


AES encryption and decryption implementation for PHP, Java, Net and Javascript
# PHP article
<?php $privateKey = "1234567812345678"; $iv = "1234567812345678"; $data = "Test String"; //加密 $encrypted = mcrypt_encrypt(MCRYPT_RIJNDAEL_128, $privateKey, $data, MCRYPT_MODE_CBC, $iv); echo '<br/>'; echo(base64_encode($encrypted)); echo '<br>'; //解密 $encryptedData = base64_decode("2fbwW9+8vPId2/foafZq6Q=="); $decrypted = mcrypt_decrypt(MCRYPT_RIJNDAEL_128, $privateKey, $encryptedData, MCRYPT_MODE_CBC, $iv); echo($decrypted); ?>
#Javascript article
<script type="text/javascript" src="aes.js"></script> <script type="text/javascript" src="pad-zeropadding.js"></script> <script type="text/javascript">         var data = "Test String";         var key  = CryptoJS.enc.Latin1.parse('1234567812345678');         var iv   = CryptoJS.enc.Latin1.parse('1234567812345678');         //加密         var encrypted = CryptoJS.AES.encrypt(data,key,{iv:iv,mode:CryptoJS.mode.CBC,padding:CryptoJS.pad.ZeroPadding});         document.write(encrypted.ciphertext);         document.write('<br/>');         document.write(encrypted.key);         document.write('<br/>');         document.write(encrypted.iv);         document.write('<br/>');         document.write(encrypted.salt);         document.write('<br/>');         document.write(encrypted);         document.write('<br/>');         //解密         var decrypted = CryptoJS.AES.decrypt(encrypted,key,{iv:iv,padding:CryptoJS.pad.ZeroPadding});         console.log(decrypted.toString(CryptoJS.enc.Utf8));     </script>
# Java article
import javax.crypto.Cipher; import javax.crypto.spec.IvParameterSpec; import javax.crypto.spec.SecretKeySpec; import org.junit.Test; @Test public void testCrossLanguageEncrypt() throws Exception{ System.out.println(encrypt()); System.out.println(desEncrypt()); } public static String encrypt() throws Exception { try { String data = "Test String"; String key = "1234567812345678"; String iv = "1234567812345678"; Cipher cipher = Cipher.getInstance("AES/CBC/NoPadding"); int blockSize = cipher.getBlockSize(); byte[] dataBytes = data.getBytes(); int plaintextLength = dataBytes.length; if (plaintextLength % blockSize != 0) { plaintextLength = plaintextLength + (blockSize - (plaintextLength % blockSize)); } byte[] plaintext = new byte[plaintextLength]; System.arraycopy(dataBytes, 0, plaintext, 0, dataBytes.length); SecretKeySpec keyspec = new SecretKeySpec(key.getBytes(), "AES"); IvParameterSpec ivspec = new IvParameterSpec(iv.getBytes()); cipher.init(Cipher.ENCRYPT_MODE, keyspec, ivspec); byte[] encrypted = cipher.doFinal(plaintext); return new sun.misc.BASE64Encoder().encode(encrypted); } catch (Exception e) { e.printStackTrace(); return null; } } public static String desEncrypt() throws Exception { try { String data = "2fbwW9+8vPId2/foafZq6Q=="; String key = "1234567812345678"; String iv = "1234567812345678"; byte[] encrypted1 = new sun.misc.BASE64Decoder().decodeBuffer(data); Cipher cipher = Cipher.getInstance("AES/CBC/NoPadding"); SecretKeySpec keyspec = new SecretKeySpec(key.getBytes(), "AES"); IvParameterSpec ivspec = new IvParameterSpec(iv.getBytes()); cipher.init(Cipher.DECRYPT_MODE, keyspec, ivspec); byte[] original = cipher.doFinal(encrypted1); String originalString = new String(original); return originalString; } catch (Exception e) { e.printStackTrace(); return null; } }
# .Net article
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Security.Cryptography; namespace test { class Class1 { static void Main(string[] args) { Console.WriteLine("I am comming"); String source = "Test String"; String encryptData = Class1.Encrypt(source, "1234567812345678", "1234567812345678"); Console.WriteLine("=1=="); Console.WriteLine(encryptData); Console.WriteLine("=2=="); String decryptData = Class1.Decrypt("2fbwW9+8vPId2/foafZq6Q==", "1234567812345678", "1234567812345678"); Console.WriteLine(decryptData); Console.WriteLine("=3=="); Console.WriteLine("I will go out"); } public static string Encrypt(string toEncrypt, string key, string iv) { byte[] keyArray = UTF8Encoding.UTF8.GetBytes(key); byte[] ivArray = UTF8Encoding.UTF8.GetBytes(iv); byte[] toEncryptArray = UTF8Encoding.UTF8.GetBytes(toEncrypt); RijndaelManaged rDel = new RijndaelManaged(); rDel.Key = keyArray; rDel.IV = ivArray; rDel.Mode = CipherMode.CBC; rDel.Padding = PaddingMode.Zeros; ICryptoTransform cTransform = rDel.CreateEncryptor(); byte[] resultArray = cTransform.TransformFinalBlock(toEncryptArray, 0, toEncryptArray.Length); return Convert.ToBase64String(resultArray, 0, resultArray.Length); } public static string Decrypt(string toDecrypt, string key, string iv) { byte[] keyArray = UTF8Encoding.UTF8.GetBytes(key); byte[] ivArray = UTF8Encoding.UTF8.GetBytes(iv); byte[] toEncryptArray = Convert.FromBase64String(toDecrypt); RijndaelManaged rDel = new RijndaelManaged(); rDel.Key = keyArray; rDel.IV = ivArray; rDel.Mode = CipherMode.CBC; rDel.Padding = PaddingMode.Zeros; ICryptoTransform cTransform = rDel.CreateDecryptor(); byte[] resultArray = cTransform.TransformFinalBlock(toEncryptArray, 0, toEncryptArray.Length); return UTF8Encoding.UTF8.GetString(resultArray); } } }
The requirements for cross-language encryption and decryption are: AES/CBC/ZeroPadding 128-bit mode, the key and iv are the same, and the encoding is unified Use utf-8. If ZeroPadding is not supported, use NoPadding.
The above introduces the AES encryption and decryption implementation of PHP, Java, Net and Javascript, including the relevant content. I hope it will be helpful to friends who are interested in PHP tutorials.
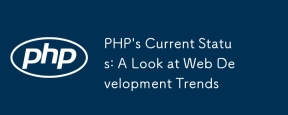
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
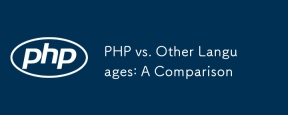
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
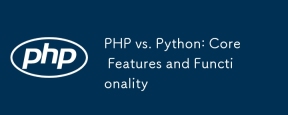
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
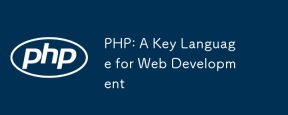
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
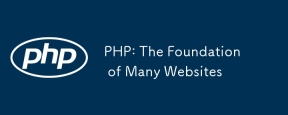
The reasons why PHP is the preferred technology stack for many websites include its ease of use, strong community support, and widespread use. 1) Easy to learn and use, suitable for beginners. 2) Have a huge developer community and rich resources. 3) Widely used in WordPress, Drupal and other platforms. 4) Integrate tightly with web servers to simplify development deployment.
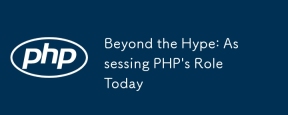
PHP remains a powerful and widely used tool in modern programming, especially in the field of web development. 1) PHP is easy to use and seamlessly integrated with databases, and is the first choice for many developers. 2) It supports dynamic content generation and object-oriented programming, suitable for quickly creating and maintaining websites. 3) PHP's performance can be improved by caching and optimizing database queries, and its extensive community and rich ecosystem make it still important in today's technology stack.
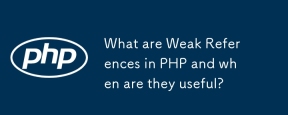
In PHP, weak references are implemented through the WeakReference class and will not prevent the garbage collector from reclaiming objects. Weak references are suitable for scenarios such as caching systems and event listeners. It should be noted that it cannot guarantee the survival of objects and that garbage collection may be delayed.
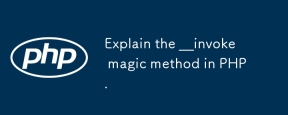
The \_\_invoke method allows objects to be called like functions. 1. Define the \_\_invoke method so that the object can be called. 2. When using the $obj(...) syntax, PHP will execute the \_\_invoke method. 3. Suitable for scenarios such as logging and calculator, improving code flexibility and readability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
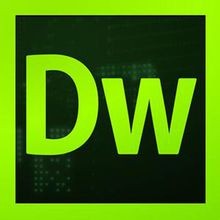
Dreamweaver CS6
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.