


A relatively complete shopping cart class implemented in PHP, php shopping cart class_PHP tutorial
A relatively complete shopping cart class implemented in PHP, php shopping cart class
The example in this article describes a relatively complete shopping cart class implemented in PHP. Share it with everyone for your reference. The specific implementation method is as follows:
I recently completed a project that required the use of a shopping cart. Considering that it may be used frequently, I encapsulated it into a class so that it can be called later. Interested readers can simply modify this class slightly and use it. It’s in my own program.
/* /* file type: Contains files, the recommended suffix is .inc /* /* file name: cart.inc /* /* Description: Define a car purchase category */
/* /* Func list: class cart /* /* author: bigeagle /* /* /*****************************************************************************/
//Define constants for this file
define("_CART_INC_" , "exists") ;
/*Shopping cart class*/
class TCart
{
var $SortCount; //Number of product categories
var $TotalCost; //Total value of goods
var $Id; //ID of each type of product (array)
var $Name; //The name of each type of product (array)
var $Price; //The price of each type of product (array)
var $Discount; //Product discount (array)
var $GoodPrice ; //The preferential price of the product (array)
var $Count; //The number of pieces of each type of product (array)
var $MaxCount ; //Product limit (array)
//******构造函数
function TCart()
{
$this->SortCount=0;
session_start(); //初始化一个session
session_register('sId');
session_register('sName');
session_register('sPrice');
session_register('sDiscount');
session_register('sGoodPrice') ;
session_register('sCount') ;
session_register('sMaxCount') ;
$this->Update();
$this->Calculate();
}
//********私有,根据session的值更新类中相应数据
function Update()
{
global $sId,$sName,$sPrice,$sCount,$sDiscount,$sMaxCount,$sGoodPrice;
if(!isset($sId) or !isset($sName) or !isset($sPrice)
or !isset($sDiscount) or !isset($sMaxCount)
or !isset($sGoodPrice) or !isset($sCount)) return;
$this->Id =$sId;
$this->Name =$sName;
$this->Price =$sPrice;
$this->Count =$sCount;
$this->Discount = $sDiscount ;
$this->GoodPrice = $sGoodPrice ;
$this->MaxCount = $sMaxCount ;
//计算商品总数
$this->SortCount=count($sId);
}
//********私有,根据新的数据计算每类商品的价值及全部商品的总价
function Calculate()
{
for($i=0;$iSortCount;$i++)
{
/*计算每件商品的价值,如果折扣是0 ,则为优惠价格*/
$GiftPrice = ($this->Discount[$i] == 0 ? $this->GoodPrice :
ceil($this->Price[$i] * $this->Discount[$i])/100 );
$this->TotalCost += $GiftPrice * $this->Count[$i] ;
}
}
//**************The following are interface functions
//*** Add an item
// Determine whether there is already one in the blue, if so, add count, otherwise add a new product
//First, change the value of the session, and then call update() and calculate() to update the member variables
function Add($a_ID , $a_Name , $a_Price , $a_Discount ,
$a_GoodPrice , $a_MaxCount , $a_Count)
{
global $sId , $sName , $sCount , $sPrice , $sDiscount ,
$sGoodPrice , $sMaxCount ;
$k=count($sId);
for ($i=0; $i { //First check if this product has been added
If($sId[$i]==$a_ID)
{
$sCount[$i] += $a_Count ;
break;
}
}
if($i >= $k)
{ //If not, add a new product category
$sId[] = $a_ID;
$sName[] = $a_Name;
$sPrice[] = $a_Price;
$sCount[] = $a_Count;
$sGoodPrice[] = $a_GoodPrice;
$sDiscount[] = $a_Discount;
$sMaxCount[] = $a_MaxCount;
}
$this->Update(); //Update the member data of the class
$this->Calculate();
}
//Remove an item
function Remove($a_ID)
{
global $sId , $sName , $sCount , $sPrice , $sDiscount ,
$sGoodPrice , $sMaxCount ;
$k = count($sId);
for($i=0; $i {
If($sId[$i] == $a_ID)
{
$sCount[$i] = 0;
break;
}
}
$this->Update();
$this->Calculate();
}
//Change the number of items
function ModifyCount($a_i,$a_Count)
{
global $sCount;
$sCount[$a_i] = $a_Count ;
$this->Update();
$this->Calculate();
}
/*****************************
Clear all products
*******************************/
function RemoveAll()
{
session_unregister('sId');
Session_unregister('sName');
Session_unregister('sPrice');
Session_unregister('sDiscount');
session_unregister('sGoodPrice') ;
session_unregister('sCount') ;
Session_unregister('sMaxCount') ;
$this->SortCount = 0;
$this->TotalCost = 0;
}
//Whether a certain product is already in the blue, the parameter is the ID of this product
function Exists($a_ID)
{
for($i=0; $iSortCount; $i++)
{
If($this->Id[$i]==$a_ID) return TRUE;
}
Return FALSE;
}
//The position of a certain item in the blue
function IndexOf($a_ID)
{
for($i=0; $iSortCount; $i++)
{
If($this->Id[$i]==$id) return $i;
}
Return 0;
}
//Get information about a product, main working function
//Return an associative array,
function Item($i)
{
$Result[id] = $this->Id[$i];
$Result[name] = $this->Name[$i];
$Result[price] = $this->Price[$i];
$Result[count] = $this->Count[$i];
$Result[discount] = $this->Discount[$i] ;
$Result[goodprice] = $this->GoodPrice[$i] ;
$Result[maxcount] = $this->MaxCount[i];
Return $Result;
}
//Get the total number of product categories
function CartCount()
{
Return $this->SortCount;
}
//Get the total product value
function GetTotalCost()
{
Return $this->TotalCost;
}
}
?>
I hope this article will be helpful to everyone’s PHP programming design.
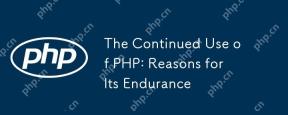
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
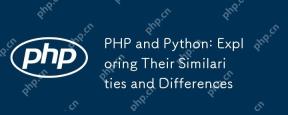
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
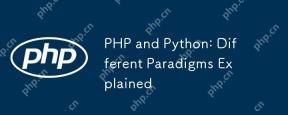
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
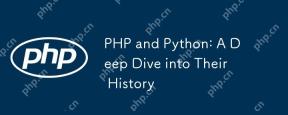
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
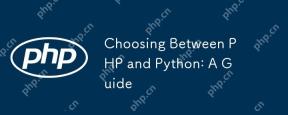
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
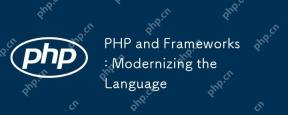
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
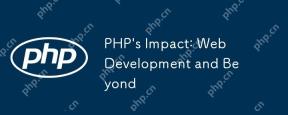
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
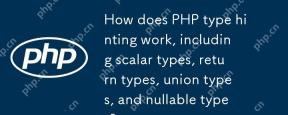
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
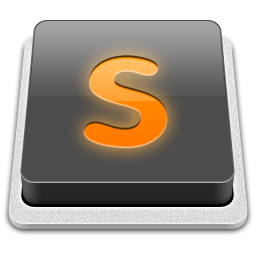
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor