1. Inheritance destroys encapsulation.
2. Inheritance is tightly coupled.
3. Inheritance and extension are complicated.
4. Improper use of inheritance may violate real-world logic. When creating a combined object, the combination needs to create local objects one by one, which adds some code to a certain extent, but inheritance does not require this step, because the subclass automatically has the methods of the parent class. How to use inheritance:
1. Carefully design it specifically for the inherited class. The abstraction layer of the inheritance tree should be relatively stable, generally no more than three layers.
2. For classes that are not specifically designed to be inherited, prohibit them from being inherited, that is, use the final modifier. Using the final modifier can not only prevent important methods from being illegally overwritten, but also give the editor opportunities to find optimizations.
3. Prioritize using combination relationships to improve code reusability.
4. The subclass is a special type, not just a role of the parent class.
5. Subclass extensions, rather than overriding or invalidating the functions of the parent class.
6. The bottom code mostly uses combination, and the top-level/business layer code mostly uses inheritance. Using combination at the bottom level can improve efficiency and avoid bloated objects. Using inheritance for top-level code can improve flexibility and make business use more convenient. Multiple inheritance mechanism: Traits. Polymorphism:
Meaning: When objects of the same class receive the same message, they will get different results. And the news was unpredictable.
Overloading is not something in object-oriented, and it is not the same concept as polymorphism. It is a form of polymorphism.
Polymorphism is a programming way of describing the same object through multiple states or phases. Its true meaning is: in actual development, you only need to care about the programming of an interface or base class, and do not have to care about the specific class to which an object belongs. Implementation of polymorphism:
1. Polymorphism refers to the reification of the same type of objects at runtime.
2. The PHP language is weakly typed, making it easier and more flexible to implement polymorphism.
3. Type conversion is not polymorphism.
4. In PHP, the parent class and the child class are regarded as the "stepfather" and "stepson" relationships. They have an inheritance relationship, but there is no blood relationship. Therefore, the subclass cannot be transformed upward into the parent class, thereby losing the most typical feature of polymorphism.
5. The essence of polymorphism is if...else, but the level of implementation is different. Interface defines a set of specifications to describe the functions of a "thing", requiring that if the "thing" in reality wants to be usable, it must implement these basic functions. Abstract classes and interfaces. In a sense, Traits and interfaces are both a disguised implementation of "multiple inheritance". Several concepts of interfaces:
1. Interfaces exist as a specification and contract. As a specification, the interface should ensure availability; as a contract, the interface should ensure controllability.
2. An interface is just a declaration, once you use the interface keyword, you should implement it. It can be implemented by the programmer (external interface) or by the system (internal interface). The interface itself does nothing, but it can tell us what it can do.
3. There are two shortcomings in the interfaces in PHP. One is that there are no contract restrictions, and the other is that there are not enough internal interfaces. Reflection:
In object-oriented programming, objects are given the ability to introspect, and this introspection process is reflection.
Reflection, intuitive understanding is to find the departure point and source based on the arrival place.
Reflection refers to extending the analysis of PHP programs in the running state of PHP, exporting or extracting detailed information about classes, methods, properties, parameters, etc., including comments. This function of dynamically obtaining information and dynamically calling object methods is called reflection API.
The role of reflection: can be used for document generation. (hook?)
Its uses include: automatically loading plug-ins, automatically generating documents, and can even be used to expand the PHP language
The purpose of reflection is to expand unknown applications
Exception and error handling:
The concepts of exception and error are different.
The above introduces the PHP study notes - the core concepts of object-oriented, including aspects of the content. I hope it will be helpful to friends who are interested in PHP tutorials.
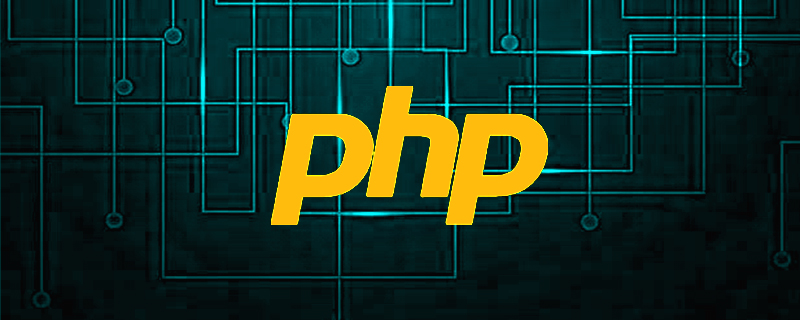
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
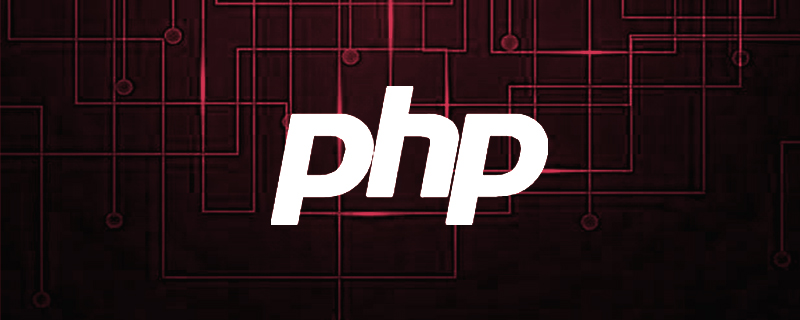
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
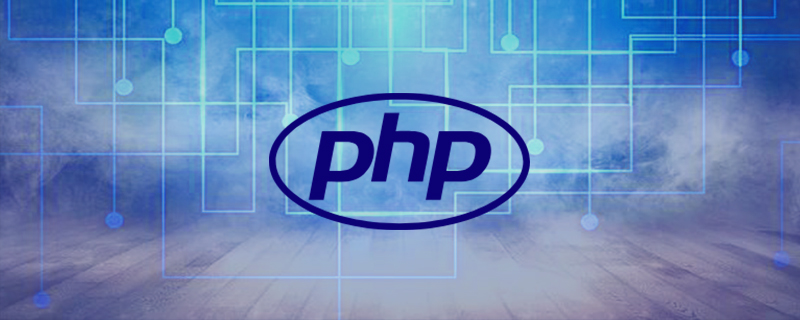
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
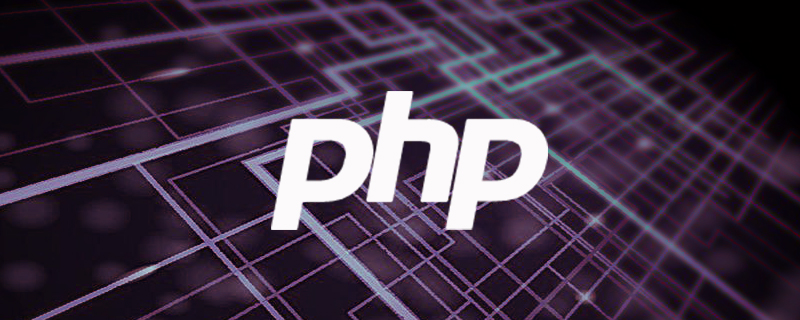
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
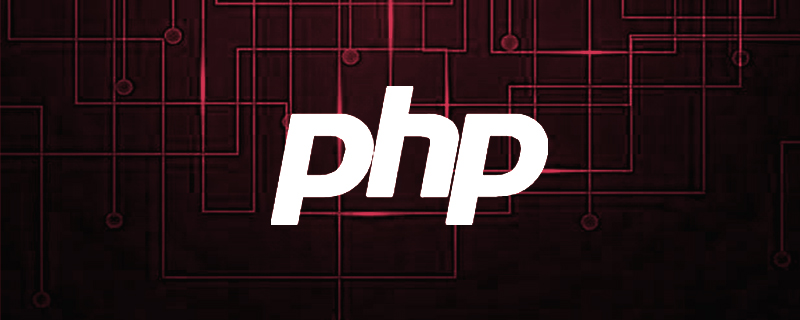
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
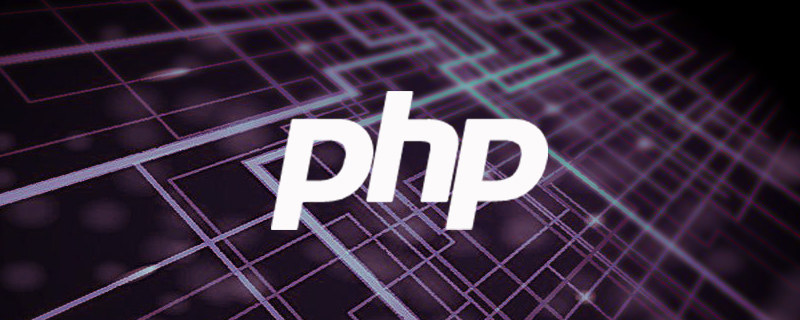
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
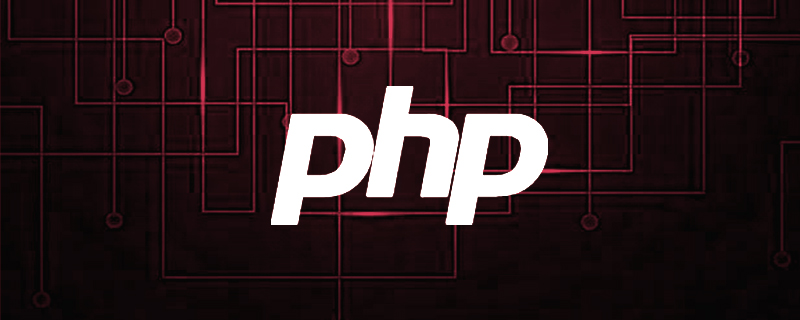
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
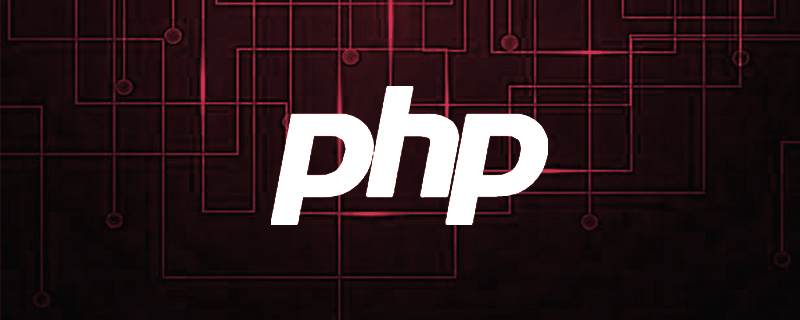
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
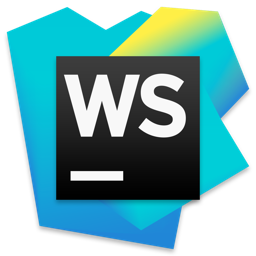
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
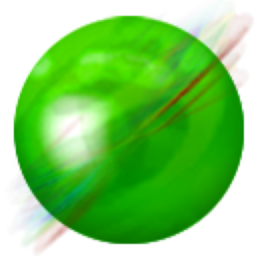
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
