


Ajax_upload upload progress bar code implemented by PHP apc ajax_php skills
The example in this article describes the ajax_upload upload progress bar code implemented by PHP apc ajax. Share it with everyone for your reference, the details are as follows:
How is the upload progress bar implemented? What is the principle? When we browse and select upload, a temporary file will be generated. When uploading, upload this temporary file to the server. After the upload is completed, this Temporary files will be deleted. If we can read the size of this temporary file, we will know the upload progress. The php apc module can implement this function.
1. Install apc module
Download address: http://pecl.php.net/package/apc
tar zxvf APC-3.1.8.tgz cd APC-3.1.8/ /usr/local/php/bin/phpize ./configure --with-php-config=/usr/local/php/bin/php-config make && make install
2. Modify php.ini
extension = apc.so apc.rfc1867 = 1 apc.max_file_size = 200M upload_max_filesize = 1000M post_max_size = 1000M max_execution_time = 600 max_input_time = 600 memory_limit = 128M
After modification, restart apache or other to check
[root@BlackGhost php]# php -m [PHP Modules] apc cgi-fcgi ctype curl date dom eAccelerator 。。。。。。。。
3. upload_test.php
<?php $id = uniqid(rand(), true); ?> <html> <script type='text/javascript' src='jquery-1.3.2.js'></script> <script type='text/javascript' src='ajaxupload.3.1.js'></script> <script type='text/javascript' src='upload.js'></script> <body style="text-align:center;"> <h1 id="上传测试">上传测试</h1><form enctype="multipart/form-data" id="upload" method="POST"> <input type="hidden" name="APC_UPLOAD_PROGRESS" id="progress_key" value="<?=$id?>" /> <input type="file" id="file" name="file" value=""/><br/><input id="submit" type="submit" value="Upload!" /> </form> <div id="progressouter" style="width: 500px; height: 20px; border: 1px solid black; display:none;"> <div id="progressinner" style="position: relative; height: 20px; background-color: red; width: 0%; "> </div> </div> <br /> <div id='showNum'></div><br> <div id='showInfo'></div><br> </body> </html> <script type="text/javascript"> $(document).ready(function(){ form_submit(); }); </script>
What is the use of APC_UPLOAD_PROGRESS? It adds a tag to the uploaded file, and you can use this tag to access it in other PHP programs. Provide support for apc reading.
js file uploaded asynchronously by upload.js:
function form_submit (){ new AjaxUpload('#upload', { action: 'upload.php', name: 'file', data: { APC_UPLOAD_PROGRESS:$("#progress_key").val() }, autoSubmit: true, onSubmit: function(file, extension){ $('#progressouter').css('display', 'block'); progress(); }, onComplete: function(file, response){ $("#showInfo").html(response); } }); } function progress (){ $.ajax({ type: "GET", url: "progress.php?progress_key="+$("#progress_key").val(), dataType: "json", cache:false, success: function(data){ if(data == 0) { var precent = 0; } else { for (i in data) { if (i == "current") { var json_current = parseInt(data[i]); } if (i == "total") { var json_total = parseInt(data[i]); } } var precent = parseInt(json_current/json_total * 100); $("#progressinner").css("width",precent+"%"); $("#showNum").html(precent+"%"); $("#showInfo").html("ok"); } if ( precent < 100) { setTimeout("progress()", 100); } } }); }
One thing to note above is APC_UPLOAD_PROGRESS:$("#progress_key").val(). Here, the key is APC_UPLOAD_PROGRESS. If it is not this, apc cannot find the temporary file. Why should I use ajax_upload.js here? Because the ajax that comes with jquery does not have parameters for uploading files, that is, the content in type='file' cannot be obtained at the PHP end.
4. upload.php upload file
<?php if($_SERVER['REQUEST_METHOD'] == 'POST') { if(empty($_FILES["file"]["tmp_name"])){ echo "no file"; die; } $tmp_name = $_FILES["file"]["tmp_name"]; $name = dirname($_SERVER['SCRIPT_FILENAME'])."/upload/".$_FILES["file"]["name"]; move_uploaded_file($tmp_name, $name); echo "<p>File uploaded.</p>"; } ?>
If the file is relatively large, do not use http to upload it. It is too slow and affects the stability of the website.
5. progress.php is the progress file, used for ajax calls
<?php if(isset($_GET['progress_key'])) { $status = apc_fetch('upload_'.$_GET['progress_key']); if($status['total']!=0 && !empty($status['total'])) { echo json_encode($status); } else { echo 0; } } ?>
Look at the data generated by ajax asynchronous request.
php apc ajax upload progress bar
Parameter description:
total file size
current Uploaded size
filename Upload file name
name Tag name
done Successful upload is 1
cancel_upload The user cancels the upload, which will only happen when the upload is completed
rate upload speed, only available when the upload is completed
start_time Start time
Readers who are interested in more content related to PHP files and ajax operations can check out the special topics of this site: "php file operation summary" and "PHP ajax skills and application summary"
I hope this article will be helpful to everyone in PHP programming.
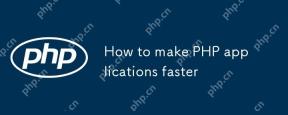
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
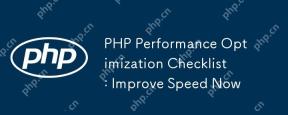
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
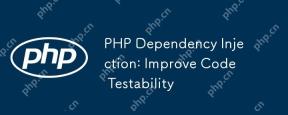
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
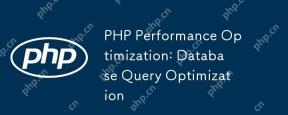
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
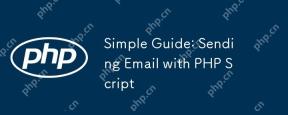
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
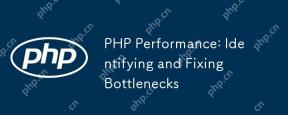
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
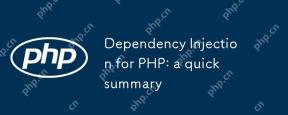
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
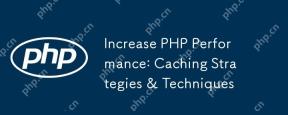
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
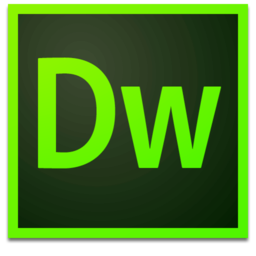
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!
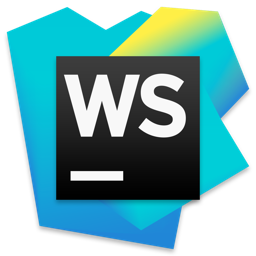
WebStorm Mac version
Useful JavaScript development tools
