A good PHP basic study note_PHP tutorial
1. Four representation forms of PHP fragments.
Standard tags:
short tags: ?> You need to set short _open_tag=on in php.ini, the default is on >Need to set asp_tags=on in php.ini, the default is off
script tags:
2. PHP variables and data types
1) $variable, variables start with letters, _, and cannot have spaces
2) Assignment $variable=value;
3) Weak type, direct assignment, no need to display the declared data type
4) Basic data Type: Integer, Double, String, Boolean, Object (object or class), Array (array)
5) Special data types: Resource (reference to third-party resources (such as database)), Null (empty, uninitialized) Variables)
3. Operators
1) Assignment operators: =
2) Arithmetic operators: +, -, *, /, % (modulo)
3) Connection operators :., no matter what the operand is, it will be treated as String, and the result will be returned String
4) Combined Assignment Operators total assignment operators: +=, *=, /=, -=, %=, .=
5 ) Automatically Incrementing and Decrementing automatic increase and decrease operators:
(1)$variable+=1 $variable++;$variable-=1 $variable-, just like c language, do it first For other operations, follow ++ or -
(2) ++$variable, -$variable, first ++ or -, and then do other operations
6) Comparison operator: = = (left side is equal to right side), != (the left side is not equal to the right side), = = (the left side is equal to the right side, and the data type is the same), >=, >, 7) Logical operators: || ó or, &&óand , xor (when one and only one of the left and right sides is true, return true),!
4. Comments:
Single-line comments: //, #
Multi-line comments: /* */
5 , Each statement ends with ;, the same as java
6. Define constants: define("CONSTANS_NAME",value)
7. Print statement: print, the same as c language
8. Process control statement
1) If statement:
(1) if(expression)
{
//code to excute if expression evaluates to true
}
(2) if(expression)
{
}
else
{
}
(3)if(expression1)
{
}
elseif(expression2)
{
}
else
{
}
2) swich statement
switch (expression)
{
case result
// execute this if expression results in result1
break;
case result
// execute this if expression results in result2
break;
default:
// execute this if no break statement
// has been encountered hitherto
}
3) ? Operator:
(expression)?returned_if_expression_is_true:returned_if_expression_is_false;
4) while statement:
(1) while (expression)
{
// do something
}
(2) do
{
// code to be executed
} while (expression);
5) for statement:
for (initialization expression; test expression; modification expression) {
// code to be executed
}
6) break; continue
9. Write function
1) Define function:
function function_name($argument1,$argument2,……) //Formal parameters
{
//function code here;
}
2) Function call
function_name($argument1,$argument2,……); //Formal parameters
3) Dynamic Function Calls:
function sayHello() { //Define function sayHello
print "hello
";
}
$function_holder = "sayHello"; //Assign the function name to the variable $function_holder
$function_holder(); //The variable $function_holder becomes a reference to the function sayHello. Calling $function_holder() is equivalent to calling sayHello
? >
4) Variable scope:
Global variables:
$life=42;
function meaningOfLife() {
global $life;
/*Redeclare $life as a global variable here. Accessing global variables within a function must be done this way. If the value of the variable is changed within the function, it will be changed in all code fragments*/
print "The meaning of life is $life
";
}
meaningOfLife();
?>
5) Use static
function numberedHeading( $txt ) {
static $num_of_calls = 0;
$num_of_calls++;
print "
$num_of_calls. $txt
";}
numberedHeading("Widgets"); //When called for the first time, print $num_of_calls value is 1
print("We build a fine range of widgets
");
numberedHeading("Doodads"); /*When called for the first time, print $num_of_calls value is 2, because the variable is static type, static type is resident in memory*/
print("Finest in the world
?>
6) Passing value (value) and passing reference (reference):
Passing value: function function_name($argument)
function addFive( $num ) {
$num += 5;
}
$orignum = 10;
addFive( &$orignum );
print ( $orignum );
?>
Result: 10
Address: funciton function_name(&$argument)
< ;html>
function addFive( &$num ) {
$num += 5; /*What is passed is a reference to the variable $num, so changing the value of the formal parameter $num is actually changing the value stored in the physical memory of the variable $orignum*/
}
$orignum = 10;
addFive( $orignum );
print( $orignum );
?>
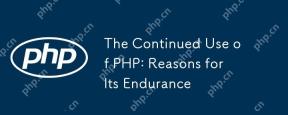
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
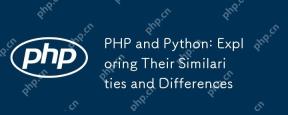
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
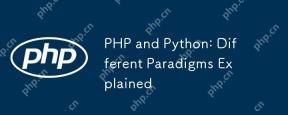
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
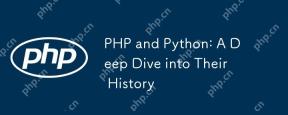
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
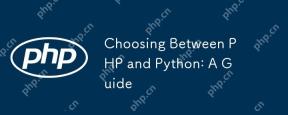
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
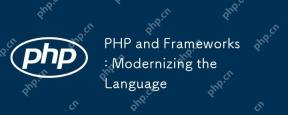
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
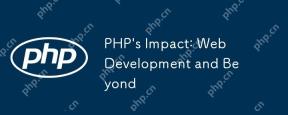
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
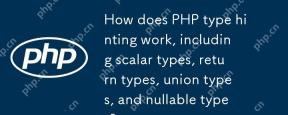
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
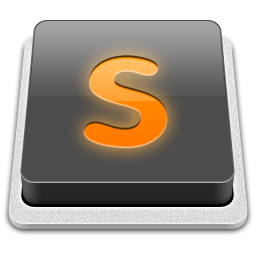
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment