mysql operation class_PHP tutorial
/**
* Database operation class
* 2011/8/25
* kcj
**/
class MyDB {
Private $db_host; //Database host name
Private $db_user; //Database user name
Private $db_pwd; //Database password
Private $db_database; //Database name
private $conn; //Connection ID
Private $result; //Result resource identifier of executing query command
private $row; //Number of entries returned
private $sql; //sql execution statement
Private $coding; //Database encoding
Private $bulletin=true; // Whether to enable error logging
Private $show_error=false; //During the testing phase, all errors are displayed, which has security risks and is closed by default
Private $is_error=false; //Whether to terminate immediately when an error is detected, the default is true, it is recommended not to enable it, because it is very distressing for users to not see anything when there is a problem
//Constructor
Function __construct($db_host,$db_user,$db_pwd,$db_database,$conn,$doding){
$this->db_host=$db_host;
$this->db_user=$db_user;
$this->db_pwd=$db_pwd;
$this->db_database=$db_database;
$this->conn=$conn;
$this->coding=$coding;
$this->connect();
}
//Database connection
Public function connect(){
If($this->conn=="pconn"){
// Permanent connection
$this->conn=mysql_pconnect($this->db_host,$this->db_user,$this->db_pwd);
}else{
use using
$this->conn=mysql_connect($this->db_host,$this->db_user,$this->db_pwd);
}
If(!mysql_select_db($this->db_database,$this->conn)){
If($this->show_error){
$ This-& gt; show_error ("Database is unavailable:", $ this-& gt; db_database);
}
}
//Database execution statement, any sql statement such as executable query, addition, modification, deletion etc.
Public function query($sql){
if($sql==""){
$this->show_error("SQL statement error:", "SQL statement is empty");
}
$this->sql=$sql;
$result=mysql_query($this->sql,$this->conn);
If(!$result){
If($this->show_error){
$this->show_error("Error sql statement: ",$this->sql);
$this->result;
}
return $result;
}
//Create and add a new database
Public function create_database($database_name){
$database=$database_name;
$sqlDatabase='create database'.$database;
$this->query($sqlDatabase);
}
//Query all databases on the server
//Separate the system database from the user for a more intuitive display
Public function show_database(){
$this->query("show databases");
echo "The current database:".$amount=$this->db_num_rows($rs);
echo "
";
$i=1;
While ($row=$this->fetch_array($rs)){
echo "$i $row[Database]";
echo "
";
$i++;
}
}
//Return all database names in the host in the form of an array
Public function databases(){
$rsPtr=mysql_list_dbs($this->conn);
$i=0;
$cnt=mysql_num_rows($rsPtr);
while ($i
$rs[]=mysql_db_name($rsPtr,$i);
$i++;
}
return $rs;
}
//Query all tables under the database
Public function show_tables($database_name){
$this->query("show tables");
echo "Existing database:".$amount=$this->db_num_rows($rs);
echo "
";
$i=1;
While ($row=$this->fetch_array($rs)){
$columnName="Tables_in_".$database_name;
echo "$i $row[$columnName]";
echo "
";
$i++;
}
}
// Get the result set
Public function fetch_array($resultt=""){
If($resultt!=""){
return mysql_fetch_array($resultt);
return mysql_fetch_array($this->result);
}
}
//Get the number of results $row['content']
Public function mysql_result_li(){
return mysql_result($str);
}
//Get the associative array $row['field name']
Public function fetch_assoc(){
return mysql_fetch_assoc($this->result);
}
//Get the numeric index array $row[0] $row[1] $row[2]
Public function fetch_row(){
return mysql_fetch_row($this->result);
}
//Get the object array, use $row->content
Public function fetch_Object(){
return mysql_fetch_object($this->result);
}
//Simplified query select
Public function findall($table){
$this->query("select* from $table");
}
//Simplified query select
Public function select($table,$columnName="*",$condition='',$debug=''){
$condition=$condition?'where'.$condition:null;
if($debug){
echo "select $columnName from $table $condition";
}else{
$this->query("select $columnName from $table $condition");
}
}
//Simplify deletion del
Public function delete($table,$condition,$url=''){
If($this->query("delete from $table where $condition")){
If(!emptyempty($url)){
$this->Get_admin_msg($url,'Delete successfully');
}
}
//Simplified insertion
Public function insert($table,$columnName,$value,$url=''){
If($this->query("insert into $table ($columnName) values ($value)")){
If(!emptyempty($url)){
$this->Get_admin_msg($url,'Added successfully');
}
//Simplified update update
Public function update($table,$mod_content,$condition,$url=''){
If($this->query("update $table set $mod_content where $condition")){
If(!emptyempty($url)){
$this->Get_admin_msg($url);
}
}
//Get the id of the previous insert operation
public function insert_id(){
return mysql_insert_id();
}
//指向确定的一条数据记录
public function db_data_seek($id){
if($id>0){
$id=$id-1;
}
if(!@mysql_data_seek($this->result,$id)){
$this->show_error("sql语句有误:","指定的数据为空");
}
return $this->result;
}
//根据select查询结果计算结果集条数
public function db_num_rows(){
if($this->result=null){
if($this->show_error){
$this->show_error("sql语句错误:","暂时为空,没有任何内容");
}
}else{
return mysql_num_rows($this->result);
}
}
//根据insert update delete执行的结果驱动影响行数
public function db_affected_rows(){
return mysql_affected_rows();
}
//输出显示sql语句
public function show_error($message="",$sql=""){
if(!$sql){
echo "" . $message . "";
echo "
";
}else{
echo "
echo "
";
}
//Release the result set
public function free(){
@mysql_free_result($this->result);
}
//Database selection
Public function select_db($db_database){
Return mysql_select_db($db_database);
}
//Query number of fields
Public function num_fields($table_name){
$this->query("select * from $table_name");
echo "
";
echo "Number of fields:".$total=mysql_num_fields($this->result);
for ($i=0;$i print_r(mysql_fetch_field($this->result,$i));
}
echo "";
echo "
";
}
//Get mysql server information
Public function mysql_server($num=''){
switch ($num){
case 1:
return mysql_get_server_info();
break;
case 2:
return mysql_get_host_info();
break;
case 3:
return mysql_get_client_info();
break;
case 4:
return mysql_get_proto_info();
break;
default:
return mysql_get_client_info();
}
}
public function __destruct(){
If(!emptyempty($this->result)){
$this->free();
}
Mysql_close($this->conn);
}
//Get the real ID address of the client
function getip() {
If (getenv("HTTP_CLIENT_IP") && strcasecmp(getenv("HTTP_CLIENT_IP"), "unknown")) {
$ip = getenv("HTTP_CLIENT_IP");
} else
If (getenv("HTTP_X_FORWARDED_FOR") &&strcasecmp(getenv("HTTP_X_FORWARDED_FOR"), "unknown")) {
$ip = getenv("HTTP_X_FORWARDED_FOR");
} else
If (getenv("REMOTE_ADDR") && strcasecmp(getenv("REMOTE_ADDR"), "unknown")) {
$ip = getenv("REMOTE_ADDR");
If (isset ($_SERVER['REMOTE_ADDR']) && $_SERVER['REMOTE_ADDR'] &&strcasecmp($_SERVER['REMOTE_ADDR'], "unknown")) {
$ip = $_SERVER['REMOTE_ADDR'];
$ip = "unknown";
return ($ip);
}
}
?>
/**
* Database operation class
* 2011/8/25
* kcj
**/
class MyDB {
private $db_host; //Database host name
private $db_user; //Database user name
private $db_pwd; //Database password
private $db_database; //Database name
private $conn; //Connection ID
private $result; //Result resource identifier of executing query command
private $row; //Number of entries returned
private $sql; //sql execution statement
private $coding; //Database encoding
private $bulletin=true; // Whether to enable error logging
private $show_error=false; //During the testing phase, all errors are displayed, which has security risks and is closed by default
private $is_error=false; //Whether to terminate immediately when an error is detected, the default is true, it is recommended not to enable it, because it is very distressing for users to not see anything when there is a problem
//Constructor
function __construct($db_host,$db_user,$db_pwd,$db_database,$conn,$doding){
$this->db_host=$db_host;
$this->db_user=$db_user;
$this->db_pwd=$db_pwd;
$this->db_database=$db_database;
$this->conn=$conn;
$this->coding=$coding;
$this->connect();
}
//Database connection
public function connect(){
if($this->conn=="pconn"){
//Permanent connection
$this->conn=mysql_pconnect($this->db_host,$this->db_user,$this->db_pwd);
}else{
//Even if connected
$this->conn=mysql_connect($this->db_host,$this->db_user,$this->db_pwd);
}
if(!mysql_select_db($this->db_database,$this->conn)){
if($this->show_error){
$this->show_error("Database unavailable:",$this->db_database);
}
}
}
//Database execution statement, any sql statement such as query addition, modification, deletion, etc. can be executed
public function query($sql){
if($sql==""){
$this->show_error("SQL statement error:","SQL statement is empty");
}
$this->sql=$sql;
$result=mysql_query($this->sql,$this->conn);
if(!$result){
If($this->show_error){
$this->show_error("Error sql statement:",$this->sql);
}
}else {
$this->result;
}
return $result;
}
//Create and add a new database
public function create_database($database_name){
$database=$database_name;
$sqlDatabase='create database'.$database;
$this->query($sqlDatabase);
}
//Query all databases on the server
//Separate the system database from the user for a more intuitive display
public function show_database(){
$this->query("show databases");
echo "The current database:".$amount=$this->db_num_rows($rs);
echo "
";
$i=1;
while ($row=$this->fetch_array($rs)){
echo "$i $row[Database]";
echo "
";
$i++;
}
}
//Return all database names in the host in the form of an array
public function databases(){
$rsPtr=mysql_list_dbs($this->conn);
$i=0;
$cnt=mysql_num_rows($rsPtr);
while ($i $rs[]=mysql_db_name($rsPtr,$i);
$i++;
}
Return $rs;
}
//Query all tables under the database
public function show_tables($database_name){
$this->query("show tables");
echo "Existing database:".$amount=$this->db_num_rows($rs);
echo "
";
$i=1;
while ($row=$this->fetch_array($rs)){
$columnName="Tables_in_".$database_name;
echo "$i $row[$columnName]";
echo "
";
$i++;
}
}
// Get the result set
public function fetch_array($resultt=""){
if($resultt!=""){
Return mysql_fetch_array($resultt);
}else {
Return mysql_fetch_array($this->result);
}
}
//Get the number of results $row['content']
public function mysql_result_li(){
Return mysql_result($str);
}
//Get associative array $row['field name']
public function fetch_assoc(){
Return mysql_fetch_assoc($this->result);
}
//Get the numeric index array $row[0] $row[1] $row[2]
public function fetch_row(){
Return mysql_fetch_row($this->result);
}
//Get the object array, use $row->content
public function fetch_Object(){
Return mysql_fetch_object($this->result);
}
//Simplified query select
public function findall($table){
$this->query("select* from $table");
}
//Simplified query select
public function select($table,$columnName="*",$condition='',$debug=''){
$condition=$condition?'where'.$condition:null;
if($debug){
echo "select $columnName from $table $condition";
}else{
$this->query("select $columnName from $table $condition");
}
}
//Simplify deletion del
public function delete($table,$condition,$url=''){
if($this->query("delete from $table where $condition")){
If(!empty($url)){
$this->Get_admin_msg($url,'Delete successfully');
}
}
}
//Simplify insert
public function insert($table,$columnName,$value,$url=''){
If($this->query("insert into $table ($columnName) values ($value)")){
If(!empty($url)){
$this->Get_admin_msg($url,'Added successfully');
}
}
}
//Simplified update update
public function update($table,$mod_content,$condition,$url=''){
if($this->query("update $table set $mod_content where $condition")){
if(!empty($url)){
$this->Get_admin_msg($url);
}
}
}
//取得上一步insert操作的id
public function insert_id(){
return mysql_insert_id();
}
//指向确定的一条数据记录
public function db_data_seek($id){
if($id>0){
$id=$id-1;
}
if(!@mysql_data_seek($this->result,$id)){
$this->show_error("sql语句有误:","指定的数据为空");
}
return $this->result;
}
//根据select查询结果计算结果集条数
public function db_num_rows(){
if($this->result=null){
if($this->show_error){
$this->show_error("sql语句错误:","暂时为空,没有任何内容");
}
}else{
return mysql_num_rows($this->result);
}
}
//根据insert update delete执行的结果驱动影响行数
public function db_affected_rows(){
return mysql_affected_rows();
}
//输出显示sql语句
public function show_error($message="",$sql=""){
if(!$sql){
echo "" . $message . "";
echo "
";
}else{
echo "";
echo "
";
}
//Release the result set
public function free(){
@mysql_free_result($this->result);
}
//Database selection
Public function select_db($db_database){
Return mysql_select_db($db_database);
}
//Query number of fields
Public function num_fields($table_name){
$this->query("select * from $table_name");
echo "
";
echo "Number of fields:".$total=mysql_num_fields($this->result);
for ($i=0;$i Print_r(mysql_fetch_field($this->result,$i));
}
echo "";
echo "
";
}
//Get mysql server information
Public function mysql_server($num=''){
switch ($num){
case 1:
return mysql_get_server_info();
break;
case 2:
return mysql_get_host_info();
break;
case 3:
return mysql_get_client_info();
break;
case 4:
return mysql_get_proto_info();
break;
default:
return mysql_get_client_info();
}
}
public function __destruct(){
if(!empty($this->result)){
$this->free();
}
mysql_close($this->conn);
}
//Get the real ID address of the client
function getip() {
If (getenv("HTTP_CLIENT_IP") && strcasecmp(getenv("HTTP_CLIENT_IP"), "unknown")) {
$ip = getenv("HTTP_CLIENT_IP");
} else
If (getenv("HTTP_X_FORWARDED_FOR") &&strcasecmp(getenv("HTTP_X_FORWARDED_FOR"), "unknown")) {
$ip = getenv("HTTP_X_FORWARDED_FOR");
} else
If (getenv("REMOTE_ADDR") && strcasecmp(getenv("REMOTE_ADDR"), "unknown")) {
$ip = getenv("REMOTE_ADDR");
} else
If (isset ($_SERVER['REMOTE_ADDR']) && $_SERVER['REMOTE_ADDR'] &&strcasecmp($_SERVER['REMOTE_ADDR'], "unknown")) {
$ip = $_SERVER['REMOTE_ADDR'];
} else {
$ip = "unknown";
}
return ($ip);
}
}
?>
Excerpted from chaojie2009’s column
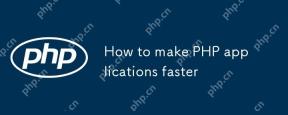
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
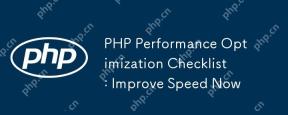
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
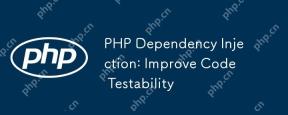
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
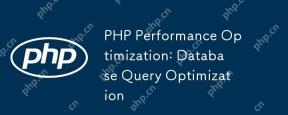
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
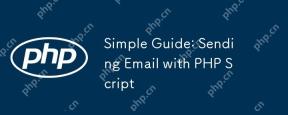
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
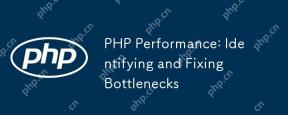
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
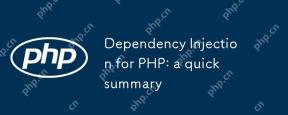
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
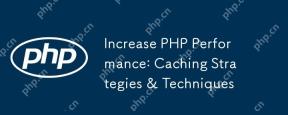
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
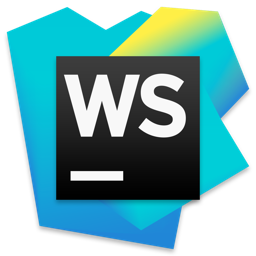
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
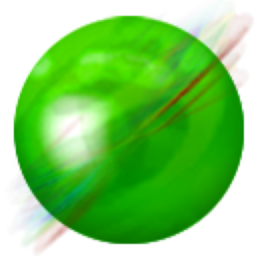
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
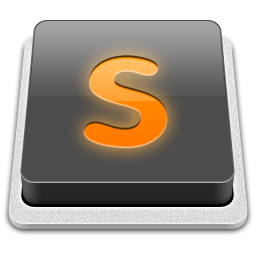
SublimeText3 Mac version
God-level code editing software (SublimeText3)
