


Summary of some techniques for deeply customizing Python's Flask framework development environment
Flask environment configuration
Your application may require a large number of packages to work properly. If you don't need the Flask package, you may have read the wrong tutorial. Your application's environment is basically the foundation for everything that happens when your application is running. We are lucky because there are many ways in which we can easily manage our environment.
Use virtualenv to manage your environment
virtualenv is a tool for isolating your applications in so-called virtual environments. A virtual environment is a directory that contains software that your application depends on. A virtual environment can also change your environment variables to maintain the environment variables contained in your development environment. Instead of downloading packages, like Flask, into your system-level or user-level packages directory, we can download them into a separate directory that is only used by our application. This makes it easy to specify the version of Python used and the packages each project depends on.
Virtualenv also allows you to use different versions of the same package in different projects. This flexibility can be important if you are using an older system and have several projects on it that require different versions.
When using virtualenv, you usually only need to install a few Python packages on your system. One of them is virtualenv itself. You can use Pip to install virtualenv packages.
Once virtualenv is installed on your system, you can start creating virtual environments. Go to the directory where your project is located and run the virtualenv command. It requires one parameter, which is the target directory of the virtual environment. Here's an overview of what it looks like.
$ virtualenv venv New python executable in venv/bin/python Installing Setuptools...........[...].....done. Installing Pip..................[...].....done.
virtualenv creates a new directory, and dependent packages will be installed in this directory.
Once the new virtual environment has been created, you must activate it by launching the bin/activate script created within the virtual environment.
$ which python /usr/local/bin/python $ source venv/bin/activate (venv)$ which python /Users/robert/Code/myapp/venv/bin/python
The bin/activate script makes some changes to your shell environment variables so that everything points to the new virtual environment instead of the global system. You can see the effect in the code block above. After activation, the python command points to the Python bin directory in the virtual environment. When the virtual environment is activated, dependency packages installed using Pip will be downloaded to the virtual environment instead of the global system.
You may notice that the prompt in the shell has also changed. virtualenv pre-sets the name of the currently active virtual environment, so you know you are not working on the global system.
You can deactivate your virtual environment by running the deactivate command.
(venv)$ deactivate
virtualenvwrapper
virtualenvwrapper is a package for managing virtual environments created by virtualenv. I don't want to mention this tool until you've seen the basics of virtualenv so you understand what it improves and why we should use it.
The virtual environment directory created in the previous section will cause some clutter in your project library. You only need to activate the virtual environment to interact with it, but it should not appear in version control, so the virtual environment directory should not be here. The solution is to use virtualenvwrapper. This package will put all your virtual environments in one directory, usually in ~/.virtualenvs/ by default.
To install virtualenvwrapper, follow the instructions in the documentation, located at http://virtualenvwrapper.readthedocs.org/en/latest/install.html.
Please make sure you have disabled all virtual environments before installing virtualenvwrapper. You need to install it globally, not in a virtual environment.
Now, instead of running virtualenv to create an environment, you need to run mkvirtualenv:
$ mkvirtualenv rocket New python executable in rocket/bin/python Installing setuptools...........[...].....done. Installing pip..................[...].....done. (rocket)$
Install dependency packages
As the project develops, you will find that the list of dependent packages will grow. It's not uncommon to need dozens of Python packages to run a Flask application. The easiest way to manage these is with a simple text file. Pip can generate a text file listing all installed packages. On a new system, or on a newly created environment, it is also possible to read the list in the file and install each of them.
(rocket)$ pip freeze > requirements.txt $ workon fresh-env (fresh-env)$ pip install -r requirements.txt [...] Successfully installed flask Werkzeug Jinja2 itsdangerous markupsafe Cleaning up... (fresh-env)$
随着项目的发展,你可能会发现 pip freeze 列出的某些包实际上并不是运行应用必须的。你安装这些包仅仅为开发用的。pip freeze 并不能区分,它仅仅列出目前已经安装的包。因此,你可能要手动地管理这些依赖包。你可以分别把那些运行应用必须的包放入 require\_run.txt 以及那些开发应用程序需要的包放入 require\_dev.txt 。
版本控制
选择一个版本控制系统并且使用它。我推荐 Git。从我所看到的,Git 是这些天来新项目最流行的选择。能够删除代码而不必担心犯了一个不可逆转的错误是非常宝贵的。你也可以让你的项目摆脱大量注释掉的代码的困扰,因为你可以删除它们,以后如有需要可以恢复它们。另外,你可以在 GitHub,Bitbucket 或者你自己的 Gitolite 服务器上备份整个项目。
置身版本控制之外的文件
我通常会让一个文件置身版本控制之外有两个原因:要么就是它会让整个项目显得混乱,要么它就是一个很隐私的密钥/证书。编译的 .pyc 文件和虚拟环境 — 如果由于某些原因你没有使用 virtualenvwrapper — 就是让项目显得很混乱的例子。它们不需要在版本控制之中因为它们能够分别地从 .py 文件和你的 requirements.txt 文件重新创建。
API 秘钥,应用程序秘钥以及数据库证书是很隐私的密钥/证书的示例。它们不应该出现在版本控制中因为它们的曝光将是一个巨大的安全漏洞。
当做跟安全有关的决定的时候,我总是喜欢假设我的版本库将在某个时候变成公开的。这就意味着要保守秘密并且从不假设一个安全漏洞不会被发现,“谁来猜猜他们能做到”这类型的假设被称为通过隐匿来实现安全,这是十分槽糕的策略。
当使用 Git 的时候,你可以在你的版本库中创建名为 .gitignore 的一个特殊文件。在这个文件里,使用列表通配符来匹配文件名。任何匹配其中一个模式的文件名都会被 Git 给忽略掉。我推荐使用 .gitignore 来控制不需要版本控制的文件。例如:
*.pyc instance/
你可以阅读更多的关于 .gitignore 的内容从这里: http://git-scm.com/docs/gitignore
调试
1.调试模式
Flask 有一个称为调试模式方便的功能。要打开调试功能的话,你只必须在你的开发配置中设置 debug = True。当它打开的时候,服务器会在代码变化的时候自动加载并且出错的时候会伴随着一个堆栈跟踪和一个交互式控制台。
小心!不要在生产环境中使用调试模式。交互式控制台允许执行任意代码并会是一个巨大的安全漏洞。
2.Flask-DebugToolbar
Flask-DebugToolbar 是另一个非常了不起的工具,它可以帮助在你的应用程序中调试问题。在调试模式下,它会把一个侧边栏置于你的应用程序的每一页上。侧边栏提供了有关 SQL 查询,日志记录,版本,模板,配置和其它有趣的信息,使得更容易地跟踪问题。
看看快速入门中的 调试模式。在 Flask 官方文档 中有一些关于错误处理,日志记录以及使用调试器等不错的信息。
Flask 工程配置
当你学习 Flask 的时候,配置看起来很简单。你只要在 config.py 中定义一些变量接着一切就能工作了。当你开始必须要管理生产应用的配置的时候,这些简单性开始消失了。你可能需要保护 API 密钥以及为不同的环境使用不同的配置(例如,开发和生产环境)。在本章节中我们会介绍 Flask 一些先进的功能,它可以使得管理配置容易些。
简单的例子
一个简单的应用程序可能不会需要任何这些复杂的功能。你可能只需要把 config.py 放在你的仓库/版本库的根目录并且在 app.py 或者 yourapp/\\_init\\_.py 中加载它。
config.py 文件中应该每行包含一个配置变量赋值。当你的应用程序初始化的时候,在 config.py 中的配置变量用于配置 Flask 和它的扩展并且它们能够通过 app.config 字典访问到 – 例如,app.config["DEBUG"]。
DEBUG = True # Turns on debugging features in Flask BCRYPT_LEVEL = 12 # Configuration for the Flask-Bcrypt extension MAIL_FROM_EMAIL = "robert@example.com" # For use in application emails
配置的变量可以被 Flask,它的扩展或者你来使用。这个例子中, 每当我们在一封事务性邮件中需要默认的 “发件人” 的时候,我们可以使用 app.config["MAIL_FROM_EMAIL"] – 例如,密码重置。把这些信息放置于一个配置变量中使得以后能够容易地修改它。
# app.py or app/__init__.pyfrom flask import Flask app = Flask(__name__) app.config.from_object('config') # Now we can access the configuration variables via app.config["VAR_NAME"].
- DEBUG: 为你提供了调试错误的一些方便的工具。 这包括一个基于 Web 的堆栈跟踪和交互式的 Python 控制台。在开发环境中设置成 True; 生产环境中设置成 False。
- SECRET\_KEY:这是 Flask 用来为 cookies 签名的密钥。 它也能被像 Flask-Bcrypt 类的扩展使用。 你应该在你的实例文件夹中定义它, 这样可以远离版本控制。 你可以在下一个章节中阅读更多关于示例文件夹的内容。一般情况下这应该是一个复杂的随机值。
- BCRYPT\_LEVEL:如果你使用 Flask-Bcrypt 来散列用户密码的话, 你需要指定一个“循环”数,这个数是在执行散列密码的 算法需要的。如果你不使用 Flask-Bcrypt,你可以 忽略这里。用于散列密码的循环数越大,攻击者猜测密码 的时间会越长。同时,循环数越大会增加散列密码的时间。后面我们会给出在 Flask 应用中 使用 Bcrypt 的一些最佳实践。
确保在生产环境中 DEBUG 设置成 False。如果保留 DEBUG 为 True,它允许用户在你的服务器上执行任意的 Python。
实例文件夹
有时候你需要定义包含敏感信息的配置变量。我们想要从 config.py 中分离这些变量并且让它们保留在仓库/版本库之外。你可能会隐藏像数据库密码以及 API 密钥的一些敏感信息,或者定义于特定于指定机器的配置变量。为让实现这些要求更加容易些,Flask 提供了一个叫做 instance folders 的功能。实例文件夹是仓库/版本库下的一个子目录并且包含专门为这个应用程序的实例的一个配置文件。我们不希望它提交到版本控制。
config.py requirements.txt run.py instance/ config.py yourapp/ __init__.py models.py views.py templates/ static/
使用实例文件夹
我们使用 app.config.from_pyfile() 来从一个实例文件夹中加载配置变量。当我们调用 Flask() 来创建我们的应用的时候,如果我们设置了 instance_relative_config=True, app.config.from_pyfile() 将会从 instance/ 目录加载指定文件。
# app.py or app/__init__.py app = Flask(__name__, instance_relative_config=True) app.config.from_object('config') app.config.from_pyfile('config.py')
现在我们可以像在 config.py 中那样在 instance/config.py 中定义配置变量。你也应该把你的实例文件夹加入到版本控制系统的忽略列表中。要使用 Git 做到这一点的话,你需要在 .gitignore 新的一行中添加 instance/ 。
密钥
实例文件夹的隐私性成为在其里面定义不想暴露到版本控制的密钥的最佳候选。这些密钥可能包含了你的应用的密钥或者第三方 API 密钥。如果你的应用是开源的或者以后可能会公开的话,这一点特别重要。我们通常要求其他用户或者贡献者使用自己的密钥。
# instance/config.py SECRET_KEY = 'Sm9obiBTY2hyb20ga2lja3MgYXNz' STRIPE_API_KEY = 'SmFjb2IgS2FwbGFuLU1vc3MgaXMgYSBoZXJv' SQLALCHEMY_DATABASE_URI= \\"postgresql://user:TWljaGHFgiBCYXJ0b3N6a2lld2ljeiEh@localhost/databasename"
基于环境的配置
如果在你的生产环境和开发环境中的差异非常小的话,你可能想要使用实例文件夹来处理配置的变化。定义在 'instance/config.py' 文件中的配置变量能够覆盖 'config.py' 中的值。你只需要在 'app.config.from_object()' 后调用 'app.config.from_pyfile()'。这样用法的好处之一就是在不同的机器上修改你的应用的配置。
# config.py DEBUG = False SQLALCHEMY_ECHO = False # instance/config.py DEBUG = True SQLALCHEMY_ECHO = True
在生产环境上,我们略去上面 'instance/-config.py' 中的配置变量,它会退回到定义在 'config.py' 中的值。
了解更多关于 Flask-SQLAlchemy 的 配置项。(中文版的位于:http://www.pythondoc.com/flask-sqlalchemy/config.html#configuration-keys)
基于环境变量配置
实例文件夹不应该出现在版本控制中。这就意味着你将无法跟踪你的实例配置的变化。如果只是一、两个变量这可能不是什么问题,但是如果你在不同的环境上(生产,预升级,开发,等等)配置都有些微调话,你就不会想要存在丢失它们的风险。
Flask 给我们选择配置文件的能力,它可以基于一个环境变量的值来加载不同的配置文件。这就意味着在我们的仓库/版本库里,我们可以有多个配置文件并且总会加载正确的那一个。一旦我们有多个配置文件的话,我可以把它们移入它们自己 config 文件夹中。
requirements.txt run.py config/ __init__.py # Empty, just here to tell Python that it's a package. default.py production.py development.py staging.py instance/ config.py yourapp/ __init__.py models.py views.py static/ templates/
在上面的文件列表中我们有多个不同的配置文件。
- config/default.py: 默认的配置值,可用于所有的环境或者被个人的环境给覆盖。
- config/development.py: 用于开发环境的配置值。这里你可能会指定本地数据库的 URI。
- config/production.py: 用于生产环境的配置值。在这里 DEBUG 一定要设置成 False。
- config/staging.py: 根据开发进度,你可能会有一个模拟生产环境,这个文件主要用于这种场景。
为了决定要加载哪个配置文件,我们会调用 'app.config.from_envvar()'。
# yourapp/\\_\\_init\\_\\_.py app = Flask(__name__, instance_relative_config=True) # Load the default configuration app.config.from_object('config.default') # Load the configuration from the instance folder app.config.from_pyfile('config.py') # Load the file specified by the APP\\_CONFIG\\_FILE environment variable# Variables defined here will override those in the default configuration app.config.from_envvar('APP_CONFIG_FILE')
环境变量的值应该是配置文件的绝对路径。
我们如何设置这个环境变量取决于我们运行应用所在的平台。如果我们运行在一个普通的 Linux 服务器上,我们可以编写一个设置环境变量的 shell 脚本并且运行 run.py。
# start.sh APP\\_CONFIG\\_FILE=/var/www/yourapp/config/production.py python run.py
start.sh 对于每一个环境都是独一无二的,因此它应该被排除在版本控制之外。在 Heroku 上,我们需要使用 Heroku 工具来设置环境变量。这种设置方式也适用于其它的 PaaS 平台。
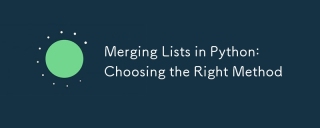
TomergelistsinPython,youcanusethe operator,extendmethod,listcomprehension,oritertools.chain,eachwithspecificadvantages:1)The operatorissimplebutlessefficientforlargelists;2)extendismemory-efficientbutmodifiestheoriginallist;3)listcomprehensionoffersf
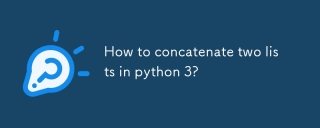
In Python 3, two lists can be connected through a variety of methods: 1) Use operator, which is suitable for small lists, but is inefficient for large lists; 2) Use extend method, which is suitable for large lists, with high memory efficiency, but will modify the original list; 3) Use * operator, which is suitable for merging multiple lists, without modifying the original list; 4) Use itertools.chain, which is suitable for large data sets, with high memory efficiency.
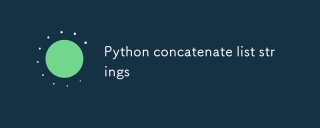
Using the join() method is the most efficient way to connect strings from lists in Python. 1) Use the join() method to be efficient and easy to read. 2) The cycle uses operators inefficiently for large lists. 3) The combination of list comprehension and join() is suitable for scenarios that require conversion. 4) The reduce() method is suitable for other types of reductions, but is inefficient for string concatenation. The complete sentence ends.
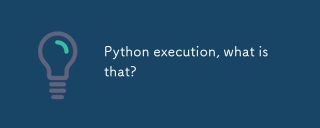
PythonexecutionistheprocessoftransformingPythoncodeintoexecutableinstructions.1)Theinterpreterreadsthecode,convertingitintobytecode,whichthePythonVirtualMachine(PVM)executes.2)TheGlobalInterpreterLock(GIL)managesthreadexecution,potentiallylimitingmul
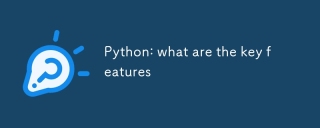
Key features of Python include: 1. The syntax is concise and easy to understand, suitable for beginners; 2. Dynamic type system, improving development speed; 3. Rich standard library, supporting multiple tasks; 4. Strong community and ecosystem, providing extensive support; 5. Interpretation, suitable for scripting and rapid prototyping; 6. Multi-paradigm support, suitable for various programming styles.
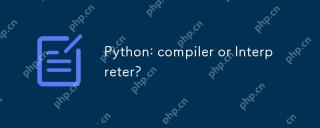
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
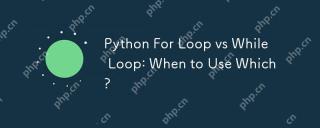
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
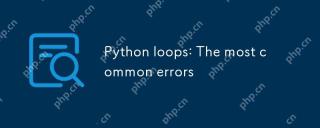
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
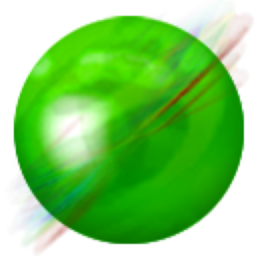
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
