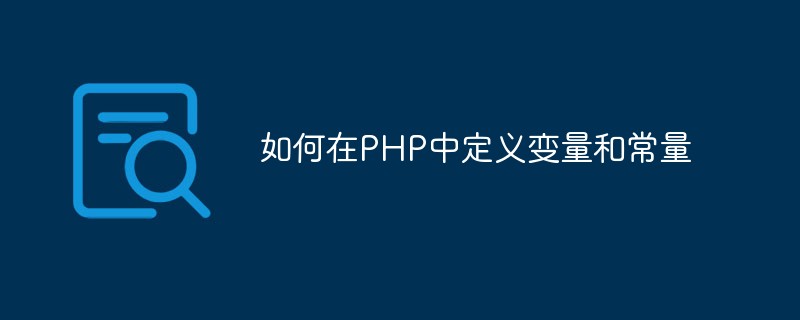
PHP是一种被广泛使用的编程语言,具有卓越的可扩展性和实用性。在PHP中,变量和常量是两种十分重要的概念,它们可以用来存储和表示值以及存储重要的信息。在这篇文章中,我们将会详细介绍如何在PHP中定义变量和常量,以帮助初学者快速上手。一、定义变量变量是用于存储值的名字或标识符。在PHP中,变量的定义可以分为三个步骤:变量的声明、变量的赋值和使用变量。下面我们详
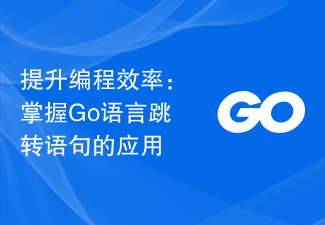
提升编程效率:掌握Go语言跳转语句的应用在Go语言编程中,跳转语句是一种常用的控制语句,能够帮助我们实现代码逻辑的跳转和控制,提高编程效率。掌握跳转语句的使用可以让我们更灵活地处理各种复杂的逻辑,减少代码冗余,提高代码可读性和执行效率。本文将介绍Go语言中常用的跳转语句,并通过具体的代码示例来说明它们的应用。1.break语句break语句用于跳出当前循环
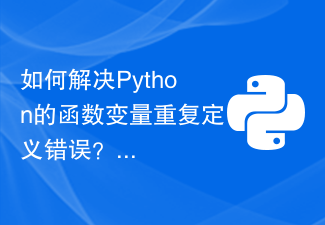
Python的函数变量重复定义错误是一个常见问题,当一个函数中重复定义了相同名称的变量时,Python会抛出“localvariable'xxxx'redefined”错误。这个错误通常是由于函数内部的变量名和外部的变量名重复导致的。在Python中,变量作用域分为局部作用域和全局作用域,当在一个函数中定义变量时,该变量默认为局部变量,并且只能在该函数
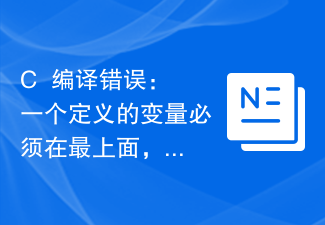
在C++编程中,有时会遇到一个常见的错误,即“一个定义的变量必须在最上面”的错误。这通常是由于变量定义的位置不正确导致的。在本文中,我们将讨论如何修复这个错误。在C++中,变量的定义通常需要在函数体或作用域的开始处进行。如果你定义的变量放在下面,而在调用之前,则会出现“一个定义的变量必须在最上面”的编译错误。出现这个错误的解决方案就是将变量定义移到函数或作用


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
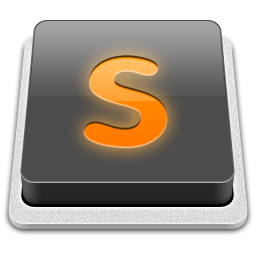
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment
