How to start multiple processes in php_PHP tutorial
How to start multiple processes in php
This article explains how to start multiple processes in php. Share it with everyone for your reference. The specific implementation method is as follows:
The code is as follows:
$IP='192.168.1.1';//IP of Windows computer
$Port='5900'; //Port used by VNC
$ServerPort='9999';//Port used by Linux Server externally
$RemoteSocket=false;//Connect to VNC’s Socket
function SignalFunction($Signal){
//This is the message processing function of the main Process
global $PID;//Child Process’s PID
switch ($Signal)
{
case SIGTRAP:
case SIGTERM:
//Receive the Signal to end the program
if($PID)
{
//Send a SIGTERM signal to Child to tell him to finish it quickly
posix_kill($PID,SIGTERM);
//Wait for the Child Process to end to avoid zombie
pcntl_wait($Status);
}
//Close the Socket opened by the main Process
DestroySocket();
exit(0); //End the main Process
break;
case SIGCHLD:
/*
When the Child Process ends, Child will send a SIGCHLD signal to Parent
When Parent receives SIGCHLD, it knows that the Child Process has ended and it should do something
Ending action*/
unset($PID); //Clear $PID to indicate that the Child Process has ended
pcntl_wait($Status); //Avoid Zombie
break;
default:
}
}
function ChildSignalFunction($Signal){
//This is the message processing function of Child Process
switch ($Signal)
{
case SIGTRAP:
case SIGTERM:
//Child Process receives the end message
DestroySocket(); //Close Socket
exit(0); //End Child Process
default:
}
}
function ProcessSocket($ConnectedServerSocket){
//Child Process Socket processing function
//$ConnectedServerSocket -> Externally connected Socket
global $ServerSocket,$RemoteSocket,$IP,$Port;
$ServerSocket=$ConnectedServerSocket;
declare(ticks = 1); //This line must be added, otherwise the message processing function cannot be set.
//Set message processing function
if(!pcntl_signal(SIGTERM, "ChildSignalFunction")) return;
if(!pcntl_signal(SIGTRAP, "ChildSignalFunction")) return;
//Establish a Socket connected to VNC
$RemoteSocket=socket_create(AF_INET, SOCK_STREAM,SOL_TCP);
//Connect to the internal VNC
@$RemoteConnected=socket_connect($RemoteSocket,$IP,$Port);
if(!$RemoteConnected) return; //Unable to connect to VNC end
//Set Socket processing to Nonblock to prevent the program from being blocked
if(!socket_set_nonblock($RemoteSocket)) return;
if(!socket_set_nonblock($ServerSocket)) return;
while(true)
{
//Here we use pooling to obtain data
$NoRecvData=false; //This variable is used to determine whether the external connection has read data
$NoRemoteRecvData=false;//This variable is used to determine whether the VNC connection has read data
@$RecvData=socket_read($ServerSocket,4096,PHP_BINARY_READ);
//Read 4096 bytes of data from external connection
@$RemoteRecvData=socket_read($RemoteSocket,4096,PHP_BINARY_READ);
//Read 4096 bytes of data from the vnc connection
if($RemoteRecvData==='')
{
//VNC connection is interrupted, it’s time to end
echo"Remote Connection Closen";
return;
}
if($RemoteRecvData===false)
{
/*
Since we are using nonblobk mode
The situation here is that the vnc connection has no data to read
*/
$NoRemoteRecvData=true;
//Clear Last Error
socket_clear_error($RemoteSocket);
}
if($RecvData==='')
{
//The external connection is interrupted, it’s time to end
echo "Client Connection Closen";
return;
}
if($RecvData===false)
{
/*
Since we are using nonblobk mode
The situation here is that the external connection has no data to read
*/
$NoRecvData=true;
//Clear Last Error
socket_clear_error($ServerSocket);
}
if($NoRecvData&&$NoRemoteRecvData)
{
//If neither the external connection nor the VNC connection has data to read,
//Let the program sleep for 0.1 seconds to avoid long-term use of CPU resources
usleep(100000);
//After waking up, continue the pooling action to read the socket
continue;
}
//Recv Data
if(!$NoRecvData)
{
//External connection reads data
while(true)
{
//Transfer the data read from the external connection to the VNC connection
@$WriteLen=socket_write($RemoteSocket,$RecvData);
if($WriteLen===false)
{
//Due to network transmission problems, data cannot be written at the moment
//Sleep for 0.1 seconds before trying again.
usleep(100000);
continue;
}
if($WriteLen===0)
{
//The remote connection is interrupted, the program should end
echo"Remote Write Connection Closen";
return;
}
//When the data read from the external connection has been completely sent to the VNC connection, this loop is interrupted.
if($WriteLen==strlen($RecvData)) break;
//If the data cannot be sent in one go, it will have to be split into several transmissions until all the data is sent
$RecvData=substr($RecvData,$WriteLen);
}
}
if(!$NoRemoteRecvData)
{
//Here is the data read from the VNC connection and then transferred back to the external connection
//The principle is almost the same as above and I won’t go into details
while(true)
{
@$WriteLen=socket_write($ServerSocket,$RemoteRecvData);
if($WriteLen===false)
{
usleep(100000);
continue;
}
if($WriteLen===0)
{
echo"Remote Write Connection Closen";
return;
}
if($WriteLen==strlen($RemoteRecvData)) break;
$RemoteRecvData=substr($RemoteRecvData,$WriteLen);
}
}
}
}
function DestroySocket(){
//Used to close the opened Socket
global$ServerSocket,$RemoteSocket;
if($RemoteSocket)
{
//If VNC connection has been enabled
//You must shut down the Socket before closing the Socket, otherwise the other party will not know that you have closed the connection
@socket_shutdown($RemoteSocket,2);
socket_clear_error($RemoteSocket);
//Close Socket
socket_close($RemoteSocket);
}
//Close external connections
@socket_shutdown($ServerSocket,2);
socket_clear_error($ServerSocket);
socket_close($ServerSocket);
}
//This is the beginning of the entire program, and the program starts executing from here
//First execute a fork
here $PID=pcntl_fork();
if($PID==-1) die("could not fork");
//If $PID is not 0, it means this is a Parent Process
//$PID is Child Process
//This is the Parent Process. End it yourself and let Child become a Daemon.
if($PID) die("Daemon PID:$PIDn");
//From here, Daemon mode is executed
//Detach the current Process from the terminal and enter daemon mode
if(!posix_setsid()) die("could not detach from terminaln");
//Set the daemon's message processing function
declare(ticks = 1);
if(!pcntl_signal(SIGTERM, "SignalFunction")) die("Error!!!n");
if(!pcntl_signal(SIGTRAP, "SignalFunction")) die("Error!!!n");
if(!pcntl_signal(SIGCHLD, "SignalFunction")) die("Error!!!n");
//Establish a Socket for external connection
$ServerSocket=socket_create(AF_INET, SOCK_STREAM,SOL_TCP);
//Set the IP and Port for external connection monitoring. Set the IP field to 0, which means listening to the IPs of all interfaces
if(!socket_bind($ServerSocket,0,$ServerPort)) die("Cannot Bind Socket!n");
//Start listening to Port
if(!socket_listen($ServerSocket)) die("Cannot Listen!n");
//Set Socket to nonblock mode
if(!socket_set_nonblock($ServerSocket)) die("Cannot Set Server Socket to Block!n");
//Clear the $PID variable, indicating that there is currently no Child Process
unset($PID);
while(true)
{
//Enter pooling mode and check whether there is a connection coming in every 1 second.
sleep(1);
//Check if there is a connection coming in
@$ConnectedServerSocket=socket_accept($ServerSocket);
if($ConnectedServerSocket!==false)
{
//Someone is coming in
//Start a Child Process to handle connections
$PID=pcntl_fork();
if($PID==-1) die("could not fork");
if($PID) continue;//This is the daemon process, continue to monitor.
//Here is the start of Child Process
//Execute the function in Socket
ProcessSocket($ConnectedServerSocket);
//After processing the Socket, end the Socket
DestroySocket();
//End Child Process
exit(0);
}
}
I hope this article will be helpful to everyone’s PHP programming design.

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
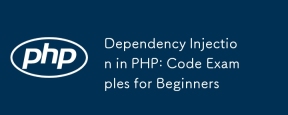
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
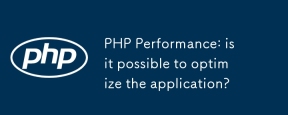
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
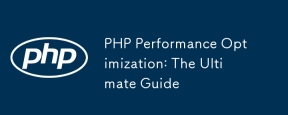
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
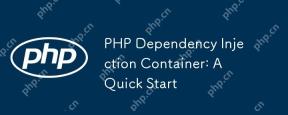
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
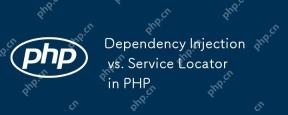
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
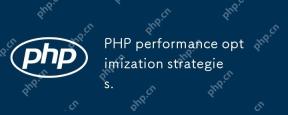
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
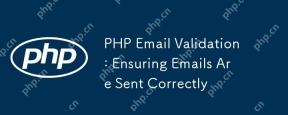
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
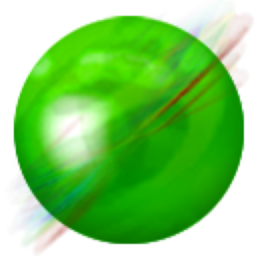
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
