1. Singleton pattern (Singleton)
If the application contains and only contains one object at a time, then this object is a singleton. Used to replace global variables.
Copy code The code is as follows:
require_once("DB.php");
class DatabaseConnection{
public static function get(){
static $db = null;
if ( $db == null )
$db = new DatabaseConnection();
return $db;
}
private $_handle = null;
private function __construct() {
$dsn = 'mysql://root:password@localhost/photos';
$this- >_handle =& DB::Connect( $dsn, array() );
}
public function handle()
{
return $this->_handle;
}
}
print( "Handle = ".DatabaseConnection::get()->handle()."n" );
print( "Handle = ".DatabaseConnection::get()-> handle()."n" );
?>
2. Problems to be solved by the factory method pattern:
1> The object type to be generated is known only when the code is running; 2> The object type may need to be expanded with new product types; 3> Each product type can be customized with specific functions; the factory method pattern combines the creator class with the required The produced product class is separated. The creator is a factory class, which defines the class method used to generate product objects. If no default implementation is provided, the instantiation is performed by a subclass of the creator class. Generally, each A subclass of the creator class instantiates a corresponding product subclass. The advantage of the factory pattern lies in creating objects. Its task is to encapsulate the object creation process and then return a required new class. If you want to change the structure of the object and the way to create the object, you only need to select the object factory, and you only need to change the code once. (The function of the factory pattern is so powerful, it is at the bottom of the application, so it will continue to appear in many other complex patterns and applications.) Different processing objects are automatically offloaded internally, but for the user, only A simple and convenient example of using the interface method to practice the factory pattern:
Copy the code The code is as follows:
interface Hello{
function say_hello();
}
class English implements Hello{
public function say_hello(){
echo "Hello!";
}
}
class Chinese implements Hello{
public function say_hello(){
echo "Hello";
}
}
class speak{
public static function factory($type){
if($type == 1) $temp = new English();
else if($type == 2) $temp = new Chinese();
else{
die("Not supported! ");
}
return $temp;
}
}
$test = Speak::factory(1);
$test->say_hello();
In
, the above is called a simple factory pattern, because this factory must be able to identify all the products to be produced. If there are new products, the factory must be modified accordingly , add corresponding business logic or judgment. A sign of the simple factory pattern is that the static method implements the factory production function. (Not simple) Factory method pattern: The factory method is an abstract class or interface, and the specific factory implements this method (interface), so that The user calls to create a specific product object (each product has a corresponding specific factory). The following is the rewritten hello
Copy code The code is as follows:
//Abstract factory
interface Speaker{
function assignSpeaker();
}
//Concrete factory 1
class EnglishSpeaker implements Speaker{
public function assignSpeaker(){
return new English();
}
}
//Specific factory 2
class ChineseSpeaker implements Speaker{
public function assignSpeaker(){
return new Chinese();
}
}
//Abstract product
interface Hello{
function say_hello();
}
//Concrete product 1
class English implements Hello{
public function say_hello(){
echo "Hello!";
}
}
//Specific product 2
class Chinese implements Hello{
public function say_hello(){
echo "Hello";
}
}
Use:
Copy code The code is as follows:
if(!empty($_GET['t'])){
switch($_GET['t']){
case 1: $temp=new EnglishSpeaker();
break;
case 2: $temp=new ChineseSpeaker();
break;
}
$man=$temp->assignSpeaker() ;
$man->say_hello();
}
3. Abstract Factory model product family; each entity factory is responsible for the products of a product family (1, 2...), and each product family is divided into several different categories (A, B ...) Looking at a certain entity factory, it is actually a factory method pattern
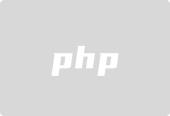
If the hello example above has more expressions, normal and singing expressions (2 product families)
Copy code The code is as follows:
//Abstract factory
abstract class Speaker{
const NORMAL =1 ;
const SING =2;
abstract function assignSpeaker($flag_int);
}
//Specific factory 1
class EnglishSpeaker extends Speaker {
public function assignSpeaker($flag_int) {
switch($flag_int){
case self::NORMAL:
return new NormalEnglish();
break;
case self::SING:
return new SingEnglish() ;
break;
}
}
}
//Specific factory 2
class ChineseSpeaker extends Speaker{
public function assignSpeaker($flag_int){
switch( $flag_int){
case self::NORMAL:
return new NormalChinese();
break;
case self::SING:
return new SingChinese();
break;
}
}
}
//Abstract product
interface Hello{
function say_hello();
}
//Concrete product A1
class NormalEnglish implements Hello{
public function say_hello(){
echo "Hello!";
}
}
//Specific product B1
class NormalChinese implements Hello{
public function say_hello(){
echo "Hello!";
}
}
//Specific product A2
class SingEnglish implements Hello{
public function say_hello(){
echo "Oh, jingle bells, jingle bells, Hello! Hello! Hello!";
}
}
//Specific product B2
class SingChinese implements Hello{
public function say_hello() {
echo "Ding Ding Dong, Ding Ding Dong, hello! Hello! Hello!";
}
}
Use:
Copy code The code is as follows:
//Determine the specific factory according to the business logic of the program
switch($_GET['language']){
case 1: $temp=new EnglishSpeaker();
break;
case 2: $temp=new ChineseSpeaker();
break;
}
//According to the business logic of the program Determine the specific product, no need to worry about which specific factory it is, and the maintainability is improved
$man=$temp->assignSpeaker( $_GET['style']);
//When using the product, you don’t need to worry about which specific factory it is. Product
$man->say_hello();
4. Prototype (Prototype)
Use clone to copy an existing specific product, and then specify The product categories themselves become the basis for their own generation.
http://www.bkjia.com/PHPjc/326063.htmlwww.bkjia.comtruehttp: //www.bkjia.com/PHPjc/326063.htmlTechArticle1. Singleton pattern (Singleton) If the application contains and only contains one object at a time, then this object is A singleton. Used to replace global variables. Copy the code as follows: ?php r...