XML is widely used in web development, usually as a carrier for data transmission. Generally, when data is passed to the front end, it needs to be parsed with JavaScript before it can be used. Therefore, parsing XML with JavaScript is very common.
There is the following XML file:
<?xml version="1.0" encoding="ISO-8859-1" ?> <note> <to>duncan</to> <from>John</from> <heading>Reminder</heading> <body>Don't forget the meeting!</body> </note>
can be parsed using the following method:
<html> <head> <script type="text/javascript"> function parseXML() { try //Internet Explorer { xmlDoc=new ActiveXObject("Microsoft.XMLDOM"); } catch(e) { try //Firefox, Mozilla, Opera, etc. { xmlDoc=document.implementation.createDocument("","",null); } catch(e) { alert(e.message); return; } } xmlDoc.async=false; xmlDoc.load("note.xml"); document.getElementById("to").innerHTML= xmlDoc.getElementsByTagName("to")[0].childNodes[0].nodeValue; document.getElementById("from").innerHTML= xmlDoc.getElementsByTagName("from")[0].childNodes[0].nodeValue; document.getElementById("message").innerHTML= xmlDoc.getElementsByTagName("body")[0].childNodes[0].nodeValue; </script> </head> <body onload="parseXML()"> <h1 id="www-nowamagic-net">www.nowamagic.net</h1> <p><b>To:</b> <span id="to"></span><br /> <b>From:</b> <span id="from"></span><br /> <b>Message:</b> <span id="message"></span> </p> </body> </html>
xmlDoc.getElementsByTagName("to")[0].childNodes[0].nodeValue How to understand this code?
xmlDoc - XML document created by a parser.
getElementsByTagName("to")[0] - the first
childNodes[0] - The first child element (text node) of the
nodeValue - the value of the node (the text itself).
If the xml file is:
<?xml version="1.0" encoding="ISO-8859-1" ?> <note> <to>asdfsd <too>duncan1</too> </to> <too>duncan2</too> <from>John</from> <heading>Reminder</heading> <body>Don't forget the meeting!</body> </note>
Read the first
Read the second
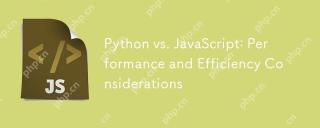
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
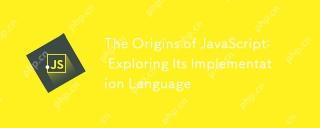
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
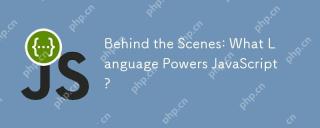
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
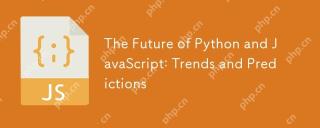
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
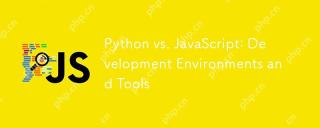
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
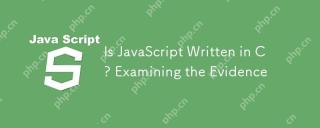
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
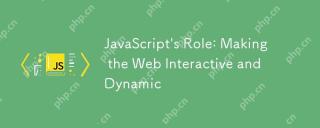
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
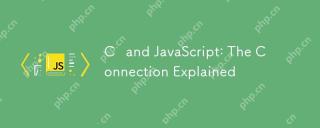
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment
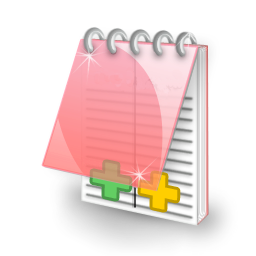
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
