


以下内容以一个简单的计算器程序作为案例分析。第一步,定义Operation,是一个父类,有两个属性,表示用于计算的两个参数。
<?php /* * 计算类 */ class Operation{ private $numA=0; private $numB=0; public function setNumA($numA) { $this->numA=$numA; } public function getNumA() { return $this->numA; } public function setNumB($numB) { $this->numB=$numB; } public function getNumB() { return $this->numB; } } ?>
The second step is to define an interface, which declares the method to implement the operation
<?php /* *工厂接口 */ interface InterOperate{ function getResult(); } ?>
The third step is an addition operation class (omitting the subtraction class, multiplication class, trigger class, etc.)
<?php /** * 加法运算类 */ include_once "IOperate.php"; include_once 'Operation.php'; class OperationAdd extends Operation implements InterOperate { function getResult() { $result=$this->getNumA()+$this->getNumB(); return $result; } } ?>
Finally, define a simple factory class for creating object instances of various classes. Usually the objects returned by simple factory classes have a common parent class. In this example, the common parent class is the Operation class, and the addition class and the subtraction class are both subclasses of Operation.
<?php include_once "OperationAdd.php"; include_once "OperationMinus.php"; class SimpleFactory { static function createAdd() { return new OperationAdd; } static function createMinus() { return new OperationMinus; } } ?>
The client code is as follows:
<?php /* * 客户端代码 */ include_once "OperationAdd.php"; include_once 'Operation.php'; include_once 'SimpleFactory.php'; $op=SimpleFactory::createAdd(); $op->setNumA(2); $op->setNumB(4); echo $op->getResult(); $om=SimpleFactory::createMinus(); $om->setNumA(45); $om->setNumB(34); echo "<br>"; echo $om->getResult(); ?>
The above introduces the PHP implementation of the simple factory pattern, including the content of the simple factory pattern. I hope it will be helpful to friends who are interested in PHP tutorials.
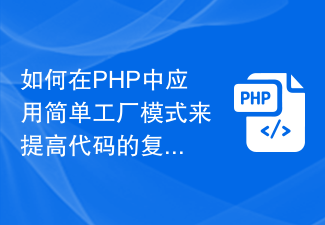
如何在PHP中应用简单工厂模式来提高代码的复用性简单工厂模式(SimpleFactoryPattern)是一种常用的设计模式,可以在创建对象时提供一种统一的接口,以便根据不同的条件来创建不同的实例。这种模式可以有效地降低代码的耦合度,提高代码的可维护性和复用性。在PHP中,我们可以利用简单工厂模式来优化代码的结构和逻辑。理解简单工厂模式简单工厂模式由三个
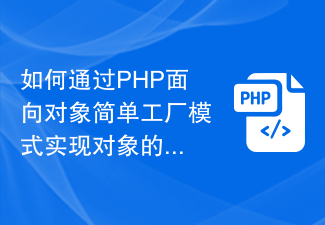
如何通过PHP面向对象简单工厂模式实现对象的版本控制和管理在开发大型的、复杂的PHP项目时,版本控制和管理是非常重要的一环。通过适当的设计模式,我们可以更好地管理和控制对象的创建和使用,从而提高代码的可维护性和扩展性。本文将介绍如何使用PHP面向对象简单工厂模式来实现对象的版本控制和管理。简单工厂模式是一种创建类的设计模式,它通过一个工厂类来实例化指定的对象
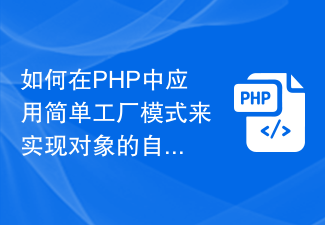
如何在PHP中应用简单工厂模式来实现对象的自动化创建简单工厂模式是一种常见的设计模式,它用于创建对象并抽象了实例化对象的过程。在PHP中,应用简单工厂模式可以帮助我们将对象的创建和具体实现解耦,使代码更加灵活和可维护。在本文中,我们将使用一个示例来说明如何在PHP中应用简单工厂模式。假设我们有一个电子产品店,它销售手机和电视机。我们需要根据用户的选择来创建相
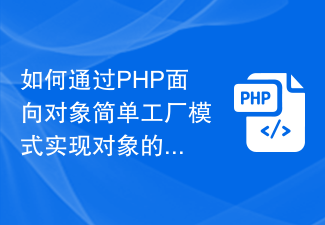
如何通过PHP面向对象简单工厂模式实现对象的多态性简单工厂模式是一种常见的设计模式,它可以通过一个共同的工厂类来创建不同类的对象,并且隐藏了对象的创建过程。PHP面向对象简单工厂模式可以帮助我们实现对象的多态性。简单工厂模式包含三个基本角色:工厂类、抽象类和具体类。首先我们来定义一个抽象类Animal,它包含一个抽象方法say():abstractclas
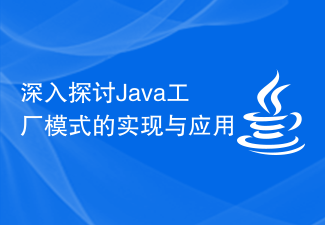
Java工厂模式的原理与应用详解工厂模式是一种常用的设计模式,它用于创建对象,以及将对象的创建过程封装起来。Java中的工厂模式有多种实现方式,其中最常见的有简单工厂模式、工厂方法模式和抽象工厂模式。本文将详细介绍这三种工厂模式的原理和应用,并给出相应的代码示例。一、简单工厂模式简单工厂模式是最简单、最常用的工厂模式。它通过一个工厂类,根据传入的参数来返回不
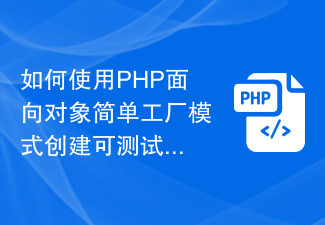
如何使用PHP面向对象简单工厂模式创建可测试的对象实例简单工厂模式是一种常用的软件设计模式,它可以帮助我们根据不同的条件创建不同的对象实例。在PHP面向对象编程中,结合简单工厂模式可以提高代码的可测试性和可维护性。在本文中,我们将学习如何使用PHP面向对象简单工厂模式创建可测试的对象实例。我们将以一个简单的示例来说明这个过程。首先,让我们定义一个接口来表示要
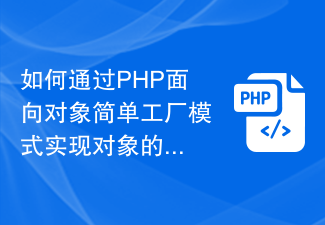
如何通过PHP面向对象简单工厂模式实现对象的封装和隐藏简介:在PHP面向对象编程中,封装是一种实现数据隐藏的重要概念。封装可以将数据和相关的操作封装在一个类中,并通过对外暴露的公共接口来操作数据。而简单工厂模式则是一种常用的创建对象的设计模式,它将对象的创建与使用分离,使得系统更加灵活,易于扩展。本文将结合示例代码,介绍如何通过PHP面向对象简单工厂模式来实
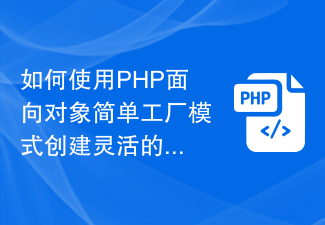
如何使用PHP面向对象简单工厂模式创建灵活的对象实例简单工厂模式是一种常见的设计模式,它可以在不暴露对象创建逻辑的情况下创建对象实例。这种模式可以提高代码的灵活性和可维护性,特别适用于需要根据输入条件动态创建不同对象的场景。在PHP中,我们可以利用面向对象编程的特性来实现简单工厂模式。下面我们来看一个例子,假设我们需要创建一个图形计算器,能够根据用户输入的形


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
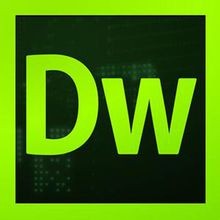
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
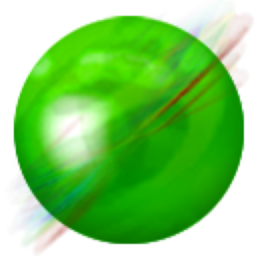
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
