


Detailed explanation of advanced operations of traversing arrays in PHP_PHP Tutorial
When traversing data in PHP, list, foreach, and each are generally used, but they may not be used in the following tutorials. Let me introduce to you some advanced examples of traversing arrays. I hope this method will be helpful to everyone. Helpful.
When learning programming language, always learn for, and then try to use while to write the effect of for and other exercises.
Let’s take a look at how to have the function of foreach before there is no foreach? Write (use while, list, and each to achieve).
See in this article: How to write the predecessor of PHP’s foreach
The code is as follows | Copy code | ||||||||||||
reset($attributes);
}
//do something }
|
The code is as follows | Copy code |
Array ( [0] => Array ( [name] => chess [price] => 12.99 ) [1] => Array ( [name] => checkers [price] => 9.99 ) [2] => Array ( [name] => backgammon [price] => 29.99 ) ) |
The code is as follows | Copy code |
function comparePrice($priceA, $priceB){ Return $priceA['price'] - $priceB['price']; } usort($games, 'comparePrice'); After executing this program fragment, the array will be sorted, and the result is as follows: Array ( [0] => Array ( [name] => checkers [price] => 9.99 ) [1] => Array ( [name] => chess [price] => 12.99 ) [2] => Array ( [name] => backgammon [price] => 29.99 ) ) |
To sort the array in descending order, just swap the positions of the two subtracted numbers in the comparePrice() function.
Traverse the array in reverse order
PHP's While Loop and For Loop are the most common methods of traversing an array. But how do you iterate over an array like the one below?
The code is as follows | Copy code | ||||||||||||
( [0] => Array
[name] => Board [games] => Array
(
(
|
The code is as follows | Copy code |
$iterator = new RecursiveArrayIterator($games); iterator_apply($iterator, 'navigateArray', array($iterator)); function navigateArray($iterator) { while ($iterator->valid()) { if ($iterator->hasChildren()) { NavigateArray($iterator->getChildren()); } else { Printf("%s: %s", $iterator->key(), $iterator->current()); } $iterator->next(); } } |
The code is as follows | Copy code |
Array ( [0] => Array ( [name] => checkers [price] => 9.99 ) [1] => Array ( [name] => chess [price] => 12.99 ) [2] => Array ( [name] => backgammon [price] => 29.99 ) ) |
It can be easily implemented using the array_reduce() function:
The code is as follows | Copy code | ||||||||
return ($game['price'] }
$names = array_filter($games, 'filterGames'); |
代码如下 | 复制代码 |
class Game { $game = new Game(); print_r(array($game));执行该例子就会产生如下结果: Array |
Convert object to array
代码如下 | 复制代码 |
class Game { public function setPrice($price) { $game = new Game(); print_r(array($game));执行该代码片段: Array |
The code is as follows | Copy code | ||||
class Game {
public $name;
|
The code is as follows | Copy code |
class Game { public $name; private $_price; public function setPrice($price) { $this->_price = $price; } } $game = new Game(); $game->name = 'chess'; $game->setPrice(12.99); print_r(array($game));Execute this code snippet: Array ( [0] => Game Object ( [name] => chess [_price:Game:private] => 12.99 ) ) |
The code is as follows | Copy code |
$arr = array( 0=>'madden2011.png', 1=>'madden2011-1.png', 2=>'madden2011-2.png', 3=>'madden2012.png' ); |
How do you sort this array? If you use sort() to sort the array, the result is like this:
代码如下 | 复制代码 |
Array ( [0] => madden2011-1.png [1] => madden2011-2.png [2] => madden2011.png [3] => madden2012.png ) |
Sometimes this is what we want, but what if we want to keep the original subscript? To solve this problem, you can use the natsort() function, which sorts the array in a natural way:
The code is as follows
|
Copy code | ||||
0=>'madden2011.png',
1=>'madden2011-1.png',
2=>'madden2011-2.png',
3=>'madden2012.png'
);
natsort($arr);
echo "
"; print_r($arr); echo "";
?> http://www.bkjia.com/PHPjc/632897.html
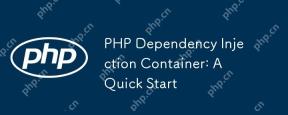
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
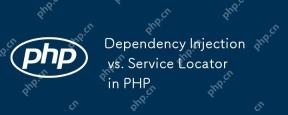
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
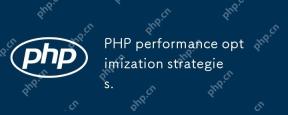
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
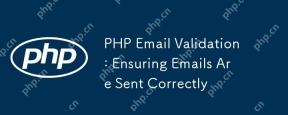
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
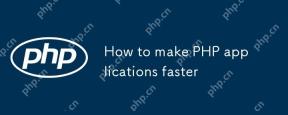
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
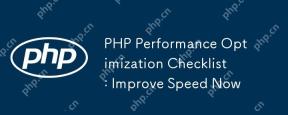
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
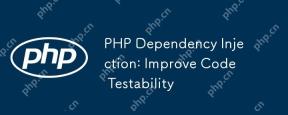
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
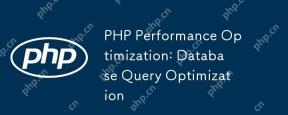
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
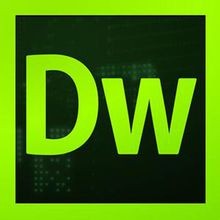
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
