


javascript - Regarding the inheritance method of js, please solve it!
<code>function person(name,age){ this.name = name; this.age = age; } person.prototype.say = function(){ console.log(this.name+":"+this.age); } function superman(name,age){ person.call(this,name,age); } superman.prototype = new person(); var s = new superman('superman',29); </code>
I saw this inheritance method in a book and said it was perfect, but I don’t think so because its superman.prototype = new person();
This sentence will add the instance attributes of the parent class to the child class. On the prototype, although person.call(this, name, age);
has obtained the instance attributes of the parent class, it feels like this pollutes the prototype of the subclass. How to break it?
Okay, the problem is solved, using parasitic combined inheritance can solve this problem
<code>function object(obj){ function F(){} F.prototype = obj; return new F(); } function inheritProtoType(SuperType,SubType){ var prototype = object(SuperType.prototype); prototype.constructor = SubType; SubType.prototype = prototype; } function SuperType(){ this.name = 'yuhualingfeng'; this.friends = ['David','Bob','Lucy']; } SuperType.prototype.saySuperName = function(){ console.log(this.name); }; function SubType(){ SuperType.call(this); this.age = 30; } inheritProtoType(SuperType,SubType); SubType.prototype.saySubName = function(){ console.log(this.name); }; var subType = new SubType(); </code>
Reply content:
<code>function person(name,age){ this.name = name; this.age = age; } person.prototype.say = function(){ console.log(this.name+":"+this.age); } function superman(name,age){ person.call(this,name,age); } superman.prototype = new person(); var s = new superman('superman',29); </code>
I saw this inheritance method in a book and said it was perfect, but I don’t think so because its superman.prototype = new person();
This sentence will add the instance attributes of the parent class to the child class. On the prototype, although person.call(this, name, age);
has obtained the instance attributes of the parent class, it feels like this pollutes the prototype of the subclass. How to break it?
Okay, the problem is solved, using parasitic combined inheritance can solve this problem
<code>function object(obj){ function F(){} F.prototype = obj; return new F(); } function inheritProtoType(SuperType,SubType){ var prototype = object(SuperType.prototype); prototype.constructor = SubType; SubType.prototype = prototype; } function SuperType(){ this.name = 'yuhualingfeng'; this.friends = ['David','Bob','Lucy']; } SuperType.prototype.saySuperName = function(){ console.log(this.name); }; function SubType(){ SuperType.call(this); this.age = 30; } inheritProtoType(SuperType,SubType); SubType.prototype.saySubName = function(){ console.log(this.name); }; var subType = new SubType(); </code>
Object.create(Person.prototype);
This can be effectively solved, but please pay attention to compatibility
<code>function create(obj) { if (Object.create) { return Object.create(obj); } function f() {}; f.prototype = obj; return new f(); }</code>
Inherit person.prototype
Inherit instead of pollute

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
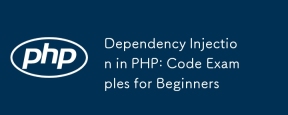
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
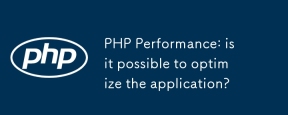
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
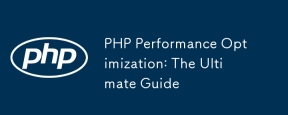
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
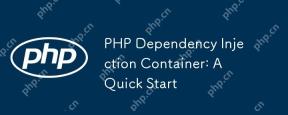
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
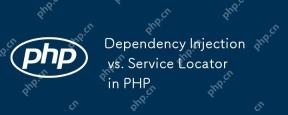
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
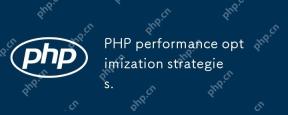
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
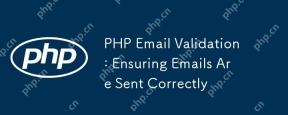
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
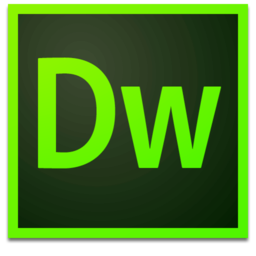
Dreamweaver Mac version
Visual web development tools
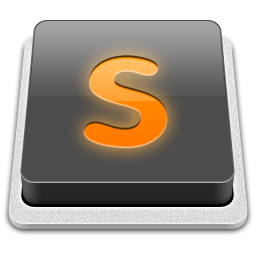
SublimeText3 Mac version
God-level code editing software (SublimeText3)
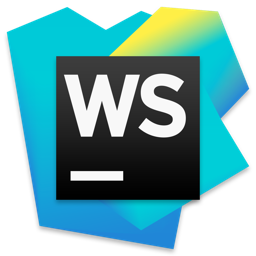
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
