The null coalescing operator is a good thing. With it, we can easily get a parameter and provide a default value when it is empty. For example, in js, you can use || to do:
function setSomething(a){ a = a || 'some-default-value'; // ... }
. But in PHP, unfortunately, PHP's || always returns true or false, so you can't do it this way.
PHP7 has just officially added this operator:
// 获取user参数的值(如果为空,则用'nobody') $username = $_GET['user'] ?? 'nobody'; // 等价于: $username = isset($_GET['user']) ? $_GET['user'] : 'nobody';
It is estimated that PHP7 will take a long time to be used in the production environment. So are there any alternatives in the current PHP5?
According to research, there is a very convenient alternative:
// 获取user参数的值(如果为空,则用'nobody') $username = @$_GET['user'] ?: 'nobody'; // 等价于: $username = isset($_GET['user']) ? $_GET['user'] : 'nobody';
-- Run this code: https://3v4l.org/aDUW8
Look at it with wide eyes, it is similar to the previous PHP7 example, The main thing is to replace ?? with ?:. What the hell is this? In fact, this is the omission pattern of (expr1) ? (expr2) : (expr3) expression:
Expression (expr1) ? (expr2) : (expr3) When expr1 evaluates to TRUE, the value is expr2, and when expr1 evaluates to The value when FALSE is expr3.
Since PHP 5.3, you can omit the middle part of the ternary operator. The expression expr1 ?: expr3 returns expr1 if expr1 evaluates to TRUE and expr3 otherwise.
-- http://php.net/manual/zh/language.operators.comparison.php
Of course, this alternative is not perfect - if there is no 'user' in $_GET, there will be a Notice : Undefined index: user error, so you need to use @ to suppress this error, or turn off the E_NOTICE error.
ps: PHP7 null coalescing operator Say goodbye to isset()
the previous way of writing
$info = isset($_GET['email']) ? $_GET['email'] : ‘noemail';
Now you can just write it like this
$info = $_GET['email'] ?? noemail;
You can also write it in conjunction like this
$info = $_GET['email'] ?? $_POST['email'] ?? ‘noemail';
The above has introduced a detailed explanation of the null coalescing operator in PHP, including relevant aspects. I hope it will be helpful to friends who are interested in PHP tutorials.
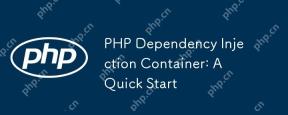
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
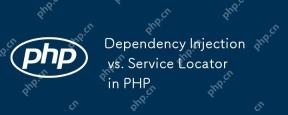
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
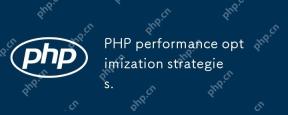
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
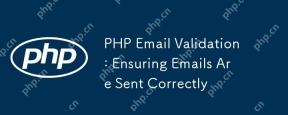
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
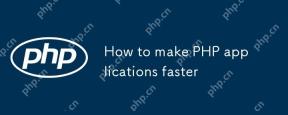
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
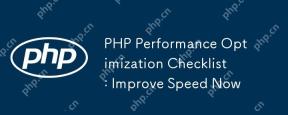
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
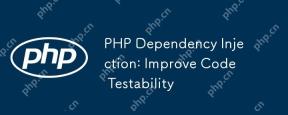
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
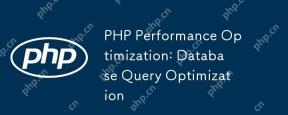
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
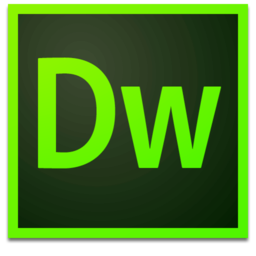
Dreamweaver Mac version
Visual web development tools
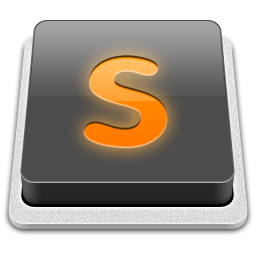
SublimeText3 Mac version
God-level code editing software (SublimeText3)
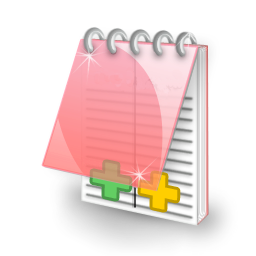
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
