jQuery.data() is used to attach (and obtain) data to ordinary objects or DOM Elements.
The following will analyze its implementation in three parts:
1. Use name and value to append data to the object; that is, pass in three parameters, the first parameter is the object that needs to be appended with data, and the second parameter is the name of the data, and the third parameter is the value of the data. Of course, if you just get the value, you don't need to pass in the third parameter.
2. Use another object to append data to the object; that is, pass in two parameters, the first parameter is the data object that needs to be appended (we call it "obj"), and the second parameter is also an object (we Call it "another"); the key-value pairs contained in "another" will be copied to the data cache of "obj" (we call it "cache").
3. Attach data to DOM Element; DOM Element is also a kind of Object, but IE6 and IE7 have problems with garbage collection of objects directly attached to DOM Element; therefore we store these data in the global cache (we call it ("globalCache"), that is, "globalCache" contains the "cache" of multiple DOM Elements, and adds an attribute on the DOM Element to store the uid corresponding to the "cache".
Use name and value to append data to objects
When using jQuery.data() to append data to ordinary objects, the essence is to attach a "cache" to the object and use a special attribute name.
The "cache" that stores data is also an object. The data we attach to "obj" actually becomes the attribute of "cache". And "cache" is an attribute of "obj". In jQuery 1.6, the name of this attribute is "jQuery16" plus a random number (such as "jQuery16018518865841457738" mentioned below).
We can use the following code to test the functionality of jQuery.data():
<script type="text/javascript" src="jqueryjs"></script> <script> obj = {}; $data(obj, 'name', 'value'); documentwrite("$data(obj, 'name') = " + $data(obj, 'name') + '<br />'); for (var key in obj) { documentwrite("obj" + key + 'name = ' + obj[key]name); } </script>
The displayed result is:
$.data(obj, 'name') = value obj.jQuery16018518865841457738.name = value
In this code, we first append on "obj" An attribute (name is "name", value is "value"), and then the attached data is obtained through $.data(obj, 'name'). In order to gain a deeper understanding of the implementation mechanism, we have used a loop to obtain the properties of "obj", which actually removes the "cache" object attached to "obj".
You can see that jQuery.data() actually attaches "obj" to an object named "jQuery16018518865841457738" (the name is random), which is "cache". The attributes attached to the object using jquery.data() actually become attributes of this "cache".
We can use the following code to achieve a similar function:
$ = function() { var expando = "jQuery" + ("6" + Mathrandom())replace(/\D/g, ''); function getData(cache, name) { return cache[name]; } function setData(cache, name, value) { cache[name] = value; } function getCache(obj) { obj[expando] = obj[expando] || {}; return obj[expando]; } return { data : function(obj, name, value) { var cache = getCache(obj); if (value === undefined) { return getData(cache, name); } else { setData(cache, name, value); } } } }();
The first line of code in
function defines "expando", which is "jQuery1.6" plus a random number (0.xxxx), And remove the non-numeric part; this format will be used in other places in jQuery and will not be discussed here; you only need to know that this is a special name and can be used to identify different pages (such as in different iframes) "expando" will make a difference).
Next, the function getData() for obtaining data is defined, which is to obtain an attribute from "cache"; in fact, it returns cache[name].
Then there is the setData() function, which is used to set the attribute of "cache"; in fact, it is to set the value of cache[name].
is followed by getCache() to get the "cache" on "obj", that is, obj[expando]; if obj[expando] is empty, initialize it.
Finally, the data method is exposed. First, according to the incoming "obj", get the "cache" attached to "obj"; when two parameters are passed in, the getData() method is called; when three parameters are passed in , the setData() method is called.
Use another object to append data to the object
In addition to assigning values by providing name and value, we can also directly pass in another object ("another") as a parameter. In this case, the attribute name and attribute value of "another" will be treated as multiple key-value pairs, and the "name" and "value" extracted from them will be copied to the cache of the target object.
The functional test code is as follows:
<script type="text/javascript" src="jqueryjs"></script> <script> obj = {}; $data(obj, {name1: 'value1', name2: 'value2'}); documentwrite("$data(obj, 'name1') = " + $data(obj, 'name1') + '<br />' ); documentwrite("$data(obj, 'name2') = " + $data(obj, 'name2') + '<br />'); for (var key in obj) { documentwrite("obj" + key + 'name1 = ' + obj[key]name1 + '<br />'); documentwrite("obj" + key + 'name2 = ' + obj[key]name2); } </script>
The displayed result is as follows:
$.data(obj, 'name1') = value1 $.data(obj, 'name2') = value2 obj.jQuery1600233050178663064.name1 = value1 obj.jQuery1600233050178663064.name2 = value2
In the above test code, we first pass in an "another" object with two key-value pairs, Then use $.data(obj, 'name1') and $.data(obj, 'name2') to obtain additional data; similarly, in order to deeply understand the mechanism, we took out the hidden data by traversing "obj" "cache" object, and obtained the values of the "name1" attribute and "name2" attribute of the "cache" object.
You can see that jQuery.data() actually attaches an object named "obj.jQuery1600233050178663064" to "obj", which is "cache". Key-value pairs passed in using jquery.data() are copied to "cache".
We can use the following code to achieve similar functions:
$ = function() { // Other codes function setDataWithObject(cache, another) { for (var name in another) { cache[name] = another[name]; } } // Other codes return { data : function(obj, name, value) { var cache = getCache(obj); if (name instanceof Object) { setDataWithObject(cache, name) } else if (value === undefined) { return getData(cache, name); } else { setData(cache, name, value); } } } }();
这段代码是在之前的代码的基础上进行修改的。首先增加了内部函数 setDataWithObject() ,这个函数的实现是遍历 “another” 的属性,并复制到 “cache” 中。
然后,在对外开放的 data 函数中,先判断传入的第二个参数的名称,如果这个参数是一个 Object 类型的实例,则调用 setDataWithObject() 方法。
为 DOM Element 附加数据
由于 DOM Element 也是一种 Object,因此之前的方式也可以为 DOM Element 赋值;但考虑到 IE6、IE7 中垃圾回收的问题(不能有效回收 DOM Element 上附加的对象引用),jQuery采用了与普通对象有所不同的方式附加数据。
测试代码如下:
<div id="div_test" /> <script type="text/javascript" src="datajs"></script> <script> windowonload = function() { div = documentgetElementById('div_test'); $data(div, 'name', 'value'); documentwrite($data(div, 'name')); } </script>
显示结果如下:
value
测试代码中,首先通过 document.getElementById 方法获取了一个 DOM Element (当然,也可以用 jQuery 的选择器),然后在这个 DOM Element 上附加了一个属性,随后就从 DOM Element 上取出了附加的属性并输出。
因为考虑到 IE6、IE7 对 DOM Element 上的对象引用的垃圾回收存在问题,我们不会直接在 DOM Element 上附加对象;而是使用全局cache,并在 DOM Element 上附加一个 uid。
实现方式如下:
$ = function() { var expando = "jQuery" + ("6" + Mathrandom())replace(/\D/g, ''); var globalCache = {}; var uuid = 0; // Other codes function getCache(obj) { if (objnodeType) { var id = obj[expando] = obj[expando] || ++uuid; globalCache[id] = globalCache[id] || {}; return globalCache[id]; } else { obj[expando] = obj[expando] || {}; return obj[expando]; } } // Other codes }();
这段代码与之前的代码相比,增加了 globalCache 和 uuid,并修改了 getCache() 方法。
globalCache 对象用于存放附加到 DOM Element 上的 “cache”,可以视为 “cache” 的“容器”。uuid 表示 “cache” 对应的唯一标识,是唯一且自增长的。uuid 或被存放在 DOM Element 的 “expando” 属性中。
getCache() 函数中增加了一个判断,即 “obj” 具有 “nodeType” 属性,就认为这是一个 DOM Element;这种情况下,就先取出附加在 “obj” 上的 id ,即 obj[expando] ;如果 obj[expando] 未定义,则先用 ++uuid 对其进行初始化;取出 id 之后,就到 globalCache 中找到对应的 “cache” ,即 globalCache[id], 并返回。
到此为止,jQuery.data() 函数的实现就介绍完了;但是,这里还有一个需要思考的问题:为什不都统一用 “globalCache” 存储,而要将 “cache” 直接附加到普通对象上?我认为这应该是一种性能优化的方式,毕竟少一个引用的层次,存取速度应该会略快一些。 jQuery 中这刻意优化的地方非常多,在许多原本可以统一处理的对方都进行了特殊处理。但这在一定程度上,也造成了阅读源码的障碍。当然这是作者(及其他代码贡献者)本身的编程哲学,这里就不加评论了。
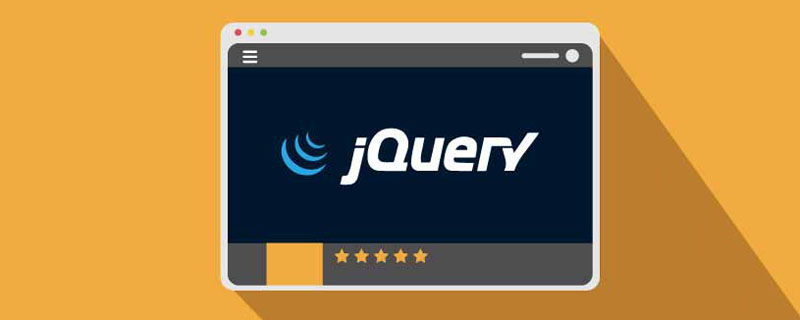
实现方法:1、用“$("img").delay(毫秒数).fadeOut()”语句,delay()设置延迟秒数;2、用“setTimeout(function(){ $("img").hide(); },毫秒值);”语句,通过定时器来延迟。
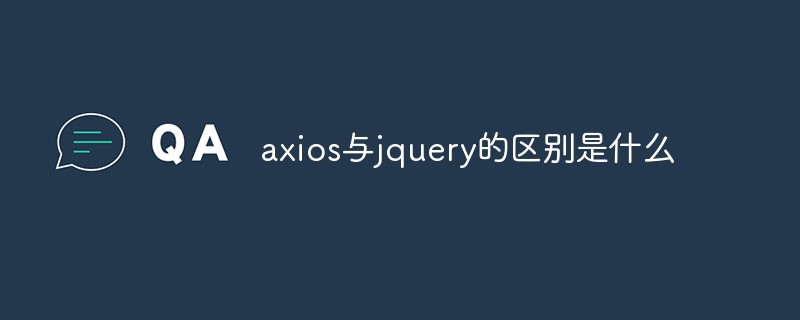
区别:1、axios是一个异步请求框架,用于封装底层的XMLHttpRequest,而jquery是一个JavaScript库,只是顺便封装了dom操作;2、axios是基于承诺对象的,可以用承诺对象中的方法,而jquery不基于承诺对象。
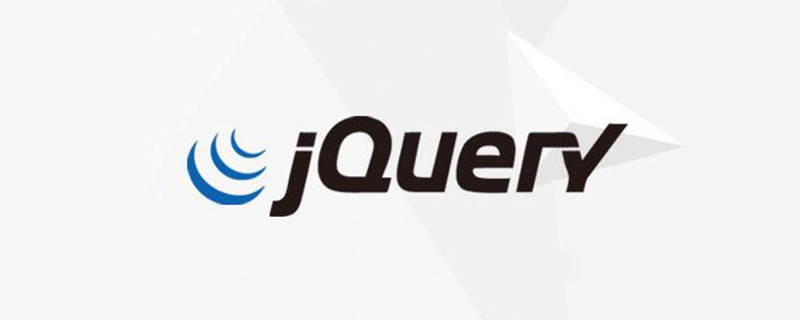
修改方法:1、用css()设置新样式,语法“$(元素).css("min-height","新值")”;2、用attr(),通过设置style属性来添加新样式,语法“$(元素).attr("style","min-height:新值")”。
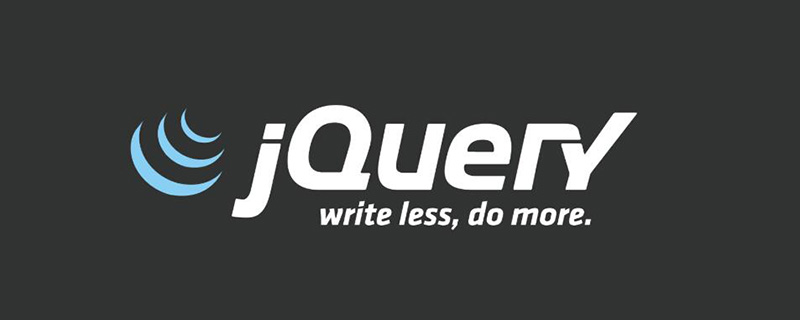
增加元素的方法:1、用append(),语法“$("body").append(新元素)”,可向body内部的末尾处增加元素;2、用prepend(),语法“$("body").prepend(新元素)”,可向body内部的开始处增加元素。
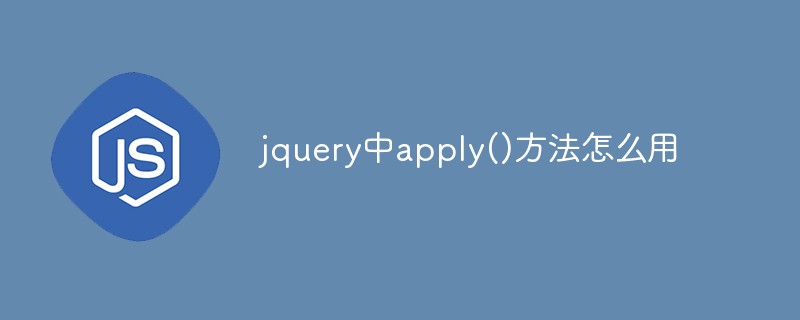
在jquery中,apply()方法用于改变this指向,使用另一个对象替换当前对象,是应用某一对象的一个方法,语法为“apply(thisobj,[argarray])”;参数argarray表示的是以数组的形式进行传递。
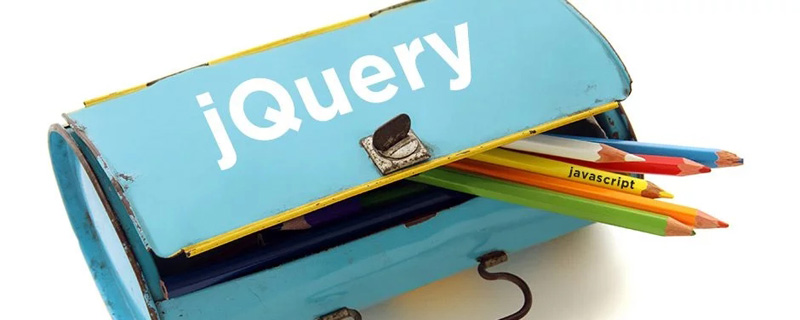
删除方法:1、用empty(),语法“$("div").empty();”,可删除所有子节点和内容;2、用children()和remove(),语法“$("div").children().remove();”,只删除子元素,不删除内容。
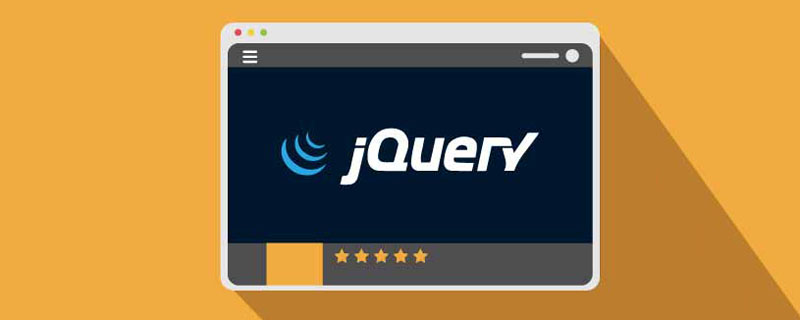
on()方法有4个参数:1、第一个参数不可省略,规定要从被选元素添加的一个或多个事件或命名空间;2、第二个参数可省略,规定元素的事件处理程序;3、第三个参数可省略,规定传递到函数的额外数据;4、第四个参数可省略,规定当事件发生时运行的函数。
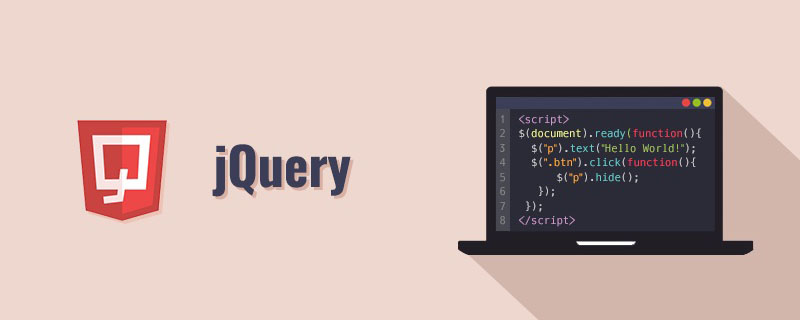
去掉方法:1、用“$(selector).removeAttr("readonly")”语句删除readonly属性;2、用“$(selector).attr("readonly",false)”将readonly属性的值设置为false。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
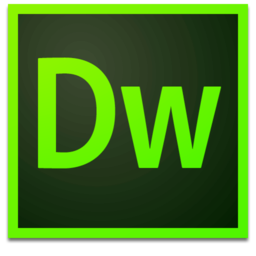
Dreamweaver Mac version
Visual web development tools
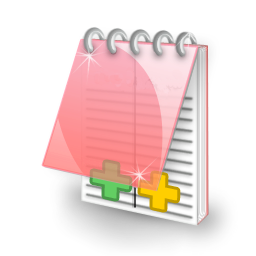
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.