


array('a1','b2','c3','d4','e5','x1','y1','z1');
For example, the above array
I want different combinations of the three elements in this array element, for example:
array('a1','b2','c3');
array('a1','b2', 'd4');
array('a1','b2','e5');
array('a1','b2','x1');
array('a1', 'b2','y1');
....
And finally return such an array
array(
<code>array('a1','b2','c3'), array('a1','b2','d4'), array('a1','b2','e5'), array('a1','b2','x1'), array('a1','b2','y1'), ......</code>
)
Reply content:
array('a1','b2','c3','d4','e5','x1','y1','z1');
For example, the above array
I want different combinations of the three elements in this array element, for example:
array('a1','b2','c3');
array('a1','b2', 'd4');
array('a1','b2','e5');
array('a1','b2','x1');
array('a1', 'b2','y1');
....
And finally return such an array
array(
<code>array('a1','b2','c3'), array('a1','b2','d4'), array('a1','b2','e5'), array('a1','b2','x1'), array('a1','b2','y1'), ......</code>
)
Three-layer foreach solution
<code>$data = array('a1','b2','c3','d4','e5','x1','y1','z1'); foreach ($data as $k_1 => $v_1) { foreach ($data as $k_2 => $v_2) { foreach ($data as $k_3 => $v_3) { if ($v_1 !== $v_2 && $v_1 !== $v_3 && $v_2 !== $v_3) { var_dump([$v_1,$v_2,$v_3]); } } } }</code>
As for permutations and combinations, the indexes of three numbers have sizes after all. Arrange them from small to large.
Set them as a b c d, and then (this is a math problem)
Thanks for the invitation. If you haven’t learned math well, go check out the data sorting/merging function usage in the manual
<code><?php $arr = array('a','b','c','d'); $result = array(); $t = getCombinationToString($arr, 3); print_r($t); function getCombinationToString($arr, $m) { if ($m ==1) { return $arr; } $result = array(); $tmpArr = $arr; unset($tmpArr[0]); for($i=0;$i<count($arr);$i++) { $s = $arr[$i]; $ret = getCombinationToString(array_values($tmpArr), ($m-1)); foreach($ret as $row) { $result[] = $s . $row; } } return $result; } ?> </code>
Array
(
<code>[0] => abc [1] => abd [2] => acc [3] => acd [4] => adc [5] => add [6] => bbc [7] => bbd [8] => bcc [9] => bcd [10] => bdc [11] => bdd [12] => cbc [13] => cbd [14] => ccc [15] => ccd [16] => cdc [17] => cdd [18] => dbc [19] => dbd [20] => dcc [21] => dcd [22] => ddc [23] => ddd</code>
)
Give you an idea
Are array('a1','b1','c1') and array('c1','a1','b1') considered duplicates?
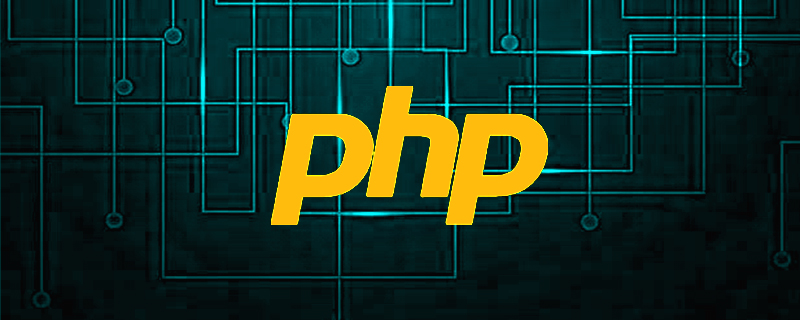
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
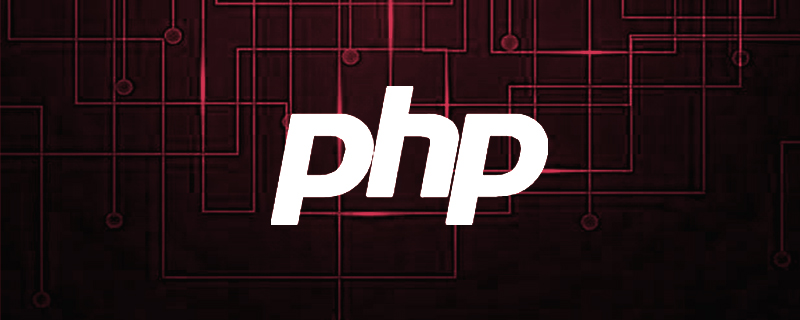
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
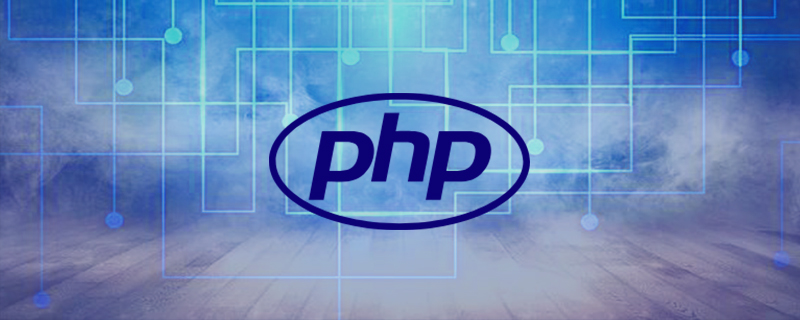
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
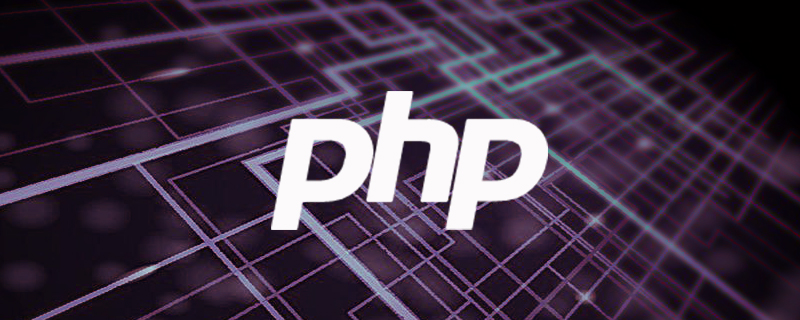
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
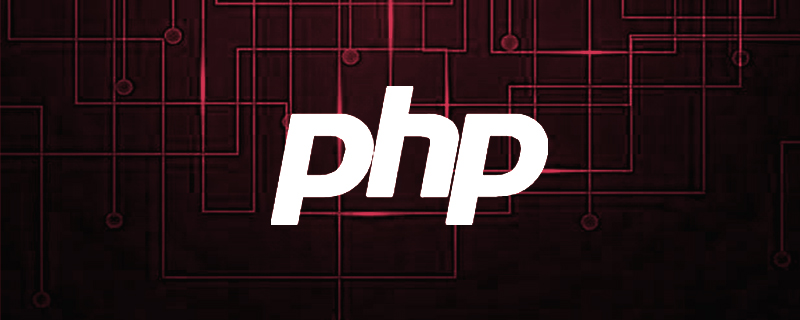
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
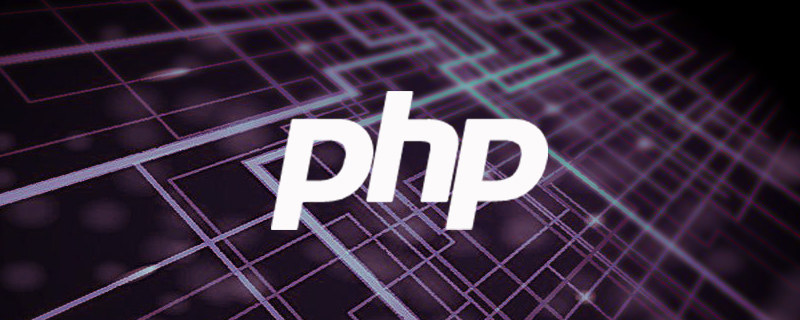
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
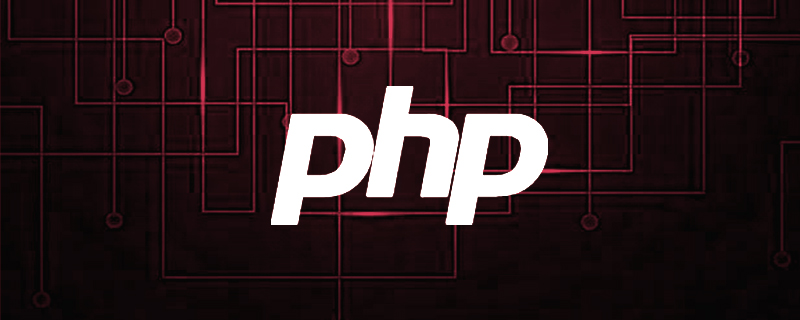
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
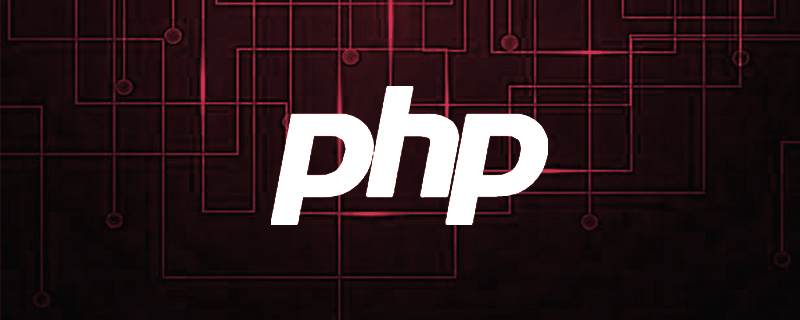
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
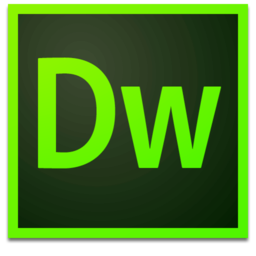
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
