What is XML?
XML refers to eXtensible Markup Language. You can learn XML tutorials through this site
XML is designed to transmit and store data.
XML is a set of rules that define semantic markup that divides a document into components and identifies those components.
It is also a meta-markup language, that is, it defines a syntactic language used to define other semantic and structured markup languages related to specific fields.
Python’s parsing of XML
Common XML programming interfaces include DOM and SAX. These two interfaces process XML files in different ways, and of course the usage scenarios are also different.
Python has three methods to parse XML, SAX, DOM, and ElementTree:
1.SAX (simple API for XML)
pyhton The standard library contains a SAX parser. SAX uses an event-driven model, through the process of parsing XML Trigger events one by one and call user-defined callback functions to process XML files.
2.DOM (Document Object Model)
parses XML data into a tree in memory, and operates XML by operating on the tree.
3.ElementTree (Element Tree)
ElementTree is like a lightweight DOM with a convenient and friendly API. The code has good usability, is fast, and consumes less memory.
Note: Because DOM needs to map XML data to a tree in memory, it is relatively slow and consumes more memory. SAX streaming reading of XML files is faster and takes up less memory, but requires the user to implement a callback function. (handler).
The content of the XML example file movies.xml used in this chapter is as follows:
War, Thriller
< ;description>A schientific fiction
python use SAX parses xml
SAX is an event-driven API.
Using SAX to parse XML documents involves two parts: the parser and the event handler.
The parser is responsible for reading the XML document and sending events to the event processor, such as element start and element end events;
The event processor is responsible for responding to the event and processing the passed XML data.
1. Process large files;
2. Only need part of the file, or only need specific information from the file.
3. When you want to build your own object model.
To use sax to process xml in python, you must first introduce the parse function in xml.sax and the ContentHandler in xml.sax.handler.
ContentHandler class method introduction
characters(content) method
Calling timing:
Starting from the line, before encountering the label, there are characters, and the value of content is these strings.
From one label, there are characters before encountering the next label, and the value of content is these strings.
From a label, there are characters before encountering the line terminator, and the value of content is these strings. The
tag can be a start tag or an end tag.
startDocument() method
is called when the document is started.
endDocument() method
is called when the parser reaches the end of the document. The
startElement(name, attrs) method
is called when an XML start tag is encountered. name is the name of the tag, and attrs is the attribute value dictionary of the tag.
endElement(name) method
is called when an XML end tag is encountered.
make_parser method
The following method creates a new parser object and returns it.
xml.sax.make_parser([parser_list])
Parameter description:
parser_list - optional parameters, parser list
parser method
The following method creates a SAX parser device and Parse xml document:
xml.sax.parse(xmlfile, contenthandler[, errorhandler])
Parameter description:
xmlfile - xml file name
contenthandler - must be a ContentHan dler object
errorhandler - If this parameter is specified, errorhandler must be a SAX ErrorHandler object
parseString method
parseString method creates an XML parser and parses an xml string:
xml.sax.parseString(xmlstring, contenthandler [, errorhandler])
Parameter description:
xmlstring - xml string
contenthandler - must be a ContentHandler object
errorhandler - if this parameter is specified, errorhandler must be a SAX ErrorHandler object
Python Parse XML instance
#!/usr/bin/python
import xml.sax
class MovieHandler( xml.sax.ContentHandler ):
def __init__(self):
self .CurrentData = ""
self.type . ""
self.description = ""
# Element start event processing
def startElement(self, tag, attributes):
self.CurrentData = tag
if tag == "movie":
print "***** Movie*****"
Movie**** if self. CurrentData == "type":
CurrentData == " year":
.
T Print "start:", seld.stars elif Self.currentData == "Description": Print "Description:", Self.Descript Self.currentdata = " #
def characters(self, content): if self.CurrentData == "type": self.type = content elif self.CurrentData == "format":
self.format = content
elif self.CurrentData == "year":
self.year = content
elif self.CurrentData == "rating":
self.rating = content
elif self.CurrentData == "stars":
self.stars = content
elif self.CurrentData == "description":
self.description = content
if ( __name__ == "__main__"):
# 创建一个 XMLReader
parser = xml.sax.make_parser()
# turn off namepsaces
parser.setFeature(xml.sax.handler.feature_namespaces, 0)
# 重写 ContextHandler
Handler = MovieHandler()
parser.setContentHandler( Handler )
parser.parse("movies.xml")
以上代码执行结果如下:
*****Movie*****
Title: Enemy Behind
Type: War, Thriller
Format: DVD
Year: 2003
Rating: PG
Stars: 10
Description: Talk about a US-Japan war
*****Movie*****
Title: Transformers
Type: Anime, Science Fiction
Format: DVD
Year: 1989
Rating: R
Stars: 8
Description: A schientific fiction
*****Movie*****
Title: Trigun
Type: Anime, Action
Format: DVD
Rating: PG
Stars: 10
Description: Vash the Stampede!
*****Movie*****
Title: Ishtar
Type: Comedy
Format: VHS
Rating: PG
Stars: 2
Description: Viewable boredom
完整的 SAX API 文档请查阅Python SAX APIs
使用xml.dom解析xml
文件对象模型(Document Object Model,简称DOM),是W3C组织推荐的处理可扩展置标语言的标准编程接口。
一个 DOM 的解析器在解析一个 XML 文档时,一次性读取整个文档,把文档中所有元素保存在内存中的一个树结构里,之后你可以利用DOM 提供的不同的函数来读取或修改文档的内容和结构,也可以把修改过的内容写入xml文件。
python中用xml.dom.minidom来解析xml文件,实例如下:
#!/usr/bin/python
from xml.dom.minidom import parse
import xml.dom.minidom
# 使用minidom解析器打开 XML 文档
DOMTree = xml.dom.minidom.parse("movies.xml")
collection = DOMTree.documentElement
if collection.hasAttribute("shelf"):
print "Root element : %s" % collection.getAttribute("shelf")
# 在集合中获取所有电影
movies = collection.getElementsByTagName("movie")
# 打印每部电影的详细信息
for movie in movies:
print "*****Movie*****"
if movie.hasAttribute("title"):
print "Title: %s" % movie.getAttribute("title")
type = movie.getElementsByTagName('type')[0]
print "Type: %s" % type.childNodes[0].data
format = movie.getElementsByTagName('format')[0]
print "Format: %s" % format.childNodes[0].data
rating = movie.getElementsByTagName('rating')[0]
print "Rating: %s" % rating.childNodes[0].data
description = movie.getElementsByTagName('description')[0]
print "Description: %s" % description.childNodes[0].data
The execution result of the above program is as follows:
Root element : New Arrivals
*****Movie*****
Title: Enemy Behind
Type: War, Thriller
Format: DVD
Rating : PG
Description: Talk about a US-Japan war
*****Movie*****
Title: Transformers
Type: Anime, Science Fiction
Format: DVD
Rating: R
Description: A schientific fiction
*****Movie*****
Title: Trigun
Type: Anime, Action
Format: DVD
Rating: PG
Description: Vash the Stampede!
*****Movie*****
Title: Ishtar
Type: Comedy
Format: VHS
Rating: PG
Description: Viewable boredom
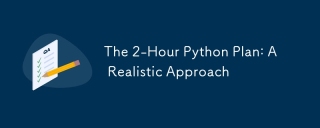
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
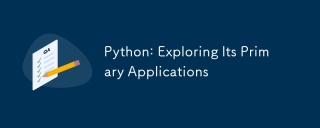
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
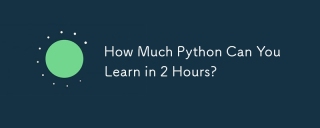
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
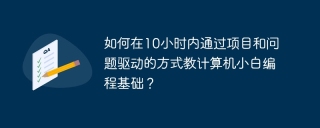
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
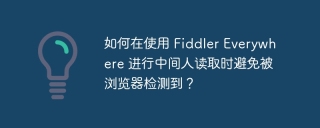
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
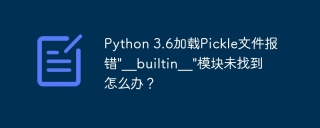
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
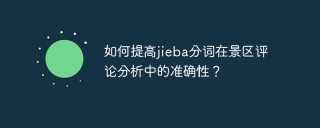
How to solve the problem of Jieba word segmentation in scenic spot comment analysis? When we are conducting scenic spot comments and analysis, we often use the jieba word segmentation tool to process the text...

How to use regular expression to match the first closed tag and stop? When dealing with HTML or other markup languages, regular expressions are often required to...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
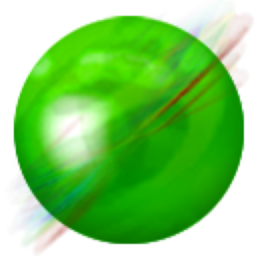
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
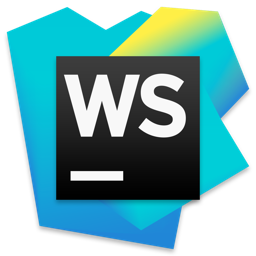
WebStorm Mac version
Useful JavaScript development tools