


Commonly used array operations in PHP Array split times Key name difference set_PHP tutorial
This tutorial collects a large number of array examples that PHP beginners need to use, including creating an array and using the first two array values as the key and value data of the new array to return the data into strings to split the array without retaining the original array key names. The number of occurrences of array elements is assigned to the new array and data operations such as calculating differences on key names are displayed.
This tutorial collects a large number of array examples for beginners in PHP tutorials, including creating an array and using the first two array values as the keys and values of the new array. The data is returned as a string and the array is split without retaining the original array. Key name: Assign the number of occurrences of the original array element to the new array and display data operations such as calculating differences on the key name
*/
//
$array=array(1,1,1,1,1,8=>1,4=>1,19,3=>13); //Create array
print_r($array); //Output array content
//
$a=array('green','red','yellow'); //Define the first array
$b=array('avocado','apple','banana'); //Define the second array
$c=array_combine($a,$b); //Use the first two array values as the keys and values of the new array
print_r($c); //Output the newly created array
//
foreach(range(0,12)as $number) //Return array 0-12
{
echo $number.",";
}
echo "
";
foreach(range(0,100,10)as $number) //Return array 0, 10, 20...100
{
echo $number.",";
}
echo "
";
foreach(range('a','i')as $letter)
{
echo $letter.",";
}
echo "
";
foreach(range('c','a')as $letter) //Return array c, b, a
{
echo $letter.",";
}
//
$input_array=array('a','b','c','d','e'); //Define the initial array
print_r(array_chunk($input_array,2)); //Split the array without retaining the original array key name
print_r(array_chunk($input_array,2,true)); //Split the array and keep the original array key name
//
$array=array(1,"hello",1,"php","hello"); //Define an array
print_r(array_count_values($array)); //Assign the number of occurrences of the original array elements to the new array and display
//
$array1=array("a"=>"green","b"=>"brown","c"=>"blue","red"); //Define array 1
$array2=array("a"=>"green","yellow","red"); //Define array 2
$result=array_diff_assoc($array1,$array2); //Assign the difference between the two arrays to the array
print_r($result); //Output the content of the difference set
//
$array1=array('blue'=>1,'red'=>2,'green'=>3); //Define array 1
$array2=array('green'=>4,'blue'=>5,'yellow'=>6); //Define array 2
$result=var_dump(array_diff_key($array1,$array2)); //Calculate the difference set for the key name
print_r($result);
//
//Define callback function
function key_compare_func($a, $b)
{
if($a==$b)
{
Return 0; //If the two parameters are equal, return 0
}
Return($a>$b)?1:-1; //If $a>$b returns 1, if it is less than 1, it returns -1
}
//Define two arrays respectively
$array1=array("a"=>"green","b"=>"brown","c"=>"blue","red");
$array2=array("a"=>"green","yellow","red");
//Use the callback function to do index checking to calculate the difference set of the array
$result=array_diff_uassoc($array1,$array2,"key_compare_func");
print_r($result);
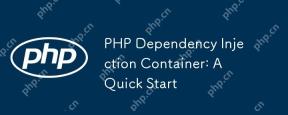
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
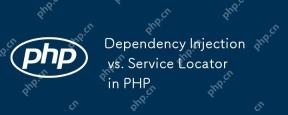
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
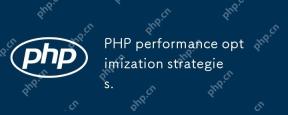
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
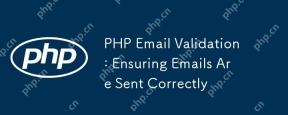
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
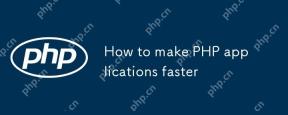
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
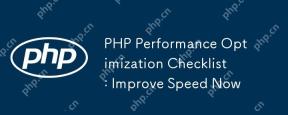
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
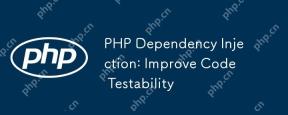
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
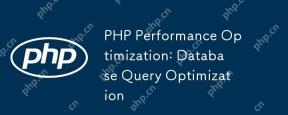
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
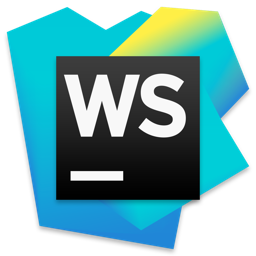
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
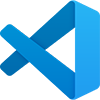
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
