define('BASE_PATH',$_SERVER['DOCUMENT_ROOT']);
define('SMARTY_PATH','smartTestSmarty\');
require BASE_PATH.SMARTY_PATH.'Smarty.class.php';
/*The path of $dir2 is displayed on the performance page and the following strings are the same, causing smarty to not be able to find the templates path*/
//$dir2 = "../Smarty/templates/";
class SmartyProject extends Smarty{
Function SmartyProject(){
/*You must add this parent::__construct();, otherwise, Smarty will not be constructed, truncate, etc. cannot be used,
* This doesn’t seem to make sense, but in fact,
* This does solve the problem that truncate cannot be used*/
parent::__construct();
$this->template_dir = BASE_PATH.SMARTY_PATH.'/templates/';
$this->compile_dir = BASE_PATH.SMARTY_PATH.'/templates_c/';
$this->config_dir = BASE_PATH.SMARTY_PATH.'/configs/';
$this->cache_dir = BASE_PATH.SMARTY_PATH.'/cache/';
}
}
class ConnDB{
var $dbtype;
var $host;
var $user;
var $pwd;
var $dbname;
var $debug;
var $conn;
Function ConnDB($dbtype, $host, $user, $pwd, $dbname, $debug=FALSE){//Constructor
$this->dbtype=$dbtype;
$this->host=$host;
$this->user=$user;
$this->pwd=$pwd;
$this->dbname=$dbname;
$this->debug=$debug;
}
Function GetConnId(){
require BASE_PATH.'/smartTest/adodb5/adodb.inc.php';//Reference the main file of adodb
If($this->dbtype == 'mysql'){
$this->conn = NewADOConnection('mysql');
$this->conn->Connect($this->host, $this->user, $this->pwd, $this->dbname);
}else if($this->dbtype == 'mssql'){
$this->conn = NewADOConnection('mssql');
$this->conn->Connect($this->host, $this->user, $this->pwd, $this->dbname);
}else if($this->dbtype == 'access'){
$this->conn = NewADOConnection('access');
$this->conn->Connect("Driver={Microsoft Access Driver(*.mdb)};Dbq=".$this->dbname.";Uid=".$this->user." ;Pwd=".$this->pwd.";");
}
$this->conn->Execute("set names utf-8");
If($this->dbtype == 'mysql')
$this->conn->debug = $this->debug;
Return $this->conn;
}
Function CloseConnId(){
$this->conn->Disconnect();
}
}
class AdminDB{
Function ExecSQL($sqlstr, $conn){
$sqltype = strtolower(substr(trim($sqlstr), 0,6));//Remove the first 6 characters of the sql statement
$rs = $conn->Execute($sqlstr);
If($sqltype == 'select'){
$array = $rs->GetRows();
If(count($array)==0 || $rs == false)
return false;
else
return $array;
}else if($sqltype == 'update'||$sqltype == 'insert'||$sqltype == 'delete'){
//This does not return a result set, or returns an empty set
If($rs)
return true;
else
return false;
}
}
}
class SepPage{
var $rs;
var $pagesize;
var $nowpage;
var $array;
var $conn;
var $sqlstr;
Function ShowData($sqlstr,$conn,$pagesize,$nowpage){
If(!isset($nowpage)||$nowpage==""){
$this->nowpage = 1;
}else{
$this->nowpage = $nowpage;
}
$this->pagesize = $pagesize;
$this->conn = $conn;
$this->sqlstr = $sqlstr;
//Execute query statement
$this->rs = $this->conn->PageExecute($this->sqlstr,$this->pagesize,$this->nowpage);//Call this method in the ADODO class
@$this->array = $this->rs->GetRows();
If(count($this->array)==0||$this->rs == false)
return false;
else
Return $this->array;
}
Function ShowPage($contentname, $utits, $anotherserchstr, $anotherserchstrs, $class){
$allrs = $this->conn->Execute($this->sqlstr); //Execute query statement
$record = count($allrs->GetRows());//Total number of statistical records
$pagecount = ceil($record/$this->pagesize);//Calculate how many pages there are
@$str.="Total ".$contentname." ".$record." ".$utits." Each page displays ".$this->pagesize.
".$utits." ".$this->rs->AbsolutePage()." Pages/Total ".$pagecount." Pages";
$str.="
If(!$this->rs->AtFirstPage())
$str.=" $anotherserchstrs."class=".$class.">首页";
else
$str.="首页";
$str.=" ";
if (!$this->rs->AtFirstPage())
$str.="rs->AbsolutePage()-1)."¶meter1=".$anotherserchstr."¶meter2=".
$anotherserchstrs."class=".$class.">上一页";
else
$str.="上一页";
$str.=" ";
if(!$this->rs->AtLastPage())
$str.="rs->AbsolutePage()+1)."¶meter1=".$anotherserchstr."¶meter2=".
$anotherserchstrs."class=".$class.">下一页";
else
$str.="下一页";
$str.=" ";
if(!$this->rs->AtLastPage())
$str.="
$anotherserchstrs."class=".$class.">尾页";
else
$str.="尾页";
if(count($this->array)== 0||$this->rs==false)
return "";
else
return $str;
}
}
以上代码定义了几个类,用来实现数据库的连接啊,操作数据库,分页实现,数据库的存储,以及一个smarty类的子类,其实这个子类也没有实现什么特别的功能。将其命名为
smartyDAO.php或这随便取个什么名字都可以,当然,还有一点要注意的是,我里面试用了require语句,对于这些路径,大家都要该到自己对应的目录去。
require 'smartyDao.php';
//数据库类实例
$conobj = new ConnDB("mysql", "localhost", "root", "brave", "bank");
$conn = $conobj->GetConnId();
//数据库操作类对象
$admindb = new AdminDB();
//Smarty模板配置类对象
$smarty = new SmartyProject();
$seppage = new SepPage();//实例化分页类
This file is an instantiation of the class in the above file. It can be named smartyDaoImpl.php, or named as you like. The function is very simple, right?
The next thing to do is to create a test case to test the paging code. Of course, this test also contains two files, one is the controller and the other is the view. The code of the controller is:
include_once 'conn/smartyDaoImpl.php';
$arr_page = $seppage->ShowData("select * from localinfo", $conn, 5, $_GET['page']);//Execute paging query
if(!$arr_page)
$smarty->assign('page',"F");
else {
$smarty->assign('page','T');
$smarty->assign('showpage',$seppage->ShowPage("record", "a", "", "", "a1"));//Assignment paging template
$smarty->assign('arr_page',$arr_page);//Copy the query record to the template
}
$smarty->display('view/fenyeShow.html');//Specify the template for displaying data
Give it a name, let’s call it smarty_ADODB_fenye.php. As you can see, we used it at the beginning:
include_once 'conn/smartyDaoImpl.php';
Here, please explain that the previous two files are placed in the conn folder, and the test cases are written in the same level directory as conn
Then view file, don’t put the view file in the wrong place. See this statement in the first class we wrote
$this->template_dir = BASE_PATH.SMARTY_PATH.'/templates/';
The absolute path to the view file is specified here, because,
$smarty->display('view/fenyeShow.html');//Specify the template for displaying data
This statement,
$smarty->display('view/fenyeShow.html');//Specify the template for displaying data
So, we are going to
BASE_PATH.SMARTY_PATH.'/templates/
Create a folder named view under the path, and then create a file named fenyeShow.html. Of course, you can choose a name you like, but you must ensure the consistency specified in the program
The content in the view template is as follows:
{$arr_page[id].Id} | {$arr_page[id].temperature} | {$arr_page[id].illumination} | {$arr_page[id].moisture} | {$arr_page[id].times|truncate:5:"..."} |
{$showpage} |
Okay, it’s done. Just locate the smarty_ADODB_fenye.php file in the browser, start the server, OK, and you will see the paging effect.
In the process of doing it, I also encountered some problems. One is that I cannot try the truncate statement. This problem is very strange. I checked it on the smarty official website and found that when the smarty subclass is instantiated, its parent class Smarty will not be instantiated. , therefore, will cause truncate to be unavailable. The solution is naturally to call the parent class constructor in the subclass.
Excerpted from 0+0+0+...=1
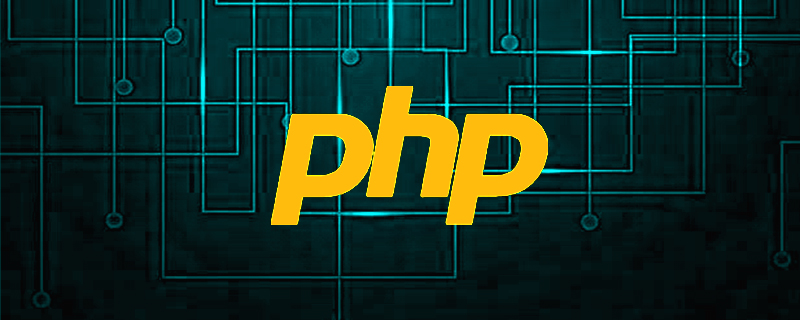
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
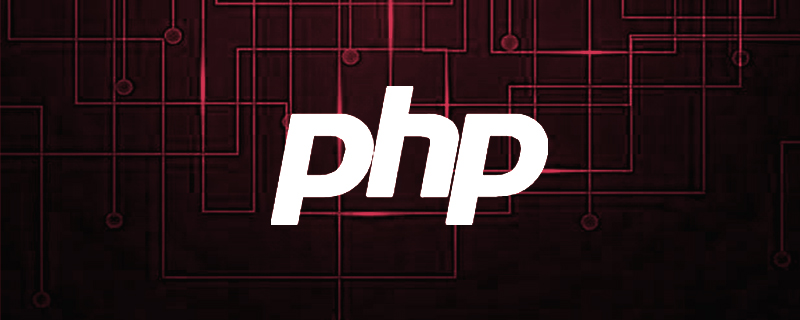
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
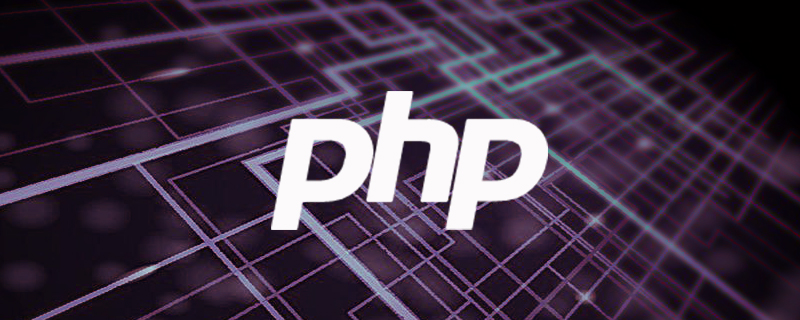
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
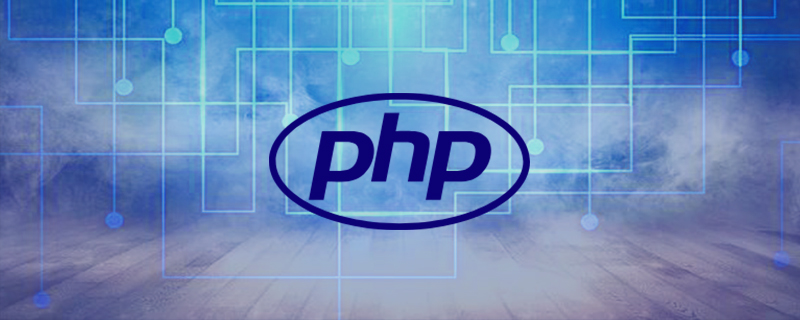
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
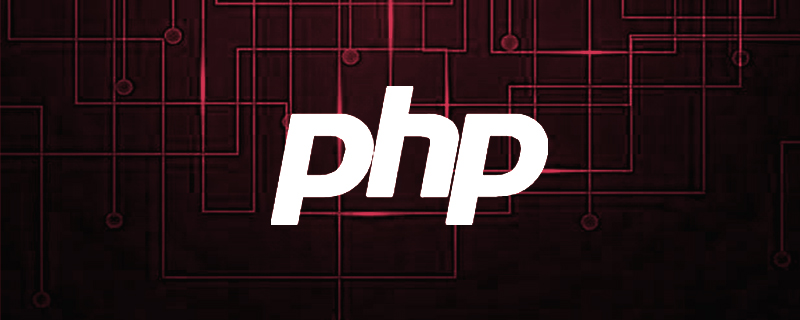
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
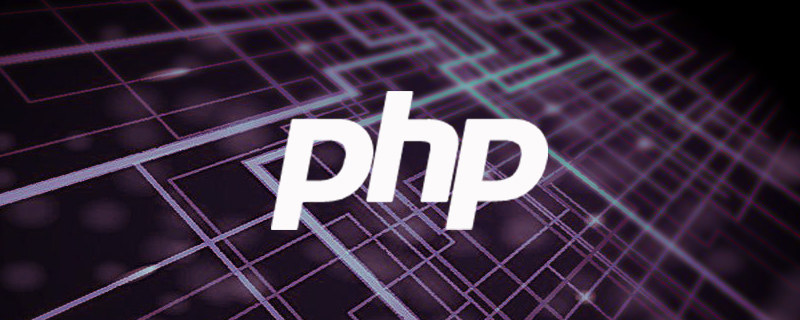
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
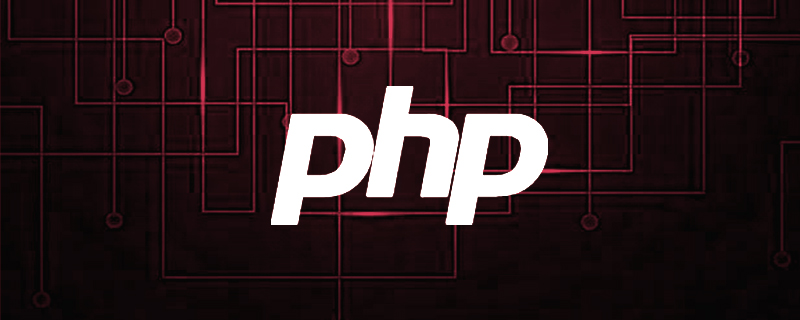
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
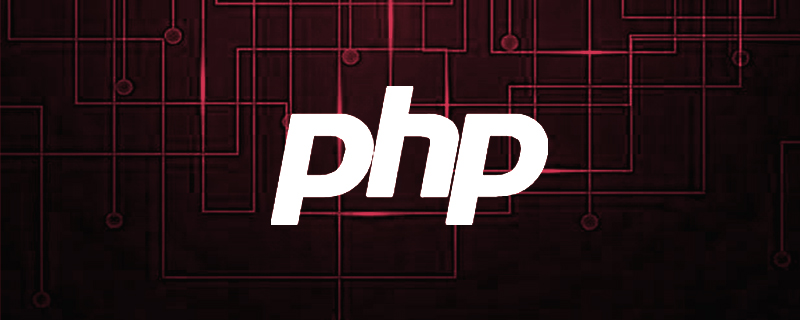
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
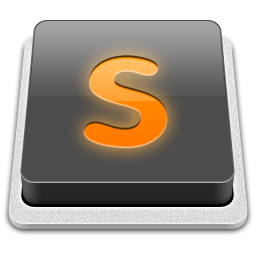
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.