


PHP file upload classes and examples (single file upload, multiple file upload)
本文分享一个不错的php文件上传,可以实现单个文件的上传、多个文件的上传功能。有需要的朋友参考下吧。
1,php文件上传类 <?php /** * php 文件上传类 * by bbs.it-home.org */ class file_upload { var $the_file; var $the_temp_file; var $upload_dir; var $replace; var $do_filename_check; var $max_length_filename = 100; var $extensions; var $ext_string; var $language; var $http_error; var $rename_file; // if this var is true the file copy get a new name var $file_copy; // the new name var $message = array(); var $create_directory = true; function file_upload() { $this->language = "en"; // choice of en, nl, es $this->rename_file = false; $this->ext_string = ""; } function show_error_string() { $msg_string = ""; foreach ($this->message as $value) { $msg_string .= $value."<br>\n"; } return $msg_string; } function set_file_name($new_name = "") { // this "conversion" is used for unique/new filenames if ($this->rename_file) { if ($this->the_file == "") return; $name = ($new_name == "") ? strtotime("now") : $new_name; $name = $name.$this->get_extension($this->the_file); } else { $name = $this->the_file; } return $name; } function upload($to_name = "") { $new_name = $this->set_file_name($to_name); if ($this->check_file_name($new_name)) { if ($this->validateExtension()) { if (is_uploaded_file($this->the_temp_file)) { $this->file_copy = $new_name; if ($this->move_upload($this->the_temp_file, $this->file_copy)) { $this->message[] = $this->error_text($this->http_error); if ($this->rename_file) $this->message[] = $this->error_text(16); return true; } } else { $this->message[] = $this->error_text($this->http_error); return false; } } else { $this->show_extensions(); $this->message[] = $this->error_text(11); return false; } } else { return false; } } function check_file_name($the_name) { if ($the_name != "") { if (strlen($the_name) > $this->max_length_filename) { $this->message[] = $this->error_text(13); return false; } else { if ($this->do_filename_check == "y") { if (preg_match("/^[a-z0-9_]*\.(.){1,5}$/i", $the_name)) { return true; } else { $this->message[] = $this->error_text(12); return false; } } else { return true; } } } else { $this->message[] = $this->error_text(10); return false; } } function get_extension($from_file) { $ext = strtolower(strrchr($from_file,".")); return $ext; } function validateExtension() { $extension = $this->get_extension($this->the_file); $ext_array = $this->extensions; if (in_array($extension, $ext_array)) { // check mime type hier too against allowed/restricted mime types (boolean check mimetype) return true; } else { return false; } } // this method is only used for detailed error reporting function show_extensions() { $this->ext_string = implode(" ", $this->extensions); } function move_upload($tmp_file, $new_file) { umask(0); if ($this->existing_file($new_file)) { $newfile = $this->upload_dir.$new_file; if ($this->check_dir($this->upload_dir)) { if (move_uploaded_file($tmp_file, $newfile)) { if ($this->replace == "y") { //system("chmod 0777 $newfile"); // maybe you need to use the system command in some cases... chmod($newfile , 0777); } else { // system("chmod 0755 $newfile"); chmod($newfile , 0755); } return true; } else { return false; } } else { $this->message[] = $this->error_text(14); return false; } } else { $this->message[] = $this->error_text(15); return false; } } function check_dir($directory) { if (!is_dir($directory)) { if ($this->create_directory) { umask(0); mkdir($directory, 0777); return true; } else { return false; } } else { return true; } } function existing_file($file_name) { if ($this->replace == "y") { return true; } else { if (file_exists($this->upload_dir.$file_name)) { return false; } else { return true; } } } function get_uploaded_file_info($name) { $str = "File name: ".basename($name)."\n"; $str .= "File size: ".filesize($name)." bytes\n"; if (function_exists("mime_content_type")) { $str .= "Mime type: ".mime_content_type($name)."\n"; } if ($img_dim = getimagesize($name)) { $str .= "Image dimensions: x = ".$img_dim[0]."px, y = ".$img_dim[1]."px\n"; } return $str; } // this method was first located inside the foto_upload extension function del_temp_file($file) { $delete = @unlink($file); clearstatcache(); if (@file_exists($file)) { $filesys = eregi_replace("/","\\",$file); $delete = @system("del $filesys"); clearstatcache(); if (@file_exists($file)) { $delete = @chmod ($file, 0775); $delete = @unlink($file); $delete = @system("del $filesys"); } } } // some error (HTTP)reporting, change the messages or remove options if you like. function error_text($err_num) { switch ($this->language) { case "nl": $error[0] = "Foto succesvol kopieert."; $error[1] = "Het bestand is te groot, controlleer de max. toegelaten bestandsgrootte."; $error[2] = "Het bestand is te groot, controlleer de max. toegelaten bestandsgrootte."; $error[3] = "Fout bij het uploaden, probeer het nog een keer."; $error[4] = "Fout bij het uploaden, probeer het nog een keer."; $error[10] = "Selecteer een bestand."; $error[11] = "Het zijn alleen bestanden van dit type toegestaan: <b>".$this->ext_string."</b>"; $error[12] = "Sorry, de bestandsnaam bevat tekens die niet zijn toegestaan. Gebruik alleen nummer, letters en het underscore teken. <br> Een geldige naam eindigt met een punt en de extensie."; $error[13] = "De bestandsnaam is te lang, het maximum is: ".$this->max_length_filename." teken."; $error[14] = "Sorry, het opgegeven directory bestaat niet!"; $error[15] = "Uploading <b>".$this->the_file."...Fout!</b> Sorry, er is al een bestand met deze naam aanwezig."; $error[16] = "Het gekopieerde bestand is hernoemd naar <b>".$this->file_copy."</b>."; break; case "de": $error[0] = "Die Datei: <b>".$this->the_file."</b> wurde hochgeladen!"; $error[1] = "Die hochzuladende Datei ist größer als der Wert in der Server-Konfiguration!"; $error[2] = "Die hochzuladende Datei ist größer als der Wert in der Klassen-Konfiguration!"; $error[3] = "Die hochzuladende Datei wurde nur teilweise übertragen"; $error[4] = "Es wurde keine Datei hochgeladen"; $error[10] = "Wählen Sie eine Datei aus!."; $error[11] = "Es sind nur Dateien mit folgenden Endungen erlaubt: <b>".$this->ext_string."</b>"; $error[12] = "Der Dateiname enthält ungültige Zeichen. Benutzen Sie nur alphanumerische Zeichen für den Dateinamen mit Unterstrich. <br>Ein gültiger Dateiname endet mit einem Punkt, gefolgt von der Endung."; $error[13] = "Der Dateiname überschreitet die maximale Anzahl von ".$this->max_length_filename." Zeichen."; $error[14] = "Das Upload-Verzeichnis existiert nicht!"; $error[15] = "Upload <b>".$this->the_file."...Fehler!</b> Eine Datei mit gleichem Dateinamen existiert bereits."; $error[16] = "Die hochgeladene Datei ist umbenannt in <b>".$this->file_copy."</b>."; break; // // place here the translations (if you need) from the directory "add_translations" // default: // start http errors $error[0] = "File: <b>".$this->the_file."</b> successfully uploaded!"; $error[1] = "The uploaded file exceeds the max. upload filesize directive in the server configuration."; $error[2] = "The uploaded file exceeds the MAX_FILE_SIZE directive that was specified in the html form."; $error[3] = "The uploaded file was only partially uploaded"; $error[4] = "No file was uploaded"; // end http errors $error[10] = "Please select a file for upload."; $error[11] = "Only files with the following extensions are allowed: <b>".$this->ext_string."</b>"; $error[12] = "Sorry, the filename contains invalid characters. Use only alphanumerical chars and separate parts of the name (if needed) with an underscore. <br>A valid filename ends with one dot followed by the extension."; $error[13] = "The filename exceeds the maximum length of ".$this->max_length_filename." characters."; $error[14] = "Sorry, the upload directory doesn't exist!"; $error[15] = "Uploading <b>".$this->the_file."...Error!</b> Sorry, a file with this name already exitst."; $error[16] = "The uploaded file is renamed to <b>".$this->file_copy."</b>."; } return $error[$err_num]; } } ?> 2,单文件上传的例子: <?php include ($_SERVER['DOCUMENT_ROOT']."/classes/upload/upload_class.php"); //classes is the map where the class file is stored (one above the root) $max_size = 1024*250; // the max. size for uploading $my_upload = new file_upload; $my_upload->upload_dir = $_SERVER['DOCUMENT_ROOT']."/files/new/"; // "files" is the folder for the uploaded files (you have to create this folder) $my_upload->extensions = array(".png", ".zip", ".pdf"); // specify the allowed extensions here // $my_upload->extensions = "de"; // use this to switch the messages into an other language (translate first!!!) $my_upload->max_length_filename = 50; // change this value to fit your field length in your database (standard 100) $my_upload->rename_file = true; if(isset($_POST['Submit'])) { $my_upload->the_temp_file = $_FILES['upload']['tmp_name']; $my_upload->the_file = $_FILES['upload']['name']; $my_upload->http_error = $_FILES['upload']['error']; $my_upload->replace = (isset($_POST['replace'])) ? $_POST['replace'] : "n"; // because only a checked checkboxes is true $my_upload->do_filename_check = (isset($_POST['check'])) ? $_POST['check'] : "n"; // use this boolean to check for a valid filename $new_name = (isset($_POST['name'])) ? $_POST['name'] : ""; if ($my_upload->upload($new_name)) { // new name is an additional filename information, use this to rename the uploaded file $full_path = $my_upload->upload_dir.$my_upload->file_copy; $info = $my_upload->get_uploaded_file_info($full_path); // ... or do something like insert the filename to the database } } ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> <title>php文件上传的例子-bbs.it-home.org</title> <style type="text/css"> <!-- label { float:left; display:block; width:120px; } input { float:left; } --> </style> </head> <body> <h3 id="php文件上传">php文件上传:</h3> <p>Max. filesize = <?php echo $max_size; ?> bytes.</p> <form name="form1" enctype="multipart/form-data" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <input type="hidden" name="MAX_FILE_SIZE" value="<?php echo $max_size; ?>"><br> <label for="upload">选择文件:</label><input type="file" name="upload" size="30"><br clear="all"> <label for="name">新的文件名称:</label><input type="text" name="name" size="20"> (without extension!) <br clear="all"> <label for="replace">是否替换</label><input type="checkbox" name="replace" value="y"><br clear="all"> <label for="check">验证文件名称:</label><input name="check" type="checkbox" value="y" checked><br clear="all"> <input style="margin-left:120px;" type="submit" name="Submit" value="Submit"> </form> <br clear="all"> <p><?php echo $my_upload->show_error_string(); ?></p> <?php if (isset($info)) echo "<blockquote>".nl2br($info)."</blockquote>"; ?> </body> </html> 3,多文件上传的例子 <?php include ($_SERVER['DOCUMENT_ROOT']."/classes/upload/upload_class.php"); //classes is the map where the class file is stored (one above the root) //error_reporting(E_ALL); $max_size = 1024*100; // the max. size for uploading class muli_files extends file_upload { //扩展file_upload类,以实现多文件上传功能 var $number_of_files = 0; var $names_array; var $tmp_names_array; var $error_array; var $wrong_extensions = 0; var $bad_filenames = 0; function extra_text($msg_num) { switch ($this->language) { case "de": // add you translations here break; default: $extra_msg[1] = "Error for: <b>".$this->the_file."</b>"; $extra_msg[2] = "You have tried to upload ".$this->wrong_extensions." files with a bad extension, the following extensions are allowed: <b>".$this->ext_string."</b>"; $extra_msg[3] = "Select at least on file."; $extra_msg[4] = "Select the file(s) for upload."; $extra_msg[5] = "You have tried to upload <b>".$this->bad_filenames." files</b> with invalid characters inside the filename."; } return $extra_msg[$msg_num]; } // this method checkes the number of files for upload // this example works with one or more files function count_files() { foreach ($this->names_array as $test) { if ($test != "") { $this->number_of_files++; } } if ($this->number_of_files > 0) { return true; } else { return false; } } function upload_multi_files () { $this->message = ""; if ($this->count_files()) { foreach ($this->names_array as $key => $value) { if ($value != "") { $this->the_file = $value; $new_name = $this->set_file_name(); if ($this->check_file_name($new_name)) { if ($this->validateExtension()) { $this->file_copy = $new_name; $this->the_temp_file = $this->tmp_names_array[$key]; if (is_uploaded_file($this->the_temp_file)) { if ($this->move_upload($this->the_temp_file, $this->file_copy)) { $this->message[] = $this->error_text($this->error_array[$key]); if ($this->rename_file) $this->message[] = $this->error_text(16); sleep(1); // wait a seconds to get an new timestamp (if rename is set) } } else { $this->message[] = $this->extra_text(1); $this->message[] = $this->error_text($this->error_array[$key]); } } else { $this->wrong_extensions++; } } else { $this->bad_filenames++; } } } if ($this->bad_filenames > 0) $this->message[] = $this->extra_text(5); if ($this->wrong_extensions > 0) { $this->show_extensions(); $this->message[] = $this->extra_text(2); } } else { $this->message[] = $this->extra_text(3); } } } $multi_upload = new muli_files; $multi_upload->upload_dir = $_SERVER['DOCUMENT_ROOT']."/files/"; // "files" is the folder for the uploaded files (you have to create this folder) $multi_upload->extensions = array(".png", ".zip"); // specify the allowed extensions here $multi_upload->message[] = $multi_upload->extra_text(4); // a different standard message for multiple files //$multi_upload->rename_file = true; // set to "true" if you want to rename all files with a timestamp value $multi_upload->do_filename_check = "y"; // check filename ... if(isset($_POST['Submit'])) { $multi_upload->tmp_names_array = $_FILES['upload']['tmp_name']; $multi_upload->names_array = $_FILES['upload']['name']; $multi_upload->error_array = $_FILES['upload']['error']; $multi_upload->replace = (isset($_POST['replace'])) ? $_POST['replace'] : "n"; // because only a checked checkboxes is true $multi_upload->upload_multi_files(); } ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"> <title>php多文件上传的例子-bbs.it-home.org</title> <style type="text/css"> <!-- label { width: 80px; } input { margin-bottom:3px; margin-left:5px; } --> </style> </head> <body> <h3 id="PHP-多文件上传">PHP 多文件上传:</h3> <p>Max. filesize = <?php echo $max_size; ?> bytes. (each) </p> <form name="form1" enctype="multipart/form-data" method="post" action="<?php echo $_SERVER['PHP_SELF']; ?>"> <input type="hidden" name="MAX_FILE_SIZE" value="<?php echo $max_size; ?>"> <label for="upload[]">File 1:</label> <input type="file" name="upload[]" size="30"><br> <label for="upload[]">File 2:</label> <input type="file" name="upload[]" size="30"><br> <label for="upload[]">File 3:</label> <input type="file" name="upload[]" size="30"><br> <!-- Add here more file fields if you need. --> Replace files? <input type="checkbox" name="replace" value="y"> <input type="submit" name="Submit" value="上传文件"> </form> <p><?php echo $multi_upload->show_error_string(); ?></p> </body> </html> |
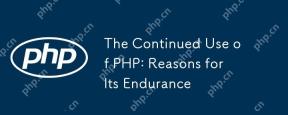
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
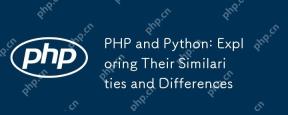
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
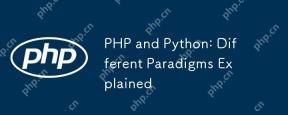
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
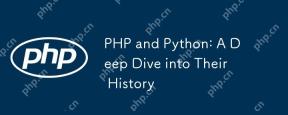
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
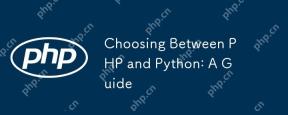
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
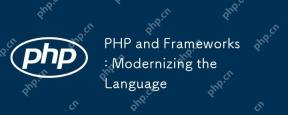
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
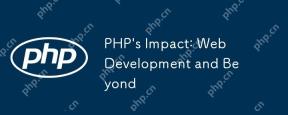
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
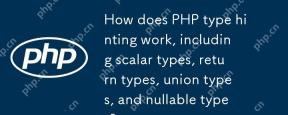
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
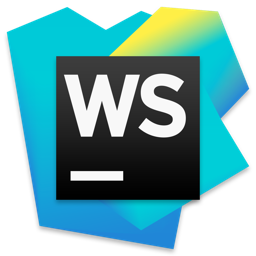
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
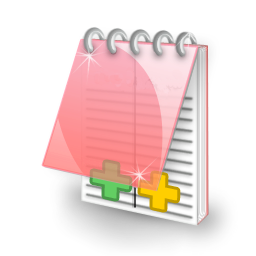
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software