JSON is a lightweight text data exchange format. It is smaller, faster, and easier to parse than XML. Therefore, during the PHP development process, we often use it to transfer data. In this article, UncleToo will introduce how to use PHP. Manipulating JSON data
PHP is generally used more often in AJAX to manipulate JSON data. You can pass JSON format data to AJAX, or you can parse the JSON data returned by AJAX into the strings we need. In PHP, you can use the json_decode() function to parse JSON format data, and use the json_encode() function to generate a string (array) into JSON format.
Look at the example first:
Example 1:
Php code
<?php $json = '{"a":1, "b":2, "c":3, "d":4, "e":5 }'; var_dump(json_decode($json)); echo "<br/>"; var_dump(json_decode($json,true)); ?>
Output:
object(stdClass)#1 (5) { ["a"]=> int(1) ["b" ]=> int(2) ["c"]=> int(3) ["d"]=> int(4) ["e"]=> int(5) }
array(5 ) { ["a"]=> int(1) ["b"]=> int(2) ["c"]=> int(3) ["d"]=> int(4) ["e"]=> int(5) }
Example 2:
Php code
<?php $arr = array ('a'=>1,'b'=>2,'c'=>3,'d'=>4,'e'=>5); echo json_encode($arr); ?>
Output:
{"a":1,"b":2,"c": 3,"d":4,"e":5}
From Example 1, we can see that the json_decode function can be used to convert JSON data into an array. However, if JSON data is nested in JSON data, Then you can't write it directly like this. You need to use a custom function to convert the nested JSON data into an array.
Example:
Php code
<?php function json_to_array($web){ $arr=array(); foreach($web as $k=>$w){ if(is_object($w)) $arr[$k]=json_to_array($w); //判断类型是不是object else $arr[$k]=$w; } return $arr; } ?>
Calling example:
Php code
<?php $s='{"webname":"UncleToo","url":"www.uncletoo.com","menu":{"PHP":"1","DataBase":"2","Web":"3"}}'; $web=json_decode($s); $arr=json_to_array($web); print_r($arr); ?>
Output:
Array ( [webname] => UncleToo [url] => www.uncletoo.com [ menu] => Array ( [PHP] => 1 [DataBase] => 2 [Web] => 3 ) )
The above are the common methods for operating JSON data in PHP. If you have other ideas and methods, you can Discuss with UncleToo.
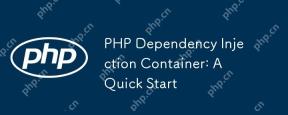
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
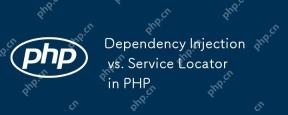
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
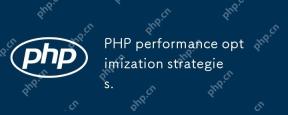
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
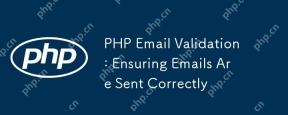
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
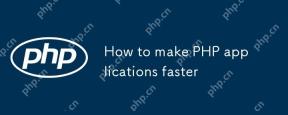
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
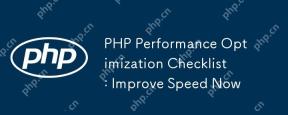
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
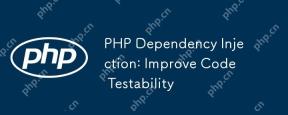
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
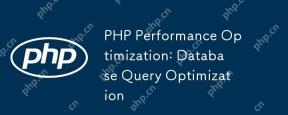
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
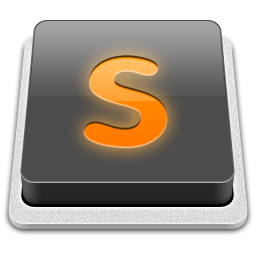
SublimeText3 Mac version
God-level code editing software (SublimeText3)
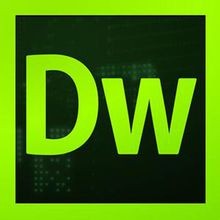
Dreamweaver CS6
Visual web development tools
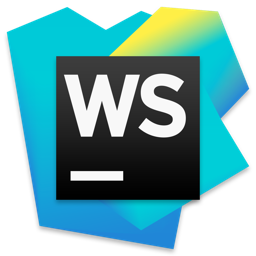
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
