Sequence is the most basic data structure in Python. Each element in the sequence is assigned a number - its position, or index, with the first index being 0, the second index being 1, and so on.
Python has 6 built-in types for sequences, but the most common ones are lists and tuples.
Operations that can be performed on sequences include indexing, slicing, adding, multiplying, and checking members.
In addition, Python has built-in methods for determining the length of a sequence and determining the largest and smallest elements.
List is the most commonly used Python data type, which can appear as a comma-separated value within square brackets.
The data items of the list do not need to be of the same type
To create a list, just enclose the different data items separated by commas in square brackets. As shown below:
list1 = ['physics', 'chemistry', 1997, 2000];
list2 = [1, 2, 3, 4, 5];
list3 = ["a" , "b", "c", "d"];
The same as the index of the string, the list index starts from 0. Lists can be intercepted, combined, etc.
Access the values in the list
Use subscript index to access the values in the list. You can also use square brackets to intercept characters, as shown below:
#!/usr/bin/python
list1 = ['physics', 'chemistry', 1997, 2000];
list2 = [1, 2, 3, 4, 5, 6, 7];
print "list1[0] : ", list1[0]
print "list2[1:5]: ", list2[1:5]
The output result of the above example:
list1[0]: physics
list2[1:5]: [2, 3, 4, 5]
Update list
You can modify or update the data items in the list, you can also use append() method to add list items as follows:
#!/usr/bin/python
list = ['physics', 'chemistry', 1997, 2000];
print " Value available at index 2 : "
print list[2];
list[2] = 2001;
print "New value available at index 2 : "
print list[2];
Note: We will discuss the use of the append() method in the next chapter
The above example output result:
Value available at index 2:
1997
New value available at index 2:
2001
Delete list elements
You can use the del statement to delete elements of the list, as shown in the following example:
#!/usr/bin/python
list1 =[ 'physics', 'chemistry', 1997, 2000];
print list1;
del list1[2];
print "After deleting value at index 2 : "
print list1;
The output result of the above example:
['physics', 'chemistry', 1997, 2000]
After deleting value at index 2 :
['physics', 'chemistry', 2000]
Note: We will discuss the use of the remove() method in the next chapter
Python list script operators
The + and * operators for lists are similar to strings. The + sign is used for combined lists, and the * sign is used for repeated lists.
looks like this:
Python expression
Result
Description
len([1, 2, 3]) 3 Length
[1, 2, 3] + [4, 5, 6] [1, 2, 3, 4, 5, 6] Combination
['Hi!'] * 4 ['Hi!', 'Hi!', 'Hi!', 'Hi!'] Repeat
3 in [1, 2, 3] True Whether the element exists in the list
for x in [1, 2, 3]: print x, 1 2 3 Iteration
Python list interception
Python list interception and string The operation type is as follows:
L = ['spam', 'Spam', 'SPAM!']
Operation:
Python expression
result
Description
L[2] 'SPAM!' Read the third element in the list
L[-2] 'Spam' Read the second to last element in the list
L[1:] ['Spam', 'SPAM!'] Intercept the list starting from the second element
Python list functions & methods
Python includes the following functions:
Serial number
Function
1 cmp(list1, list2)
Compare the elements of two lists
2 len(list)
The number of list elements
3 max(list)
Return the maximum value of the list elements
4 min(list)
Return the list elements Minimum value
5 list(seq)
Convert tuple to list
Python contains the following methods:
serial number
method
1 list.append(obj)
Add a new object at the end of the list
2 list.count(obj)
Count the number of times an element appears in the list
3 list.extend(seq)
Append multiple values from another sequence at the end of the list at once (extend the original with a new list list)
4 list.index(obj)
Find the index position of the first matching item of a value from the list
5 list.insert(index, obj)
Insert the object into the list
6 list. pop([index])
Removes an element in the list (the last element by default) and returns the value of the element
7 list.remove(obj)
Removes the first match of a value in the list
8 list.reverse()
reverse the elements in the list
9 list.sort([func])
sort the original list
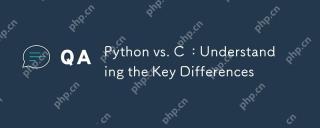
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
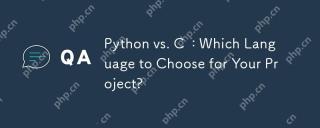
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
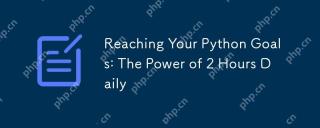
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
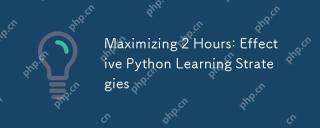
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
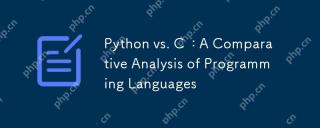
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
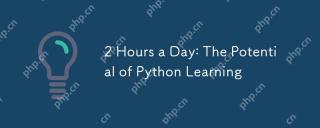
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
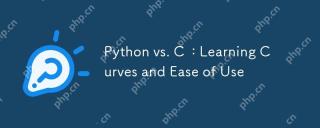
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
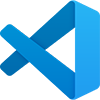
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
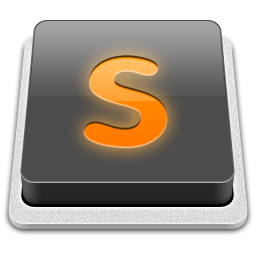
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
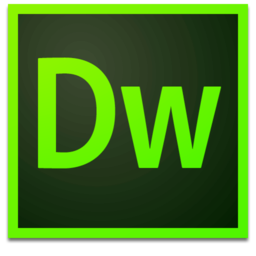
Dreamweaver Mac version
Visual web development tools