Summary of yii model layer operations_PHP tutorial
Yii model layer operation summary
Yii model layer operation attributes and methods summary.
tableName – Set the table name corresponding to the Model, for example:
public function tableName(){return 'gshop_order_ext';}
rules – Set validation rules for each field in the Model
relations – Set association rules
attributeLabels – Set aliases for each field
safeAttributes – Set fields that can modify attributes
beforeValidate and afterValidate – functions executed before and after field validation, which need to return a true value
beforeSave and afterSave – records the functions executed before and after storage, and needs to return a true value
Secondly, the ORM in Yii uses AR, and there are several main operations, namely:
save – operation data
update – modify data
delete – delete data
validate – Validate data
When reading records, there are several methods:
findByPk – Find a record by primary key, the result is a single record
findByAttribute – Find a record by attribute, the result is a single record
findAllByAttributes – Find data by attributes, the result is a recordset
findAll – Find data through CDbCriteria object, the result is a record set *
There are two types of parameters received by the search method. The one without an asterisk accepts an array as a parameter, and the one with an asterisk receives a CDbCriteria object as a parameter. When using an object, more search conditions can be provided. An example is given below. :
$criteria = new CDbCriteria; // Create CDbCriteria object
$criteria->condition = 'title LIKE %' . 'php' . '%'; // Set query conditions
$criteria->order = 'createdTime DESC'; // Set sorting criteria
$criteria->limit = 10; //Limit the number of records
$criteria->select = 'id,title,content'; // Set the fields included in the result
$articles = Article::model()->findAll($criteria); //The result is an array, each element of which is a record object
Again, Yii adopts the LazyLoad loading method for related data by default, that is, it reads it only when needed. In this way, when we do not need the related data, Yii will not help us read it, which greatly speeds up the response. Speed. But there are also times when we need to associate data. For example, when reading an article, we need the category to which the article belongs. If we use LazyLoad, how many articles will there be and how many times will be queried, which is very inefficient. , then EagerLoad is needed, that is, reading all the data in the related table at one time.
For example:
$articles = Article::model()->with('category')->findAll();
Use with to read all the data of the related table at once. The settings of the related table are set in the relationship in the Model.
For example:
public function relations() { return array( 'category' => array(self::BELONGS_TO, 'Category', 'categoryId'), ); }
Very clear and concise.
Articles you may be interested in
- Yii framework module development analysis
- Understanding of Yii framework Yiiapp()
- yii database addition , modification, deletion related operations summary
- yii database query operation summary
- Summary of common methods of Yii CDbCriteria
- Yii Get the current controller name and action name
- Yii uses PHPExcel to import Excel files
- Yii action method tips
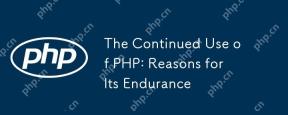
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
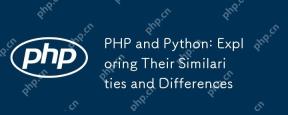
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
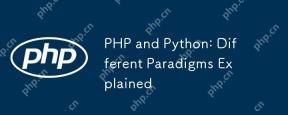
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
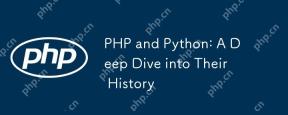
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
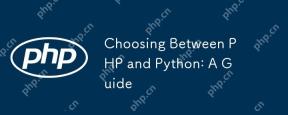
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
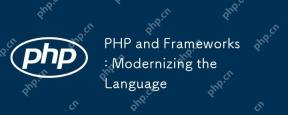
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
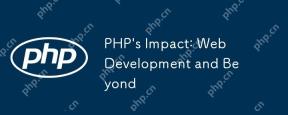
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
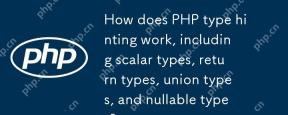
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
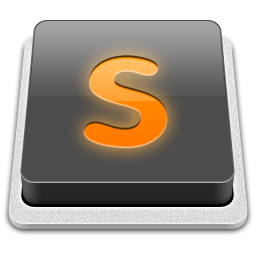
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor