Do you want to work on Monday -PHP MySQLi, -phpmysqli
hi
I was originally ambitious to work, but unexpectedly, something unexpected happened. It was cloudy in the morning and I couldn't get up. At noon, I went back to the dormitory to fiddle with clothes (I'm a female worker, so I'm not awesome) and didn't take a nap. I was in such a confused state. , how to do scientific research, just write this.
1. OOP programming in PHP
4.7 Polymorphism
--Definition
Since there are various method implementations of interfaces, this feature is called polymorphism
--Chestnut
function eat($obj){
if($obj instanceof ICanEat){
$obj->eat("FOOD"); // You don’t need to know whether it is Human or Animal, just eat it
}else{
echo "Can't eat!n";
}
}
$man = new Human();
$monkey = new Animal();
// The same code behaves differently when passing in different implementation classes of the interface. That's why it becomes polymorphic.
eat($man);
eat($monkey);
--Summary
/**
* Polymorphism
* 1. As long as an object implements the interface (instanceof), you can directly call the interface method on the object
*/
4.8 Abstract Class
--Question
Some methods of the classes connected to the interface are the same, so is it possible to allow them to be implemented in the interface instead of being implemented in the class?
For example, humans and animals eat different things but breathe the same.
--Chestnut
abstract class ACanEat{ //Keyword changes
abstract public function eat($food);//If the class needs to implement it by itself, add the abstract keyword in front of it
public function breath(){
echo "Breath use the air.
";
}
}
class Human extends ACanEat{ //implements are used to implement the interface, and extends
public function eat($food){
echo "Human eating ".$food."
";
}
}
class Animal extends ACanEat{ //implements are used to implement the interface, here extends
public function eat($food){
echo "Animal eating ". $food."
";
}
}
$xiaoming=new Human();
$xiaohei=new Animal();
$xiaoming->breath();$xiaoming->eat("food");
$xiaohei->breath();$xiaohei->eat("shit");
--Summary
/**
* Abstract class
* 1. Abstract classes allow some methods in the class to be temporarily not implemented concretely. We call these methods abstract methods
* 2. Once there are abstract methods in a class, the class must be abstract Class
* 3. Abstract classes, like interfaces, cannot be directly instantiated into objects
*/
5. Magic methods
5.1 Introduction
Note that all magic methods are preceded by two underscores__
Special to OOP in PHP.
Such as constructor and destructor.
5.2 __tostring() and __invoke()
--Definition
__tostring(), this method will be automatically called when the object is used as String; echo $obj;
__invoke(), when the object is called as a method (function), this method is automatically called; $obj(4);
--Chestnut
/*
* tostring() magic method
* invoke() magic method
*/
class MagicTest{
public function __toString(){
return "This is the class magictest.";
}
public function __invoke($x){
echo "".$x;
}
}
$obj=new MagicTest();
echo $obj;
$obj(5);
Usage is similar to constructor and destructor. More automated (automatically called, even if not declared), but at the same time more error-prone, be careful.
5.3 __call() and __callStatic() or overloading
--Definition
When the object accesses a method name that does not exist, __call() will be automatically called;
When the object accesses a non-existent static method name, __callStatic() will be automatically called;
These two methods are also called overloading (different from rewriting); through these two methods, calls with the same method name can be implemented corresponding to different methods
--Chestnut
/*
* tostring() magic method
* invoke() magic method
*/
class MagicTest{
public function __toString(){
return "This is the class magictest.";
}
public function __invoke($x){
echo "".$x."
";
}
public function __call($name,$arguments){ //The format of __call is fixed, first The first is the method name, and the second is the parameters within the method
echo "Calling ".$name." with parameters: ".implode(",", $arguments)."
";
}
public static function __callstatic($name,$arguments){
echo "Static calling ".$name." with parameters: ".implode("," , $arguments)."
";
}
}
$obj=new MagicTest();
echo $obj;
$obj(5);
$obj->runTest("para1","para2");
$obj::runTest("para3","para4");
Note that the format required when defining methods is fixed.
5.4 __get()__set()__isset()__unset
--Definition
These methods are also called the magic methods of Attribute overloading.
__set(), called when assigning a value to inaccessible attributes (one is that the attribute is undefined, the other is that there is no access permission, such as private) ;
__get(), called when reading the value of an inaccessible attribute;
__isset(), called when isset() or empty() is called on an inaccessible property;
__unset(),. . . . . . . . . unset(). . . . . . . . . .
--Chestnut
/*
* tostring() magic method
* invoke() magic method
*/
class MagicTest{
public function __toString(){
return "This is the class magictest.";
}
public function __invoke($x){
echo "".$x."
";
}
public function __call($name,$arguments){ //The format of __call is fixed, the first one is the method name, the second one is the parameter within the method
echo "Calling ".$name." with parameters: ".implode(",", $arguments)."
";
}
public static function __callstatic($name,$arguments){
echo "Static calling ".$name." with parameters: ".implode(",", $arguments)."
";
}
public function __get($name){ //get must have name
return "Getting the property ".$name."
" ;
}
public function __set($name,$value){ //set must have a name and value
echo "Setting the property ".$name." to value ". $value.".
";
}
public function __isset($name){ //Determine whether the attribute is defined
echo "__isset invoked
";
return true;
}
public function __unset($name){ //Undo
echo "unsetting protery ".$name."";
return true;
}
}
$obj=new MagicTest();
echo $obj;
$obj(5);
$obj->runTest("para1","para2");
$obj::runTest("para3","para4");
echo $obj->classname;
$obj->classname="shit";
echo isset($obj->classname)."
";
unset($obj->classname);echo "
";
echo empty($obj->classname)."
";
The result is
This is the class magictest.
5
Calling runTest with parameters: para1,para2
Static calling runTest with parameters: para3,para4
Getting the property classname
Setting the property classname to value shit.
__isset invoked
1
unsetting protery classname
__isset invoked
As you can see, actually isset and empty have opposite operations when calling __isset.
Then, __set($name, $value) and __unset($name) are a pair of opposite operations, but the required elements are different;
__isset($name), __get($name)all only require names (remember the function of each magic method, it will be easier to remember if you understand it).
5.5 __clone()
--Definition
It’s cloning, or cloning
--Chestnut
First give the usage of clone keyword.
/*
* Clone magic method
*/
class nbaPlayer{
public $name;
}
$james=new nbaPlayer();
$james->name='James';
echo $james->name."
";
$kobe=clone $james;
$kobe->name='Kobe';
echo $kobe->name;
After cloning, it is a separate object, and operations on it do not affect the original object.
Add __clone()
/*
* Clone magic method
*/
class nbaPlayer{
public $name;
public function __clone(){
$this->name="shit";
}
}
$james=new nbaPlayer();
$james->name='James';
echo $james->name."
";
$kobe=clone $james;
echo $kobe->name."
";
$kobe->name='Kobe';
echo $kobe->name."
";
Generally speaking, it is useful for initialization after cloning; or, concealing certain information that you don’t want to disclose after copying.
I often use this one in my work, because I often operate on an object, and I don’t want to affect the original data , so I just clone/copy it.
----------------------------------------
2. MySQLi extension
1. Installation and download
1.1 Advantages and Introduction
Updated and better, PHP5 and later are recommended (or PDO).
--Advantages
Based on OOP and process-oriented use;
Supports prepared statements;
Support transactions.
--Others
Faster. Better security
1.2 Installation and Configuration
--Install
Configure php and open php_mysqli.dll;
Configure extension_dir='ext directory location';
Restart the server.
(I use WAMP, just check the box)
--Verification
/*
* Verify whether mysqli is enabled
*/
//phpinfo();
//2. Check whether the extension has been loaded
var_dump(extension_loaded('mysqli'));
var_dump(extension_loaded('curl'));
echo '
';
//3. Check whether the function exists
var_dump(function_exists('mysqli_connect'));
echo '
';
//4. Get the currently enabled extensions
print_r(get_loaded_extensions());
echo '
';
---
I’m sleepy, go back and wash up and go to bed. . .

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
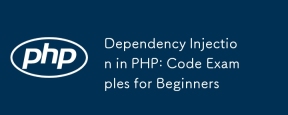
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
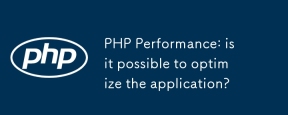
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
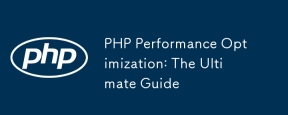
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
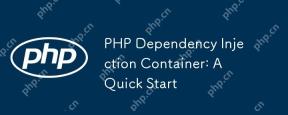
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
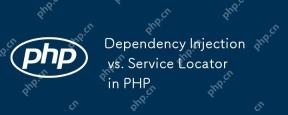
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
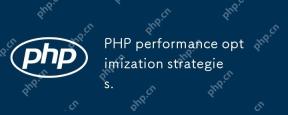
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
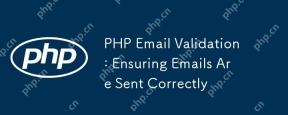
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
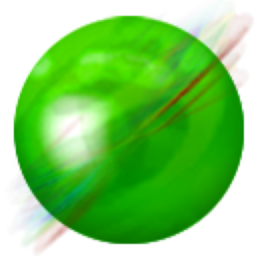
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
