


null coalescing operator in PHP, PHP null coalescing operator_PHP tutorial
null coalescing operator in PHP, PHP null coalescing operator
<code class="language-meta">project: blog target: null-coalesce-operator-in-php.md date: 2015-12-30 status: publish tags: - Null Coalesce - PHP categories: - PHP </code>
The null coalescing operator is a good thing. With it, we can easily get a parameter and provide a default value when it is empty. For example, you can use ||
in js:
<code class="language-js">function setSomething(a){ a = a || 'some-default-value'; // ... } </code>
In PHP, unfortunately, PHP's ||
always returns true
or false
, so it cannot be done this way.
PHP7 has only officially added the ??
operator:
<code class="language-php">// 获取user参数的值(如果为空,则用'nobody') $username = $_GET['user'] ?? 'nobody'; // 等价于: $username = isset($_GET['user']) ? $_GET['user'] : 'nobody'; </code>
It is estimated that PHP7 will take a long time to be used in the production environment. So are there any alternatives to the current PHP5?
According to research, there is a very convenient alternative:
<code class="language-php">// 获取user参数的值(如果为空,则用'nobody') $username = @$_GET['user'] ?: 'nobody'; // 等价于: $username = isset($_GET['user']) ? $_GET['user'] : 'nobody'; </code>
-- Run this code: https://3v4l.org/aDUW8
I looked at it with my eyes wide open. It was similar to the previous PHP7 example, mainly replacing ??
with ?:
. What the hell is this? In fact, this is the omission pattern of the (expr1) ? (expr2) : (expr3)
expression:
<p>表达式 (expr1) ? (expr2) : (expr3) 在 expr1 求值为 TRUE 时的值为 expr2,在 expr1 求值为 FALSE 时的值为 expr3。<br/> 自 PHP 5.3 起,可以省略三元运算符中间那部分。表达式 expr1 ?: expr3 在 expr1 求值为 TRUE 时返回 expr1,否则返回 expr3。<br/> -- http://php.net/manual/zh/language.operators.comparison.php</p>
Of course, this alternative is not perfect - if there is no $_GET
in 'user'
, there will be an Notice: Undefined index: user
error, so you need to use @
to suppress this error, or turn off E_NOTICE
mistake.
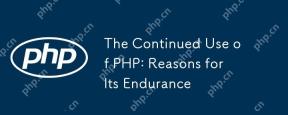
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
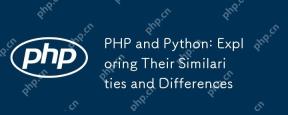
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
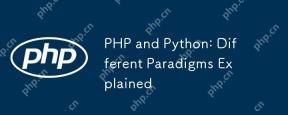
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
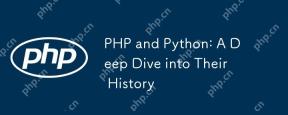
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
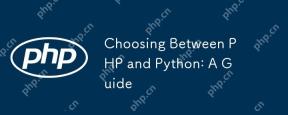
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
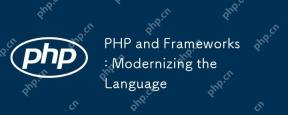
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
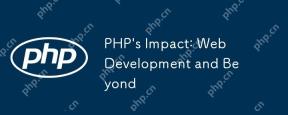
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
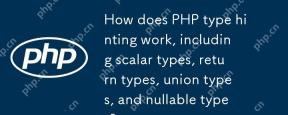
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
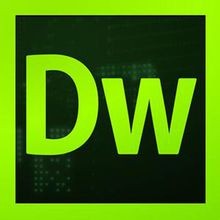
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor