


Detailed explanation of php mysql_real_escape_string anti-sql injection_PHP tutorial
Preventing sql injection is a must-do step in our program development. Let me introduce to you some methods of using mysql_real_escape_string to prevent sql injection in PHP and mysql development.
The mysql_real_escape_string() function escapes special characters in strings used in SQL statements. The following characters are affected:
代码如下 | 复制代码 |
x00 n r ' " x1a |
If successful, the function returns the escaped string. If failed, returns false.
Easy to use the function below to effectively filter.
The code is as follows | Copy code | ||||||||
if(get_magic_quotes_gpc()){ $s=stripslashes($s); } $s=mysql_real_escape_string($s);
} |
The code is as follows | Copy code | ||||
if(get_magic_quotes_gpc()) {
$_REQUEST = array_map( 'mysql_real_escape_string', $_REQUEST); |
代码如下 | 复制代码 |
$search=addslashes($search); $search=str_replace(“_”,”_”,$search); $search=str_replace(“%”,”%”,$search); |
The code is as follows | Copy code |
$id=intval($id); mysql_query=”select *from example where articieid=’$id’”; Or write like this: mysql_query(”SELECT * FROM article WHERE articleid=”.intval($id).””) |
The code is as follows | Copy code |
$search=addslashes($search); $search=str_replace(“_”,”_”,$search); $search=str_replace(“%”,”%”,$search); |
Of course you can also add PHP universal anti-injection code:
/***************************
PHP universal anti-injection security code
Description:
Determine whether the passed variable contains illegal characters
Such as $_POST, $_GET
Function:
Anti-injection
The code is as follows
|
Copy code
|
||||
********** ****************/ //Illegal characters to filter $ArrFiltrate=array(”‘”,”;”,”union”); //The URL to jump to after an error occurs. If not filled in, the previous page will be defaulted
$StrGoUrl=””; |
$ArrPostAndGet[]=$value;
foreach($ArrPostAndGet as $key=>$value){
/***************************
ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
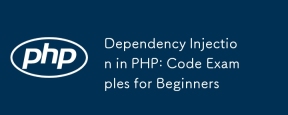
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
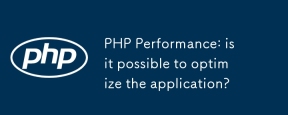
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
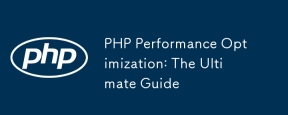
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
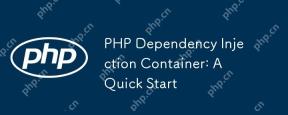
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
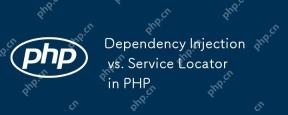
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
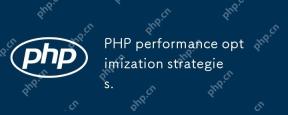
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
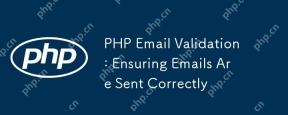
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
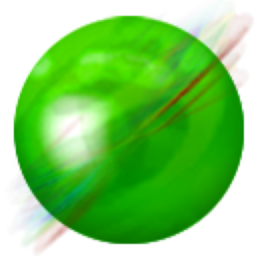
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
