


If your website has a membership system, you must have an automatic password retrieval function, which is the forgotten password function. If the user forgets the password, he can directly retrieve the password through his email or mobile phone number. Let me introduce the email retrieval function. Password method.
Setting ideas
1. Users need to provide an E-MAIL when registering. The purpose is to use this email to retrieve the password.
2. When the user forgets their password or user name, click the "Retrieve Password" hyperlink on the login page, open the form, enter the email address used for registration, and submit.
3. The system finds the user information from the database through the mailbox, and updates the user's password to a temporary password (for example: 12345678).
4. The system uses the Jmail function to send the user's information to the user's mailbox (the content includes: user name, temporary password, and prompts to remind the user to change the temporary password in time).
5. Users can log in with a temporary password.
HTML
We place a page on the password retrieval page that requires the user to enter the email address used for registration, and then submit the front-end js to handle the interaction.
The code is as follows | Copy code | ||||
Enter your registered email address to retrieve your password:
|
代码如下 | 复制代码 |
$(function(){ "+msg+"");} }); } }); }) |
The code is as follows | Copy code |
$(function(){
$("#sub_btn").click(function(){
var email = $("#email").val();
var preg = /^w+([-+.]w+)*@w+([-.]w+)*.w+([-.]w+)*/; //Match Email
If(email=='' || !preg.test(email)){
$("#chkmsg").html("Please fill in the correct email address!");
}else{
$("#sub_btn").attr("disabled","disabled").val('Submitting..').css("cursor","default");
$.post("sendmail.php",{mail:email},function(msg){
If(msg=="noreg"){
$("#sub_btn").removeAttr("disabled").val('submit').css("cursor","pointer");
}else{
$(".demo").html(""+msg+""); }); } }); }) |
The jQuery code used above is very convenient and concise to complete the front-end interactive operation. If you have a certain jQuery foundation, the above code is clear at a glance and does not require much explanation.
Of course, don’t forget to load the jQuery library file in the page. Some students often ask me why the demo downloaded from www.bKjia.c0m cannot be used. 80% of the time it is because the loading path of jquery or other files is wrong and it is not loaded. Necessary documents.
PHP
sendmail.php needs to verify whether the email exists in the system user table. If so, read the user information, encrypt the user ID, user name and password using md5 to generate a special string as a verification code to retrieve the password, and then Construct URL. At the same time, in order to control the timeliness of the URL link, we will record the operation time when the user submits the password retrieval action, and finally call the email sending class to send the email to the user's mailbox. The sending email class smtp.class.php has been packaged, please download it.
The code is as follows | Copy code | ||||
Please log in to your mailbox to reset your password in time! '; //Update data sending time mysql_query("update `t_user` set `getpasstime`='$getpasstime' where id='$uid '"); }else{ $msg = $result; } echo $msg; } //Send email function sendmail($time,$email,$url){ Include_once("smtp.class.php"); $smtpserver = ""; //SMTP server, such as smtp.163.com $smtpserverport = 25; //SMTP server port $smtpusermail = ""; //The user's email address of the SMTP server $smtpuser = ""; //SMTP server user account $smtppass = ""; //User password of SMTP server $smtp = new Smtp($smtpserver, $smtpserverport, true, $smtpuser, $smtppass); //A true here means that authentication is used, otherwise authentication is not used. $emailtype = "HTML"; //Email type, text: text; web page: HTML $smtpemailto = $email; $smtpemailfrom = $smtpusermail; $emailsubject = "www.bKjia.c0m - Retrieve password"; $emailbody = "Dear ".$email.": You submitted a password retrieval request at ".$time.". Please click the link below to reset your password (The button is valid for 24 hours). ".$url.""; $rs = $smtp->sendmail($smtpemailto, $smtpemailfrom, $emailsubject, $emailbody, $emailtype); Return $rs; } |
Okay, at this time, you will receive a password retrieval email from helloweba in your email. There is a URL link in the email content. Click the link to reset.php of www.bKjia.c0m to verify your email.
The code is as follows | Copy code | ||||
$token = stripslashes(trim($_GET['token'])); $email = stripslashes(trim($_GET['email'])); $sql = "select * from `t_user` where email='$email'"; $query = mysql_query($sql); $row = mysql_fetch_array($query); if($row){ $mt = md5($row['id'].$row['username'].$row['password']); If($mt==$token){ If(time()-$row['getpasstime']>24*60*60){ $msg = 'This link has expired! '; }else{ //Reset password... Please reset the password, displays the reset password form, is displayed. This is just a demonstration, skip it. '; }else{ $msg = 'Invalid link'; } }else{ $msg = 'Wrong link! '; } echo $msg;
|
Summary: Through registered email verification and password retrieval via email in this article, we know the application of sending emails in website development and its importance. Of course, SMS verification applications are also popular now. This requires related SMS interface docking. .
Finally, attach the data table t_user structure:
代码如下 | 复制代码 |
CREATE TABLE `t_user` ( |
The code is as follows | Copy code |
CREATE TABLE `t_user` ( `id` int(11) NOT NULL auto_increment, `username` varchar(30) NOT NULL, `password` varchar(32) NOT NULL, `email` varchar(50) NOT NULL, `getpasstime` int(10) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=utf8; |
smtp.class.php类文件
代码如下 | 复制代码 |
class Smtp{ /* Public Variables */ var $smtp_port; var $time_out; var $host_name; var $log_file; var $relay_host; var $debug; var $auth; var $user; var $pass; /* Private Variables */ /* Constractor */ function smtp($relay_host = "", $smtp_port = 25, $auth = false, $user, $pass) { $this->smtp_port = $smtp_port; $this->relay_host = $relay_host; $this->time_out = 30; //is used in fsockopen() $this->auth = $auth; //auth $this->user = $user; $this->pass = $pass; $this->host_name = "localhost"; //is used in HELO command $this->sock = false; /* Main Function */ function sendmail($to, $from, $subject = "", $body = "", $mailtype, $cc = "", $bcc = "", $additional_headers = "") { $body = ereg_replace("(^|(rn))(.)", "1.3", $body); $header .= "MIME-Version:1.0rn"; if ($mailtype == "HTML") { $header .= "To: " . $to . "rn"; if ($cc != "") { $header .= "From: $fromrn"; $header .= "Subject: " . $subject . "rn"; $header .= $additional_headers; $header .= "Date: " . date("r") . "rn"; $header .= "X-Mailer:By Redhat (PHP/" . phpversion() . ")rn"; list ($msec, $sec) = explode(" ", microtime()); $header .= "Message-ID: rn"; $TO = explode(",", $this->strip_comment($to)); if ($cc != "") { if ($bcc != "") { $sent = true; foreach ($TO as $rcpt_to) { if (!$this->smtp_sockopen($rcpt_to)) { $sent = false; continue; if ($this->smtp_send($this->host_name, $mail_from, $rcpt_to, $header, $body)) { $sent = false; fclose($this->sock); $this->log_write("Disconnected from remote hostn"); return $sent; /* Private Functions */ function smtp_send($helo, $from, $to, $header, $body = "") { if (!$this->smtp_putcmd("", base64_encode($this->pass))) { if (!$this->smtp_putcmd("MAIL", "FROM:")) { if (!$this->smtp_putcmd("RCPT", "TO:")) { if (!$this->smtp_putcmd("DATA")) { if (!$this->smtp_message($header, $body)) { if (!$this->smtp_eom()) { if (!$this->smtp_putcmd("QUIT")) { return true; function smtp_sockopen($address) { function smtp_sockopen_relay() { $this->sock = @ fsockopen($this->relay_host, $this->smtp_port, $errno, $errstr, $this->time_out); if (!($this->sock && $this->smtp_ok())) { $this->log_write("Error: " . $errstr . " (" . $errno . ")n"); return false; $this->log_write("Connected to relay host " . $this->relay_host . "n"); return true; function smtp_sockopen_mx($address) { if (!@ getmxrr($domain, $MXHOSTS)) { return false; foreach ($MXHOSTS as $host) { $this->sock = @ fsockopen($host, $this->smtp_port, $errno, $errstr, $this->time_out); if (!($this->sock && $this->smtp_ok())) { $this->log_write("Error: " . $errstr . " (" . $errno . ")n"); continue; $this->log_write("Connected to mx host " . $host . "n"); return true; $this->log_write("Error: Cannot connect to any mx hosts (" . implode(", ", $MXHOSTS) . ")n"); return false; function smtp_message($header, $body) { $this->smtp_debug("> " . str_replace("rn", "n" . "> ", $header . "n> " . $body . "n> ")); return true; function smtp_eom() { $this->smtp_debug(". [EOM]n"); return $this->smtp_ok(); function smtp_ok() { $this->smtp_debug($response . "n"); if (!ereg("^[23]", $response)) { fgets($this->sock, 512); $this->log_write("Error: Remote host returned "" . $response . ""n"); return false; return true; function smtp_putcmd($cmd, $arg = "") { else fputs($this->sock, $cmd . "rn"); $this->smtp_debug("> " . $cmd . "n"); return $this->smtp_ok(); function smtp_error($string) { return false; function log_write($message) { if ($this->log_file == "") { $message = date("M d H:i:s ") . get_current_user() . "[" . getmypid() . "]: " . $message; if (!@ file_exists($this->log_file) || !($fp = @ fopen($this->log_file, "a"))) { return false; flock($fp, LOCK_EX); fputs($fp, $message); fclose($fp); return true; function strip_comment($address) { while (ereg($comment, $address)) { return $address; function get_address($address) { $address = ereg_replace("^.*.*$", "1", $address); return $address; function smtp_debug($message) { |
最后面有个数据库连接类,这里就不介绍了大大家可以百本站找相关的数据库连接mysql类哦。
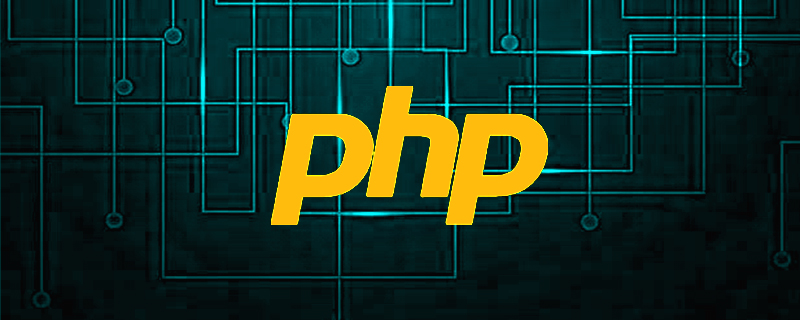
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
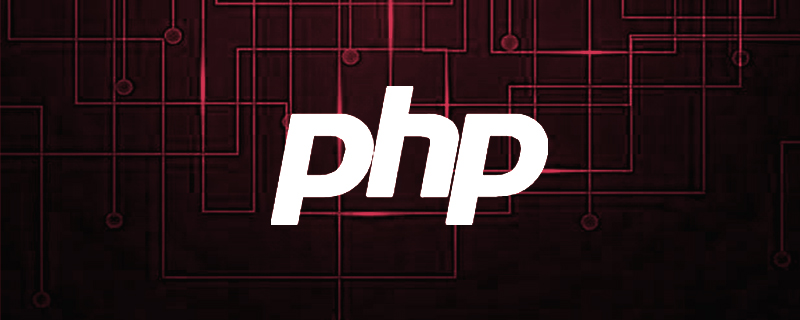
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
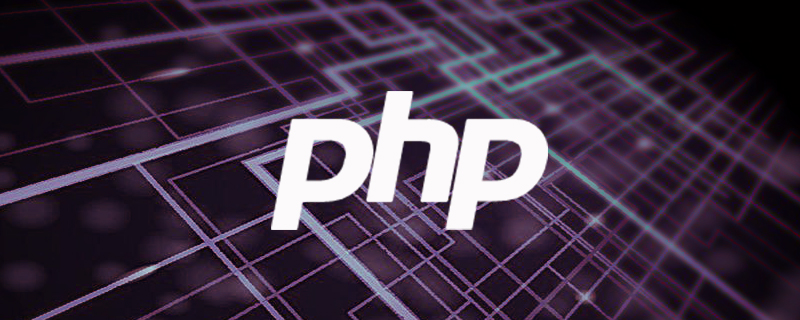
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
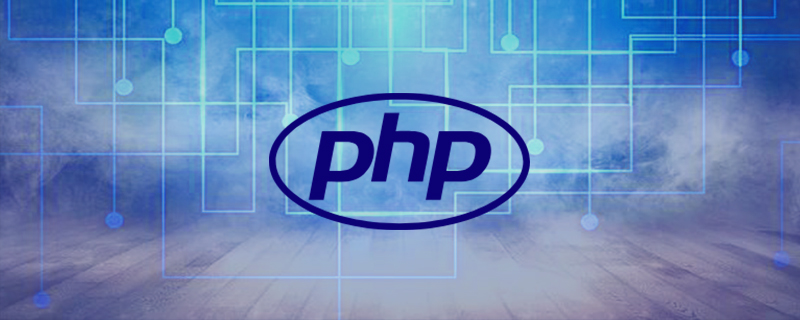
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
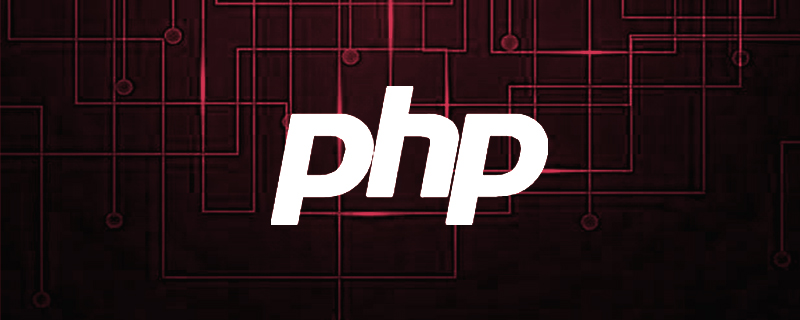
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
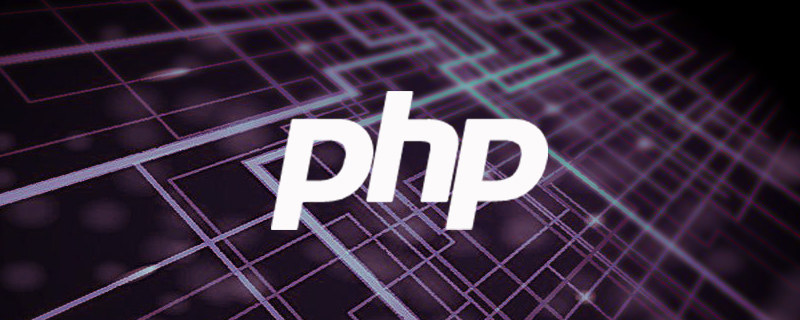
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
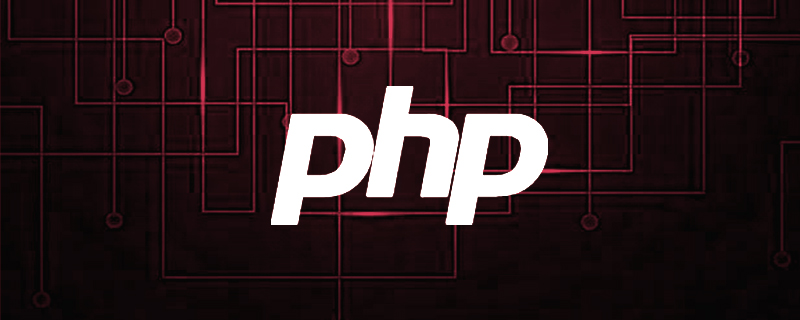
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
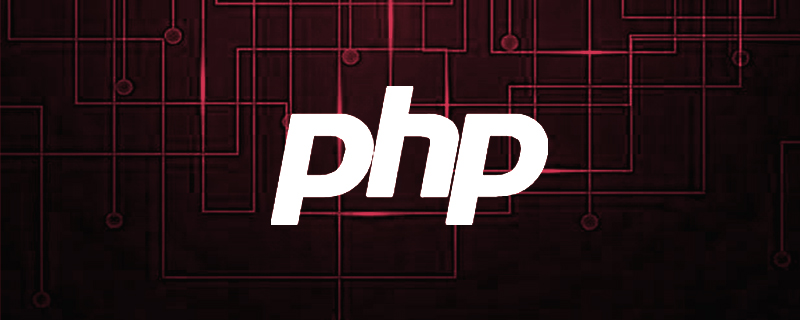
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
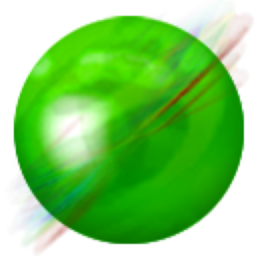
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
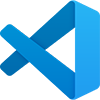
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.