


(5) Object-oriented design principles 1---General outline and single responsibility principle
1. General outline:
1. The five principles of object-oriented: single responsibility principle, interface isolation principle, open-closed principle, replacement principle, and dependency inversion principle.
2. Single responsibility principle:
1. As far as a class is concerned, there is only one reason for its change: the single responsibility principle.
2. Single responsibility has two meanings:
a. Avoid spreading the same responsibilities to different classes
b. Avoid one class taking on too many responsibilities
3. Reasons for following the single responsibility principle: Reduce coupling between classes, Improve class reusability.
Three. Factory pattern:
1. The factory pattern allows objects to be instantiated when code is executed. Able to 'produce' objects.
2. Example:
<?php /* * 单一职责原则 */ interface Db_Adapter{ /* * 数据库连接 * @param $config数据库配置 * @return resource */ public function connect($config); /* * 执行数据库查询 * @param string $query 数据库查询sql字符串 * @param mixed $handld 连接对象 * @return resource */ public function query($query,$handle); } class Db_Adapter_Mysql implements Db_Adapter{ private $_dbLink; /* * 数据库连接 * * @param $config 数据库配置 * @throws Db_Exception * @return resource */ public function connect($config){ if ($this->_dbLink = @mysql_connect($config->host.(empty($config->port) ? '' : ':'.$config->port), $config->user,$config->password,true)){ if (@mysql_select_db($config->database,$this->_dbLink)){ if ( $config->charset){ mysql_query("SET NAMES '{$config->charset}'",$this->_dbLink); } return $this->_dbLink; } } /*数据库异常*/ throw new Db_Exception(@mysql_error($this->_dbLink)); //这一句报了很多错 } /* * 执行数据库查询 * * @param string $query 数据库查询sql字符串 * @param mixed $handle 连接对象 * @return resource */ public function query($query,$handle){ $resource = ""; if ($resource == @mysql_query($query,$handle)){ return $resource; } } } class Db_Adapter_sqlite implements Db_Adapter{ private $_dbLink; //数据库连接字段标记 /* * 数据库连接函数 * * @param $config数据库配置 * @throws DB_exception * @return resource */ public function connect($config){ if ($this->_dbLink = @mysql_connect($config->host. (empty($config->port) ? '' : ':'.$config->port), $config->user,$config->password,true)){ if (@mysql_select_db($config->database, $this->_dbLink)){ if ($config->charset){ mysql_query("SET NAMES '{$config->charset}'",$this->_dbLink); } return $this->_dbLink; } } /*数据库异常*/ throw new Db_exception(@mysql_error($this->dbLink)); } /* * 执行数据库查询 * * @param string $query 数据库查询sql字符串 * @param mixed $handle 连接对象 * @return resource */ public function query($query,$handle){ $resource = ""; if ($resource == @mysql_query($query,$handle)){ return $resource; } } } $testDb = new Db_Adapter_Mysql(); $config = array( //这里写数据库配置 'host'=>'localhost', ); $testDb->connect($config); $testDb->query($sql,$handle);
Copyright statement: This article is an original article by the blogger and may not be reproduced without the permission of the blogger.
The above has introduced (5) Object-oriented design principle 1---the general outline and the single responsibility principle, including aspects of the content. I hope it will be helpful to friends who are interested in PHP tutorials.
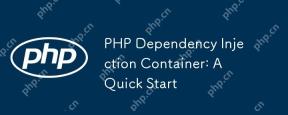
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
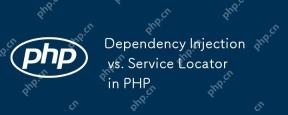
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
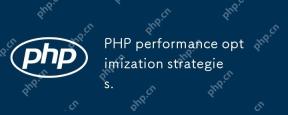
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
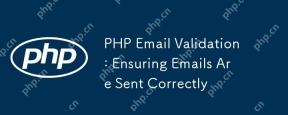
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
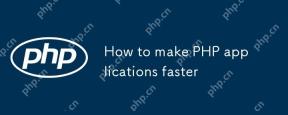
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
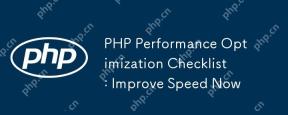
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
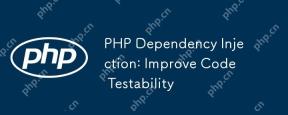
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
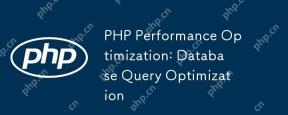
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
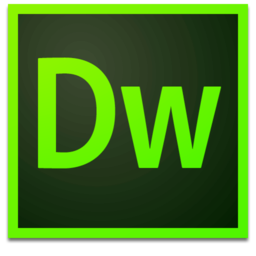
Dreamweaver Mac version
Visual web development tools
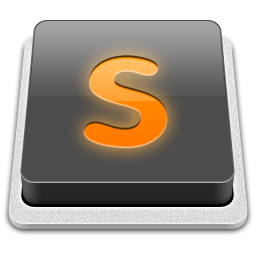
SublimeText3 Mac version
God-level code editing software (SublimeText3)
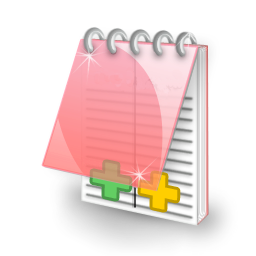
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
