


Summary of operations related to adding, modifying, and deleting data in yii database
This article summarizes the related operations of adding data, modifying data, and deleting data in yii. I have just learned a lot. Today, I only recorded a few, and will gradually flesh them out later. Friends in need can take a look.
How to add data
(1) save method (object form operation)
$user=new User;
$user->username='phpernote';
$user->password='123456';
if($user->save()>0){
echo 'Added successfully';
}else{
echo 'Add failed';
}
(2) insert method (array form operation)
Yii::app()->dbName->createCommand()->insert('tbl_user',
array(
'username'=>'phpernote',
'password'=>'123456'
)
);
(3) insert method (object form operation)
$user=new User();
$user->username='phpernote';
$user->password='123456';
if($user->insert()){
echo 'Added successfully';
}else{
echo 'Add failed';
}
How to modify data
(1)Post::model()->updateAll($attributes,$condition,$params);
$count=User::model()->updateAll(array('username'=>'phpernote','password'=>'123456'),'id=:id',array(':id' =>$id));
if($count>0){
echo 'Modification successful';
}else{
echo 'Modification failed';
}
(2) Post::model()->updateByPk($pk,$attributes,$condition,$params);
$count=User::model()->updateByPk(1,array('username'=>'admin','password'=>'123456'));
or
$count=User::model()->updateByPk(array(1,2),array('username'=>'admin','password'=>'123456'),'username= :name',array(':name'=>'admin'));
if($count>0){
echo 'Modification successful';
}else{
echo 'Modification failed';
}
$pk represents the primary key, which can be one or a set. $attributes represents the set of fields to be modified. $condition represents the condition. The value passed in by $params
(3) Post::model()->updateCounters($counters,$condition,$params);
$count =User::model()->updateCounters(array('status'=>1),'username=:name',array(':name'=>'admin'));
if($count>0){
echo 'Modification successful';
}else{
echo 'Modification failed';
}
array('status'=>1) represents the admin table in the database. According to the condition username='admin', the status field of all query results will automatically add 1
User::model()->updateCounters(array('count'=>1), 'id='.User::model()->id);//Automatically superimpose 1
User::model()->updateCounters(array('count'=>-1), 'id='.User::model()->id);//Automatically decrement by 1
(4) Yii::app()->dbName->createCommand()->update($attributes,$condition,$params);
Yii::app()->dbName->createCommand()->update('tbl_user',
array(
'username'=>'phpernote'
),
'id=:id',
array(
‘:id’=>3
)
);
How to delete data
(1) Post::model()->deleteAll($condition,$params);
For example:
$count=User::model()->deleteAll('username=:name AND password=:pass',array(':name'=>'phpernote',':pass'=>'123456') );
or:
$count=User::model()->deleteAll('id in("1,2,3")'); //Delete data with these ids
if($count>0){
echo 'Delete successfully';
}else{
echo 'Deletion failed';
}
(2) Post::model()->deleteByPk($pk,$condition,$params);
For example:
$count=User::model()->deleteByPk(1);
or:
$count=User::model()->deleteByPk(array(1,2),'username=:name',array(':name'=>'admin'));
if($count>0){
echo 'Delete successfully';
}else{
echo 'Deletion failed';
}
Articles you may be interested in
- The reasons why PHP adds backslashes before quotation marks and how to remove backslashes in PHP, three ways to close php magic quotes
- Mysql database cache cache function analysis, debugging and performance summary
- php mysql database operation class
- FckEditor adds right-click menu-picture deletion function
- Summary MySQL database server gradually slows down Reasons and solutions
- Mysql server master-slave database synchronization configuration
- php clears (delete) the files in the specified directory without deleting the directory folder
- php uses array_flip Implement array key-value exchange to remove array duplicate values
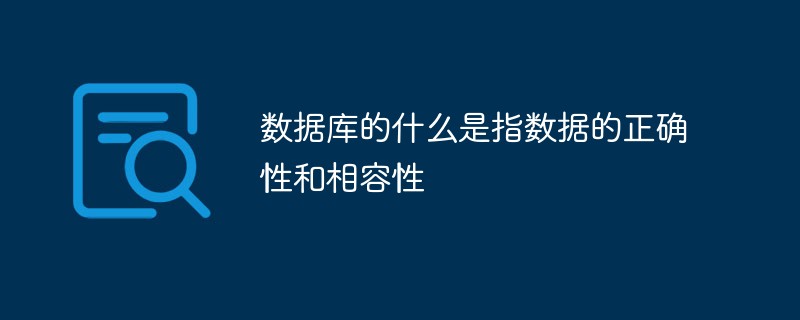
数据库的“完整性”是指数据的正确性和相容性。完整性是指数据库中数据在逻辑上的一致性、正确性、有效性和相容性。完整性对于数据库系统的重要性:1、数据库完整性约束能够防止合法用户使用数据库时向数据库中添加不合语义的数据;2、合理的数据库完整性设计,能够同时兼顾数据库的完整性和系统的效能;3、完善的数据库完整性有助于尽早发现应用软件的错误。
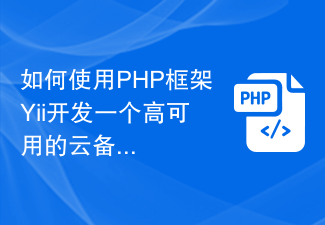
随着云计算技术的不断发展,数据的备份已经成为了每个企业必须要做的事情。在这样的背景下,开发一款高可用的云备份系统尤为重要。而PHP框架Yii是一款功能强大的框架,可以帮助开发者快速构建高性能的Web应用程序。下面将介绍如何使用Yii框架开发一款高可用的云备份系统。设计数据库模型在Yii框架中,数据库模型是非常重要的一部分。因为数据备份系统需要用到很多的表和关
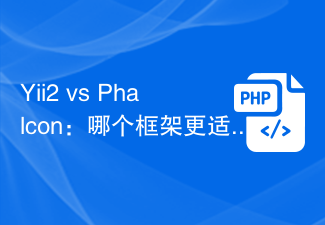
在当前信息时代,大数据、人工智能、云计算等技术已经成为了各大企业关注的热点。在这些技术中,显卡渲染技术作为一种高性能图形处理技术,受到了越来越多的关注。显卡渲染技术被广泛应用于游戏开发、影视特效、工程建模等领域。而对于开发者来说,选择一个适合自己项目的框架,是一个非常重要的决策。在当前的语言中,PHP是一种颇具活力的语言,一些优秀的PHP框架如Yii2、Ph
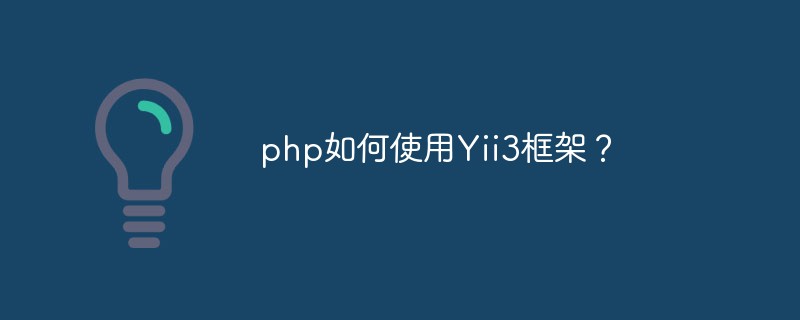
随着互联网的不断发展,Web应用程序开发的需求也越来越高。对于开发人员而言,开发应用程序需要一个稳定、高效、强大的框架,这样可以提高开发效率。Yii是一款领先的高性能PHP框架,它提供了丰富的特性和良好的性能。Yii3是Yii框架的下一代版本,它在Yii2的基础上进一步优化了性能和代码质量。在这篇文章中,我们将介绍如何使用Yii3框架来开发PHP应用程序。
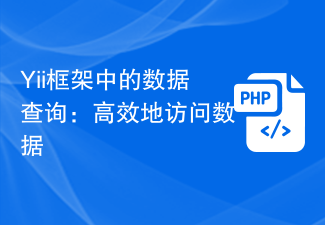
Yii框架是一个开源的PHPWeb应用程序框架,提供了众多的工具和组件,简化了Web应用程序开发的流程,其中数据查询是其中一个重要的组件之一。在Yii框架中,我们可以使用类似SQL的语法来访问数据库,从而高效地查询和操作数据。Yii框架的查询构建器主要包括以下几种类型:ActiveRecord查询、QueryBuilder查询、命令查询和原始SQL查询
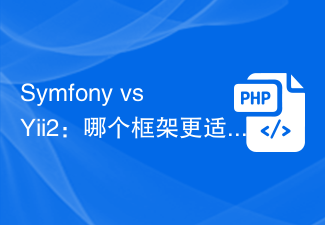
随着Web应用需求的不断增长,开发者们在选择开发框架方面也越来越有选择的余地。Symfony和Yii2是两个备受欢迎的PHP框架,它们都具有强大的功能和性能,但在面对需要开发大型Web应用时,哪个框架更适合呢?接下来我们将对Symphony和Yii2进行比较分析,以帮助你更好地进行选择。基本概述Symphony是一个由PHP编写的开源Web应用框架,它是建立
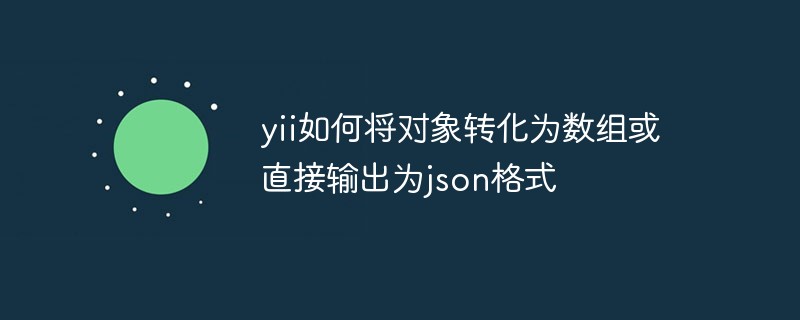
yii框架:本文为大家介绍了yii将对象转化为数组或直接输出为json格式的方法,具有一定的参考价值,希望能够帮助到大家。
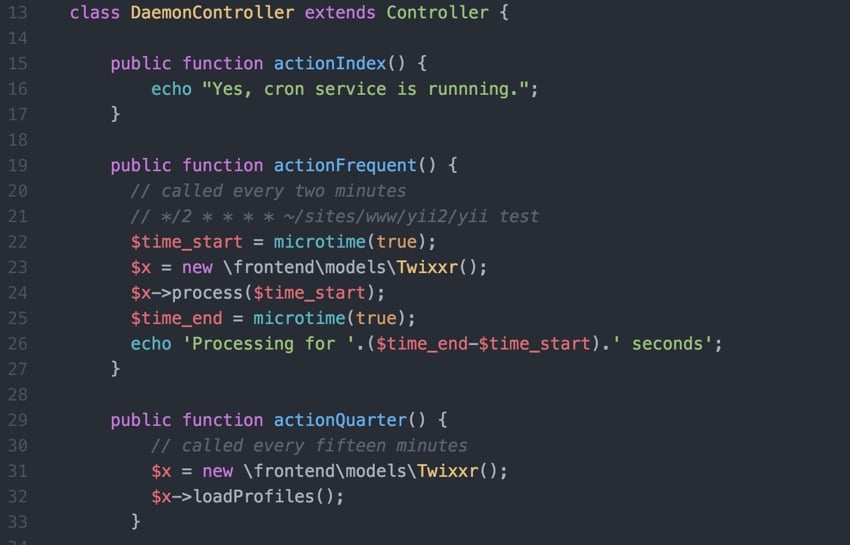
如果您问“Yii是什么?”查看我之前的教程:Yii框架简介,其中回顾了Yii的优点,并概述了2014年10月发布的Yii2.0的新增功能。嗯>在这个使用Yii2编程系列中,我将指导读者使用Yii2PHP框架。在今天的教程中,我将与您分享如何利用Yii的控制台功能来运行cron作业。过去,我在cron作业中使用了wget—可通过Web访问的URL来运行我的后台任务。这引发了安全问题并存在一些性能问题。虽然我在我们的启动系列安全性专题中讨论了一些减轻风险的方法,但我曾希望过渡到控制台驱动的命令


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
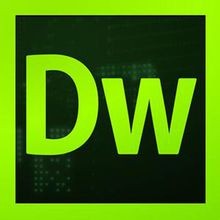
Dreamweaver CS6
Visual web development tools
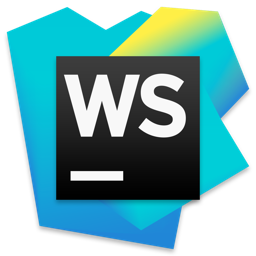
WebStorm Mac version
Useful JavaScript development tools