I need to write some php recently, I have never written it before, I only know js. It seems that variables in PHP are not referenced by scope chains like JS. If the code below is like this, how to implement it?
<code>function A(){ $num = 0; function B(){ //这里如何引用到变量$num??? } }</code>
I checked and found that the global $num in B can refer to the global variable $num, but it seems that $num is not global. . . Please give me some advice.
Reply content:
I need to write some php recently, I have never written it before, I only know js. It seems that variables in PHP are not referenced by scope chains like JS. If the code below is like this, how to implement it?
<code>function A(){ $num = 0; function B(){ //这里如何引用到变量$num??? } }</code>
I checked and found that the global $num in B can refer to the global variable $num, but it seems that $num is not global. . . Please give me some advice.
Transfer:
JavaScript can use external variables directly in anonymous functions.
PHP anonymous functions cannot directly call the context variables of the code block by default. You need to use the use keyword to connect the closure (anonymous function) and external variables.
<code>function getMoney() { $rmb = 1; $dollar = 6; $func = function() use ( $rmb ) { echo $rmb; echo $dollar; }; $func(); } getMoney(); //输出: //1 //报错,找不到dorllar变量 </code>
As you can see, dollar is not declared in the use keyword, and it cannot be obtained in this anonymous function, so pay attention to this issue during development.
Some people may think whether it is possible to change the context variables in an anonymous function, but I found that it is not possible:
<code>function getMoney() { $rmb = 1; $func = function() use ( $rmb ) { echo $rmb; //把$rmb的值加1 $rmb++; }; $func(); echo $rmb; } getMoney(); //输出: //1 //1 </code>
It turns out that what use refers to is just a copy of the variable.
If you want to fully reference the variable instead of copying it, you need to add an & symbol in front of the variable:
<code>function getMoney() { $rmb = 1; $func = function() use ( &$rmb ) { echo $rmb; //把$rmb的值加1 $rmb++; }; $func(); echo $rmb; } getMoney(); //输出: //1 //2 </code>
If the anonymous function is returned to the outside world, the anonymous function will save the variables referenced by use, but the outside world cannot get these variables. In this way, the concept of 'closure' may be clearer:
<code>function getMoneyFunc() { $rmb = 1; $func = function() use ( &$rmb ) { echo $rmb; //把$rmb的值加1 $rmb++; }; return $func; } $getMoney = getMoneyFunc(); $getMoney(); $getMoney(); $getMoney(); //输出: //1 //2 //3 </code>
function A() { $num = 0; call_user_func(function () use ($num) { //这么用$num }); }
The above answer is just to answer your question. You'd better read the manual first and be familiar with the basic syntax of PHP. Otherwise, if you encounter a question, just ask it once on the Q&A website, which is too inefficient. Never write it in js. php

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
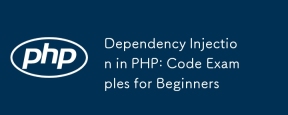
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
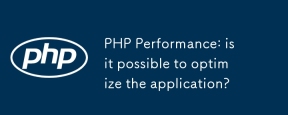
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
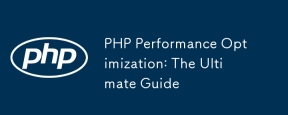
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
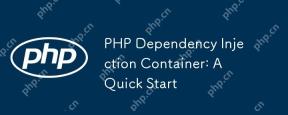
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
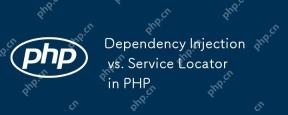
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
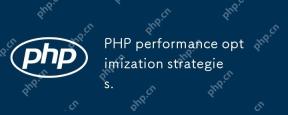
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
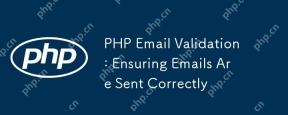
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
