1.json_decode()
json_decode
(PHP 5 >= 5.2.0, PECL json >= 1.2.0)
json_decode — for JSON Format string is encoded
Description
mixed json_decode (string $json [, bool $assoc])
Accepts a JSON format string and converts it into a PHP variable
Parameters
json
The string in json string format to be decoded.
assoc
When this parameter is TRUE, an array will be returned instead of an object.
Returns an object or if the optional assoc parameter is TRUE, an associative array is instead returned.
Example
Example #1 json_decode Example of ()
var_dump(json_decode($json));
var_dump(json_decode($ json, true));
?>
["a"] => int(1)
["b"] => int(2)
["c"] => int(3)
["d"] => int(4)
["e"] => int(5)
}
array(5) {
["a"] => int(1)
["b"] => int(2)
["c"] => int (3)
["d"] => int(4)
["e"] => int(5)
}
echo json_decode($data);
2.json_encode()
json_encode (PHP 5 >= 5.2.0, PECL json >= 1.2.0)
json_encode — JSON encoding of variables
Report a bug description
string json_encode ( mixed $value [, int $options = 0 ] )
Returns the JSON form of value
Report a bug Parameters
value
The value to be encoded, except the resource type, can be any data type
This function can only accept UTF-8 encoded data
options
A binary mask composed of the following constants Code: JSON_HEX_QUOT, JSON_HEX_TAG, JSON_HEX_AMP, JSON_HEX_APOS, JSON_NUMERIC_CHECK, JSON_PRETTY_PRINT, JSON_UNESCAPED_SLASHES, JSON_FORCE_OBJECT, JSON_UNESCAPED_UNICODE.
Report a bug Return value
If the encoding is successful, a String representation as JSON or returned on failure FALSE.
Report a bug Update log
Version Description
5.4.0 options parameters added constants: JSON_PRETTY_PRINT, JSON_UNESCAPED_SLASHES, and JSON_UNESCAPED_UNICODE.
5.3.3 The options parameter adds a constant: JSON_NUMERIC_CHECK.
5.3.0 Add options parameter.
Report a bug Example
Example #1 A json_encode() example
$arr = array ('a'=>1,'b'=>2,'c'=>3,'d'=>4, 'e'=>5);
echo json_encode($arr);
?>
The above routine will output:
{"a":1,"b":2,"c":3,"d":4, "e":5}
Example #2 Usage of options parameter in json_encode() function
$a = array('
echo "Normal: ", json_encode($a), "n";
echo "Tags: ", json_encode($a, JSON_HEX_TAG), "n";
echo "Apos: " , json_encode($a, JSON_HEX_APOS), "n";
echo "Quot: ", json_encode($a, JSON_HEX_QUOT), "n";
echo "Amp: ", json_encode($a, JSON_HEX_AMP) , “n”; , "nn";
$b = array();
echo "Empty array output as array: ", json_encode($b), "n";
echo "Empty array output as object: ", json_encode($b, JSON_FORCE_OBJECT), "nn";
$c = array(array(1,2,3));
echo "Non- associative array output as array: ", json_encode($c), "n";
echo "Non-associative array output as object: ", json_encode($c, JSON_FORCE_OBJECT), "nn";
$d = array('foo' => 'bar', 'baz' => 'long');
echo "Associative array always output as object: ", json_encode($d), "n";
echo "Associative array always output as object: ", json_encode($d, JSON_FORCE_OBJECT), "nn";
?>
The above routine will output :
Apos: ["
Quot: ["
Amp: ["
Unicode: ["
All: ["u003Cfoou003E","u0027baru0027","u0022bazu0022","u0026blongu0026","é"]
Empty array output as array: []
Empty array output as object: {}
Non-associative array output as array: [[1,2,3]]
Non- associative array output as object: {"0":{"0":1,"1":2,"2":3}}
Associative array always output as object: {"foo":" bar","baz":"long"}
Associative array always output as object: {"foo":"bar","baz":"long"}
Example #3 Examples of continuous and non-continuous arrays
$sequential = array("foo", "bar", "baz", "blong");
var_dump(
$sequential,
json_encode($sequential)
);
echo PHP_EOL."non-sequential array".PHP_EOL;
$nonsequential = array(1=>"foo", 2=>"bar", 3=>"baz" , 4=>"blong");
var_dump(
$nonsequential,
json_encode($nonsequential)
);
echo PHP_EOL."Delete a continuous array value Non-continuous array generated by ".PHP_EOL;
unset($sequential[1]);
var_dump(
$sequential,
json_encode($sequential)
);
? >
The above routine will output:
[0]=>
string(3) "foo"
[1]=>
string(3) "bar"
[2]=>
string(3) "baz"
[3]=>
string(5) "blong"
}
string(27) "["foo ","bar","baz","blong"]"
non-continuous array
array(4) {
[1]=>
string(3) "foo "
[2]=>
string(3) "bar"
[3]=>
string(3) "baz"
[4]=>
string(5) "blong"
}
string(43) "{"1":"foo","2":"bar","3":"baz","4":" blong"}"
A non-continuous array generated by deleting a continuous array value
array(3) {
[0]=>
string(3) "foo"
[2]=>
string(3) "baz"
[3]=>
string(5) "blong"
}
string(33) "{ "0":"foo","2":"baz","3":"blong"}"
$obj->Name= 'a1';$obj->Number ='123';
$obj->Contno= '000';
echo json_encode($obj ; :"a1",
"Number":"123",
}
www.bkjia.com
true
http: //www.bkjia.com/PHPjc/325399.html
TechArticle
1.json_decode() json_decode (PHP 5 = 5.2.0, PECL json = 1.2.0) json_decode — Right JSON format string encoding description mixed json_decode ( string $json [, bool $assoc ] ) accepts...
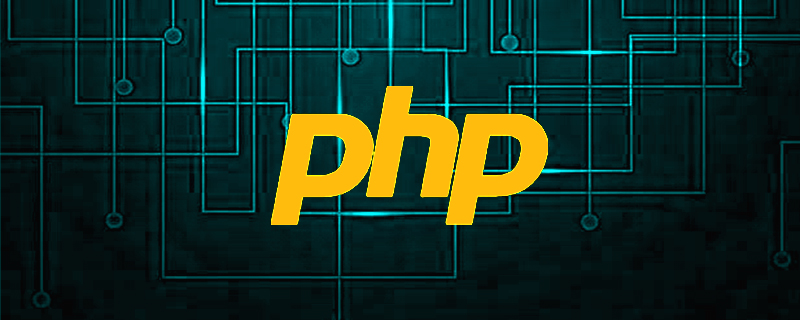
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
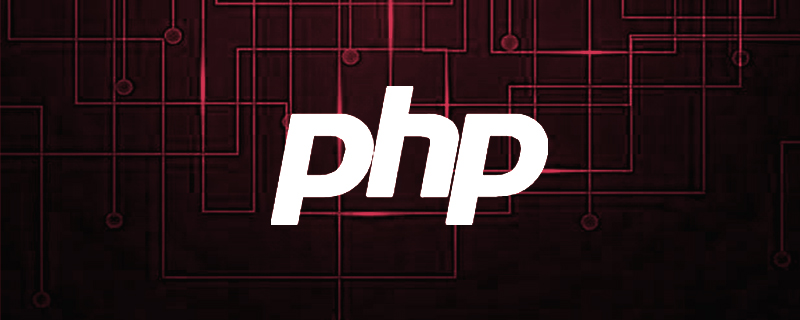
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
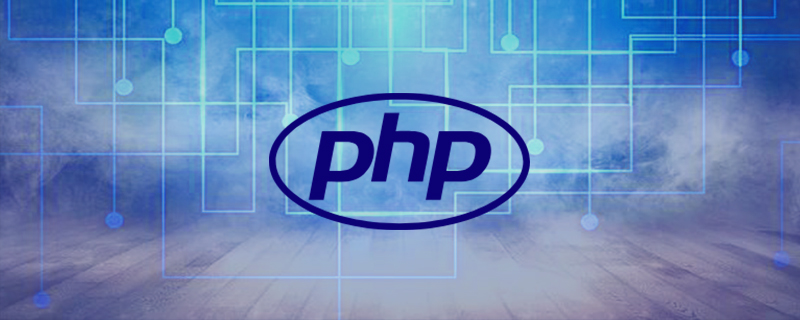
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
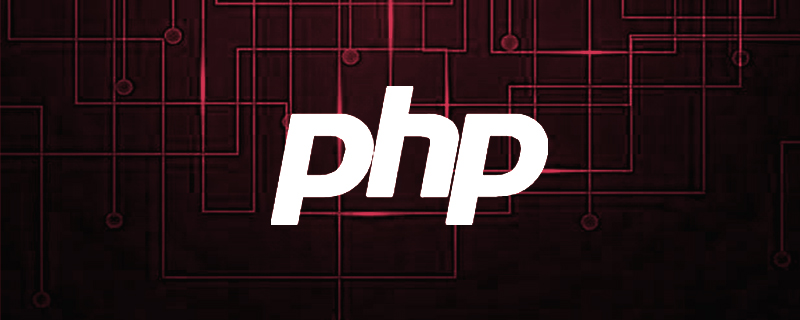
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
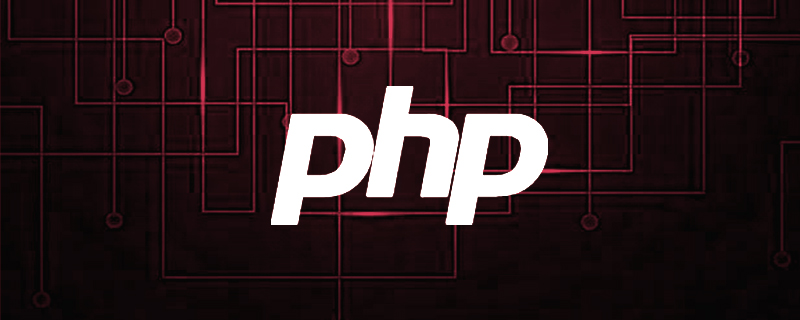
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
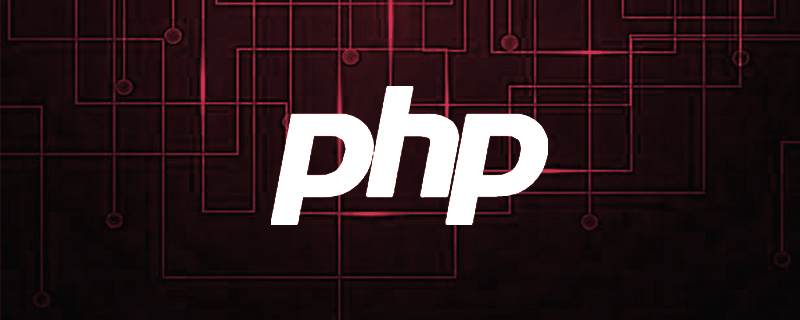
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
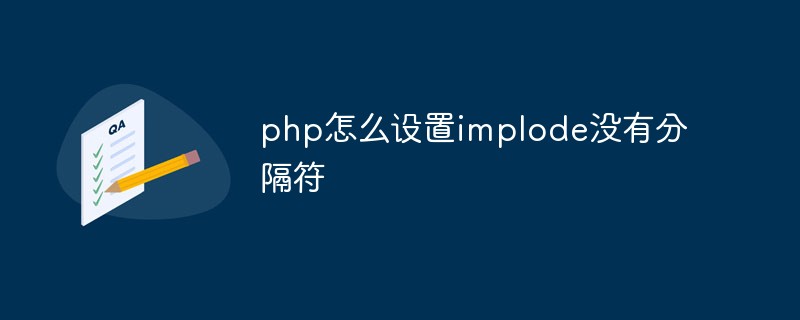
在PHP中,可以利用implode()函数的第一个参数来设置没有分隔符,该函数的第一个参数用于规定数组元素之间放置的内容,默认是空字符串,也可将第一个参数设置为空,语法为“implode(数组)”或者“implode("",数组)”。
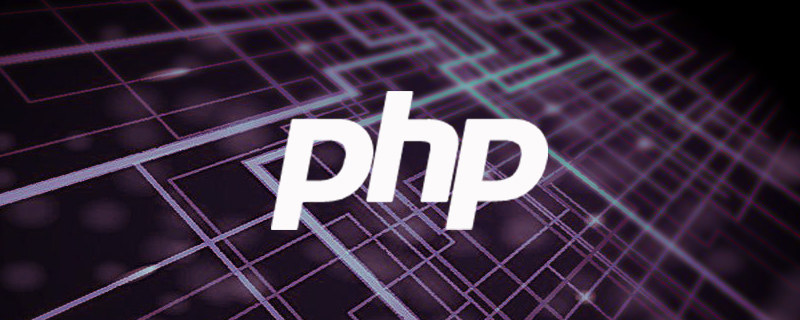
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
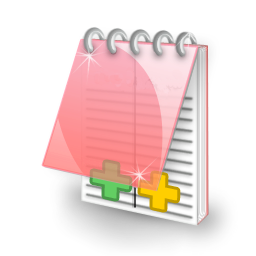
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
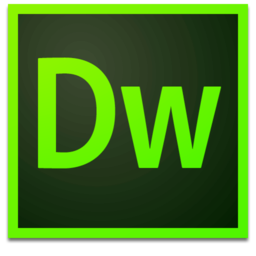
Dreamweaver Mac version
Visual web development tools
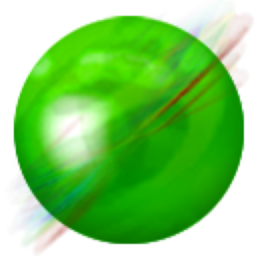
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
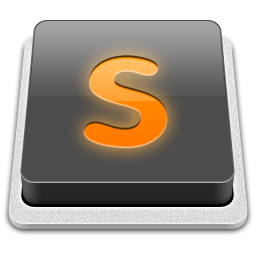
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
