


PHP creates files (folders) and directory operation codes_PHP tutorial
1. Directory operation
The first is the function to read from the directory, opendir(), readdir(), closedir(). When used, the file handle is opened first, and then iteratively listed:
$base_dir="filelist/";
$fso=opendir($base_dir) ;
echo $base_dir."
";
while($flist=readdir($fso)){
echo $flist."
";
}
closedir($fso)
?>
This is a program that returns the files and directories under the file directory (0 files will return false).
Yes When you need to know the directory information, you can use dirname($path) and basename($path) to return the directory part and file name part of the path respectively. You can use disk_free_space($path) to return the remaining space of the view space.
Create command :
mkdir($path,0777): 0777 is the permission code, which can be set by the umask() function under non-window conditions.
rmdir($path): will delete the file with the path in $path.
Two , File operations
● Create new files
First, determine the permissions of the directory where the file you want to create is located; the recommended device is 777. Then, it is recommended to use an absolute path for the name of the new file.
$filename="test.txt";
$fp=fopen("$filename", "w+"); //Open the file pointer and create the file
if ( !is_writable($filename) ){
die("File:" .$filename. "Not writable, please check!");
}
//fwrite($filename, "anything you want to write to $filename.";
fclose($fp); //Close pointer
● Reading a file
First, check whether a file can be read (permission issue), or whether it exists. We can use the is_readable function to obtain the information.:
$file = 'dirlist.php';
if (is_readable($file) = = false) {
die('File does not exist or cannot be read');
} else {
echo 'exists';
}
?>
The function to determine the existence of a file also includes file_exists (demonstrated below), but this is obviously not as comprehensive as is_readable. When a file exists, you can use
$file = "filelist.php";
if (file_exists($file) == false) {
die(' File does not exist');
}
$data = file_get_contents($file);
echo htmlentities($data);
?>
But file_get_contents function It is not supported on lower versions. You can create a handle to the file first, and then use a pointer to read the entire file:
There is also a way to read binary files:
$data = implode('', file($file));
● Write file
In the same way as reading a file, first check if it can be written:
$file = 'dirlist.php';
if (is_writable($file) == false) {
die("You have no right to write!");
}
?>
If you can write, you can use the file_put_contents function to write:
$file = 'dirlist.php';
if (is_writable($file) == false) {
die('I am a chicken, I can't ');
}
$data = 'I am despicable, I want';
file_put_contents ($file, $data);
?>
The file_put_contents function is a newly introduced function in php5 (if you don’t know it exists, use the function_exists function to determine it first). It cannot be used in lower versions of PHP. You can use the following method:
$f = fopen($file, 'w');
fwrite($f, $data);
fclose($f);
Replace it.
Sometimes you need to lock when writing a file, then write:
function cache_page($pageurl,$pagedata){
if(!$fso=fopen($pageurl,'w')){
$this->warns('Unable to open cache File.');//trigger_error
return false;
}
if(!flock($fso,LOCK_EX)){//LOCK_NB, exclusive lock
$this->warns ('Unable to lock cache file.');//trigger_error
return false;
}
if(!fwrite($fso,$pagedata)){//Write byte stream, serialize write Other formats
$this->warns('Unable to write to cache file.');//trigger_error
return false;
}
flock($fso,LOCK_UN);//Release the lock
fclose($fso);
return true;
}
● Copy and delete files
It is very easy to delete files in php, use the unlink function:
$file = 'dirlist.php';
$ result = @unlink ($file);
if ($result == false) {
echo 'The mosquitoes were driven away';
} else {
echo 'Cannot be driven away';
}
?>
That’s it.
Copying files is also easy:
$file = 'yang.txt';
$newfile = 'ji.txt'; # The parent folder of this file must be writable
if (file_exists($file) == false) {
die ('The demo is not online and cannot be copied');
}
$result = copy($file, $newfile);
if ($result == false) {
echo 'Copy memory ok';
}
?>
You can use the rename() function to rename a folder. Other operations can be achieved by combining these functions.
● Get file attributes
Let me talk about a few common functions:
Get the latest modification time:
$file = 'test.txt';
echo date('r', filemtime ($file));
?>
The returned timestamp is the unix timestamp, which is commonly used in caching technology.
Related is also getting the last time fileatime was accessed (), filectime() When the file's permissions, owner, all groups or other metadata in the inode are updated, the fileowner() function returns the file owner
$owner = posix_getpwuid(fileowner($file));
(non-window system), ileperms() obtains file permissions,
< ;?php
$file = 'dirlist.php';
$perms = substr(sprintf('%o', fileperms($file)), -4);
echo $perms;
?>
filesize() returns the number of bytes of the file size:
// Output is similar: somefile.txt: 1024 bytes
$filename = 'somefile.txt';
echo $filename . ': ' . filesize($ filename) . ' bytes';
?>
To get all the information of the file, there is a function stat() function that returns an array:
$file = 'dirlist.php';
$perms = stat($file);
var_dump($perms);
?>
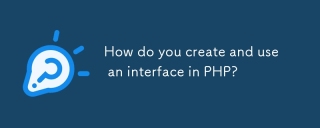
The article explains how to create, implement, and use interfaces in PHP, focusing on their benefits for code organization and maintainability.
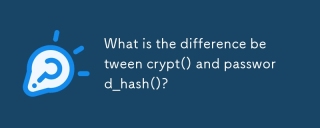
The article discusses the differences between crypt() and password_hash() in PHP for password hashing, focusing on their implementation, security, and suitability for modern web applications.
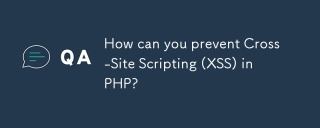
Article discusses preventing Cross-Site Scripting (XSS) in PHP through input validation, output encoding, and using tools like OWASP ESAPI and HTML Purifier.
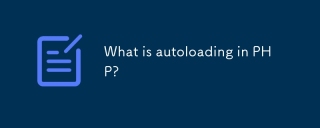
Autoloading in PHP automatically loads class files when needed, improving performance by reducing memory use and enhancing code organization. Best practices include using PSR-4 and organizing code effectively.
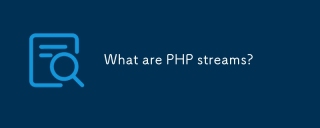
PHP streams unify handling of resources like files, network sockets, and compression formats via a consistent API, abstracting complexity and enhancing code flexibility and efficiency.
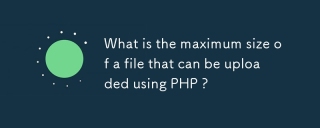
The article discusses managing file upload sizes in PHP, focusing on the default limit of 2MB and how to increase it by modifying php.ini settings.
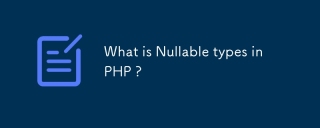
The article discusses nullable types in PHP, introduced in PHP 7.1, allowing variables or parameters to be either a specified type or null. It highlights benefits like improved readability, type safety, and explicit intent, and explains how to declar
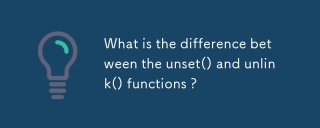
The article discusses the differences between unset() and unlink() functions in programming, focusing on their purposes and use cases. Unset() removes variables from memory, while unlink() deletes files from the filesystem. Both are crucial for effec


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
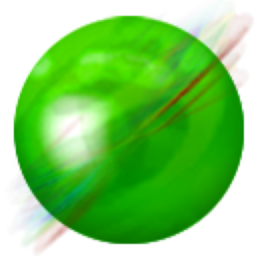
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
