This article refers to the content of Chapter 4 of "Practical Development of PHP Top Framework zendframe" and fully implements it...
First download the css file used: http://download.csdn.net/download/unityoxb/4058802
After decompression, copy the default and common files to the public/skins directory;
1. The database file used mysql.sql
create table if not exists `core_pages`( `id` int(10) unsigned not null auto_increment comment '页面唯一ID', `cid` int(10) unsigned not null default '0' comment '分类ID', `uid` int(10) unsigned not null default '0' comment '用户ID', `title` varchar(255) not null comment '页面标题', `body` text not null comment '内容', `status` tinyint(4) not null default '1' comment '是否发布', `createtime` int(11) not null default '0' comment '创建页面时间', `updatetime` int(11) not null default '0' comment '修改页面时间', `comment` tinyint(4) not null default '0' comment '页面是否评论功能', `start` tinyint(4) not null default '0' comment '页面级别', `top` tinyint(4) not null default '0' comment '置顶', primary key (`id`) )ENGINE=InnoDB default charset=utf8;
Open mysql and use source mysql.sql to create the table structure
2. Configure the application.ini file (hahacom/applicaton/configs)
Mainly configure the zend Framework to connect to mysql
[development : production] phpSettings.display_startup_errors = 1 phpSettings.display_errors = 1 resources.frontController.params.displayExceptions = 1 resources.db.adapter = "PDO_MYSQL" resources.db.params.host = "localhost" resources.db.params.username = "root" resources.db.params.password = "root" resources.db.params.dbname = "test" --这是数据名称 resources.db.isDefaultTableAdapter = "TRUE" resources.db.params.driver_options.1002 = "SET NAMES UTF8;"
3. Public/index.php
// Define application environment defined('APPLICATION_ENV') || define('APPLICATION_ENV', (getenv('APPLICATION_ENV') ? getenv('APPLICATION_ENV') : '<strong>development</strong>')); //修改成测试环境
4. Create an article display model (the model mainly stores data and the model is similar javabean, or get data from the database and save it in memory)
Execute the command: zf create model page
A models/Page.php file will be automatically generated
<?php class Application_Model_Page { protected $_name = 'core_pages'; public $result; public function getPage($where = array()) { $db = Zend_Db_Table::getDefaultAdapter(); // $db = $this->getAdapter(); $select = $db->select(); /*if($where != null) { //$select->where(' star = ? ', $where); //$sql = $db->quoteInto("select * from `core_pages` where `star`= ?", $where); //$result = $db->query($sql); $select->from('core_pages','*')->where('star = ?', $where)->limit(1); }*/ $select->from('core_pages','*'); if(count($where)>0) { foreach($where as $key=>$value) $select->where($key.' = ?',$value); } //$row = $result->fetch(); $row = $db->fetchAll($select); if($row) { return $row; } else { echo "================="; return null; } } public function getPages($where = null) { $db = Zend_Db_Table::getDefaultAdapter(); if(is_numeric($where)) { //$row = $db->find($where)->current(); $select = $db->select(); $select->from('core_pages','*'); $select->where('id = ?', $where); $row = $db->fetchRow($select); } if(is_array($where) && count($where)>0) { $select = $db->select(); $select->from('core_pages','*'); foreach($where as $key=>$value){ $select->where($key.'=?', $value); } $row = $db->fetchAll($select); } if($row) { return $row; } else { echo "================="; return null; } } } ?>
5. Create a controller
Execute the command: zf create controller news will automatically generate controllers/NewsController.php
<?php class NewsController extends Zend_Controller_Action { public function init() { /* Initialize action controller here */ } public function indexAction() { // action body $modelPage = new Application_Model_Page(); //$star = 1; $where = array('top'=>1, 'comment'=>1); $newsStar = $modelPage->getPage($where); //print_r($newsStar); $this->view->News = $newsStar; //$this->view->name = "hahaha"; } }
Execute the command: zf create controller page and zf create action detail page
will automatically generate controllers/PageController.php
<?php class PageController extends Zend_Controller_Action { public function init() { /* Initialize action controller here */ } public function indexAction() { // action body } public function detailAction() { // action body $id = $this->_request->getParam('id'); $modelPage = new Application_Model_Page($id); //if($modelPage == null) //print_r('=============================='); //print_r($id); //print_r($modelPage); $page = $modelPage->getPages($id); $this->view->page = $page; } }
5. Next create the view file
/ views/scripts/news/index.phtml
<?php echo "<h3>".$this->News[0]['title'].""; echo $this->News[0]['body']; //echo $this->name; if($this->News) { /*echo "
- ";
// print_r($this->News);
foreach($this->News as $val)
{
echo "
- "."".$val['title'].""." "; } echo "
- ";
echo $this->partialLoop('row_pages.phtml', $this->News);
echo "
/views/scripts/row_pages.phtml
/views/scripts/page/detail.phtml
<?php echo "<h2>".$this->page['title'].""; echo "发表:".date('Y-m-d', $this->page['createtime']).""; echo "<hr>"; echo $this->page['body']; ?>
Running screenshot:
Click the link:
Copyright Statement: This article is an original article by the blogger and may not be reproduced without the blogger's permission.
The above introduces PHP programming examples using zendframework, including aspects of content. I hope it will be helpful to friends who are interested in PHP tutorials.

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
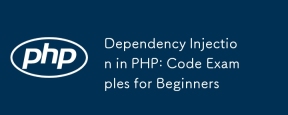
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
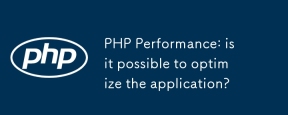
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
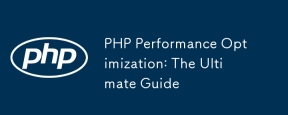
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
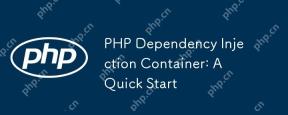
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
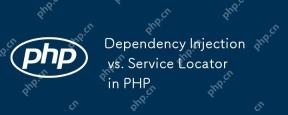
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
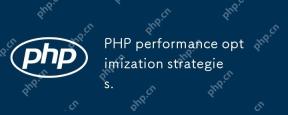
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
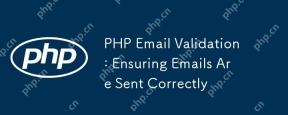
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
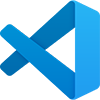
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
