


This article will introduce to you the detailed explanation of the implementation method of reading large files in PHP. Students who need to know more can refer to it for reference.
The requirements are as follows: There is a log file of about 1G with about 5 million lines. Use PHP to return the contents of the last few lines.
Implementation method:
1. Directly use the file function to operate
Note: Since the file function reads all the content into the memory at one time, PHP in order to prevent some poor writing The program takes up too much memory and causes insufficient system memory, causing the server to crash. Therefore, by default, the maximum memory usage is limited to 16M. This is set through memory_limit = 16M in php.ini. If this value is set -1, the memory usage is not limited.
The following is a piece of code that uses file to extract the last line of this file.
The entire code execution takes 116.9613 (s).
代码如下 | 复制代码 |
ini_set('memory_limit','-1'); $file = 'access.log'; $data = file($file); $line = $data[count($data)-1]; echo $line; |
$data = file($file);
$line = $data[count( $data)-1];echo $line;
My machine has 2 G of memory. When I press F5 to run, the system directly It turned gray and recovered after almost 20 minutes. It can be seen that the consequences of reading such a large file directly into the memory are serious, so it is not a last resort. The memory_limit cannot be adjusted too high, otherwise the only choice is to call the computer room. Let the machine reset.
代码如下 | 复制代码 |
file = 'access.log'; $file = escapeshellarg($file); // 对命令行参数进行安全转义 $line = `tail -n 1 $file`; echo $line; |
In the linux command line, you can directly use tail -n 10 access.log to easily display For the last few lines of the log file, you can directly use php to call the tail command. The execution of the php code is as follows.
The entire code execution takes 0.0034 (s)The code is as follows | Copy code |
file = 'access.log'; $file = escapeshellarg($file); // Safely escape command line parameters $line = `tail -n 1 $file`; echo $line; |
3. Directly use php’s fseek to perform file operations
代码如下 | 复制代码 |
function tail($fp,$n,$base=5) |
The code is as follows | Copy code |
function tail($fp,$n,$base=5){ assert($n>0); $pos = $n+1; $lines = array(); while(count($lines) try{ fseek($fp,-$pos,SEEK_END); } catch (Exception $e){ fseek(0); break; } $pos *= $base; while(!feof($fp)){ array_unshift($lines,fgets($fp)); } } return array_slice($ lines,0,$n);}var_dump(tail(fopen("access.log","r+"),10)); |
Method 2:
Still use fseek to read from the end of the file, but this time it is not reading bit by bit, but reading piece by piece. Each time a piece of data is read, it will be read The fetched data is placed in a buf, and then the number of newline characters (n) is used to determine whether the last $num rows of data have been read.
The implementation code is as follows
The entire code execution takes 0.0009 (s ).
The code is as follows
|
Copy code
|
||||
$fp = fopen($file, "r"); $line = 10;
| $t = " ";
while ($t != "n") { fseek($fp, $pos, SEEK_END);
$t = fgetc($fp);
The entire code execution takes time to complete 0.0003(s) The code is as followsCopy code |
$fp = fopen($file, "r");$num = 10;$chunk = 4096;$fs = sprintf("% u", filesize($file));$max = (intval($fs) == PHP_INT_MAX) ? PHP_INT_MAX : filesize($file);for ($len = 0; $len $seekSize = ($max - $len > $chunk) ? $chunk : $max - $len; fseek($fp, ($len + $ seekSize) * -1, SEEK_END); $readData = fread($fp, $seekSize) . $readData; if (substr_count($readData, "n") >= $num + 1) { preg_match("!(.*?n){".($num)."}$!", $readData, $match); $data = $match[0]; break; }}fclose($fp);echo $data;
http://www.bkjia.com/PHPjc/444602.htmlwww.bkjia.comtruehttp: //www.bkjia.com/PHPjc/444602.htmlTechArticleThis article will introduce you to the detailed implementation method of reading large files in php. Students who need to know more can Go to Reference. The requirements are as follows: There is a log file of about 1G, about...
|
|
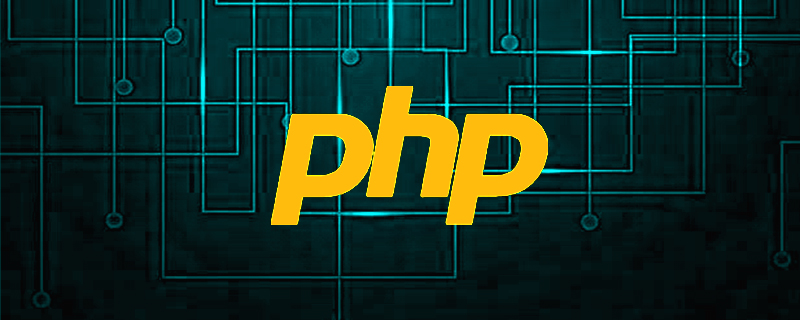
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
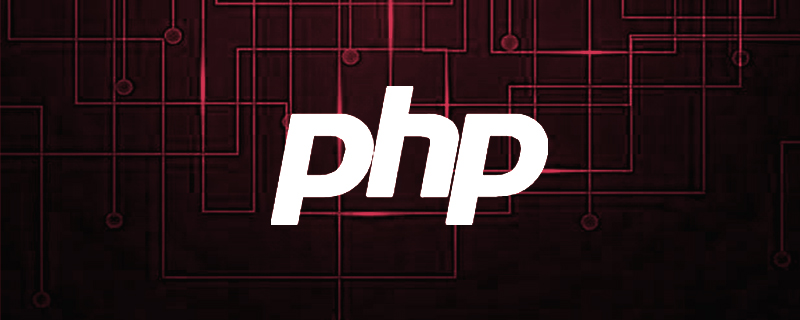
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
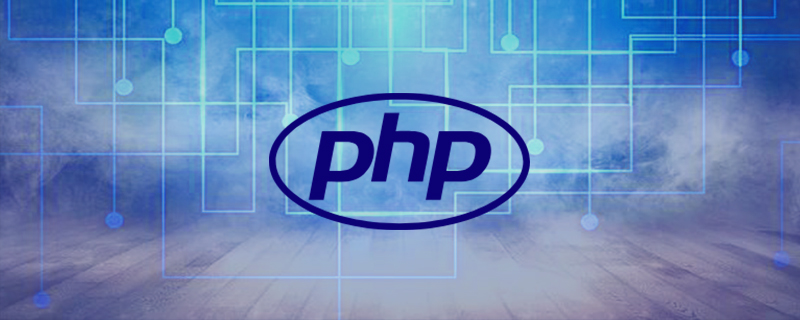
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
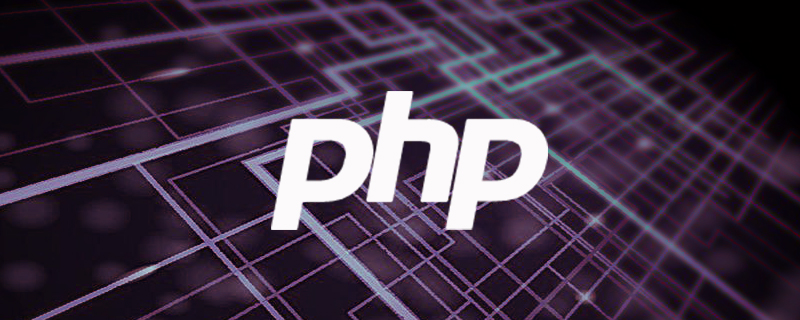
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
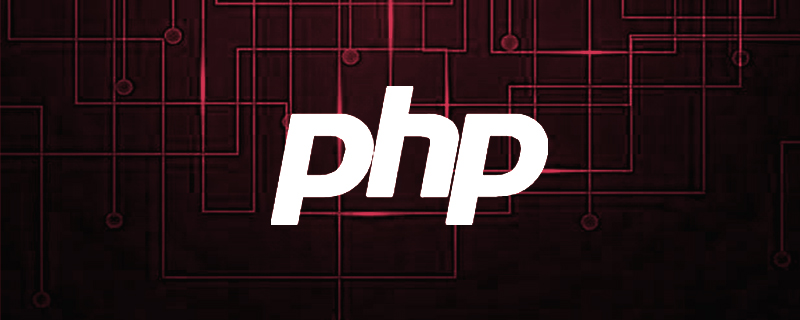
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
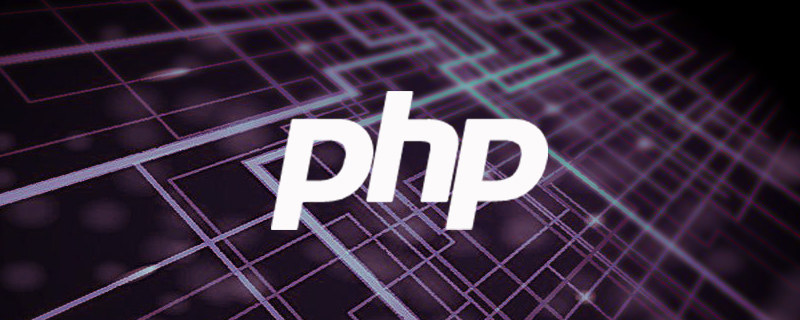
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
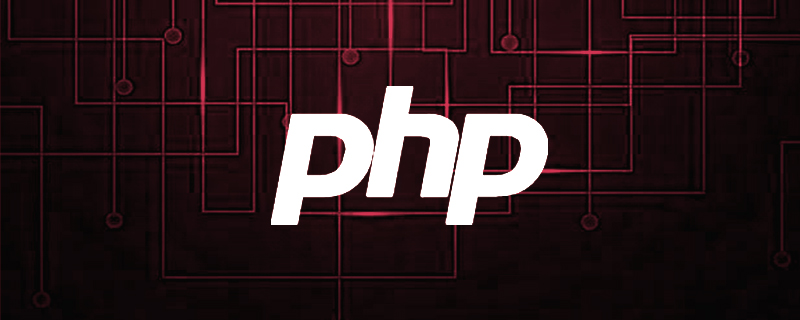
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
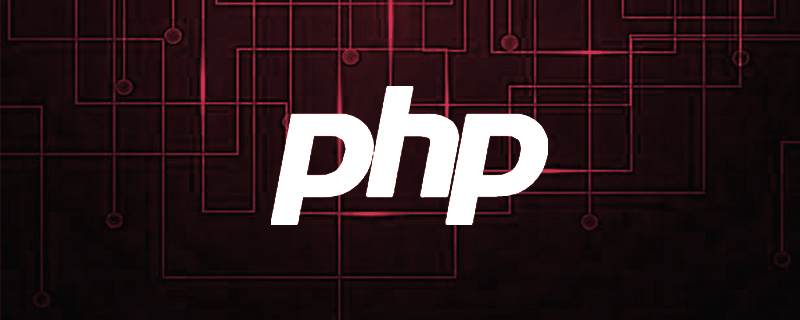
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
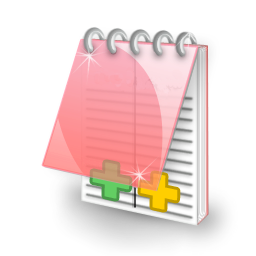
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
