


How to automatically submit forms in PHP (based on fsockopen and curl), fsockopencurl_PHP tutorial
How to automatically submit forms in PHP (based on fsockopen and curl), fsockopencurl
This article describes the method of automatically submitting forms in PHP based on fsockopen and curl. Share it with everyone for your reference, the details are as follows:
Both fsockopen and curl can do PHP automatic form submission
1. fsockopen method:
php code:
<?php /*----------------------------------------------------------- *功能:使用PHP socke 向指定页面提交数据 *作者:果冻 说明:post($url, $data) * * $url = 'http://www.xxx.com:8080/login.php'; * $data[user] = 'hong'; * $data[pass] = 'xowldo'; * echo post($url, $data); *-----------------------------------------------------------*/ function post($url, $data) { $url = parse_url($url); if (!$url) return "couldn't parse url"; if (!isset($url['port'])) { $url['port'] = ""; } if (!isset($url['query'])) { $url['query'] = ""; } $encoded = ""; while (list($k,$v) = each($data)) { $encoded .= ($encoded ? "&" : ""); $encoded .= rawurlencode($k)."=".rawurlencode($v); } $fp = fsockopen($url['host'], $url['port'] ? $url['port'] : 80); if (!$fp) return "Failed to open socket to $url[host]"; fputs($fp, sprintf("POST %s%s%s HTTP/1.0n", $url['path'], $url['query'] ? "?" : "", $url['query'])); fputs($fp, "Host: $url[host]n"); fputs($fp, "Content-type: application/x-www-form-urlencodedn"); fputs($fp, "Content-length: " . strlen($encoded) . "n"); fputs($fp, "Connection: closenn"); fputs($fp, "$encodedn"); $line = fgets($fp,1024); if (!eregi("^HTTP/1.. 200", $line)) return; $results = ""; $inheader = 1; while(!feof($fp)) { $line = fgets($fp,1024); if ($inheader && ($line == "n" || $line == "rn")) { $inheader = 0; } elseif (!$inheader) { $results .= $line; } } fclose($fp); return $results; } /* $url = 'http://video.xxx.com:80/game_vm.php'; $data['gid'] = '1'; echo post($url, $data); */ ?>
2. Curl method:
php code:
<?php $url = 'http://localhost/curl/result.php'; $params = "param=123¶m2=333"; //What will be posted $user_agent = "Mozilla/5.0 (compatible; MSIE 5.01; Windows NT 5.0)"; $ch = curl_init(); curl_setopt($ch, CURLOPT_POST,1); curl_setopt($ch, CURLOPT_POSTFIELDS,$params); curl_setopt($ch, CURLOPT_URL,$url); curl_setopt($ch, CURLOPT_USERAGENT, $user_agent); curl_setopt($ch, CURLOPT_RETURNTRANSFER,1); $result=curl_exec ($ch); //execut curl_close ($ch); echo "Results: <br>".$result; ?>
result.php (just for test)
<?php print_r($_POST); ?>
Readers who are interested in more PHP related content can check out the special topics of this site: "php socket usage summary", "php curl usage summary", "PHP array (Array) operation skills", "PHP data structure and algorithm" Tutorial", "Summary of PHP Mathematical Operation Skills", "Summary of PHP Date and Time Usage", "Introduction Tutorial on PHP Object-Oriented Programming", "Summary of PHP String Usage", "Introduction Tutorial on PHP MySQL Database Operation" and "Summary of Common Database Operation Skills in PHP"
I hope this article will be helpful to everyone in PHP programming.
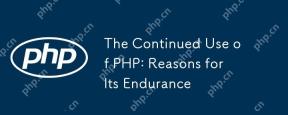
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
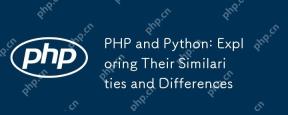
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
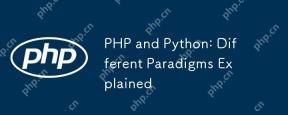
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
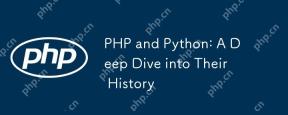
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
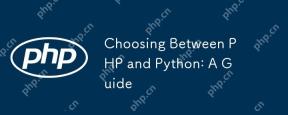
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
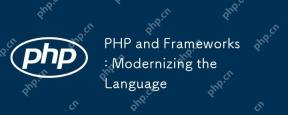
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
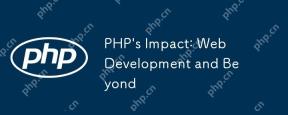
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
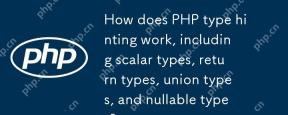
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
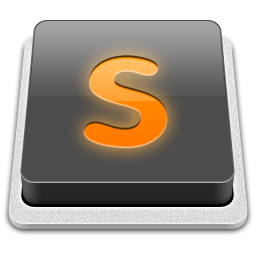
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor