PHP syntax analyzer: RE2C && BISON summary_PHP tutorial
Before this, I have tried a project that automatically generates so extensions for our PHP code,
Compiled into PHP, I call it phptoc.
However, due to various reasons, this project was suspended.
I wrote this article firstly because there is too little information in this area, and secondly to summarize what I have learned for future reference. If you can understand PHP syntax analysis
The research on PHP source code will go to a higher level ^.^…
I try to write it as plainly and understandably as possible.
This project idea originated from Facebook’s open source project HipHop.
Actually, I am skeptical about the 50%-60% performance improvement of this project. Fundamentally speaking, if PHP uses APC cache, will its performance be low?
As for HipHop, I haven’t tested it yet, so I can’t say for sure.
PHPtoc, I just want to liberate C programmers, hoping to achieve the goal of allowing PHPer to use PHP code to write an extension close to PHP extension performance,
The process is as follows: read the PHP file, parse the PHP code, perform a syntax analyzer on it, generate the corresponding ZendAPI, and compile it into an extension.
Get to the point
The most difficult thing here is the syntax analyzer. Everyone should know that PHP also has its own syntax analyzer. The current version uses re2c and Bison.
So, naturally I also used this combination.
It is not practical to use PHP's syntax analyzer, because you need to modify zend_language_parser.y and zend_language_scanner.l and recompile, which is not only difficult, but may also affect PHP itself.
So I decided to rewrite a set of my own syntax analysis rules. This function is equivalent to rewriting PHP's syntax analyzer, and of course some uncommon ones will be discarded.
re2c && yacc/bison, by referencing its corresponding files, then compile them into a *.c file, and finally compile it with gcc to generate
Into our own program. Therefore, they are not fundamentally a syntax analysis program. They just generate an independent C text based on our rules
file, this c file is the real syntax analysis program we need. I prefer to call it a syntax generator. As shown below:
Note: a.c in the picture is the final code generated by the scanner. .
re2c scanner, if the scanning rule file we write is called scanner.l, it will scan the content of the PHP file we wrote, and then scan it according to
The rules we wrote generate different tokens and pass them to parse.
The (f)lex grammar rule we wrote, for example, we call it Parse.y
It will be compiled into a parse.tab.h, parse.tab.c file through yacc/bison. Parse performs different operations according to different tokens
For example, our PHP code is “echo 1″;
Scan there is a rule:
"echo" {
return T_ECHO;
}
The scanner function scan will get the "echo 1" string, and it will loop through this piece of code. If it finds an echo string, it will return the token as a keyword: T_ECHO,
parse.y and scanner.l will generate two c files, scanner.c and parse.tab.c respectively, and compile them together with gcc.
I will talk about it in detail below
If you are interested, you can check it out. I have also translated a Chinese version,
It’s not over yet, I will post it later.
re2c provides some macro interfaces, which we use. I simply translated them. My English is not good and there may be errors. If you need the original text, you can go to the address above to view it.
Interface code:
Unlike other scanner programs, re2c does not generate a complete scanner: the user must provide some interface code. Users must define the following macros or other corresponding configurations.
YYCONDTYPE
With -c mode you can use the -to parameter to generate a file: use conditions containing enumeration types. Each value will be used as a condition in the rule set.
YYCTYPE
Used to maintain an input symbol. Usually char or unsigned char.
YYCTXMARKER
*For expressions of type YYCTYPE, the context of the generated code traceback information will be saved in YYCTXMARKER. The user needs to define this macro if the scanner rule requires the use of one or more regular expressions in the context.
YYCURSOR
The expression pointer of type *YYCTYPE points to the currently input symbol, and the generated code is matched as a symbol. At the beginning, YYCURSOR is assumed to point to the first character of the current token. At the end, YYCURSOR will point to the first character of the next token.
YYDEBUG(state,current)
This is only required when the -d flag is specified. It is very easy to debug the generated code when calling user-defined functions.
This function should have the following signature: void YYDEBUG(int state,char current). The first parameter accepts state , with a default value of -1 The second parameter accepts the current position of the input.
YYFILL(n)
When the buffer needs to be filled, the generated code will call YYFILL(n): providing at least n characters. YYFILL(n) will adjust YYCURSOR, YYLIMIT, YYMARKER and YYCTXMARKER as needed. Note that in typical programming languages, n is equal to the length of the longest keyword plus one. Users can define YYMAXFILL once in /*!max:re2c*/ to specify the maximum length. If -1 is used, YYMAXFILL will block once after /*!re2c*/.
YYGETCONDITION()
If -c mode is used, this definition will obtain the condition set before the scanner code. This value must be initialized to the type of the enumeration YYCONDTYPE.
YYGETSTATE()
If -f mode is specified, the user needs to define this macro. If so, in order to get the saved state at the beginning of the scanner, the generated code will call YYGETSTATE(). YYGETSTATE() must return a signed integer. If this value is -1, it tells the scanner that this is the first time. Execution, otherwise this value is equal to the state saved by previous YYSETSTATE(s). Otherwise, the scanner will call YYFILL(n) immediately after resuming operation.
YYLIMIT
The type of expression *YYCTYPE marks the end of the buffer (YYLIMIT(-1) is the last character of the buffer). The generated code will continuously compare YYCORSUR and YYLIMIT to determine when to fill the buffer.
YYSETCONDITION(c)
This macro is used to set conditions in conversion rules. It is only useful when -c mode is specified and conversion rules are used.
YYSETSTATE(s)
The user only needs to define this macro when specifying -f mode. If so, the generated code will call YYSETSTATE(s) before YYFILL(n). The parameter of YYSETSTATE is a signed integer called a unique identifier. A specific YYFILL(n) instance.
YYMARKER
An expression of type *YYCTYPE, the generated code saves the traceback information to YYMARKER. Some simple scanners may not be useful.
The scanner, as the name suggests, scans files to find key codes.
Scanner file structure:
/* #include file*/
/*Macro definition*/
//Scan function
int scan(char *p){
/*Scanner rules area*/
}
//Execute the scan function and return the token to yacc/bison.
int yylex(){
int token;
char *p=YYCURSOR;//YYCURSOR is a pointer pointing to our PHP text content
while(token=scan(p)){//The pointer p will be moved here to determine whether it is the scanner we defined above...
return token;
}
}
int main(int argc,char**argv){
BEGIN(INITIAL);//
YYCURSOR=argv[1];//YYCURSOR is a pointer pointing to our PHP text content,
yyparse();
}
BEGIN is a defined macro
#define YYCTYPE char //Type of input symbol
#define STATE(name) yyc##name
#define BEGIN(n) YYSETCONDITION(STATE(n))
#define LANG_SCNG(v) (sc_globals.v)
#define SCNG LANG_SCNG
#define YYGETCONDITION() SCNG(yy_state)
#define YYSETCONDITION(s) SCNG(yy_state)=s
The yyparse function is defined in yacc,
There is a key macro in it: YYLEX
#define YYLEX yylex()
It will execute the scanner's yylex
It may be a little twisted, so re-tie it:
In scanner.l, by calling parse.y parser function yyparse, this function calls yylex of scanner.l to generate the key code token, yylex
Return the scanner's
The token is returned to parse.y, and parse executes different codes based on different tokens.
Example:
scanner.l
#include "scanner.h"
#include "parse.tab.h"
int scan(char *p){
/*!re2c
return T_OPEN_TAG;
}
"echo" {
return T_ECHO;
}
[0-9]+ {
return T_LNUMBER;
}
*/
}
int yylex(){
int c;
// return T_STRING;
int token;
char *p=YYCURSOR;
while(token=scan(p)){
return token;
}
}
int main (int argc,char ** argv){
BEGIN(INITIAL);//Initialization
YYCURSOR=argv[1];//Put the string entered by the user into YYCURSOR
yyparse();//yyparse() -》yylex()-》yyparse()
return 0;
}
Such a simple scanner is made,
What about the parser?
The parsers I use are flex and bison. . .
About the file structure of flex:
%{
/*
The C code segment will be copied verbatim into the C source file generated after lex compilation
You can define some global variables, arrays, function routines, etc...
*/
#include
#include "scanner.h"
extern int yylex();//It is defined in scanner.l. .
void yyerror(char *);
# define YYPARSE_PARAM tsrm_ls
# define YYLEX_PARAM tsrm_ls
%}
{Definition section, where token is defined}
//This is the key. The token program does the switch based on this.
%token T_OPEN_TAG
%token T_ECHO
%token T_LNUMBER
%%
{Rule Section}
start:
T_OPEN_TAG{printf("startn"); }
|start statement
;
statement:
T_ECHO expr {printf("echo :%sn",$3)}
;
expr:
T_LNUMBER {$$=$1;}
%%
{User code snippet}
void yyerror(char *msg){
printf("error:%sn",msg);
}
In the rule section, start is the beginning. If scan recognizes the PHP start tag, it will return T_OPEN_TAG, then execute the code in the brackets and output start.
In scanner.l, the call to scan is a while loop, so it will check to the end of the php code,
yyparse will switch based on the tag returned by scan, and then goto to the corresponding code. For example, yyparse.y finds that the current token is T_OPEN_TAG,
It will be mapped to line 21 corresponding to parse.y through the macro #line, the position of T_OPEN_TAG, and then executed
So, what did TOKEN do after it was returned to yyparse?
In order to be more intuitive, I use gdb tracking:
At this time yychar is 258, what is 258?
258 is the enumeration type data automatically generated by bison.
Continue
YYTRANSLATE macro accepts yychar and returns the corresponding value
#define YYTRANSLATE(YYX) ((unsigned int) (YYX)
/* YYTRANSLATE[YYLEX] -- Bison symbol number corresponding to YYLEX. */
static const yytype_uint8 yytranslate[] =
{
0, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 27, 2,
22, 23, 2, 2, 28, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 21,
2, 26, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 24, 2, 25, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 2, 2, 2, 2,
2, 2, 2, 2, 2, 2, 1, 2, 3, 4,
5, 6, 7, 8, 9, 10, 11, 12, 13, 14,
15, 16, 17, 18, 19, 20
};
yyparse拿到这个值,不断地translate,
bison会生成很多用来映射的数组,将最终的translate保存到yyn,
这样bison就能找到token所对应的代码
switch (yyn)
{
case 2:
/* Line 1455 of yacc.c */
#line 30 "parse.y"
{printf("startn"); ;}
break;
这样,不断循环,生成token逐条执行,然后解析成所对应的zend 函数等,生成对应的op保存在哈希表中,这些不是本文的重点,
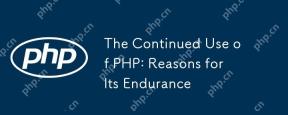
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
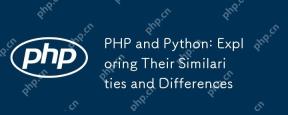
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
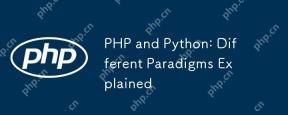
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
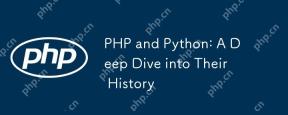
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
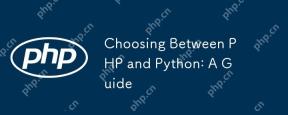
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
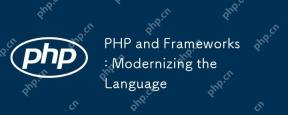
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
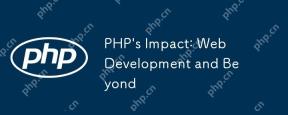
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
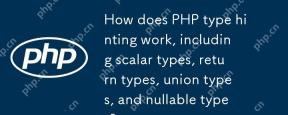
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
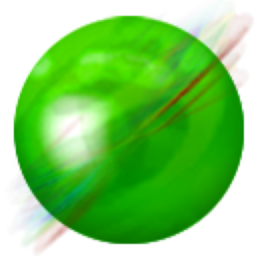
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
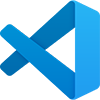
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment