


[PHP source code reading] array_slice and array_splice functions, slicesplice_PHP tutorial
[PHP source code reading]array_slice and array_splice functions, slicesplice
array_slice and array_splice functions are used to take out a slice of the array, array_splice also replaces the original deleted slice position with a new slice function. Similar to the Array.prototype.splice and Array.prototype.slice methods in javascript.
I have more detailed annotations on the PHP source code on github. If you are interested, you can take a look and give it a star. PHP5.4 source code annotations. You can view the added annotations through the commit record.
array_slice
<p>array array_slice ( array $array , int $offset [, int $length = NULL [, bool $preserve_keys = false ]] )</p>
Returns the subarray slice of the specified subscript offset and length in the array.
Parameter description
Suppose the length of the first parameter array is num_in.
offset
If offset is a positive number and less than length, the returned array will start from offset; if offset is greater than length, no operation will be performed and it will be returned directly. If offset is a negative number, offset = num_in offset, if num_in offset == 0, offset is set to 0.
length
If length is less than 0, then length will be converted to num_in - offset length; otherwise, if offset length > array_count, then length = num_in - offset. If length is still less than 0 after processing, it will be returned directly.
preserve_keys
The default is false. The original order of numeric key values is not retained by default. If set to true, the original numeric key value order of the array will be retained.
Usage examples
<?<span>php </span><span>$input</span> = <span>array</span>("a", "b", "c", "d", "e"<span>); </span><span>$output</span> = <span>array_slice</span>(<span>$input</span>, 2); <span>//</span><span> returns "c", "d", and "e"</span> <span>$output</span> = <span>array_slice</span>(<span>$input</span>, -2, 1); <span>//</span><span> returns "d"</span> <span>$output</span> = <span>array_slice</span>(<span>$input</span>, 0, 3); <span>//</span><span> returns "a", "b", and "c"</span> <span>print_r</span>(<span>array_slice</span>(<span>$input</span>, 2, -1)); <span>//</span><span> array(0 => 'c', 1 => 'd');</span> <span>print_r</span>(<span>array_slice</span>(<span>$input</span>, 2, -1, <span>true</span>)); <span>//</span><span> array(2 => 'c', 1 => 'd');</span>
Run steps
<p>处理参数:offset、length</p> <p>移动指针到offset指向的位置</p> <p>从offset开始,拷贝length个元素到返回数组</p>
The operation flow chart is as follows
php
$input = array("red", "green", "blue", "yellow");
array_splice($input, 2);
// $input becomes array("red", "green")
$input = array("red", "green", "blue", "yellow");
array_splice($input, 1, -1);
// $input becomes array("red", "yellow")
$input = array("red", "green", "blue", "yellow");
array_splice($input, 1, count($input), "orange");
// $input becomes array("red", "orange")
$input = array("red", "green", "blue", "yellow");
array_splice($input, -1, 1, array("black", "maroon"));
// $input is array("red", "green",
// "blue", "black", "maroon")
$input = array("red", "green", "blue", "yellow");
array_splice($input, 3, 0, "purple");
// $input is array("red", "green",
// "blue", "purple", "yellow");
Source code interpretation
In array_splice, there is this piece of code:
<span>/*</span><span> Don't create the array of removed elements if it's not going * to be used; e.g. only removing and/or replacing elements </span><span>*/</span> <span>if</span> (return_value_used) { <span>//</span><span> 如果有用到函数返回值则创建返回数组,否则不创建返回数组</span> <span>int</span> size =<span> length; </span><span>/*</span><span> Clamp the offset.. </span><span>*/</span> <span>if</span> (offset ><span> num_in) { offset </span>=<span> num_in; } </span><span>else</span> <span>if</span> (offset < <span>0</span> && (offset = (num_in + offset)) < <span>0</span><span>) { offset </span>= <span>0</span><span>; } </span><span>/*</span><span> ..and the length </span><span>*/</span> <span>if</span> (length < <span>0</span><span>) { size </span>= num_in - offset +<span> length; } </span><span>else</span> <span>if</span> (((unsigned <span>long</span>) offset + (unsigned <span>long</span>) length) ><span> (unsigned) num_in) { size </span>= num_in -<span> offset; } </span><span>/*</span><span> Initialize return value </span><span>*/</span><span> array_init_size(return_value, size </span>> <span>0</span> ? size : <span>0</span><span>); rem_hash </span>= &<span>Z_ARRVAL_P(return_value); }</span>
The array_splice function returns the deleted slice. The meaning of this code is that if array_splice needs to return a value, then create the return array, otherwise do not create it to avoid wasting space. This is also a little programming trick, return only when needed. For example, if $result = array_splice(...) is used in a function, return_value_used is true.
Summary
This is the end of this article. In daily programming, you should deal with the most special situations first, and then continue, just like you did when implementing these two functions, to avoid making redundant judgments; save new variables if necessary Only apply for new space when the time comes, otherwise it will cause waste.
Original article with limited writing style and limited knowledge. If there is anything wrong in the article, please let me know.
If this article is helpful to you, please click to recommend it, thank you^_^
Finally, I have more detailed annotations on the PHP source code on github. If you are interested, you can take a look and give it a star. PHP5.4 source code annotations. You can view the added annotations through the commit record.
For more source code articles, please visit your personal homepage to continue viewing: hoohack
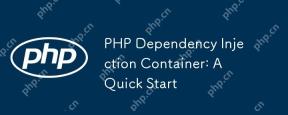
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
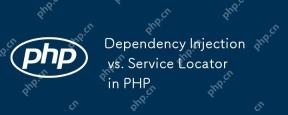
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
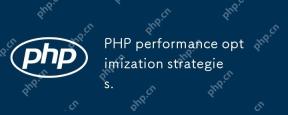
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
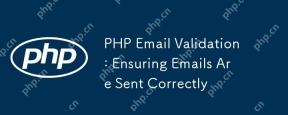
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
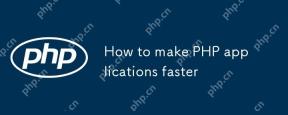
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
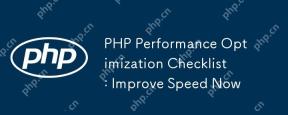
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
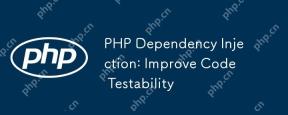
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
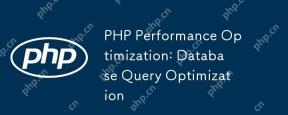
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
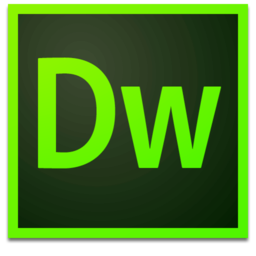
Dreamweaver Mac version
Visual web development tools
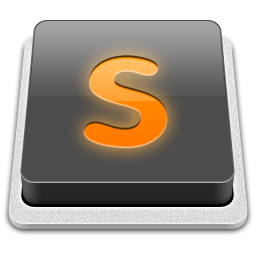
SublimeText3 Mac version
God-level code editing software (SublimeText3)
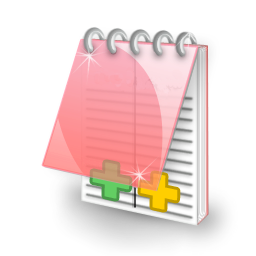
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
