PHP has supported MySQL since its early days, and included an API in its second version. Because the combination of the two is so common, this extension is enabled by default. However, PHP 5 released a newer MySQL extension called MySQL Improved, or mysqli for short.
Why release a new extension? The reasons are twofold. First, MySQL is evolving rapidly, and users who rely on older extensions are unable to take advantage of new features such as prepared statements, advanced connection options, and security improvements. Second, while the old extension was certainly fine to use, many people considered the procedural interface obsolete and preferred the object-oriented interface for not only tighter integration with other applications but the ability to extend it as needed. interface. To address these shortcomings, the MySQL developers decided it was time to revamp that extension, not only modifying the internal behavior to improve performance, but also introducing additional features that facilitate the use of features available in newer versions of MySQL.
Several key improvements:
# Object-oriented: MySQL extensions are encapsulated into a series of classes, thus encouraging the use of a programming paradigm that many people consider to be more convenient and efficient than PHP's traditional procedural approach. But those who prefer the procedural paradigm don't worry, because it also provides a traditional procedural interface.
# prepared statements: Can prevent SQL injection attacks. It eliminates the overhead and inconvenience of those queries that are executed repeatedly.
# Transaction support: Although PHP's original MySQL extension can also support transaction functions, the mysqli extension provides an object-oriented interface to these functions.
# Enhanced debugging capabilities: The mysqli extension provides many methods for debugging queries, making the development process more efficient.
# Embedded server support: MySQL 4.0 release introduces an embedded MySQL server library, so interested users can run a complete MYSQL server in client applications such as desktop applications. The mysqli extension provides methods for connecting to and operating on these embedded MySQL servers.
# Master/slave support: Starting from MySQL 3.23.15, MySQL provides support for replication. Using the mysqli extension, you can ensure that queries are routed to the master server in a replicated configuration.
Those users who are familiar with the original MySQL extension will find the enhanced mysqli extension very familiar, with almost the same naming convention. For example, the database connection function is called mysqli_connect instead of mysql_connect.
1. Prerequisites for installation
Starting from PHP 5, MySQL support is not bundled with the standard PHP distribution package. Therefore, PHP needs to be configured explicitly to take advantage of this extension.
1.1. Enable mysqli extension in Linux/UNIX
Use the --with-mysqli flag when configuring PHP. It should point to the location of the mysql_config program in MySQL 4.1 and later.
1.2. To enable the mysqli extension on Windows
You need to modify php.ini and uncomment this line: extension=php_mysqli.dll. If not, add this line. Of course, before enabling any extension, make sure that PHP's extension_dir directive points to the appropriate directory.
1.3. Use MYSQL local driver
For a long time, PHP requires that the MySQL client library be installed on the server running the PHP program, regardless of whether the MYSQL server happens to be local or elsewhere. PHP 5.3 removes this requirement and introduces a new MySQL driver called MySQL Native Driver, also called mysqlnd, which has many advantages over the driver just mentioned. It is not a new API, but a new "conduit" that existing APIs (mysql, mysqli, PDO_MySQL) can utilize to communicate with a MySQL server. It is recommended to use mysqlnd instead of other drivers (unless you have a very good reason).
To use mysqlnd with an extension, you need to recompile PHP, for example: --with-mysqli=mysqlnd. You can also specify a few more, such as %>./configure --with-mysqli=mysqlnd --with-pdo-mysql=mysqlnd. The mysqlnd driver also has some restrictions. Currently it does not provide compression or SSL support.
1.4. Managing user permissions
When a script initializes a connection to the MySQL server, permissions are passed and verified. The same is true when submitting commands that require permission verification. However, you only need to confirm the execution user when connecting; subsequent executions of the script will always be that user unless a new connection is made later.
1.5. Use sample data
It’s easy to add some examples when learning new knowledge. Database: corporate; table: products
CREATE TABLE products (
id INT NOT NULL AUTO_INCREMENT,
sku VARCHAR(8) NOT NULL,
name VARCHAR(100) NOT NULL,
price DECIMAL(5,2 ) NOT NULL,
PRIMARY KEY(id)
)
==================================== ========================================
2. Use mysqli extension
2.1 , establish and disconnect connections
First connect to the server, then select a database, and then close the connection. Object-oriented and procedural are both possible styles.
To use the object-oriented interface to interact with the MySQL server, you must first instantiate it using the constructor of the mysqli class.
mysqli([string host [, string username [, string pswd
] ) Many parameters of the function are the same as the traditional mysql_connect() function.
$mysqli = new mysqli('localhost', 'catalog_user', 'secret', 'corporate');
If at some point, you want to switch to another server or choose another database, you can use connect() and select_db() method. The parameters of the connect() method are the same as the constructor of the mysqli class.
// Instantiate the mysqli class
$mysqli = new mysqli();
// Connect to the database server and select a database
$mysqli->connect('localhost', 'root', '' , 'corporate');
------------------------------------------------ ----------------------------------------
or
// Connect to the database server
$mysqli = new mysqli('localhost', 'catalog_user', 'secret');
// Select the database
$mysqli->select_db('corporate');
Once the script is completed When executed, any open database connections are automatically closed and resources are restored. However, it is also possible that a page needs to use multiple database connections during execution, and these connections need to be closed correctly. Even if only one connection is used, it is a good practice to close it at the end of the script. $mysqli->close().
2.2. Handling connection errors
Connection errors should be carefully monitored and countermeasures taken accordingly. The mysqli extension provides some features that can be used to catch error messages. Another way is to use exceptions. For example, mysqli_connect_errno() and mysqli_connect_error() can be used to diagnose and display MySQL connection error messages.
2.3. Get the error message
2.3.1. Get the error code
errno() method returns the error code generated during the last MySQL function execution. 0 means no errors.
$mysqli = new mysqli('localhost', 'catalog_user', 'secret', 'corporate');
printf("Mysql error number generated: %d", $mysqli-> errno);
?>
2.3.2. Get the error message
error() method returns the most recently generated error message. If there is no error, an empty string is returned. The messaging language relies on the Mysql database server.
2.4. Store connection information in a separate file
In terms of secure programming practices, it is a good idea to change passwords regularly. There are also many scripts that need to access the database, and it is too troublesome to modify them one by one. The solution is to store it in a separate file and include it in your current file if necessary.
For example, you can put the mysqli constructor in a header file (mysql.connect.php):
$mysqli = new mysqli('localhost', 'catalog_user', 'secret', ' corporate');
?>
Then include it in other files:
include 'mysql.connect.php';
// begin database selection and queries.
?> ;
==================== Not finished yet, to be continued
The above has introduced the use of PHP and MySQL, including aspects of the content. I hope it will be helpful to friends who are interested in PHP tutorials.
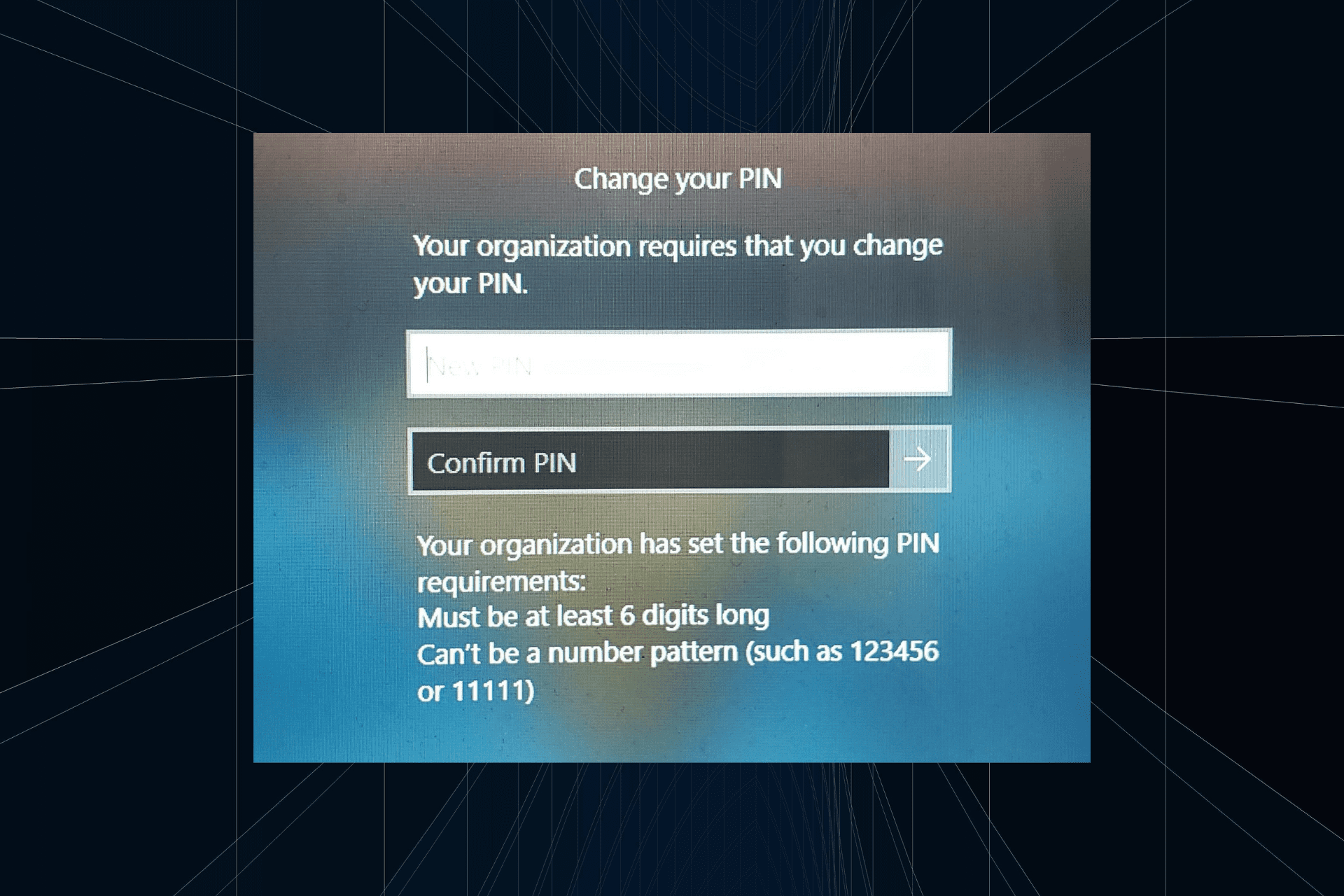
“你的组织要求你更改PIN消息”将显示在登录屏幕上。当在使用基于组织的帐户设置的电脑上达到PIN过期限制时,就会发生这种情况,在该电脑上,他们可以控制个人设备。但是,如果您使用个人帐户设置了Windows,则理想情况下不应显示错误消息。虽然情况并非总是如此。大多数遇到错误的用户使用个人帐户报告。为什么我的组织要求我在Windows11上更改我的PIN?可能是您的帐户与组织相关联,您的主要方法应该是验证这一点。联系域管理员会有所帮助!此外,配置错误的本地策略设置或不正确的注册表项也可能导致错误。即
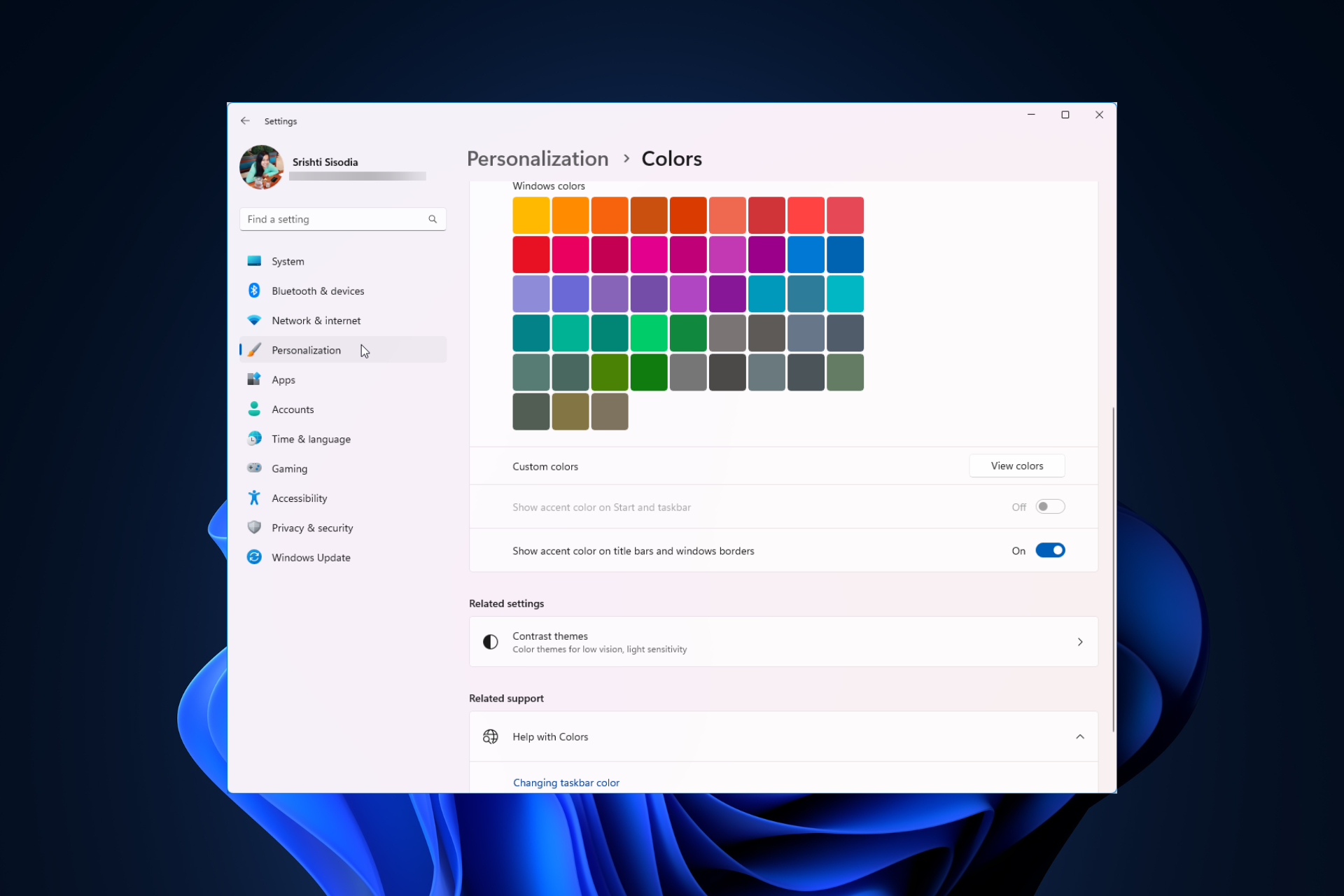
Windows11将清新优雅的设计带到了最前沿;现代界面允许您个性化和更改最精细的细节,例如窗口边框。在本指南中,我们将讨论分步说明,以帮助您在Windows操作系统中创建反映您的风格的环境。如何更改窗口边框设置?按+打开“设置”应用。WindowsI转到个性化,然后单击颜色设置。颜色更改窗口边框设置窗口11“宽度=”643“高度=”500“>找到在标题栏和窗口边框上显示强调色选项,然后切换它旁边的开关。若要在“开始”菜单和任务栏上显示主题色,请打开“在开始”菜单和任务栏上显示主题
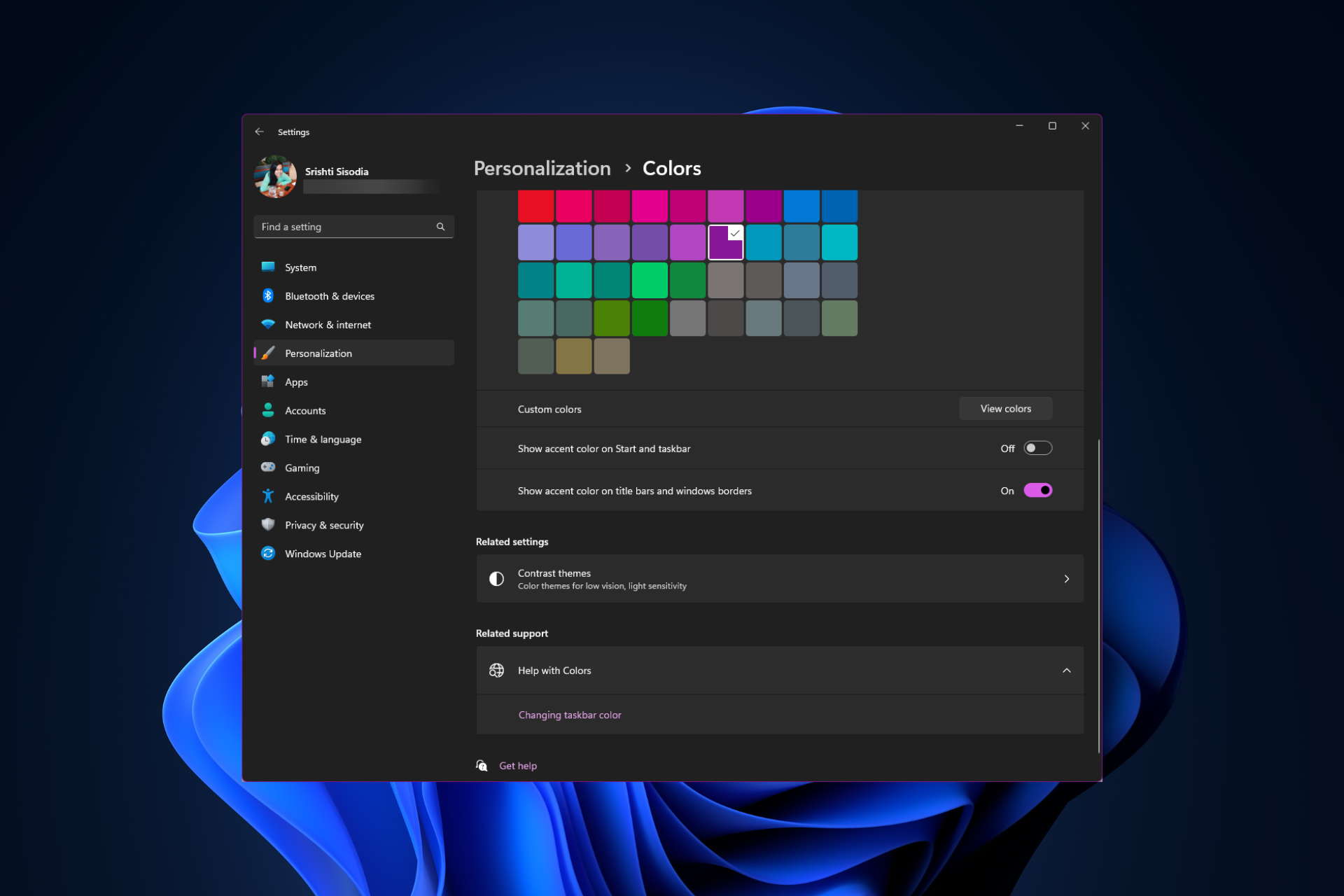
默认情况下,Windows11上的标题栏颜色取决于您选择的深色/浅色主题。但是,您可以将其更改为所需的任何颜色。在本指南中,我们将讨论三种方法的分步说明,以更改它并个性化您的桌面体验,使其具有视觉吸引力。是否可以更改活动和非活动窗口的标题栏颜色?是的,您可以使用“设置”应用更改活动窗口的标题栏颜色,也可以使用注册表编辑器更改非活动窗口的标题栏颜色。若要了解这些步骤,请转到下一部分。如何在Windows11中更改标题栏的颜色?1.使用“设置”应用按+打开设置窗口。WindowsI前往“个性化”,然
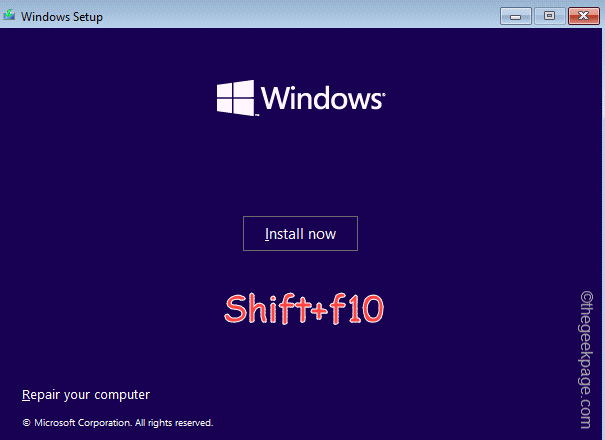
您是否在Windows安装程序页面上看到“出现问题”以及“OOBELANGUAGE”语句?Windows的安装有时会因此类错误而停止。OOBE表示开箱即用的体验。正如错误提示所表示的那样,这是与OOBE语言选择相关的问题。没有什么可担心的,你可以通过OOBE屏幕本身的漂亮注册表编辑来解决这个问题。快速修复–1.单击OOBE应用底部的“重试”按钮。这将继续进行该过程,而不会再打嗝。2.使用电源按钮强制关闭系统。系统重新启动后,OOBE应继续。3.断开系统与互联网的连接。在脱机模式下完成OOBE的所
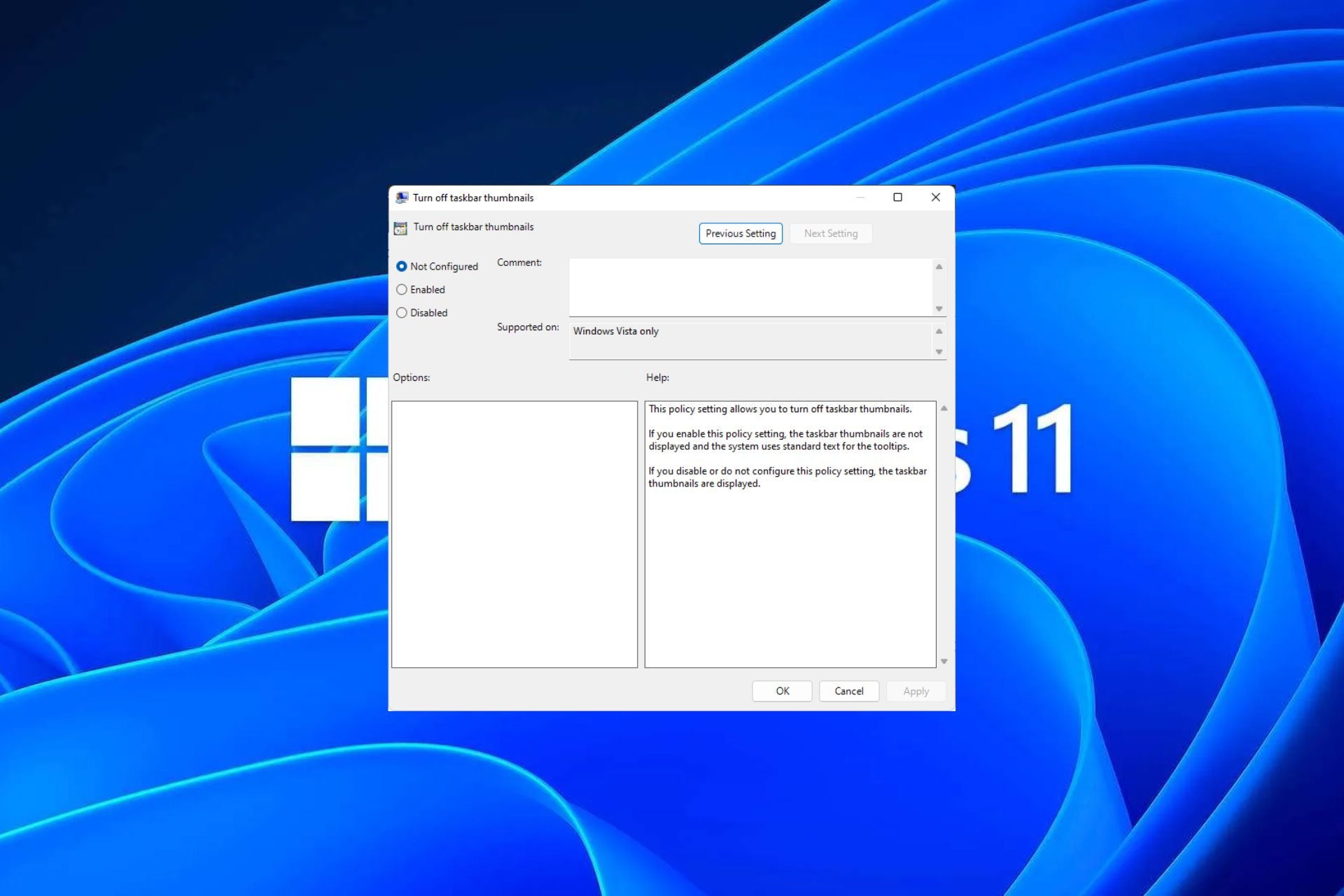
任务栏缩略图可能很有趣,但它们也可能分散注意力或烦人。考虑到您将鼠标悬停在该区域的频率,您可能无意中关闭了重要窗口几次。另一个缺点是它使用更多的系统资源,因此,如果您一直在寻找一种提高资源效率的方法,我们将向您展示如何禁用它。不过,如果您的硬件规格可以处理它并且您喜欢预览版,则可以启用它。如何在Windows11中启用任务栏缩略图预览?1.使用“设置”应用点击键并单击设置。Windows单击系统,然后选择关于。点击高级系统设置。导航到“高级”选项卡,然后选择“性能”下的“设置”。在“视觉效果”选
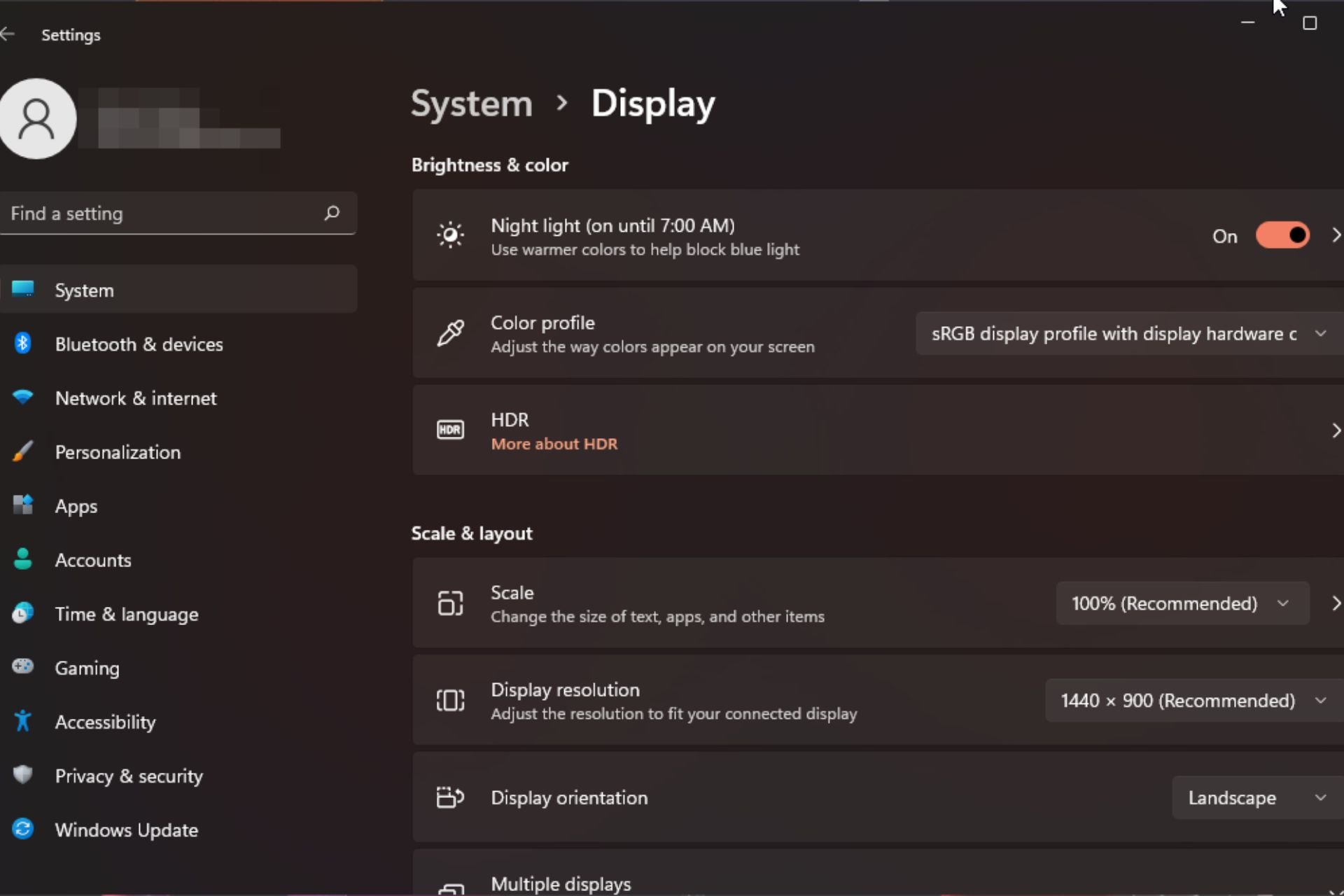
在Windows11上的显示缩放方面,我们都有不同的偏好。有些人喜欢大图标,有些人喜欢小图标。但是,我们都同意拥有正确的缩放比例很重要。字体缩放不良或图像过度缩放可能是工作时真正的生产力杀手,因此您需要知道如何对其进行自定义以充分利用系统功能。自定义缩放的优点:对于难以阅读屏幕上的文本的人来说,这是一个有用的功能。它可以帮助您一次在屏幕上查看更多内容。您可以创建仅适用于某些监视器和应用程序的自定义扩展配置文件。可以帮助提高低端硬件的性能。它使您可以更好地控制屏幕上的内容。如何在Windows11
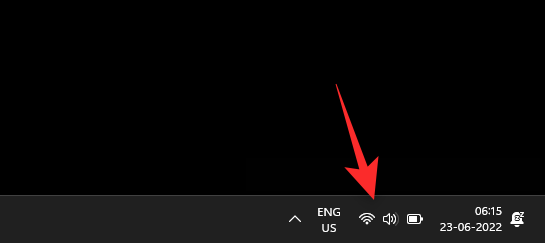
屏幕亮度是使用现代计算设备不可或缺的一部分,尤其是当您长时间注视屏幕时。它可以帮助您减轻眼睛疲劳,提高易读性,并轻松有效地查看内容。但是,根据您的设置,有时很难管理亮度,尤其是在具有新UI更改的Windows11上。如果您在调整亮度时遇到问题,以下是在Windows11上管理亮度的所有方法。如何在Windows11上更改亮度[10种方式解释]单显示器用户可以使用以下方法在Windows11上调整亮度。这包括使用单个显示器的台式机系统以及笔记本电脑。让我们开始吧。方法1:使用操作中心操作中心是访问
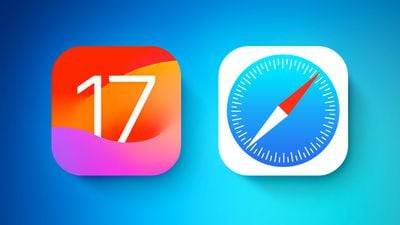
在iOS17中,Apple为其移动操作系统引入了几项新的隐私和安全功能,其中之一是能够要求对Safari中的隐私浏览选项卡进行二次身份验证。以下是它的工作原理以及如何将其关闭。在运行iOS17或iPadOS17的iPhone或iPad上,如果您在Safari浏览器中打开了任何“无痕浏览”标签页,然后退出会话或App,Apple的浏览器现在需要面容ID/触控ID认证或密码才能再次访问它们。换句话说,如果有人在解锁您的iPhone或iPad时拿到了它,他们仍然无法在不知道您的密码的情况下查看您的隐私


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
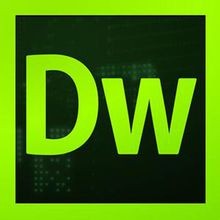
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
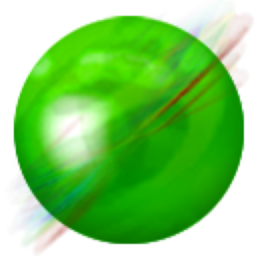
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
