It is an open source software, which is a series of PHP classes written entirely in PHP language that sends and receives SOAP messages through HTTP. It is developed by NuSphere Corporation (http://dietrich.ganx4.com/nusoap/). One advantage of NuSOAP is that it does not require extension library support. This feature allows NuSoap to be used in all PHP environments and is not affected by server security settings.
Method 1: Directly call
include('NuSoap.php');
// Create a soapclient object, the parameter is the server's WSDL
$client = new soapclient('http://localhost/Webservices/ Service.asmx?WSDL', 'wsdl');
// Convert parameters to array form and pass them
$aryPara = array('strUsername'=>'username', 'strPassword'=> MD5('password'));
//Call remote function
$aryResult = $client->call('login',$aryPara);
//echo $ client->debug_str;
$document=$client->document;
echo
$document
SoapDocument;
?>
Method 2: Proxy call
require('NuSoap.php');
//Create a soapclient object, the parameter is the server's WSDL
$client=new soapclient('http://localhost/Webservices/Service.asmx ?WSDL', 'wsdl');
//Generate proxy class
$proxy=$client->getProxy();
//Call remote function
$ aryResult=$proxy->login('username',MD5('password'));
//echo $client->debug_str;
$document=$ proxy->document;
echo
>
SoapDocument;
?>
Many friends who use NuSoap to call .NET WebService or J2EE WebService may have encountered the problem of Chinese garbled characters. The following introduces the reasons for this problem and the corresponding solutions.
The reason why NuSoap encounters garbled characters when calling WebService:
Usually we use UTF-8 encoding when developing WebService. At this time we need to set:
$client- >soap_defencoding = 'utf-8′;
At the same time, xml needs to be passed in the same encoding:
$client->xml_encoding = 'utf-8′;
Everything should be normal at this point, but when we output the results, we found that the returned code was garbled.
Solution to garbled code when NuSoap calls WebService:
In fact, friends who have turned on the debugging function, I believe that $client->response returns the correct result, why $result = $client->call($action, array('parameters' => $param)); But it's garbled code?
After studying the NuSoap code, we will find that when xml_encoding is set to UTF-8, NuSoap will detect the setting of decode_utf8. If it is true, the utf8_decode function in PHP will be executed, and NuSoap defaults to true, so , we need to set:
$client->soap_defencoding = 'utf-8′;
$client->decode_utf8 = false;
$client->xml_encoding = 'utf-8 ′;
Supplementary introduction
NuSOAP is a WEB service programming tool in the PHP environment, used to create or call WEB services. It is an open source software, the current version is 0.7.2, supports SOAP1.1, WSDL1.1, and can interoperate with other systems that support SOAP1.1 and WSDL1.1. NuSOAP is completely written in PHP language and consists of a series of PHP classes. It does not require the support of extension libraries. This feature allows NuSOAP to be used in all PHP environments and is not affected by server security settings.
1. Obtain and install NuSOAP
The NuSOAP project is built on SourceForge. The network address is: http://sourceforge.net/projects/nusoap/. Here, you can download the latest version of NuSOAP .
The installation of NuSOAP is relatively simple. Copy the downloaded NuSOAP file to the server. It can be placed in a separate directory or in the same directory as the program code, as long as your PHP code can access it. Just go to these files.
The test environment of this article is based on PHP4.3.2 and NuSOAP version 0.7.2. NuSOAP is installed in the WEB directory "/nusoap" and has two subdirectories, lib and samples. Among them, the lib directory stores all the source code files of NuSOAP, and the samples directory contains some examples provided by the NuSOAP development team. The test files are stored in the WEB directory "/nusoap".
2. Use of NuSOAP
NuSOAP consists of a PHP class, the most commonly used of which are class soap_server and class soapclient. The soap_server class is used to create WEB services, and the soapclient class is used when accessing WEB services.
2.1 A simple example: Hello World
This example will use NuSOAP to create a simple WEB service, and use NuSOAP to create a client program to call this service. The only function of this service is to return a string "Hello World" to the client. First, create the WEB service program code file "/nusoap/nusoap_server1.php":
//Include the NuSOAP source file into the current code file
require_once("lib/nusoap.php");
//Define service program
function hello() {
return 'Hello World!';
}
//Initialize the service object, which is an instance of the class soap_server
$soap = new soap_server; //Registration is required by calling the register method of the service object Programs accessed by clients.
//Only registered programs can be accessed by remote clients.
$soap->register('hello'); //The last step is to pass the data submitted by the client through post method to the service method of the service object.
//The service method processes the input data, calls the corresponding function or method, and generates correct feedback and sends it back to the client.
$soap->service($HTTP_RAW_POST_DATA);
?>
At this point, the WEB service program code file has been created. Next, create a client program code file "/nusoap/nusoap_client1.php", call WEB service:
//Include the NuSOAP source file into the current code file
require_once(“lib/nusoap.php”);
//Initialize the client object , this object is an instance of the soapclient class,
//Pass the URL address of the service program to the constructor of the soapclient class.
$client = new soapclient('http://127.0.0.1/nusoap/nusoap_server1.php'); //A program that uses the call method of the client object to call the WEB service
$str=$client-> ;call('hello'); //The getError() method of the client object can be used to check whether there is an error in the calling process.
//If there is no error, the getError() method returns false; if there is an error, the getError() method returns error information.
if (!$err=$client->getError()) {
echo ” The program returns:”,htmlentities($str,ENT_QUOTES);
} else {
echo ” Error: ",htmlentities($err,ENT_QUOTES);
}
?>
At this point, the client program has been created. Open the browser, access the client program, and take a look. result. In this example, the browser will display the string: "The program returns :Hello World! "
2.2 Methods of passing parameters and returning error information
Let's use an example to illustrate the method of passing parameters and returning error information. This example implements the connection of two strings. The parameters are two strings, and the return value is a string formed by concatenating the two parameters. First, create the service program code file "/nusoap/nusoap_server2.php". The complete code is as follows:
require_once(“lib/nusoap.php”);
function concatenate($str1,$str2) {
if (is_string($str1) && is_string($str2) )
return $str1 . $str2;
else
return new soap_fault('Client',",'The parameters of the concatenate function should be two strings');
}
$soap = new soap_server;
$soap->register('concatenate');
$soap->service($HTTP_RAW_POST_DATA);
?>
Compared with the code of the WEB service program in Section 2.1, the code structure here is generally the same. Note the following two points:
The definition of the service program is different, and the process of registering the service program with NuSOAP is still the same. It is to call the register method of the service object.
A new class of NuSOAP called soap_fault is used. When one of the two parameters passed in is not a string, the program returns the error message to the client through this class. The constructor has 4 parameters:
fault
code
Required parameter, the recommended value is "Client" or "Server", indicating whether the error is a client error or a server error. faultactor
Reserved item, not used yet
faultstring
Error description information
faultdetail
Optional, XML format data, describing detailed error information
The complete content of the client program code file "/nusoap/nusoap_client2.php" is as follows:
$client = new soapclient('http://127.0.0.1/nusoap/nusoap_server2.php');
$parameters=array(' string 1′,' string 2′);
$str=$client->call('concatenate',$parameters);
if (!$err= $client->getError()) {
echo ” Program returns:”,$str;
} else {
echo ” Error:”,$err;
}
?> ;
When the NuSOAP client calls a WEB service with parameters, it uses an array to pass parameters. $parameters is an array with the value of each parameter in turn. When the client calls a remote service program, it uses the call method with two parameters. The first parameter is the name of the service program, and the second parameter is the parameter array of the service program, here it is $parameters. Access the above client program through the browser, and the browser will display the string: "Program returns: String 1 String 2"
Next, try to pass in error parameters to the WEB service program and modify the above client client program, change the statement that generates the parameter array to: $parameters=array("String",12), and then access the client program through the browser, the browser will display the string: "Error: Client: concatenate function The parameters should be two strings". The WEB service program determines that one of the parameters passed in is not a string, and returns an error message to the client through soap_fault.
2.3 Debugging methods
There are three commonly used debugging methods in NuSOAP:
2.3.1 The request and response member variables of the soapclient class
The most direct debugging method is to check the process of accessing the WEB service. The request information sent by the client and the response information returned by the server. The request and response member variables of the soapclient class contain this information. Displaying the contents of these two variables in the program can help analyze the running status of the program. Look at the code below:
require_once(“lib/nusoap .php");
$client = new soapclient('http://127.0.0.1/nusoap/nusoap_server2.php');
$parameters=array(' string 1′,' string 2′ );
$str=$client->call('concatenate',$parameters);
if (!$err=$client->getError()) {
echo ” The program returns: ",$str;
} else {
echo " Error: ",$err;
}
//The contents of the request and response variables are shown below
echo '
echo 'Request:';
echo '
',htmlspecialchars($client->request,ENT_QUOTES),'';
echo 'Response: ';
echo '
',htmlspecialchars($client->response,ENT_QUOTES),'';
?>
2.3. 2 The debug_str member variable of the soapclient class
The debug_str member variable of the soapclient class provides more detailed debugging information. Viewing the content of this variable can better help program debugging.
2.3.3 Debugging methods provided by the WEB service program
In the WEB service program code, before creating an instance of the soap_server class, define the variable $debug=1 . The debugging information is used as a note and is placed at the end of the SOAP message and returned to the client. The client can view the debugging information by viewing the response information of the WEB service.
require_once("lib/nusoap.php");
function concatenate($str1,$str2) {
if (is_string($str1) && is_string($str2))
return $str1 . $str2;
else
return new soap_fault ('Client',",'The parameters of the concatenate function should be two strings');
}
$debug=1; //Define debugging
$soap = new soap_server;
$soap->register('concatenate');
$soap->service($HTTP_RAW_POST_DATA);
?>
2.4 Support for WSDL
NuSOAP Support for WSDL is implemented internally through the class "WSDL". For NuSOAP users, there is no need to care about how the internal WSDL class works. Support for WSDL can be achieved by correctly using the soap_server class and soapclient class. 2.4.1 Create a WEB service that supports WSDL
In order to implement the WEB service program's support for WSDL, you need to use the configureWSDL method of soap_server, and when calling the register method of soap_server to register the WEB service program, you need to provide more detailed parameters. For the code below, the file name of the code is "/nusoap/nusoap_server3.php".
require_once("lib/nusoap.php");
function concatenate($str1,$str2) {
if (is_string($str1) && is_string( $str2))
return $str1 . $str2;
else
return new soap_fault('Client',",'The parameters of the concatenate function should be two strings');
}
$soap = new soap_server;
$soap->configureWSDL('concatenate'); // Initialize support for WSDL
// Register service
$soap->register('concatenate ',
array(“str1″=>”xsd:string”,”str2″=>”xsd:string”), // Definition of input parameters
array(“return”=>” xsd:string”) // Definition of return parameters
);
$HTTP_RAW_POST_DATA = isset($HTTP_RAW_POST_DATA) ? $HTTP_RAW_POST_DATA : ”;
$soap->service($HTTP_RAW_POST_DATA);
?>
Now open the browser and access the file just created, http://127.0.0.1/nusoap/nusoap_server3.php. The results are as follows:
concatenate
View the WSDL for the service. Click on an operation name to view it's details.
concatenate
Click on the function name concatenate to see the description of the function. Click "WSDL", or access the WEB service file and append the query string "?wsdl" (http://127.0.0.1/nusoap/nusoap_server3.php?wsdl) to get the WSDL content of the WEB service.
2.4.2 Calling WEB services through WSDL
Calling WEB services through WSDL is roughly the same as calling WEB services without using WSDL. The difference is that when calling the WEB service through WSDL and initializing the soapclient class, two parameters are passed to the constructor of soapclient. The first parameter is the address of the WSDL file, and the second parameter specifies whether to use WSDL. Just specify it as true. Look at the code below, the file name of the code is "/nusoap/nusoap_client3.php"
require_once("lib/nusoap.php");
$client = new soapclient('http://127.0.0.1/nusoap/nusoap_server3.php?wsdl',true);
$parameters=array('String1′,'String2′);
$str=$client->call('concatenate',$parameters);
if (!$err=$ client->getError()) {
echo ” Program returns:”,$str;
} else {
echo ” Error:”,$err;
}
?>
2.4.3 Use of proxy
NuSOAP provides proxy methods to call remote WEB services. In this method, a remote service proxy object is created in the client program, and the remote WEB service is directly called through the proxy without going through the call method of the soapclient class. Look at the code below.
require_once("lib/nusoap.php");
$client = new soapclient('http://127.0.0.1/nusoap/nusoap_server3.php?wsdl',true);
$proxy=$client -> getProxy(); // Create proxy object (soap_proxy class)
$str=$proxy->concatenate(” Parameter 1″,” Parameter 2″); // Directly call the WEB service
if (!$err=$proxy->getError( )) {
echo ” Program returns:”,$str;
} else {
echo ” Error:”,$err;
}
?>
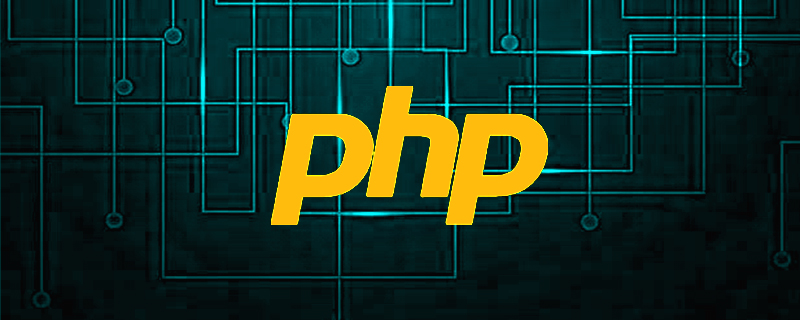
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
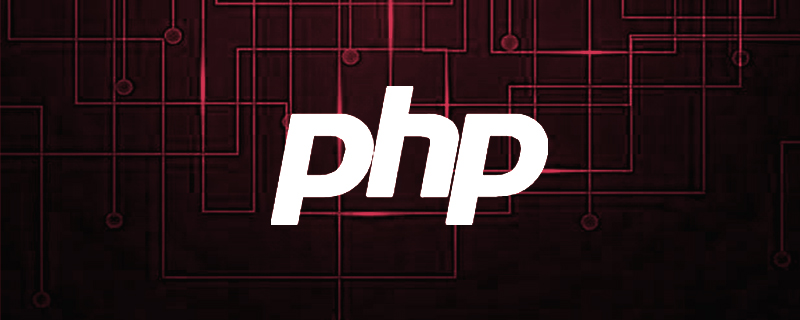
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
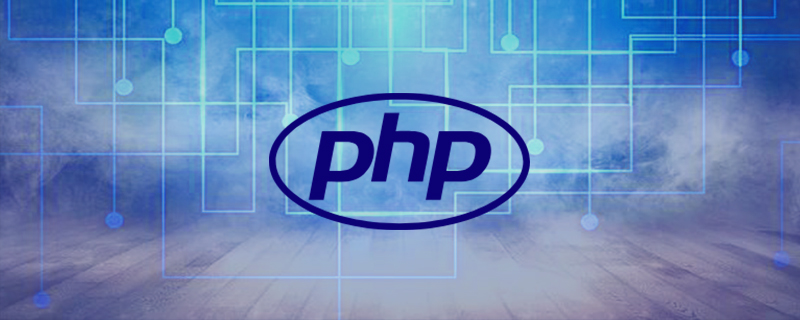
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
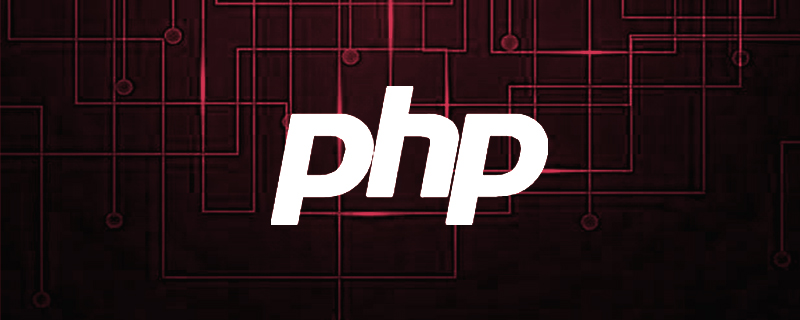
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
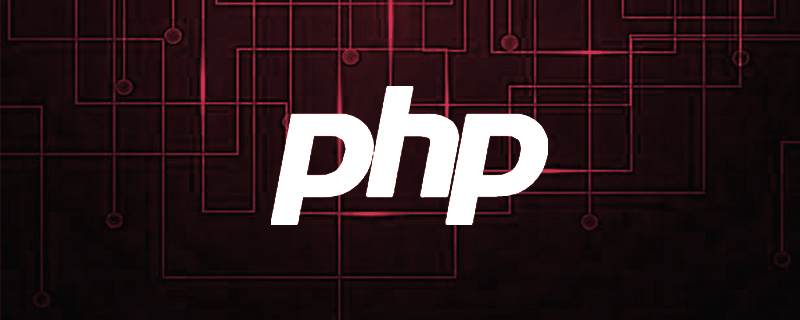
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
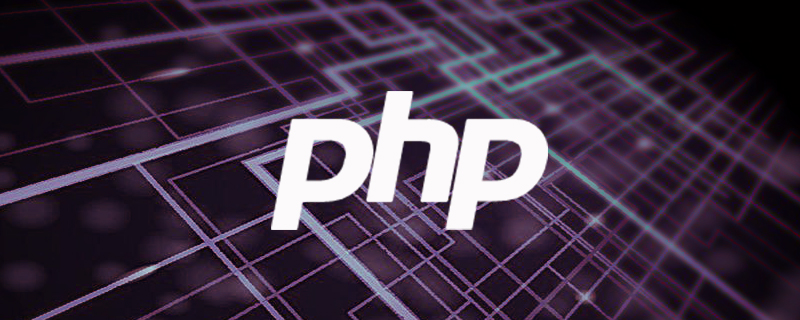
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
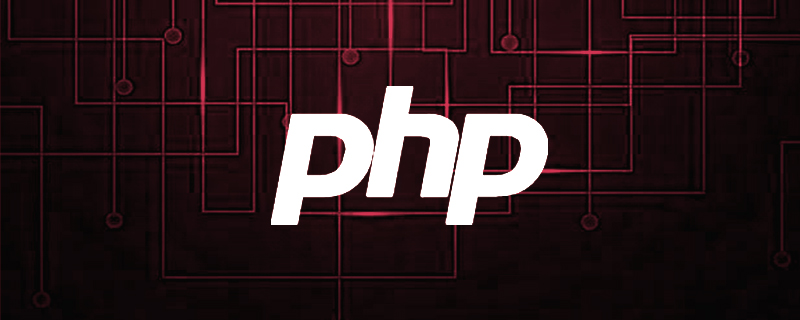
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
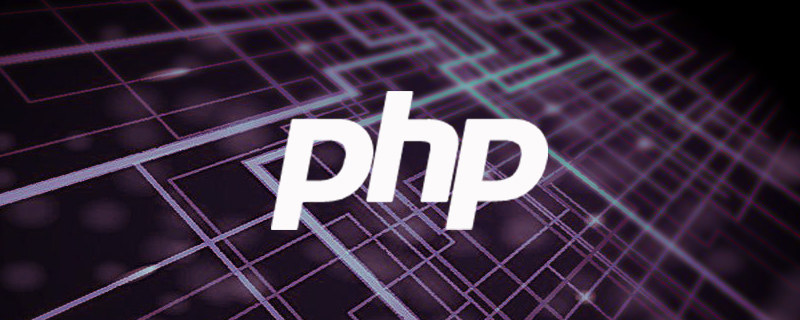
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
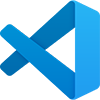
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
