


Introduction to comments, variables, arrays, constants, and function applications in PHP
What is the difference between single quotes and double quotes in php?
1. As can be seen from the following, variables with double quotes are parsed and output, while variables with single quotes are not parsed.
2. The parsing speed of single quotes is faster than that of double quotes
3. For single quotes, there are only two escapes ',\
4. Except for the above two escape characters, everything else is output as is.
5. For double quotes, in addition to \,',", there are also t, r, n, etc.
6. In addition to single quotes and double quotes used to declare string variables, there is also heredoc Method
Program code:
//$age = 22;
//$str1 = 'He is $age this year'; //''Output as is
//$str2 = "He is $age this year"; //" "To parse the variables inside, output 22
//echo $str1,"
",$str2;
Comments in php
(1) start with //.
(2) start with #.
#This is the shell single-line comment style
(3) There is a multi-line comment starting with /* and ending with */
Notes in php
1. In js, if a statement occupies one line, it can be at the end of the line Do not add the ; sign
2. However, when adding it in PHP, you must add a semicolon after each line
Although there is an exception in the last sentence of the entire PHP, it is strongly recommended to also add
3. For pure PHP pages, ?> is It doesn’t need to be written
Moreover, for files that are not run directly but are included in other pages, it is often recommended to end them without adding ?>
The pages included in this way will execute faster and faster
Variables in php
1 . There are 8 types of PHP variables
2. Integer, floating point, Boolean, string, NULL, array, object, resource type
3. In js, declare variables with var variable name [= value ], declare variables in php, direct variable name = value;
4. Variable naming convention in php
5. Variable names are composed of "letters", underscores, numbers, and numbers cannot begin
6. There is a '$' mark in front of variables in php
7. Echo is not a function, it is a grammatical structure
8. You can print out variables
9. When you want to print multiple variables, separate them with ','
10. . Passing by value of variables
11. Passing by reference and passing by assignment
15. String type
Variables and constants
(1) Constants
Variables can be reassigned at any time
//$age = 22;
//$age = 23;
//echo "
",$age;
(2) What is the difference between variables and constants?
1. They are declared in different ways
2. Once a constant is declared, its value cannot be changed
define ('PI',3.14);
PI =3.23; Syntax error
echo "
",PI;
3. Variables can be destroyed, but constants cannot be destroyed once they are established
unset($age);
var_dump($age);
4. Constants cannot be unregistered
unset(PI); //Syntax error, unregistration is not allowed
echo PI;
5. Variables have their own scope, and external ones cannot be accessed by default inside the function Variables,
and constants, once defined, whether defined globally or within a function.
Can be accessed from anywhere on the page.
(3) Naming conventions for variables and constants
1. The naming conventions for constants are the same as those for variables from a grammatical perspective.
A combination of letters, numbers, and underscores is allowed, and numbers cannot begin with them
2. From a customary perspective: general constants are in "uppercase"
//define('SF',342);
//echo SF; //Latest The version has made constant names case-sensitive
(4) What are the allowed values of constants?
1. Only scalar types (single types) can be assigned to constants;
2. Composite types, such as arrays and objects, cannot be assigned to a constant.
3. Resource type If assigned to a constant, it may cause some unpredictable errors.
The code is as follows:
Copy code The code is as follows:
1 define('AGE',22);
2 define('HEI',343.234);
3 define('ROOT','D:/www');
4 define('LOCK',true);
5 define('NON',NULL);
6 echo AGE,HEI,ROOT;
7 var_dump(LOCK);
8 var_dump(NON);
Control structure in php
(1) Any program is inseparable from variables, expressions, and control structures
(2) In php, else if can be written continuously, but it is not allowed in js. We recommend standard writing, that is, esle if separated open.
(3) In PHP, the scope of variables does not look out along the scope like in JS.
(4) In PHP, there is a special type of variables called super global variables. No matter you are in a function or inside a class, no matter how deep the code is packaged, you can access the variable.
php array and js array
(1) Two ways to create an array in js
(2) In js, the index of the array always starts from 0 and increases one by one, with no gap in the middle
1. var arr=new Array(1 ,2,3,4);
2. var arr= [1,2,3]
(3) Create an array in php
1. But in php, the index of the array is very flexible
2. It can be a number or a string
3 . It can even be a mixture of numbers and strings
4. If the index part specifies a numeric index
5. And there is a unit that does not specify an index
6. Then take the largest numeric index value that has appeared before in the unit and then +1 , as its key value
php creates an array as follows:
Copy code The code is as follows:
$arr=array(1,2,3);
print_r($arr);
//========== =================================
$arr=array(10=>'Zhao','adfdssd' =>'Qian','Sun','name'=>'Zhang Sanfeng');
print_r($arr);
7. In PHP, the key to how to reference the cell value of an array is the index
8. The index is a numeric index
//echo $arr[10];
9. If it is a string index, single quotes must be added. If no single quotes are added, it will be treated as a constant first
//define('name' ,'adfdssd');
//echo $arr[name];
(4) Associative array and index array
1. The index may be a pure number, a string, or a mixed string + number
2. If the index is a pure number, it is called an 'index array';
3. Otherwise, it is called an "associative array";
(5) The difference between functions in php and functions in js
1. In js , you can declare a function with the same name multiple times
2. But in a php page, you cannot declare a function with the same name multiple times
3. In js, function names are case-sensitive
4. In php, function names are not case-sensitive (class methods No distinction is made)
5. In php, the number of parameters when calling a function must be consistent with the parameters of the declared function
6. In php functions, when the function is declared, a certain parameter can have a "default value"
All the above knowledge Click the code display
Copy the code The code is as follows:
//================================ ================ Return to original position
//2. Integer type, floating point type, Boolean type, string type, NULL type
$age = 22;
$weight = 75.23;
$name ='Zhang San';
$money = false;
$house = null; //Equivalent to undiffed in js
echo $age,$weight,$money,
//===== ========================================= Return to original place
//10 . The value passed by the variable
$age =22;
$nian =$age;//Read the value of $age and assign it to $nian
$nian= 24;
echo $nian,'----' ,$age;
//============================================== =====
//11. Pass-by-reference assignment
$money =10000;
$credit = &$money; //Declare the $credit variable and point the credit pointer to the storage space of money
$credit = 5000;
echo $credit,'--------',$money;
unset($credit);
echo $credit;
//============== ==================================
//String type
$str1 = 'hello';
$ str2 = "world";
echo $str1,$str2,"
";
//======================== ========================
$age = 22;
function t(){
var_dump($age);
}
t();
define('HEI',88.63);
function s(){
var_dump(HEI);
}
s();
// Note: Functions in PHP cannot be declared repeatedly, and variables in functions must be packaged more strictly , only works within functions. It will not run outside and work
//5. For the above situation, you can use variables or constants, but we choose constants.
//Reason: One is ROOt, which is often quoted
//The second is: if variables are used, $ROOT ='a'; it is very likely that the value will be changed during multi-person development process
//There are also disadvantages to using constants Place:
//Constants will not be destroyed once defined
//Constants are always internal and cannot be destroyed.
//================================================== ======
//In php, variable names are also variable.
$talk='hello';
$heat= 'kill you';
$love= 'love';
echo $love,"
";
$action = 'talk';
$t ='action';
echo $$$t;
//==================================== ==================
//Advance notice: Not only variable names, but also function names are variable, and class names are also variable.
////The constant name also needs to be changed
define('PI',3.14);
define('HEI',342);
$cons= 'PI';
echo $cons,"
";
echo constant($cons); //constant is the name that treats the value of a variable as a constant and refers to the constant
//==================== ================================= Return to original position
//Control structure in php
//Any program is inseparable from variables, expressions, and control structures
if ,if/else,if/else if/ esle
$num=3;
if($num >2){
echo 'in In php, 3 is greater than 2',"
";
}
if($num >5){
echo '3 is greater than 5',"
";
}else {
echo '3 is not greater than 5';
}
//
if($nun==1){
echo 'Today is Monday';
}else if($num ==3){
echo 'Today It's Wednesday';
}else{
echo 'Not one, not two, not three';
}
////In php, else if can be written consecutively, but not in js
//We recommend standard writing Write, that is, esle if separated.
//================================================== ======
switch case statement
$num = 3;
switch($num){
case 1:
echo 'Today is Monday',"
";
break;
case 2 :
echo 'Today is Tuesday',"
";
break;
case 3:
echo 'Today is Wednesday',"
";
break;
default:
echo ' Don't know';
break;
}
/*
Suppose someone has 100,000 cash and needs to pay a fee every time he passes through an intersection.
The fee rule is that when his cash is greater than 50,000, he needs to pay 5% cash every time he passes the intersection. If the cash is less than or equal to
equal to 50,000, he needs to pay 5,000 each time. Please write a program to calculate how many times this person can pass this intersection
* /
for ($m =100000,$num=0;$m>=5000;$num++){
if ($m>50000){
$m*=0.98;
}else{
$m-=5000 ;
}
}
//============================================ =
//while ,do/while
//Use while to print $1-9;
$i =1;
while($iecho $i++,"
";
}
$i=0;
while(++$iecho $i,"
";
}
//============== =============================
while(){}
$i=0;
while(++$iif($i==5){
break;
continue;
}
echo $i,"
";
}
////========== ==================================
//Super global variable in php
$num =99;
function t(){
echo $num;
/
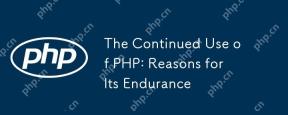
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
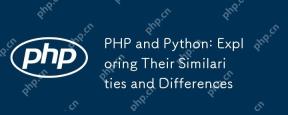
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
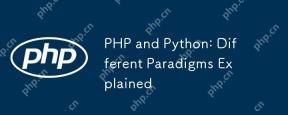
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
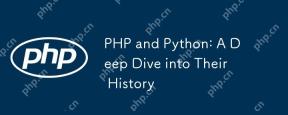
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
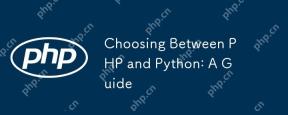
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
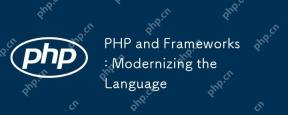
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
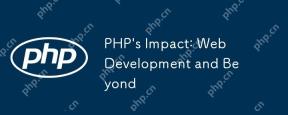
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
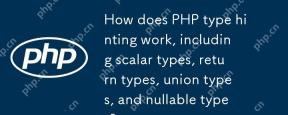
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
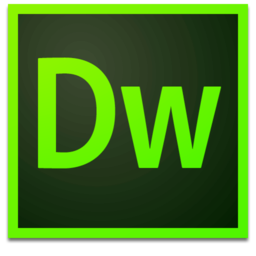
Dreamweaver Mac version
Visual web development tools
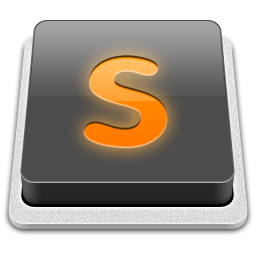
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
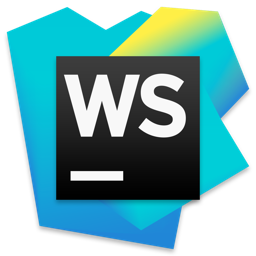
WebStorm Mac version
Useful JavaScript development tools