Collected 10 jQuery tips/code snippets to help you develop quickly.
1. Back to top button
You can use animate and scrollTop to implement the animation of returning to the top without using other plug-ins.
// Back to top $('a.top').click(function () { $(document.body).animate({scrollTop: 0}, 800); return false; }); <!-- Create an anchor tag --> <a class="top" href="#">Back to top</a>
Changing the value of scrollTop can adjust the distance between the return and the top, and the second parameter of animate is the time required to perform the return action (unit: milliseconds).
2. Preload images
If your page uses a lot of invisible images (such as: hover display), you may need to preload them:
$.preloadImages = function () { for (var i = 0; i < arguments.length; i++) { $('<img alt="10 jquery tips you must master" >').attr('src', arguments[i]); } }; $.preloadImages('img/hover1.png', 'img/hover2.png');
3. Check whether the image is loaded
Sometimes You need to ensure that the image has been loaded in order to perform the following operations:
$('img').load(function () { console.log('image load successful'); });
You can replace img with other IDs or classes to check whether the specified image is loaded.
4. Automatically modify broken images
If you happen to find broken image links on your website, you can replace them with an image that cannot be easily replaced. Adding this simple code can save you a lot of trouble:
$('img').on('error', function () { $(this).prop('src', 'img/broken.png'); });
Even if your website doesn’t have broken image links, there’s no harm in adding this code.
5. Switch class attribute on mouse hover
If you want to change the effect when the user hovers the mouse over a clickable element, the following code can be added when it hovers over the element class attribute, when the user mouses away, the class attribute will be automatically canceled:
$('.btn').hover(function () { $(this).addClass('hover'); }, function () { $(this).removeClass('hover'); });
You only need to add the necessary CSS code. If you want cleaner code, you can use the toggleClass method:
$('.btn').hover(function () { $(this).toggleClass('hover'); });
Note: Using CSS directly to achieve this effect may be a better solution, but you still need to know the method.
6. Disable input fields
Sometimes you may need to disable a form's submit button or an input field until the user performs some action (e.g., checking the "Read Terms" checkbox). You can add the disabled attribute until you want to enable it:
$('input[type="submit"]').prop('disabled', true);
All you have to do is execute the removeAttr method and pass in the attribute to be removed as a parameter:
$('input[type="submit"]').removeAttr('disabled');
7. Prevent the link from loading
Sometimes you don’t Want to link to a page or reload it, you may want it to do some other things or trigger some other scripts, you can do this:
$('a.no-link').click(function (e) { e.preventDefault(); });
8. Switch fade/slide
fade and slide are what we do in jQuery Animation effects are often used in . They can make elements display better. But if you want the first effect to be used when the element is displayed, and the second effect to be used when it disappears, you can do this:
// Fade $('.btn').click(function () { $('.element').fadeToggle('slow'); }); // Toggle $('.btn').click(function () { $('.element').slideToggle('slow'); });
9. Simple accordion effect
This is a quick and easy way to achieve an accordion effect:
// Close all panels $('#accordion').find('.content').hide(); // Accordion $('#accordion').find('.accordion-header').click(function () { var next = $(this).next(); next.slideToggle('fast'); $('.content').not(next).slideUp('fast'); return false; });
10. Make two DIVs the same height
Sometimes you need to make two divs the same height, regardless of the content inside them. You can use the following code snippet:
var $columns = $('.column'); var height = 0; $columns.each(function () { if ($(this).height() > height) { height = $(this).height(); } }); $columns.height(height);
This code will loop through a group of elements and set their height to the maximum height among the elements.
Original English text: jQuery Tips Everyone Should Know
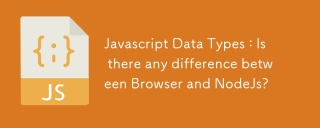
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
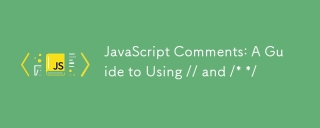
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
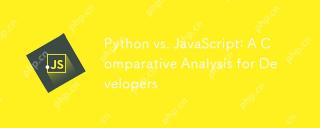
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
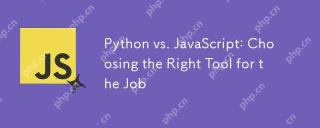
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
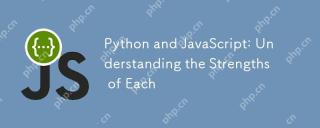
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
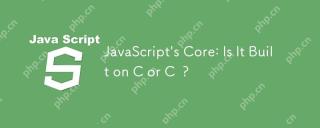
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
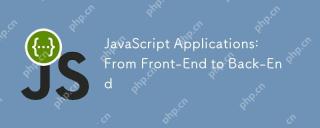
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
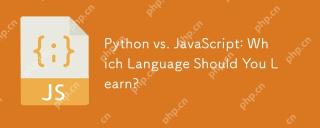
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
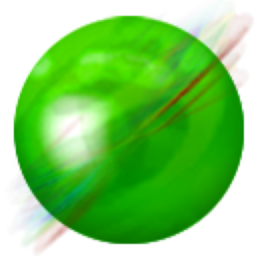
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
