


Detailed explanation of Laravel framework form verification, laravel framework form
Basic verification example
$validator = Validator::make(
array('name' => 'Dayle'),
array('name' => 'required|min:5')
);
The first parameter passed to the make function is the data to be verified, and the second parameter is the verification rule that needs to be applied to the data.
Multiple validation rules can be separated by the "|" character, or as a single element of an array.
Specify validation rules via array
$validator = Validator::make(
array('name' => 'Dayle'),
array('name' => array('required', 'min:5'))
);
Once a Validator instance is created, this validation can be performed using the fails (or passes) function.
if ($validator->fails())
{
// The given data did not pass validation
}
If validation fails, you can get an error message from the validator.
$messages = $validator->messages();
You can also use the failed function to get an array of rules that failed validation without an error message.
$failed = $validator->failed();
Document Verification
The Validator class provides some validation rules for validating files, such as size, mimes, etc. When validating a file, you can pass it to the validator just like any other validation.
With error message
After calling the messages function on a Validator instance, you will get a MessageBag instance, which has many convenient functions for handling error messages.
Get the first error message for a domain
echo $messages->first('email');
Get all error messages for a domain
foreach ($messages->get('email') as $message)
{
//
}
Get all error messages for all domains
foreach ($messages->all() as $message)
{
//
}
Check if messages exist for a domain
if ($messages->has('email'))
{
//
}
Get an error message in some format
echo $messages->first('email', '
:message
');Note: By default, messages will be formatted using Bootstrap-compatible syntax.
Get all error messages in a certain format
foreach ($messages->all('
{
//
}
Error Messages & Views
Once you've performed validation, you need an easy way to feed error messages back to the view. This can be easily handled in Lavavel. Take the following route as an example:
Route::get('register', function()
{
return View::make('user.register');
});
Route::post('register', function()
{
$rules = array(...);
$validator = Validator::make(Input::all(), $rules);
if ($validator->fails())
{
return Redirect::to('register')->withErrors($validator);
}
});
Note that when validation fails, we use the withErrors function to pass the Validator instance to Redirect. This function will refresh the error message saved in the Session so that it is available on the next request.
However, note that we do not have to explicitly bind the error message to the route in the GET route. This is because Laravel always checks the Session for errors and automatically binds them to the view if they are available. So, for every request, a $errors variable is always available in all views, allowing you to conveniently assume that $errors is always defined and safe to use. The $errors variable will be an instance of the MessageBag class.
So, after the jump, you can use the auto-bound $errors variable in the view:
first('email'); ?>
Available validation rules
Here is a list of all available validation rules and their functions:
Accepted
Active URL
After (Date)
Alpha
Alpha Dash
Alpha Numeric
Before (Date)
Between
Confirmed
Date
Date Format
Different
Exists (Database)
Image (File)
In
Integer
IP Address
Max
MIME Types
Min
Not In
Numeric
Regular Expression
Required
Required If
Required With
Required Without
Same
Size
Unique (Database)
accepted
Validate that the value of this rule must be yes, on, or 1. This is useful when verifying agreement with the Terms of Service.
active_url
Verifies that the value of this rule must be a valid URL, according to the PHP function checkdnsrr.
after:date
Validates that the value of this rule must be after the given date, which will be passed via the PHP function strtotime.
alpha
The value validating this rule must consist entirely of alphabetic characters.
alpha_dash
Validate that the value of this rule must consist entirely of letters, numbers, dashes, or underline characters.
alpha_num
The value validating this rule must consist entirely of letters and numbers.
before:date
Validates that the value of this rule must be before the given date, which will be passed through the PHP function strtotime.
between:min,max
Validates that the value of this rule must be between the given min and max. Strings, numbers, and files are compared using size rules.
confirmed
The value of this validation rule must be the same as the value of foo_confirmation. For example, if the field that needs to be validated for this rule is password, there must be an identical password_confirmation field in the input.
date
Validates that the value of this rule must be a valid date, according to the PHP function strtotime.
date_format:format
Validates that the value of this rule must conform to the given format, according to the PHP function date_parse_from_format.
different:field
Validates that the value of this rule must be different from the value of the specified field.
email
Validate that the value of this rule must be a valid email address.
exists:table,column
Validates that the value of this rule must exist in the specified database table.
Basic usage of Exists rules
Specify column name
'state' => 'exists:states,abbreviation'
You can also specify more conditions, which will be added to the query in the form of "where".
'email' => 'exists:staff,email,account_id,1'
image
The value validating this rule must be an image (jpeg, png, bmp or gif).
in:foo,bar,...
Validates that the value of this rule must exist in the given list.
integer
Validate that the value of this rule must be an integer.
Verify that the value of this rule must be a valid IP address.
max:value
Validate that the value of this rule must be less than the maximum value. Strings, numbers, and files are compared using size rules.
mimes:foo,bar,...
The MIME type of the file validating this rule must be in the given list.
Basic use of MIME rules
'photo' => 'mimes:jpeg,bmp,png'
min:value
The value that validates this rule must be greater than the minimum value. Strings, numbers, and files are compared using size rules.
not_in:foo,bar,...
Validates that the value of this rule must not exist in the given list.
numeric
Validate that the value of this rule must be a number.
regex:pattern
Validates that the value of this rule must match the given regular expression.
Note: When using regex mode, it is necessary to use an array to specify the rules instead of a pipe delimiter, especially when the regular expression contains a pipe character.
required
Validate that the value of this rule must exist in the input data.
required_if:field,value
When the specified field is a certain value, the value of this rule must exist.
required_with:foo,bar,...
Validate that the value of this rule must exist only if the specified domain exists.
required_without:foo,bar,...
Validate that the value of this rule must exist only if the specified domain does not exist.
same:field
Validate that the value of this rule must be the same as the value of the given domain.
size:value
Validates that the value of this rule must be the same size as the given value. For strings, value represents the number of characters; for numbers, value represents its integer value; for files, value represents the size of the file in KB.
unique:table,column,except,idColumn
Validates that the value of this rule must be unique within the given database table. If column is not specified, the name of the field will be used.
Basic use of Unique rules
'email' => 'unique:users'
Specify column name
'email' => 'unique:users,email_address'
Force ignore a given ID
'email' => 'unique:users,email_address,10'
url
Validate that the value of this rule must be a valid URL.
Customized error message
If necessary, you can use a custom error message instead of the default one. There are several ways to customize error messages.
Pass custom message to validator
$messages = array(
'required' => 'The :attribute field is required.',
);
$validator = Validator::make($input, $rules, $messages);
Note: The :attribute placeholder will be replaced by the name of the actual domain being validated. You can also use other placeholders in the error message.
Other validation placeholders
$messages = array(
'same' => 'The :attribute and :other must match.',
'size' => 'The :attribute must be exactly :size.',
'between' => 'The :attribute must be between :min - :max.',
'in' => 'The :attribute must be one of the following types:
:values',
);
Sometimes, you may want to specify a customized error message only for a specific domain:
Specify customized error messages for a specific domain
$messages = array(
'email.required' => 'We need to know your e-mail address!',
);
In some cases, you may want to specify error messages in a language file rather than passing them directly to the Validator. To achieve this, add your custom message to the custom array in the app/lang/xx/validation.php file.
Specify error message in language file
'custom' => array(
'email' => array(
'required' => 'We need to know your e-mail address!',
),
),
Customized verification rules
Laravel provides a range of useful validation rules; however, you may wish to add your own. One way is to register custom validation rules using the Validator::extend function:
Register a custom validation rule
Validator::extend('foo', function($attribute, $value, $parameters)
{
return $value == 'foo';
});
Note: The name of the rule passed to the extend function must comply with the "snake cased" naming rule.
The custom validator accepts three parameters: the name of the attribute to be verified, the value of the attribute to be verified, and the parameters passed to this rule.
You can also pass a class function to the extend function instead of using a closure:
Validator::extend('foo', 'FooValidator@validate');
Note that you need to define error messages for your custom rules. You can either use an inline array of custom messages or add them in the validation language file.
You can also extend the Validator class itself instead of extending the validator with a closure callback. To achieve this, add a validator class that inherits from IlluminateValidationValidator. You can add a validation function starting with validate in the class:
Extended validator class
class CustomValidator extends IlluminateValidationValidator {
public function validateFoo($attribute, $value, $parameters)
{
return $value == 'foo';
}
}
Next, you need to register your custom validator extension:
You need to register a custom validator extension
Validator::resolver(function($translator, $data, $rules, $messages)
{
return new CustomValidator($translator, $data, $rules, $messages);
});
When creating a custom validation rule, you sometimes need to define a custom placeholder for the error message. To achieve this, you can create a custom validator like above and add a replaceXXX function in the validator:
protected function replaceFoo($message, $attribute, $rule, $parameters)
{
return str_replace(':foo', $parameters[0], $message);
}
You can write a special summary of methods.
For example:
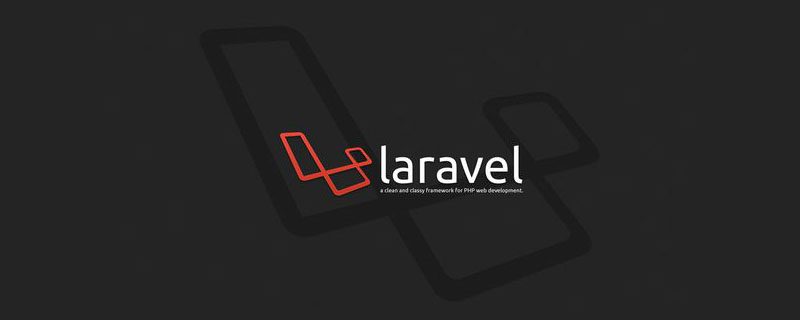
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于单点登录的相关问题,单点登录是指在多个应用系统中,用户只需要登录一次就可以访问所有相互信任的应用系统,下面一起来看一下,希望对大家有帮助。
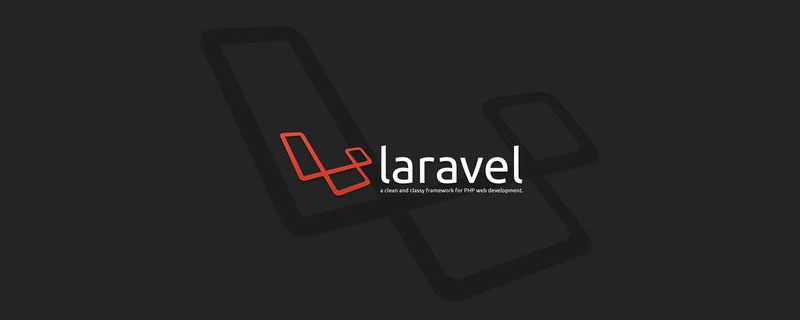
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于Laravel的生命周期相关问题,Laravel 的生命周期从public\index.php开始,从public\index.php结束,希望对大家有帮助。
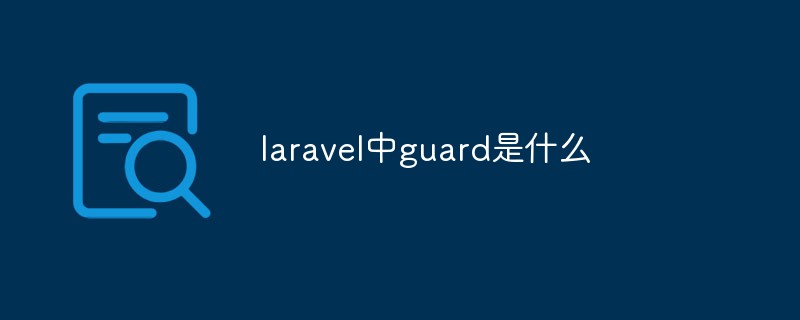
在laravel中,guard是一个用于用户认证的插件;guard的作用就是处理认证判断每一个请求,从数据库中读取数据和用户输入的对比,调用是否登录过或者允许通过的,并且Guard能非常灵活的构建一套自己的认证体系。
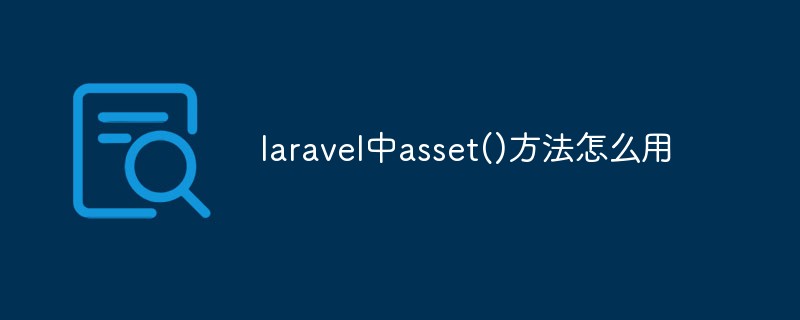
laravel中asset()方法的用法:1、用于引入静态文件,语法为“src="{{asset(‘需要引入的文件路径’)}}"”;2、用于给当前请求的scheme前端资源生成一个url,语法为“$url = asset('前端资源')”。
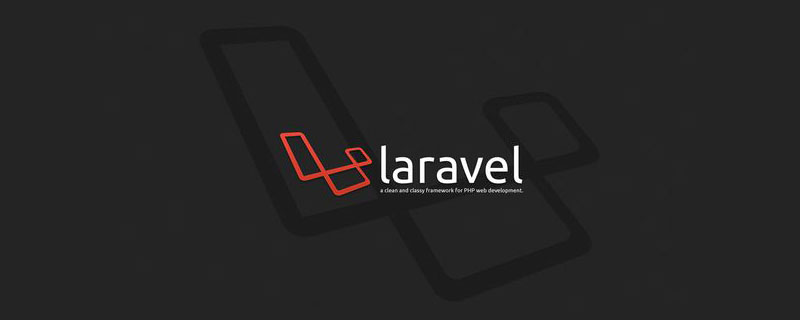
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于使用中间件记录用户请求日志的相关问题,包括了创建中间件、注册中间件、记录用户访问等等内容,下面一起来看一下,希望对大家有帮助。
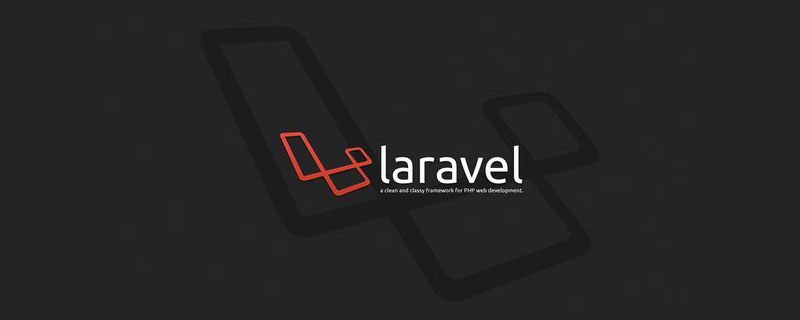
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于中间件的相关问题,包括了什么是中间件、自定义中间件等等,中间件为过滤进入应用的 HTTP 请求提供了一套便利的机制,下面一起来看一下,希望对大家有帮助。
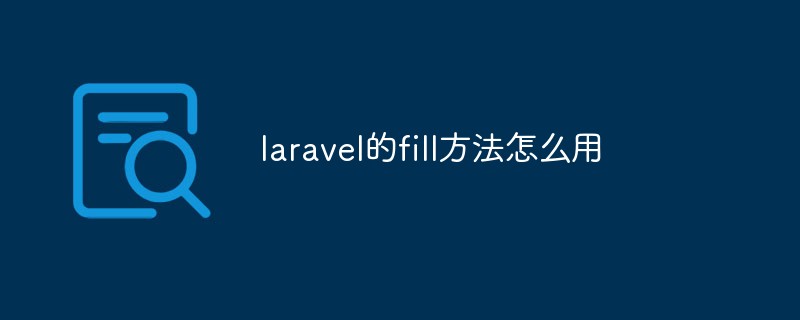
在laravel中,fill方法是一个给Eloquent实例赋值属性的方法,该方法可以理解为用于过滤前端传输过来的与模型中对应的多余字段;当调用该方法时,会先去检测当前Model的状态,根据fillable数组的设置,Model会处于不同的状态。
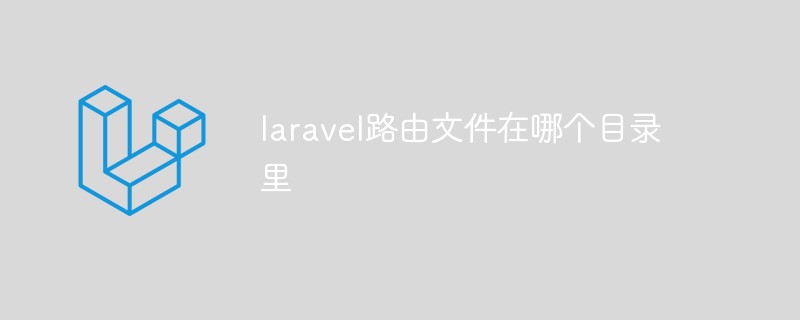
laravel路由文件在“routes”目录里。Laravel中所有的路由文件定义在routes目录下,它里面的内容会自动被框架加载;该目录下默认有四个路由文件用于给不同的入口使用:web.php、api.php、console.php等。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
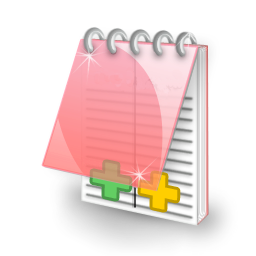
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
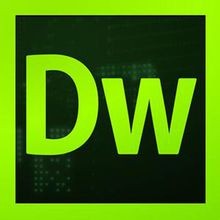
Dreamweaver CS6
Visual web development tools
