First, let’s quote some concepts:
Factory pattern: specifically define a class to be responsible for creating instances of other classes. The created instances usually have the same parent class. The factory pattern is a class creation pattern that usually returns instances of different classes based on different independent variables.
The essence of the factory pattern is that a factory class dynamically determines which product instance should be created based on the incoming parameters. The factory pattern involves factory roles, abstract product roles and specific product roles.
Factory (Creator) role: It is the core of the factory pattern. It is responsible for implementing the internal logic of creating all instances. The factory class can be called directly by the outside world to create the required product objects.
Abstract product (Product) role: It is the parent class of all objects created by the factory pattern. It is responsible for describing the public interface common to all instances.
Concrete Product role: It is the creation target of the factory pattern. All objects are instances of a specific class that plays this role.
zend_db in ZF is a good example of factory pattern.
The analysis begins next. . . . . .
When configuring zf, we can put the database connection operation information in the Bootstrap.php file
Copy the code The code is as follows:
class Bootstrap extends Zend_Application_Bootstrap_Bootstrap
{
function __construct($app){
parent::__construct($app);
$url=constant( 'APPLICATION_PATH').DIRECTORY_SEPARATOR.'configs'.DIRECTORY_SEPARATOR.'config.ini';
$dbconfig=new Zend_Config_Ini($url,null,true);
$db=Zend_Db::factory($dbconfig- >general->db->adapter,$dbconfig->general->db->params->toArray());
// var_dump($db);
$db ->query('SET NAMES UTF8');
Zend_Db_Table::setDefaultAdapter($db);
}
}
?>
In the entry file At , when bootstrap() is called through a Zend_Application object, the constructor of the Bootstrap class will be called.
In the constructor, through Zend_Db::factory() we can get an object instance for operating the database.
Read the relevant information in config.ini through a Zend_Config_Ini instance and pass it as a parameter to the factory function Zend_Db::factory()
Configuration.ini information
[general]
db.adapter =PDO_MYSQL
db.params.host =localhost
db.params.username =root
db.params.password =
db.params.dbname = database name
Zend_Db::factory()
Parameter one: Indicates the type of database to be operated, such as PDO_MYSQL
Parameter two: Indicates the information to connect to the database, including server name, user name, password, and the database to be connected
Throw out first Two questions:
①If the database we want to operate is MSSQL, what should we do
②Here we use Zend_Db::factory(), if we use the traditional method, how should we do it
Answer:
① We only need to modify PDO_MYSQL to PDO_MSSQL in the config.ini file
② Create an object instance for operating the database in the traditional way:
$db=new Zend_Db_Adapter_Pdo_Mysql($ config)
Among them: $config information is read from config.ini
Here comes the problem: If we use the traditional method to create an object instance, we must have a process to determine the type of database we currently want to operate. ?
For example:
Copy code The code is as follows:
switch ($dbType){
case 'PDO_MYSQL' :
....
case 'PDO_MSSQL':
....
case 'PDO_SQLITE':
....
}
We still have to write different statements for operating the database according to different database types, which is very troublesome
However, zf has already done all of this for us through the factory mode, and it is very convenient to use
How to implement factory mode in Zf?
First of all, there must be an abstract base class: Zend_Db_Adapter_Abstract. This class is the parent class of all objects created by the factory pattern. It is responsible for providing the interface common to all instances.
This class not only provides some implementation methods that we are very familiar with operating databases, such as: select, update, insert, delete, query, fetchRow, fetchAssoc; in addition, it also provides some interfaces for implementation in subclasses , such as: limit, getServerVersion, closeConnection, describeTable, etc.
Copy code The code is as follows:
abstract class Zend_Db_Adapter_Abstract
{
//..
}
abstract class Zend_Db_Adapter_Pdo_Abstract extends Zend_Db_Adapter_Abstract
{
//..
}
class Zend_Db_Adapter_Pdo_Mysql extends Zend_Db_Adapter_Pdo_Abstract
{
//...Implement operations for Mysql database
}
class Zend_Db_Adapter_Pdo_Mssql extends Zend_Db_Adapter_Pdo_Abstract
{
//....Implement operations for Mssql database Operations
}
class Zend_Db_Adapter_Pdo_Sqlite extends Zend_Db_Adapter_Pdo_Abstract
{
//.... Implement operations for Sqlite database
}
The above relationship can be used This picture shows it simply
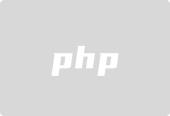
Next, let’s track how Zend_Db::Factory() selects different databases based on different parameters.
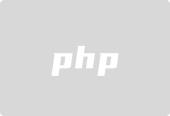
http://www.bkjia.com/PHPjc/325673.htmlwww.bkjia.comtruehttp: //www.bkjia.com/PHPjc/325673.htmlTechArticleFirst we quote some concepts: Factory pattern: specifically define a class to be responsible for creating instances of other classes, which are created Instances usually have the same parent class. Factory pattern belongs to class creation...