lesson15-QT multi-threading
1. What is a thread1. Thread
Process: an executing program, which is the smallest unit of resource allocation
thread : The smallest unit of program execution
The process has many disadvantages. First, because the process is the resource owner, there is a large time and space overhead in creation, undoing and switching, so it is necessary to introduce lightweight processes; second, because of the symmetric multi-process The emergence of processors (SMP) can satisfy multiple running units, but the parallel overhead of multiple processes is too large.
2. The terminology of threads
Concurrency means that at the same time, only one instruction can be executed, but multiple process instructions are executed in rapid rotation, which has the effect of multiple processes executing at the same time from a macro perspective. .
Appears to happen at the same time
Parallelism means that multiple instructions are executed simultaneously on multiple processors at the same time.
True simultaneous occurrence
Synchronization: Calls that are dependent on each other should not "happen at the same time", and synchronization is to prevent those things that "happen at the same time"
The concept of asynchronous is opposite to synchronization. Any two operations that are independent of each other are asynchronous, which indicates that things happen independently
3. Advantages of threads
1), parallelism in developing programs on multi-processors
2), While waiting for slow IO operations, the program can perform other operations to improve concurrency
3). Modular programming can more clearly express the relationship between independent events in the program, with a clear structure
4) and occupy a larger area. Fewer system resources
Multi-threading does not necessarily require multi-processors
Multi-threading technology is often used in GUI programs. One thread is used to respond to the interface, while other threads can handle lengthy operations in the background
Qt’s meta-object system supports communication between objects in different threads using the signal and slot mechanism
2. QT multi-threading
Using multi-threading in Qt is very simple Yes, as long as you subclass QThread, there is a protected type run function in QThread. Multi-threading can be achieved by rewriting the run function.
1. QT threads
Using multi-threading in Qt is very simple. Just subclass QThread and then rewrite the run function to achieve multi-threading
class MyThread:public Thread
{
public:
MyThread();
protected:
void run();
private:
volatile boolean stopped;
}
run function It is started through the start method of the thread. The thread also has the isRunning method to determine whether it is running. The terminate method ends the thread
2. Thread synchronization semaphore
The semaphore prevents the thread from being busy. Waiting is an extension of mutex. Using a semaphore can ensure that two critical codes will not be concurrent. When entering a critical section of code, the thread must obtain the semaphore and release it when exiting. Semaphores can be accessed by multiple threads at the same time.
Qt’s semaphore QSemaphore class:
acquire() is used to acquire resources, free() is used to release resources
Examples of producers and consumers, when producers produce It is necessary to ensure that there is enough space. When consumers consume, they must ensure that there are resources in the space
QSemaphore freeByte(100) The producer has 100 spaces
QSemaphore useByte(0) The consumer has no resources
producer
{
freeByte.acquire()
byte = n
useByte.release()
}
consumer
{
useByte.acquire()
printf byte
freeByte.release()
}
3. Condition variables for thread synchronization
QWaitCondition allows threads to wake up other threads under certain conditions, so that threads do not have to be busy. To wait, the condition variable must be used with the mutex
QMutex mutex; QWaitCondition condition;
condition.wait(&mutex)
condition.wakeAll()
wait function unlocks the mutex , and wait here, the mutex will be re-locked before this function returns.
The wakeAll function will wake up all threads waiting for the mutex
4. Thread priority
The actual task may let a certain thread run first, so you need to set the thread priority class.
The setPriority function can set the priority of the thread, or pass in the priority of the thread in the start function when the thread is started
3. Examples
1. Multi-threading
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>#ifndef MYTHREAD_H<br /> </li><li>#define MYTHREAD_H<br /></li><li><br /></li><li>#include <QThread><br /></li><li><br /></li><li>class MyThread : public QThread<br /></li><li>{<br /></li><li>Q_OBJECT<br /></li><li>public:<br /></li><li>MyThread();<br /></li><li>void stop();<br /></li><li>volatile bool stopped;<br /></li><li>protected:<br /></li><li>void run();<br /></li><li>};<br /></li><li>#endif</li></ol>
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>#include "myThread.h"<br /> </li><li>#include <QtDebug><br /></li><li><br /></li><li>MyThread::MyThread()<br /></li><li>{<br /></li><li>stopped = false;<br /></li><li>}<br /></li><li><br /></li><li>void MyThread::run()<br /></li><li>{<br /></li><li>int i=0;<br /></li><li>while(!stopped)<br /></li><li>{<br /></li><li>qDebug()<<"thread id:"<QThread::currentThreadId()<<":"<<i;<br /></li><li>i++;<br /></li><li>sleep(2);<br /></li><li>}<br /></li><li>stopped = false;<br /></li><li>}<br /></li><li><br /></li><li>void MyThread::stop()<br /></li><li>{<br /></li><li>stopped = true;<br /></li><li>} </li></ol>
2. Semaphore
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>#ifndef PRODUCER_H<br /> </li><li>#define PRODUCER_H<br /></li><li><br /></li><li>#include <QThread><br /></li><li><br /></li><li>class Producer : public QThread<br /></li><li>{<br /></li><li>Q_OBJECT<br /></li><li>public:<br /></li><li>Producer();<br /></li><li>protected:<br /></li><li>void run();<br /></li><li>};<br /></li><li><br /></li><li>#endif</li></ol>
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>#ifndef CONSUMER_H<br /> </li><li>#define CONSUMER_H<br /></li><li><br /></li><li>#include <QThread><br /></li><li><br /></li><li>class Consumer : public QThread<br /></li><li>{<br /></li><li>Q_OBJECT<br /></li><li>public:<br /></li><li>Consumer();<br /></li><li>protected:<br /></li><li>void run();<br /></li><li>};<br /></li><li><br /></li><li>#endif</li></ol>
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>#include "producer.h"<br /> </li><li>#include "consumer.h"<br /></li><li>#include <QDebug><br /></li><li>#include <QSemaphore><br /></li><li><br /></li><li>#define SIZE 50<br /></li><li>QSemaphore freeByte(SIZE);<br /></li><li>QSemaphore useByte(0);<br /></li><li><br /></li><li>Producer::Producer()<br /></li><li>{<br /></li><li><br /></li><li>}<br /></li><li>void Producer::run()<br /></li><li>{<br /></li><li>for(int i=0; i<SIZE; i++)<br /></li><li>{<br /></li><li>freeByte.acquire();<br /></li><li>qDebug()<<"produer:"<<i;<br /></li><li>useByte.release();<br /></li><li>sleep(1);<br /></li><li>}<br /></li><li>}<br /></li><li>Consumer::Consumer()<br /></li><li>{<br /></li><li><br /></li><li>}<br /></li><li>void Consumer::run()<br /></li><li>{<br /></li><li>for(int i=0; i<SIZE; i++)<br /></li><li>{<br /></li><li>useByte.acquire();<br /></li><li>qDebug()<<"consumer:"<<i;<br /></li><li>freeByte.release();<br /></li><li>sleep(2);<br /></li><li>}<br /></li><li>} </li></ol>
3. Condition variable
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>#ifndef THREAD_H<br /> </li><li>#define THREAD_H<br /></li><li><br /></li><li>#include<QThread><br /></li><li><br /></li><li>class Producer : public QThread<br /></li><li>{<br /></li><li>Q_OBJECT<br /></li><li>public:<br /></li><li>Producer();<br /></li><li>protected:<br /></li><li>void run();<br /></li><li>};<br /></li><li><br /></li><li>class Consumer : public QThread<br /></li><li>{<br /></li><li>Q_OBJECT<br /></li><li>public:<br /></li><li>Consumer();<br /></li><li>protected:<br /></li><li>void run();<br /></li><li>};<br /></li><li><br /></li><li>#endif</li></ol>
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>#include "thread.h"<br /> </li><li>#include <QDebug><br /></li><li>#include <QMutex><br /></li><li>#include <QWaitCondition><br /></li><li><br /></li><li>QMutex mutex;<br /></li><li>QWaitCondition empty, full;<br /></li><li>int num=0;<br /></li><li>int buffer[50];<br /></li><li>int useByte=0;<br /></li><li><br /></li><li><br /></li><li>Producer::Producer()<br /></li><li>{<br /></li><li><br /></li><li>}<br /></li><li>void Producer::run()<br /></li><li>{<br /></li><li>for(int i=0; i<50; i++)<br /></li><li>{<br /></li><li>mutex.lock();<br /></li><li>if(useByte==50)<br /></li><li>empty.wait(&mutex);<br /></li><li>num++;<br /></li><li>buffer[i] = num;<br /></li><li>qDebug()<<"producer:"<<num;<br /></li><li>useByte++;<br /></li><li>full.wakeAll();<br /></li><li>mutex.unlock();<br /></li><li>sleep(1);<br /></li><li>}<br /></li><li>}<br /></li><li>Consumer::Consumer()<br /></li><li>{<br /></li><li><br /></li><li>}<br /></li><li>void Consumer::run()<br /></li><li>{<br /></li><li>for(int i=0; i<50; i++)<br /></li><li>{<br /></li><li>mutex.lock();<br /></li><li>if(useByte==0)<br /></li><li>full.wait(&mutex);<br /></li><li>qDebug()<<"consumer"<<buffer[i];<br /></li><li>useByte--;<br /></li><li>empty.wakeAll();<br /></li><li>mutex.unlock();<br /></li><li>sleep(2);<br /></li><li>}<br /></li><li>} </li></ol>
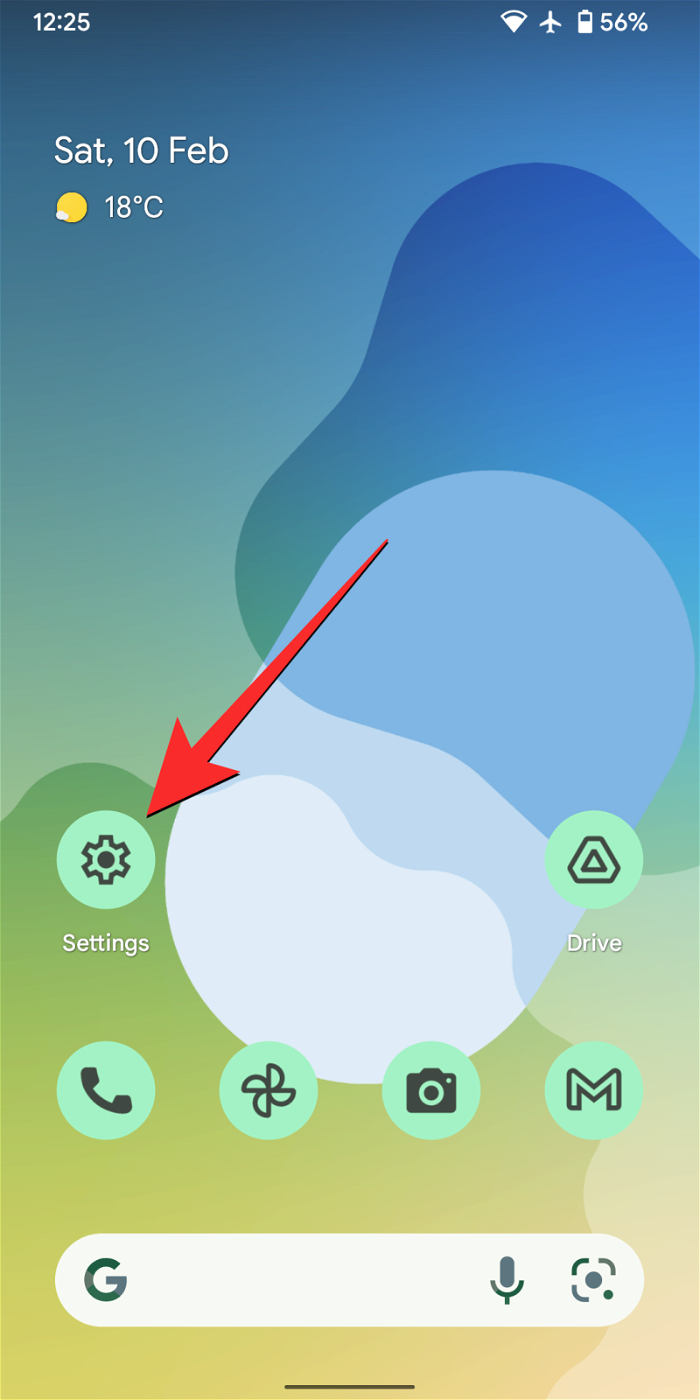
根据美国司法部的解释,蓝色警报旨在提供关于可能对执法人员构成直接和紧急威胁的个人的重要信息。这种警报的目的是及时通知公众,并让他们了解与这些罪犯相关的潜在危险。通过这种主动的方式,蓝色警报有助于增强社区的安全意识,促使人们采取必要的预防措施以保护自己和周围的人。这种警报系统的建立旨在提高对潜在威胁的警觉性,并加强执法机构与公众之间的沟通,以共尽管这些紧急通知对我们社会至关重要,但有时可能会对日常生活造成干扰,尤其是在午夜或重要活动时收到通知时。为了确保安全,我们建议您保持这些通知功能开启,但如果
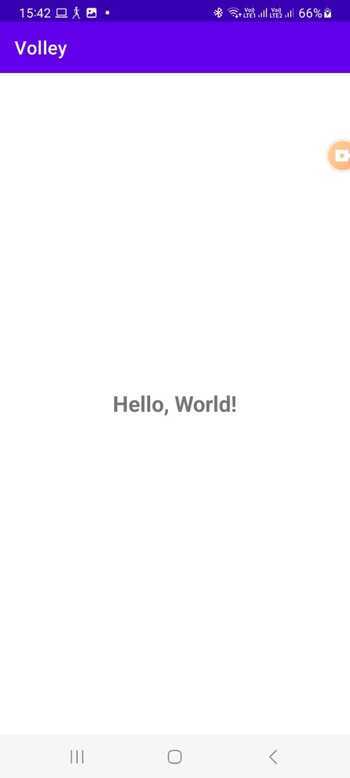
Android中的轮询是一项关键技术,它允许应用程序定期从服务器或数据源检索和更新信息。通过实施轮询,开发人员可以确保实时数据同步并向用户提供最新的内容。它涉及定期向服务器或数据源发送请求并获取最新信息。Android提供了定时器、线程、后台服务等多种机制来高效地完成轮询。这使开发人员能够设计与远程数据源保持同步的响应式动态应用程序。本文探讨了如何在Android中实现轮询。它涵盖了实现此功能所涉及的关键注意事项和步骤。轮询定期检查更新并从服务器或源检索数据的过程在Android中称为轮询。通过
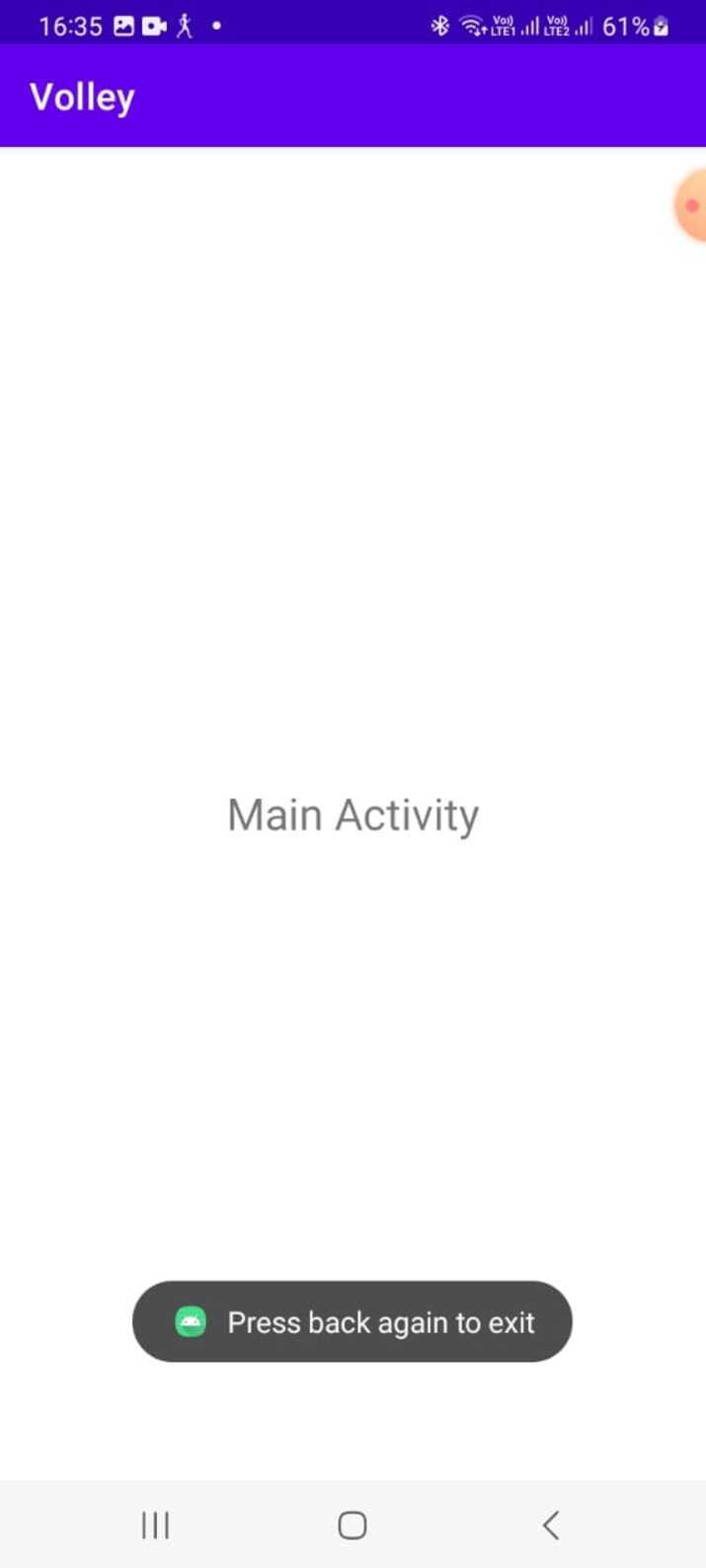
为了提升用户体验并防止数据或进度丢失,Android应用程序开发者必须避免意外退出。他们可以通过加入“再次按返回退出”功能来实现这一点,该功能要求用户在特定时间内连续按两次返回按钮才能退出应用程序。这种实现显著提升了用户参与度和满意度,确保他们不会意外丢失任何重要信息Thisguideexaminesthepracticalstepstoadd"PressBackAgaintoExit"capabilityinAndroid.Itpresentsasystematicguid
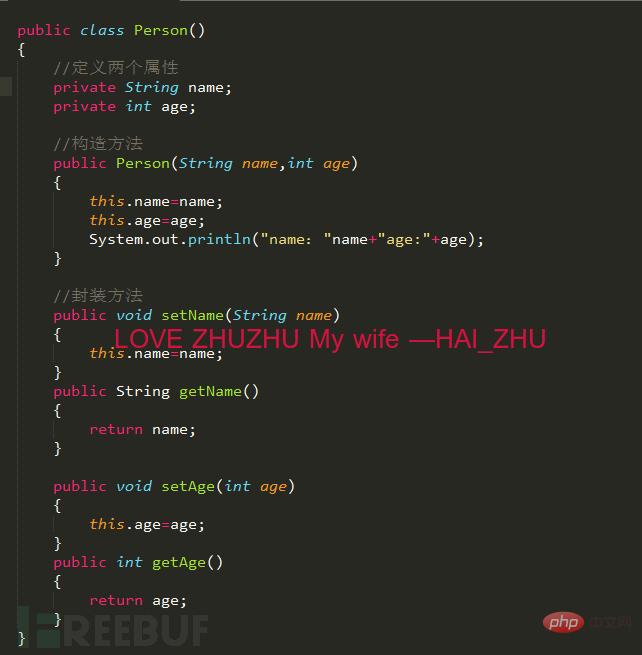
1.java复杂类如果有什么地方不懂,请看:JAVA总纲或者构造方法这里贴代码,很简单没有难度。2.smali代码我们要把java代码转为smali代码,可以参考java转smali我们还是分模块来看。2.1第一个模块——信息模块这个模块就是基本信息,说明了类名等,知道就好对分析帮助不大。2.2第二个模块——构造方法我们来一句一句解析,如果有之前解析重复的地方就不再重复了。但是会提供链接。.methodpublicconstructor(Ljava/lang/String;I)V这一句话分为.m
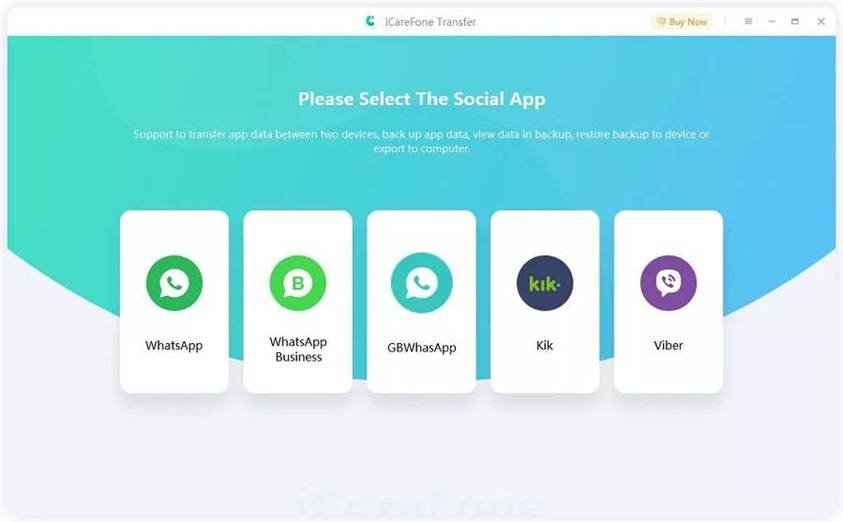
如何将WhatsApp聊天从Android转移到iPhone?你已经拿到了新的iPhone15,并且你正在从Android跳跃?如果是这种情况,您可能还对将WhatsApp从Android转移到iPhone感到好奇。但是,老实说,这有点棘手,因为Android和iPhone的操作系统不兼容。但不要失去希望。这不是什么不可能完成的任务。让我们在本文中讨论几种将WhatsApp从Android转移到iPhone15的方法。因此,坚持到最后以彻底学习解决方案。如何在不删除数据的情况下将WhatsApp
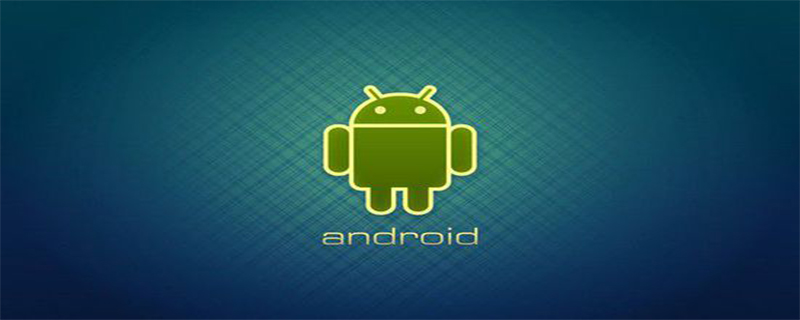
原因:1、安卓系统上设置了一个JAVA虚拟机来支持Java应用程序的运行,而这种虚拟机对硬件的消耗是非常大的;2、手机生产厂商对安卓系统的定制与开发,增加了安卓系统的负担,拖慢其运行速度影响其流畅性;3、应用软件太臃肿,同质化严重,在一定程度上拖慢安卓手机的运行速度。
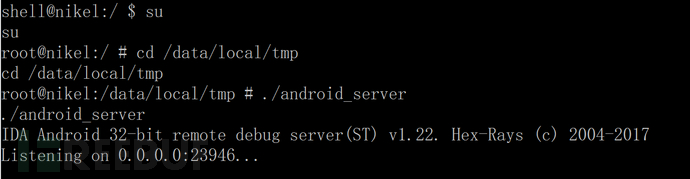
1.启动ida端口监听1.1启动Android_server服务1.2端口转发1.3软件进入调试模式2.ida下断2.1attach附加进程2.2断三项2.3选择进程2.4打开Modules搜索artPS:小知识Android4.4版本之前系统函数在libdvm.soAndroid5.0之后系统函数在libart.so2.5打开Openmemory()函数在libart.so中搜索Openmemory函数并且跟进去。PS:小知识一般来说,系统dex都会在这个函数中进行加载,但是会出现一个问题,后
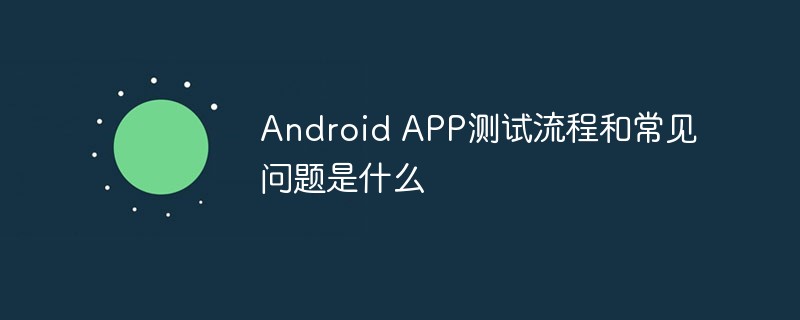
1.自动化测试自动化测试主要包括几个部分,UI功能的自动化测试、接口的自动化测试、其他专项的自动化测试。1.1UI功能自动化测试UI功能的自动化测试,也就是大家常说的自动化测试,主要是基于UI界面进行的自动化测试,通过脚本实现UI功能的点击,替代人工进行自动化测试。这个测试的优势在于对高度重复的界面特性功能测试的测试人力进行有效的释放,利用脚本的执行,实现功能的快速高效回归。但这种测试的不足之处也是显而易见的,主要包括维护成本高,易发生误判,兼容性不足等。因为是基于界面操作,界面的稳定程度便成了


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
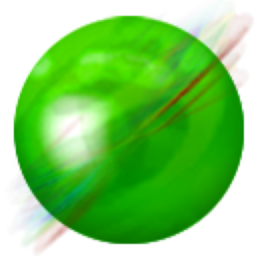
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
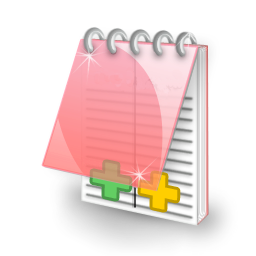
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
